Wallaroo Observability
- 1: Wallaroo Edge Computer Vision Observability
- 2: Wallaroo Model Observability: Anomaly Detection with CCFraud
- 3: Wallaroo Model Observability: Anomaly Detection with House Price Prediction
- 4: Wallaroo Edge Observability with Classification Financial Models
- 5: Wallaroo Assays Model Insights Tutorial
- 6: Computer Vision Pipeline Logs MLOps API Tutorial
- 7: Wallaroo Edge Observability with Classification Financial Models through API
- 8: Pipeline Logs MLOps API Tutorial
- 9: Pipeline Logs Tutorial
- 10: Wallaroo Edge Observability with Wallaroo Assays
- 11: House Price Testing Life Cycle
1 - Wallaroo Edge Computer Vision Observability
This tutorial and the assets can be downloaded as part of the Wallaroo Tutorials repository.
Computer Vision for Object Detection in Retail
The following tutorial demonstrates using Wallaroo for observability of edge deployments of computer vision models.
Introduction
This tutorial focuses on the resnet50 computer vision model. By default, this provides the following outputs from receiving an image converted to tensor values:
boxes
: The bounding boxes for detected objects.classes
: The class of the detected object (bottle, coat, person, etc).confidences
: The confidence the model has that the detected model is the class.
For this demonstration, the model is modified with Wallaroo Bring Your Own Predict (BYOP) to add two additional fields:
avg_px_intensity
: the average pixel intensity checks the input to determine the average value of the Red/Green/Blue pixel values. This is used as a benchmark to determine if the two images are significantly different. For example, an all white photo would have anavg_px_intensity
of1
, while an all blank photo would have a value of0
.avg_confidence
: The average confidence of all detected objects.
This demonstration will use avg_confidence
to demonstrate observability in our edge deployed computer vision model.
Prerequisites
- A Wallaroo Ops instance 2023.4 and above with [edge deployment enabled](Edge Deployment Registry Guide).
- An x64 edge device with Docker installed, recommended with at least 8 cores.
In order for the wallaroo tutorial notebooks to run properly, the videos directory must contain these models in the models directory.
To download the Wallaroo Computer Vision models, use the following link:
https://storage.googleapis.com/wallaroo-public-data/cv-demo-models/cv-retail-models.zip
Unzip the contents into the directory models
.
The following models are required to run the tutorial:
onnx==1.12.0
onnxruntime==1.12.1
torchvision
torch
matplotlib==3.5.0
opencv-python
imutils
pytz
ipywidgets
To run this tutorial outside of a Wallaroo Ops center, the Wallaroo SDK is available and is installed via pip
with:
pip install wallaroo==2023.4.1
References
Steps
Import Libraries
The following libraries are used to execute this tutorial. The utils.py
provides additional helper methods for rendering the images into tensor fields and other useful tasks.
# preload needed libraries
import wallaroo
from wallaroo.object import EntityNotFoundError
from wallaroo.framework import Framework
from IPython.display import display
from IPython.display import Image
import pandas as pd
import json
import datetime
import time
import cv2
import matplotlib.pyplot as plt
import string
import random
import pyarrow as pa
import sys
import asyncio
import numpy as np
import utils
pd.set_option('display.max_colwidth', None)
import datetime
# api based inference request
import requests
Connect to the Wallaroo Instance
The first step is to connect to Wallaroo through the Wallaroo client. The Python library is included in the Wallaroo install and available through the Jupyter Hub interface provided with your Wallaroo environment.
This is accomplished using the wallaroo.Client()
command, which provides a URL to grant the SDK permission to your specific Wallaroo environment. When displayed, enter the URL into a browser and confirm permissions. Store the connection into a variable that can be referenced later.
If logging into the Wallaroo instance through the internal JupyterHub service, use wl = wallaroo.Client()
. For more information on Wallaroo Client settings, see the Client Connection guide.
The option request_timeout
provides additional time for the Wallaroo model upload process to complete.
wl = wallaroo.Client(request_timeout=600)
Create Workspace
We will create a workspace to manage our pipeline and models. The following variables will set the name of our sample workspace then set it as the current workspace.
Workspace names must be unique. The following helper function will either create a new workspace, or retrieve an existing one with the same name. Verify that a pre-existing workspace has been shared with the targeted user.
Set the variables workspace_name
to ensure a unique workspace name if required.
The workspace will then be set as the Current Workspace. Model uploads and
def get_workspace(name, client):
workspace = None
for ws in client.list_workspaces():
if ws.name() == name:
workspace= ws
if(workspace == None):
workspace = client.create_workspace(name)
return workspace
workspace_name = "cv-retail-edge-observability"
model_name = "resnet-with-intensity"
model_file_name = "./models/model-with-pixel-intensity.zip"
pipeline_name = "retail-inv-tracker-edge-obs"
workspace = get_workspace(workspace_name, wl)
wl.set_current_workspace(workspace)
{'name': 'cv-retail-edge-observability', 'id': 8, 'archived': False, 'created_by': 'cc3619bb-2cec-4a44-9333-55a0dc6b3997', 'created_at': '2024-01-10T17:37:38.659309+00:00', 'models': [], 'pipelines': []}
Upload Model
The model is uploaded as a BYOP model, where the model, Python script and other artifacts are included in a .zip file. This requires the input and output schemas for the model specified in Apache Arrow Schema format.
input_schema = pa.schema([
pa.field('tensor', pa.list_(
pa.list_(
pa.list_(
pa.float32(), # images are normalized
list_size=640
),
list_size=480
),
list_size=3
)),
])
output_schema = pa.schema([
pa.field('boxes', pa.list_(pa.list_(pa.float32(), list_size=4))),
pa.field('classes', pa.list_(pa.int64())),
pa.field('confidences', pa.list_(pa.float32())),
pa.field('avg_px_intensity', pa.list_(pa.float32())),
pa.field('avg_confidence', pa.list_(pa.float32())),
])
model = wl.upload_model(model_name,
model_file_name,
framework=Framework.CUSTOM,
input_schema=input_schema,
output_schema=output_schema)
Waiting for model loading - this will take up to 10.0min.
Model is pending loading to a container runtime......
Model is attempting loading to a container runtime.............successful
Ready
Deploy Pipeline
Next we configure the hardware we want to use for deployment. If we plan on eventually deploying to edge, this is a good way to simulate edge hardware conditions. The BYOP model is deployed as a Wallaroo Containerized Runtime, so the hardware allocation is performed through the sidekick
options.
deployment_config = wallaroo.DeploymentConfigBuilder() \
.replica_count(1) \
.cpus(1) \
.memory("2Gi") \
.sidekick_cpus(model, 1) \
.sidekick_memory(model, '6Gi') \
.build()
We create the pipeline with the wallaroo.client.build_pipeline
method, and assign our model as a model pipeline step. Once complete, we will deploy the pipeline to allocate resources from the Kuberntes cluster hosting the Wallaroo Ops to the pipeline.
pipeline = wl.build_pipeline(pipeline_name)
pipeline.clear()
pipeline.add_model_step(model)
pipeline.deploy(deployment_config = deployment_config)
name | retail-inv-tracker-edge-obs |
---|---|
created | 2024-01-10 17:50:59.035145+00:00 |
last_updated | 2024-01-10 17:50:59.700103+00:00 |
deployed | True |
arch | None |
tags | |
versions | d0c2ed1c-3691-49f1-8fc8-e6510e4c39f8, 773099d3-6d64-4a92-b5a7-f614a916965d |
steps | resnet-with-intensity |
published | False |
Monitoring for Model Drift
For this example, we want to track the average confidence of object predictions and get alerted if we see a drop in confidence.
We will convert a set of images to pandas DataFrames with the images converted to tensor values. The first set of images baseline_images
as well formed images. The second set blurred_images
are the same photos intentionally blurred for our observability demonstration.
baseline_images = [
"./data/images/input/example/dairy_bottles.png",
"./data/images/input/example/dairy_products.png",
"./data/images/input/example/product_cheeses.png"
]
blurred_images = [
"./data/images/input/example/blurred-dairy_bottles.png",
"./data/images/input/example/blurred-dairy_products.png",
"./data/images/input/example/blurred-product_cheeses.png"
]
baseline_images_list = utils.processImages(baseline_images)
blurred_images_list = utils.processImages(blurred_images)
The Wallaroo SDK is capable of using numpy arrays in a pandas DataFrame for inference requests. Our demonstration will focus on using API calls for inference requests, so we will flatten the numpy array and use that value for our inference inputs. The following examples show using a baseline and blurred image for inference requests and the sample outputs.
# baseline image
df_test = pd.DataFrame({'tensor': wallaroo.utils.flatten_np_array_columns(baseline_images_list[0], 'tensor')})
df_test
tensor | |
---|---|
0 | [0.9372549, 0.9529412, 0.9490196, 0.94509804, 0.94509804, 0.94509804, 0.94509804, 0.94509804, 0.94509804, 0.94509804, 0.94509804, 0.94509804, 0.94509804, 0.94509804, 0.94509804, 0.94509804, 0.94509804, 0.94509804, 0.9490196, 0.9490196, 0.9529412, 0.9529412, 0.9490196, 0.9607843, 0.96862745, 0.9647059, 0.96862745, 0.9647059, 0.95686275, 0.9607843, 0.9647059, 0.9647059, 0.9607843, 0.9647059, 0.972549, 0.95686275, 0.9607843, 0.91764706, 0.95686275, 0.91764706, 0.8784314, 0.89411765, 0.84313726, 0.8784314, 0.8627451, 0.8509804, 0.9254902, 0.84705883, 0.96862745, 0.89411765, 0.81960785, 0.8509804, 0.92941177, 0.8666667, 0.8784314, 0.8666667, 0.9647059, 0.9764706, 0.98039216, 0.9764706, 0.972549, 0.972549, 0.972549, 0.972549, 0.972549, 0.972549, 0.98039216, 0.89411765, 0.48235294, 0.4627451, 0.43137255, 0.27058825, 0.25882354, 0.29411766, 0.34509805, 0.36862746, 0.4117647, 0.45490196, 0.4862745, 0.5254902, 0.56078434, 0.6039216, 0.64705884, 0.6862745, 0.72156864, 0.74509805, 0.7490196, 0.7882353, 0.8666667, 0.98039216, 0.9882353, 0.96862745, 0.9647059, 0.96862745, 0.972549, 0.9647059, 0.9607843, 0.9607843, 0.9607843, 0.9607843, ...] |
result = pipeline.infer(df_test,
dataset=['time', 'out.avg_confidence','out.avg_px_intensity','out.boxes','out.classes','out.confidences','check_failures','metadata'])
result
time | out.avg_confidence | out.avg_px_intensity | out.boxes | out.classes | out.confidences | metadata.partition | |
---|---|---|---|---|---|---|---|
0 | 2024-01-10 17:53:50.655 | [0.35880384] | [0.425382] | [[2.1511102, 193.98323, 76.26535, 475.40292], [610.82245, 98.606316, 639.8868, 232.27054], [544.2867, 98.726524, 581.28845, 230.20497], [454.99344, 113.08567, 484.78464, 210.1282], [502.58884, 331.87665, 551.2269, 476.49182], [538.54254, 292.1205, 587.46545, 468.1288], [578.5417, 99.70756, 617.2247, 233.57082], [548.552, 191.84564, 577.30585, 238.47737], [459.83328, 344.29712, 505.42633, 456.7118], [483.47168, 110.56585, 514.0936, 205.00156], [262.1222, 190.36658, 323.4903, 405.20584], [511.6675, 104.53834, 547.01715, 228.23663], [75.39197, 205.62312, 168.49893, 453.44086], [362.50656, 173.16858, 398.66956, 371.8243], [490.42468, 337.627, 534.1234, 461.0242], [351.3856, 169.14897, 390.7583, 244.06992], [525.19824, 291.73895, 570.5553, 417.6439], [563.4224, 285.3889, 609.30853, 452.25943], [425.57935, 366.24915, 480.63535, 474.54], [154.538, 198.0377, 227.64284, 439.84412], [597.02893, 273.60458, 637.2067, 439.03214], [473.88763, 293.41992, 519.7537, 349.2304], [262.77597, 192.03581, 313.3096, 258.3466], [521.1493, 152.89026, 534.8596, 246.52365], [389.89633, 178.07867, 431.87555, 360.59323], [215.99901, 179.52965, 280.2847, 421.9092], [523.6454, 310.7387, 560.36487, 473.57968], [151.7131, 191.41077, 228.71013, 443.32187], [0.50784916, 14.856098, 504.5198, 405.7277], [443.83676, 340.1249, 532.83734, 475.77713], [472.37848, 329.13092, 494.03647, 352.5906], [572.41455, 286.26132, 601.8677, 384.5899], [532.7721, 189.89102, 551.9026, 241.76051], [564.0309, 105.75121, 597.0351, 225.32579], [551.25854, 287.16034, 590.9206, 405.71548], [70.46805, 0.39822236, 92.786545, 84.40113], [349.44534, 3.6184297, 392.61484, 98.43363], [64.40484, 215.14934, 104.09457, 436.50797], [615.1218, 269.46683, 633.30853, 306.03452], [238.31851, 0.73957217, 290.2898, 91.30623], [449.37347, 337.39554, 480.13208, 369.35126], [74.95623, 191.84235, 164.21284, 457.00143], [391.96646, 6.255016, 429.23056, 100.72327], [597.48663, 276.6981, 618.0615, 298.62778], [384.51172, 171.95828, 407.01276, 205.28722], [341.5734, 179.8058, 365.88345, 208.57889], [555.0278, 288.62695, 582.6162, 358.0912], [615.92035, 264.9265, 632.3316, 280.2552], [297.95154, 0.5227982, 347.18744, 95.13106], [311.64868, 203.67934, 369.6169, 392.58063], [163.10356, 0.0, 227.67468, 86.49685], [68.518974, 1.870926, 161.25877, 82.89816], [593.6093, 103.263596, 617.124, 200.96545], [263.3115, 200.12204, 275.699, 234.26517], [592.2285, 279.66064, 619.70496, 379.14764], [597.7549, 269.6543, 618.5473, 286.25214], [478.04306, 204.36165, 530.00745, 239.35196], [501.34528, 289.28003, 525.766, 333.00262], [462.4777, 336.92056, 491.32016, 358.18915], [254.40384, 203.89566, 273.66177, 237.13142], [307.96042, 154.70946, 440.4545, 386.88065], [195.53915, 187.13596, 367.6518, 404.91095], [77.52113, 2.9323518, 160.15236, 81.59642], [577.648, 283.4004, 601.4359, 307.41882], [516.63873, 129.74504, 540.40936, 242.17572], [543.2536, 253.38689, 631.3576, 466.62567], [271.1358, 45.97066, 640.0, 456.88235], [568.77203, 188.66449, 595.866, 235.05397], [400.997, 169.88531, 419.35645, 185.80077], [473.9581, 328.07736, 493.2316, 339.41437], [602.9851, 376.39505, 633.74243, 438.61758], [480.95963, 117.62996, 525.08826, 242.74872], [215.71178, 194.87447, 276.59906, 414.3832], [462.2966, 331.94638, 493.14175, 347.97647], [543.94977, 196.90623, 556.04315, 238.99141], [386.8205, 446.6134, 428.81064, 480.00003], [152.22516, 0.0, 226.98564, 85.44817], [616.91815, 246.15547, 630.8656, 273.48447], [576.33356, 100.67465, 601.9629, 183.61014], [260.99304, 202.73479, 319.40524, 304.172], [333.5814, 169.75925, 390.9659, 278.09406], [286.26767, 3.700516, 319.3962, 88.77158], [532.5545, 209.36183, 552.58417, 240.08965], [572.99915, 207.65208, 595.62415, 235.46635], [6.2744417, 0.5994095, 96.04993, 81.27411], [203.32298, 188.94266, 227.27435, 254.29417], [513.7689, 154.48575, 531.3786, 245.21616], [547.53815, 369.9145, 583.13916, 470.30206], [316.64532, 252.80664, 336.65454, 272.82834], [234.79965, 0.0003570557, 298.05707, 92.014496], [523.0663, 195.29694, 534.485, 242.23671], [467.7621, 202.35623, 536.2362, 245.53342], [146.07208, 0.9752747, 178.93895, 83.210175], [18.780663, 1.0261598, 249.98721, 88.49467], [600.9064, 270.41983, 625.3632, 297.94083], [556.40955, 287.84702, 580.2647, 322.64728], [80.6706, 161.22787, 638.6261, 480.00003], [455.98315, 334.6629, 480.16724, 349.97784], [613.9905, 267.98065, 635.95807, 375.1775], [345.75964, 2.459102, 395.91183, 99.68698]] | [44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 44, 86, 82, 44, 44, 44, 44, 44, 44, 84, 84, 44, 44, 44, 44, 86, 84, 44, 44, 44, 44, 44, 84, 44, 44, 84, 44, 44, 44, 44, 51, 44, 44, 44, 44, 44, 44, 44, 44, 44, 82, 44, 44, 44, 44, 44, 86, 44, 44, 1, 84, 44, 44, 44, 44, 84, 47, 47, 84, 14, 44, 44, 53, 84, 47, 47, 44, 84, 44, 44, 82, 44, 44, 44] | [0.9965358, 0.9883404, 0.9700247, 0.9696426, 0.96478045, 0.96037567, 0.9542889, 0.9467539, 0.946524, 0.94484967, 0.93611854, 0.91653466, 0.9133634, 0.8874814, 0.84405905, 0.825526, 0.82326967, 0.81740034, 0.7956525, 0.78669065, 0.7731486, 0.75193685, 0.7360918, 0.7009186, 0.6932351, 0.65077204, 0.63243586, 0.57877576, 0.5023476, 0.50163734, 0.44628552, 0.42804396, 0.4253787, 0.39086252, 0.36836442, 0.3473236, 0.32950658, 0.3105372, 0.29076362, 0.28558296, 0.26680034, 0.26302803, 0.25444376, 0.24568668, 0.2353662, 0.23321979, 0.22612995, 0.22483191, 0.22332378, 0.21442991, 0.20122288, 0.19754867, 0.19439234, 0.19083925, 0.1871393, 0.17646024, 0.16628945, 0.16326219, 0.14825206, 0.13694529, 0.12920643, 0.12815322, 0.122357555, 0.121289656, 0.116281696, 0.11498632, 0.111848116, 0.11016138, 0.1095062, 0.1039151, 0.10385688, 0.097573474, 0.09632071, 0.09557622, 0.091599144, 0.09062039, 0.08262358, 0.08223499, 0.07993951, 0.07989185, 0.078758545, 0.078201495, 0.07737936, 0.07690251, 0.07593444, 0.07503418, 0.07482597, 0.068981, 0.06841128, 0.06764157, 0.065750405, 0.064908616, 0.061884128, 0.06010121, 0.0578873, 0.05717648, 0.056616478, 0.056017116, 0.05458274, 0.053669468] | engine-fc59fccbc-bs2sg |
# example of an inference from a bad photo
df_test = pd.DataFrame({'tensor': wallaroo.utils.flatten_np_array_columns(blurred_images_list[0], 'tensor')})
result = pipeline.infer(df_test, dataset=['time', 'out.avg_confidence','out.avg_px_intensity','out.boxes','out.classes','out.confidences','check_failures','metadata'])
result
time | out.avg_confidence | out.avg_px_intensity | out.boxes | out.classes | out.confidences | metadata.partition | |
---|---|---|---|---|---|---|---|
0 | 2024-01-10 17:53:59.016 | [0.29440108] | [0.42540666] | [[1.104647, 203.48311, 81.29011, 472.4321], [67.34002, 195.64558, 163.41652, 470.1668], [218.88916, 180.21216, 281.3725, 422.2332], [156.47955, 189.82559, 227.1866, 443.35718], [393.81195, 172.34473, 434.30322, 363.96057], [266.56137, 201.46182, 326.2503, 406.3162], [542.941, 99.440956, 588.42365, 229.33481], [426.12668, 260.50723, 638.6193, 476.10742], [511.26102, 106.84715, 546.8103, 243.0127], [0.0, 68.56848, 482.48538, 472.53766], [347.34027, 0.0, 401.10968, 97.51968], [289.03827, 0.32189485, 347.78888, 93.458755], [91.05826, 183.34473, 207.86084, 469.46518], [613.1202, 102.11072, 639.4794, 228.7474], [369.04257, 177.80518, 419.34775, 371.53873], [512.19727, 92.89032, 548.08636, 239.37686], [458.50125, 115.80958, 485.57538, 236.75961], [571.35834, 102.115395, 620.06, 230.47636], [481.23752, 105.288246, 516.5597, 246.37486], [74.288246, 0.4324219, 162.55719, 80.09118], [566.6188, 102.72982, 623.63257, 226.31448], [14.5338335, 0.0, 410.35077, 100.371155], [67.72321, 186.76591, 144.67079, 272.91965], [171.88432, 1.3620621, 220.8489, 82.6873], [455.16003, 109.83146, 486.36246, 243.25917], [320.3717, 211.61632, 373.62762, 397.29614], [476.53476, 105.55374, 517.4519, 240.22443], [530.3071, 97.575066, 617.83466, 235.27464], [146.26923, 184.24777, 186.76619, 459.51907], [610.5376, 99.28521, 638.6954, 235.62247], [316.39325, 194.10446, 375.8869, 401.48578], [540.51245, 105.909325, 584.589, 239.12834], [460.5496, 313.47333, 536.9969, 447.4658], [222.15643, 206.45018, 282.35947, 423.1165], [80.06503, 0.0, 157.40846, 79.61287], [396.70865, 235.83214, 638.7461, 473.58328], [494.6364, 115.012085, 520.81445, 241.98145], [432.90045, 145.19109, 464.7877, 264.47726], [200.3818, 181.47552, 232.85869, 429.13736], [50.631256, 161.2574, 321.71106, 465.66733], [545.57556, 106.189095, 593.3653, 227.64984], [338.0726, 1.0913361, 413.84973, 101.5233], [364.8136, 178.95511, 410.21368, 373.15686], [392.6712, 173.77844, 434.40182, 370.18982], [361.36926, 175.07799, 397.51382, 371.78812], [158.44263, 182.24762, 228.91519, 445.61328], [282.683, 0.0, 348.24307, 92.91383], [0.0, 194.40187, 640.0, 474.7329], [276.38458, 260.773, 326.8054, 407.18048], [528.4028, 105.582886, 561.3014, 239.953], [506.40353, 115.89468, 526.7106, 233.26082], [20.692535, 4.8851624, 441.1723, 215.57448], [193.52037, 188.48592, 329.2185, 428.5391], [1.6791562, 122.02866, 481.69287, 463.82855], [255.57025, 0.0, 396.8555, 100.11973], [457.83475, 91.354, 534.8592, 250.44174], [313.2646, 156.99405, 445.05853, 389.01157], [344.55948, 0.0, 370.23212, 94.05032], [24.93765, 11.427448, 439.70956, 184.92136], [433.3421, 132.6041, 471.16473, 259.3983]] | [44, 44, 44, 44, 44, 44, 44, 61, 90, 82, 44, 84, 44, 47, 44, 44, 90, 44, 44, 84, 47, 84, 44, 84, 44, 84, 90, 44, 44, 44, 44, 90, 61, 84, 44, 67, 90, 44, 44, 44, 47, 84, 84, 84, 44, 86, 44, 67, 84, 90, 90, 82, 44, 78, 84, 44, 44, 44, 78, 84] | [0.99679935, 0.9928388, 0.95979476, 0.94534546, 0.76680815, 0.7245405, 0.6529537, 0.6196737, 0.61694986, 0.6146526, 0.52818304, 0.51962215, 0.51650614, 0.50039023, 0.48194215, 0.48113948, 0.4220569, 0.35743266, 0.3185851, 0.31218198, 0.3114053, 0.29015902, 0.2836629, 0.24364658, 0.23470096, 0.23113059, 0.20228004, 0.19990075, 0.19283496, 0.18304716, 0.17492934, 0.16523221, 0.1606256, 0.15927774, 0.14796422, 0.1388699, 0.1340389, 0.13308196, 0.11703869, 0.10279331, 0.10200763, 0.0987304, 0.09823867, 0.09219642, 0.09162199, 0.088787705, 0.08765345, 0.080090344, 0.07868707, 0.07560313, 0.07533865, 0.07433937, 0.07159829, 0.069288105, 0.065867245, 0.06332389, 0.057103153, 0.05622299, 0.052092217, 0.05025773] | engine-fc59fccbc-bs2sg |
Inference Request via API
The following code performs the same inference request through the pipeline’s inference URL. This is used to demonstrate how the API inference result appears, which is used for the later examples.
headers = wl.auth.auth_header()
headers['Content-Type'] = 'application/json; format=pandas-records'
deploy_url = pipeline._deployment._url()
response = requests.post(
deploy_url,
headers=headers,
data=df_test.to_json(orient="records")
)
display(pd.DataFrame(response.json()))
time | out | metadata | |
---|---|---|---|
0 | 1704909276772 | {'avg_confidence': [0.29440108], 'avg_px_intensity': [0.42540666], 'boxes': [[1.104647, 203.48311, 81.29011, 472.4321], [67.34002, 195.64558, 163.41652, 470.1668], [218.88916, 180.21216, 281.3725, 422.2332], [156.47955, 189.82559, 227.1866, 443.35718], [393.81195, 172.34473, 434.30322, 363.96057], [266.56137, 201.46182, 326.2503, 406.3162], [542.941, 99.440956, 588.42365, 229.33481], [426.12668, 260.50723, 638.6193, 476.10742], [511.26102, 106.84715, 546.8103, 243.0127], [0.0, 68.56848, 482.48538, 472.53766], [347.34027, 0.0, 401.10968, 97.51968], [289.03827, 0.32189485, 347.78888, 93.458755], [91.05826, 183.34473, 207.86084, 469.46518], [613.1202, 102.11072, 639.4794, 228.7474], [369.04257, 177.80518, 419.34775, 371.53873], [512.19727, 92.89032, 548.08636, 239.37686], [458.50125, 115.80958, 485.57538, 236.75961], [571.35834, 102.115395, 620.06, 230.47636], [481.23752, 105.288246, 516.5597, 246.37486], [74.288246, 0.4324219, 162.55719, 80.09118], [566.6188, 102.72982, 623.63257, 226.31448], [14.5338335, 0.0, 410.35077, 100.371155], [67.72321, 186.76591, 144.67079, 272.91965], [171.88432, 1.3620621, 220.8489, 82.6873], [455.16003, 109.83146, 486.36246, 243.25917], [320.3717, 211.61632, 373.62762, 397.29614], [476.53476, 105.55374, 517.4519, 240.22443], [530.3071, 97.575066, 617.83466, 235.27464], [146.26923, 184.24777, 186.76619, 459.51907], [610.5376, 99.28521, 638.6954, 235.62247], [316.39325, 194.10446, 375.8869, 401.48578], [540.51245, 105.909325, 584.589, 239.12834], [460.5496, 313.47333, 536.9969, 447.4658], [222.15643, 206.45018, 282.35947, 423.1165], [80.06503, 0.0, 157.40846, 79.61287], [396.70865, 235.83214, 638.7461, 473.58328], [494.6364, 115.012085, 520.81445, 241.98145], [432.90045, 145.19109, 464.7877, 264.47726], [200.3818, 181.47552, 232.85869, 429.13736], [50.631256, 161.2574, 321.71106, 465.66733], [545.57556, 106.189095, 593.3653, 227.64984], [338.0726, 1.0913361, 413.84973, 101.5233], [364.8136, 178.95511, 410.21368, 373.15686], [392.6712, 173.77844, 434.40182, 370.18982], [361.36926, 175.07799, 397.51382, 371.78812], [158.44263, 182.24762, 228.91519, 445.61328], [282.683, 0.0, 348.24307, 92.91383], [0.0, 194.40187, 640.0, 474.7329], [276.38458, 260.773, 326.8054, 407.18048], [528.4028, 105.582886, 561.3014, 239.953], [506.40353, 115.89468, 526.7106, 233.26082], [20.692535, 4.8851624, 441.1723, 215.57448], [193.52037, 188.48592, 329.2185, 428.5391], [1.6791562, 122.02866, 481.69287, 463.82855], [255.57025, 0.0, 396.8555, 100.11973], [457.83475, 91.354, 534.8592, 250.44174], [313.2646, 156.99405, 445.05853, 389.01157], [344.55948, 0.0, 370.23212, 94.05032], [24.93765, 11.427448, 439.70956, 184.92136], [433.3421, 132.6041, 471.16473, 259.3983]], 'classes': [44, 44, 44, 44, 44, 44, 44, 61, 90, 82, 44, 84, 44, 47, 44, 44, 90, 44, 44, 84, 47, 84, 44, 84, 44, 84, 90, 44, 44, 44, 44, 90, 61, 84, 44, 67, 90, 44, 44, 44, 47, 84, 84, 84, 44, 86, 44, 67, 84, 90, 90, 82, 44, 78, 84, 44, 44, 44, 78, 84], 'confidences': [0.99679935, 0.9928388, 0.95979476, 0.94534546, 0.76680815, 0.7245405, 0.6529537, 0.6196737, 0.61694986, 0.6146526, 0.52818304, 0.51962215, 0.51650614, 0.50039023, 0.48194215, 0.48113948, 0.4220569, 0.35743266, 0.3185851, 0.31218198, 0.3114053, 0.29015902, 0.2836629, 0.24364658, 0.23470096, 0.23113059, 0.20228004, 0.19990075, 0.19283496, 0.18304716, 0.17492934, 0.16523221, 0.1606256, 0.15927774, 0.14796422, 0.1388699, 0.1340389, 0.13308196, 0.11703869, 0.10279331, 0.10200763, 0.0987304, 0.09823867, 0.09219642, 0.09162199, 0.088787705, 0.08765345, 0.080090344, 0.07868707, 0.07560313, 0.07533865, 0.07433937, 0.07159829, 0.069288105, 0.065867245, 0.06332389, 0.057103153, 0.05622299, 0.052092217, 0.05025773]} | {'last_model': '{"model_name":"resnet-with-intensity","model_sha":"6d58039b1a02c5cce85646292965d29056deabdfcc6b18c34adf566922c212b0"}', 'pipeline_version': '', 'elapsed': [146497846, 6506643289], 'dropped': [], 'partition': 'engine-fc59fccbc-bs2sg'} |
Store the Ops Pipeline Partition
Wallaroo pipeline logs include the metadata.partition
field that indicates what instance of the pipeline performed the inference. This partition name updates each time a new pipeline version is created; modifying the pipeline steps and other actions changes the pipeline version.
ops_partition = result.loc[0, 'metadata.partition']
ops_partition
'engine-fc59fccbc-bs2sg'
API Inference Helper Functions
The following helper functions are set up to perform inferences through either the Wallaroo Ops pipeline, or an edge deployed version of the pipeline.
For this example, update the hostname testboy.local
to the hostname of the deployed edge device.
import requests
def ops_pipeline_inference(df):
df_flattened = pd.DataFrame({'tensor': wallaroo.utils.flatten_np_array_columns(df, 'tensor')})
# api based inference request
headers = wl.auth.auth_header()
headers['Content-Type'] = 'application/json; format=pandas-records'
deploy_url = pipeline._deployment._url()
response = requests.post(
deploy_url,
headers=headers,
data=df_flattened.to_json(orient="records")
)
display(pd.DataFrame(response.json()).loc[:, ['time', 'metadata']])
def edge_pipeline_inference(df):
df_flattened = pd.DataFrame({'tensor': wallaroo.utils.flatten_np_array_columns(df, 'tensor')})
# api based inference request
# headers = wl.auth.auth_header()
headers = {
'Content-Type': 'application/json; format=pandas-records'
}
deploy_url = 'http://testboy.local:8080/pipelines/retail-inv-tracker-edge-obs'
response = requests.post(
deploy_url,
headers=headers,
data=df_flattened.to_json(orient="records")
)
display(pd.DataFrame(response.json()).loc[:, ['time', 'out', 'metadata']])
Edge Deployment
We can now deploy the pipeline to an edge device. This will require the following steps:
- Publish the pipeline: Publishes the pipeline to the OCI registry.
- Add Edge: Add the edge location to the pipeline publish.
- Deploy Edge: Deploy the edge device with the edge location settings.
pup = pipeline.publish()
Waiting for pipeline publish... It may take up to 600 sec.
Pipeline is Publishing......Published.
display(pup)
ID | 5 |
Pipeline Version | c2dd2c7d-618d-497f-8515-3aa1469d7985 |
Status | Published |
Engine URL | ghcr.io/wallaroolabs/doc-samples/engines/proxy/wallaroo/ghcr.io/wallaroolabs/standalone-mini:v2023.4.1-4351 |
Pipeline URL | ghcr.io/wallaroolabs/doc-samples/pipelines/retail-inv-tracker-edge-obs:c2dd2c7d-618d-497f-8515-3aa1469d7985 |
Helm Chart URL | oci://ghcr.io/wallaroolabs/doc-samples/charts/retail-inv-tracker-edge-obs |
Helm Chart Reference | ghcr.io/wallaroolabs/doc-samples/charts@sha256:306df0921321c299d0b04c8e0499ab0678082a197da97676e280e5d752ab415b |
Helm Chart Version | 0.0.1-c2dd2c7d-618d-497f-8515-3aa1469d7985 |
Engine Config | {'engine': {'resources': {'limits': {'cpu': 4.0, 'memory': '3Gi'}, 'requests': {'cpu': 4.0, 'memory': '3Gi'}, 'arch': 'x86', 'gpu': False}}, 'engineAux': {}, 'enginelb': {'resources': {'limits': {'cpu': 1.0, 'memory': '512Mi'}, 'requests': {'cpu': 0.2, 'memory': '512Mi'}, 'arch': 'x86', 'gpu': False}}} |
User Images | [] |
Created By | john.hummel@wallaroo.ai |
Created At | 2024-01-10 18:09:25.001491+00:00 |
Updated At | 2024-01-10 18:09:25.001491+00:00 |
Docker Run Variables | {} |
Add Edge
The edge location is added with the publish.add_edge(name)
method. This returns the OCI registration information, and the EDGE_BUNDLE
information. The EDGE_BUNDLE
data is a base64 encoded set of parameters for the pipeline that the edge device is associated with.
Edge names must be unique. Update the edge name below if required.
edge_name = 'cv-observability-demo-sample'
edge_publish = pup.add_edge(edge_name)
display(edge_publish)
ID | 5 |
Pipeline Version | c2dd2c7d-618d-497f-8515-3aa1469d7985 |
Status | Published |
Engine URL | ghcr.io/wallaroolabs/doc-samples/engines/proxy/wallaroo/ghcr.io/wallaroolabs/standalone-mini:v2023.4.1-4351 |
Pipeline URL | ghcr.io/wallaroolabs/doc-samples/pipelines/retail-inv-tracker-edge-obs:c2dd2c7d-618d-497f-8515-3aa1469d7985 |
Helm Chart URL | oci://ghcr.io/wallaroolabs/doc-samples/charts/retail-inv-tracker-edge-obs |
Helm Chart Reference | ghcr.io/wallaroolabs/doc-samples/charts@sha256:306df0921321c299d0b04c8e0499ab0678082a197da97676e280e5d752ab415b |
Helm Chart Version | 0.0.1-c2dd2c7d-618d-497f-8515-3aa1469d7985 |
Engine Config | {'engine': {'resources': {'limits': {'cpu': 4.0, 'memory': '3Gi'}, 'requests': {'cpu': 4.0, 'memory': '3Gi'}, 'arch': 'x86', 'gpu': False}}, 'engineAux': {}, 'enginelb': {'resources': {'limits': {'cpu': 1.0, 'memory': '512Mi'}, 'requests': {'cpu': 0.2, 'memory': '512Mi'}, 'arch': 'x86', 'gpu': False}}} |
User Images | [] |
Created By | john.hummel@wallaroo.ai |
Created At | 2024-01-10 18:09:25.001491+00:00 |
Updated At | 2024-01-10 18:09:25.001491+00:00 |
Docker Run Variables | {'EDGE_BUNDLE': 'abcde'} |
DevOps Deployment
The edge deployment is performed with docker run
, docker compose
, or helm
installations. The following command generates the docker run
command, with the following values provided by the DevOps Engineer:
$REGISTRYURL
$REGISTRYUSERNAME
$REGISTRYPASSWORD
Before deploying, create the ./data
directory that is used to store the authentication credentials.
# create docker run
docker_command = f'''
docker run -p 8080:8080 \\
-v ./data:/persist \\
-e DEBUG=true \\
-e OCI_REGISTRY=$REGISTRYURL \\
-e EDGE_BUNDLE={edge_publish.docker_run_variables['EDGE_BUNDLE']} \\
-e CONFIG_CPUS=6 \\
-e OCI_USERNAME=$REGISTRYUSERNAME \\
-e OCI_PASSWORD=$REGISTRYPASSWORD \\
-e PIPELINE_URL={edge_publish.pipeline_url} \\
{edge_publish.engine_url}
'''
print(docker_command)
docker run -p 8080:8080 \
-v ./data:/persist \
-e DEBUG=true \
-e OCI_REGISTRY=$REGISTRYURL \
-e EDGE_BUNDLE=ZXhwb3J0IEJVTkRMRV9WRVJTSU9OPTEKZXhwb3J0IEVER0VfTkFNRT1jdi1vYnNlcnZhYmlsaXR5LWRlbW8tc2FtcGxlCmV4cG9ydCBKT0lOX1RPS0VOPWQxN2E0NzJlLTYzNjQtNDcxMi05MWUyLWRhNzUzMjQ1MzNiYwpleHBvcnQgT1BTQ0VOVEVSX0hPU1Q9ZG9jLXRlc3QuZWRnZS53YWxsYXJvb2NvbW11bml0eS5uaW5qYQpleHBvcnQgUElQRUxJTkVfVVJMPWdoY3IuaW8vd2FsbGFyb29sYWJzL2RvYy1zYW1wbGVzL3BpcGVsaW5lcy9yZXRhaWwtaW52LXRyYWNrZXItZWRnZS1vYnM6YzJkZDJjN2QtNjE4ZC00OTdmLTg1MTUtM2FhMTQ2OWQ3OTg1CmV4cG9ydCBXT1JLU1BBQ0VfSUQ9OA== \
-e CONFIG_CPUS=6 \
-e OCI_USERNAME=$REGISTRYUSERNAME \
-e OCI_PASSWORD=$REGISTRYPASSWORD \
-e PIPELINE_URL=ghcr.io/wallaroolabs/doc-samples/pipelines/retail-inv-tracker-edge-obs:c2dd2c7d-618d-497f-8515-3aa1469d7985 \
ghcr.io/wallaroolabs/doc-samples/engines/proxy/wallaroo/ghcr.io/wallaroolabs/standalone-mini:v2023.4.1-4351
Verify Logs
Before we perform inferences on the edge deployment, we’ll collect the pipeline logs and display the current partitions. These should only include the Wallaroo Ops pipeline.
logs = pipeline.logs(dataset=['time', 'metadata'])
ops_locations = [pd.unique(logs['metadata.partition']).tolist()][0]
display(ops_locations)
ops_location = ops_locations[0]
Warning: The inference log is above the allowable limit and the following columns may have been suppressed for various rows in the logs: ['in.tensor']. To review the dropped columns for an individual inference’s suppressed data, include dataset=["metadata"] in the log request.
['engine-fc59fccbc-bs2sg']
Drift Detection Example
The following uses our baseline and blurred data to create observability values. We start with creating a set of baseline images inference results through our deployed Ops pipeline, storing the start and end dates.
baseline_start = datetime.datetime.now(datetime.timezone.utc)
for i in range(10):
for good_image in baseline_images_list:
ops_pipeline_inference(good_image)
time.sleep(10)
baseline_end = datetime.datetime.now(datetime.timezone.utc)
Build Assay with Baseline
We will use the baseline values to create our assay, specifying the start and end dates to use for the baseline values. From there we will run an interactive assay to view the current values against the baseline. Since they are just the baseline values, everything should be fine.
For our assay window, we will set the locations to both the Ops pipeline and the edge deployed pipeline. This gathers the pipeline log values from both partitions for the assay.
# create baseline from numpy
assay_name_from_dates = "average confidence drift detection example"
step_name = "resnet-with-intensity"
assay_builder_from_dates = wl.build_assay(assay_name_from_dates,
pipeline,
step_name,
iopath="output avg_confidence 0",
baseline_start=baseline_start,
baseline_end=baseline_end)
# assay from recent updates
assay_builder_from_dates = assay_builder_from_dates.add_run_until(baseline_end)
# View 1 minute intervals
# just ops
(assay_builder_from_dates
.window_builder()
.add_width(minutes=1)
.add_interval(minutes=1)
.add_start(baseline_start)
.add_location_filter([ops_partition, edge_name])
)
<wallaroo.assay_config.WindowBuilder at 0x14850dd00>
assay_config = assay_builder_from_dates.build()
assay_results = assay_config.interactive_run()
print(f"Generated {len(assay_results)} analyses")
assay_results.chart_scores()
Generated 6 analyses
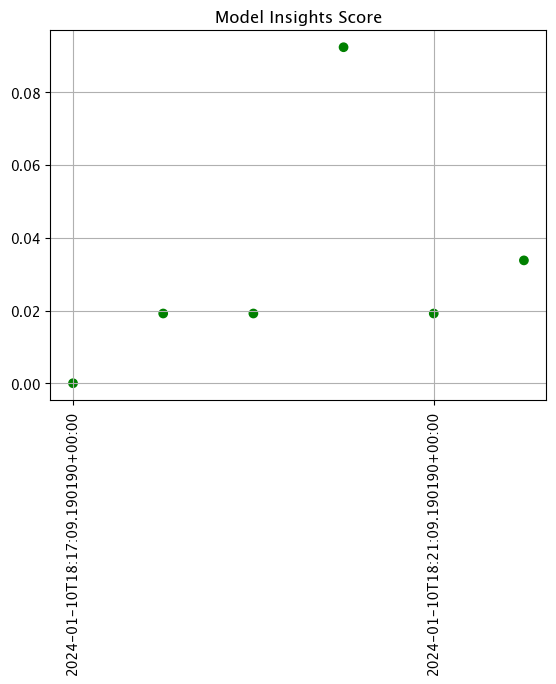
Set Observability Data
The next set of inferences will send all blurred image data to the edge deployed pipeline, and all good to the Ops pipeline. By the end, we will be able to demonstrate viewing the assay results to show detecting the blurred images causing more and more scores outside of the baseline.
Every so often we will rerun the interactive assay to show the updated results.
assay_window_start = datetime.datetime.now(datetime.timezone.utc)
for i in range(1):
for bad_image in blurred_images_list:
edge_pipeline_inference(bad_image)
for i in range(9):
for good_image in baseline_images_list:
ops_pipeline_inference(good_image)
# assay window from dates
assay_window_end = datetime.datetime.now(datetime.timezone.utc)
assay_builder_from_dates = assay_builder_from_dates.add_run_until(assay_window_end)
# View 1 minute intervals
# just combined
(assay_builder_from_dates
.window_builder()
.add_width(minutes=6)
.add_interval(minutes=6)
.add_start(assay_window_start)
.add_location_filter([ops_partition, edge_name])
)
<wallaroo.assay_config.WindowBuilder at 0x14850dd00>
assay_config = assay_builder_from_dates.build()
assay_results = assay_config.interactive_run()
print(f"Generated {len(assay_results)} analyses")
assay_results.chart_scores()
Generated 1 analyses
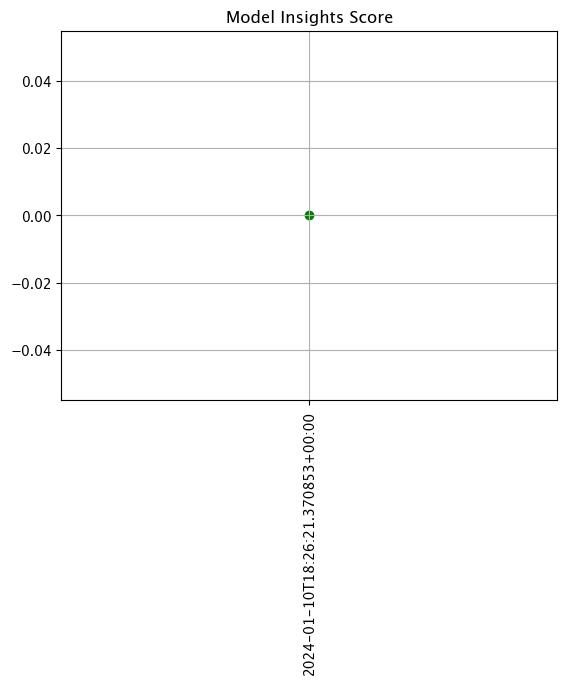
for i in range(8):
for good_image in baseline_images_list:
ops_pipeline_inference(good_image)
for i in range(2):
for bad_image in blurred_images_list:
edge_pipeline_inference(bad_image)
for i in range(7):
print(i)
for good_image in baseline_images_list:
ops_pipeline_inference(good_image)
for i in range(3):
print(i)
for bad_image in blurred_images_list:
edge_pipeline_inference(bad_image)
for i in range(6):
print(i)
for good_image in baseline_images_list:
ops_pipeline_inference(good_image)
for i in range(4):
print(i)
for bad_image in blurred_images_list:
edge_pipeline_inference(bad_image)
# assay window from dates
assay_window_end = datetime.datetime.now(datetime.timezone.utc)
assay_builder_from_dates = assay_builder_from_dates.add_run_until(assay_window_end)
# View 1 minute intervals
# just combined
(assay_builder_from_dates
.window_builder()
.add_width(minutes=7)
.add_interval(minutes=7)
.add_start(assay_window_start)
.add_location_filter([ops_partition, edge_name])
)
<wallaroo.assay_config.WindowBuilder at 0x14850dd00>
assay_config = assay_builder_from_dates.build()
assay_results = assay_config.interactive_run()
print(f"Generated {len(assay_results)} analyses")
assay_results.chart_scores()
Generated 7 analyses
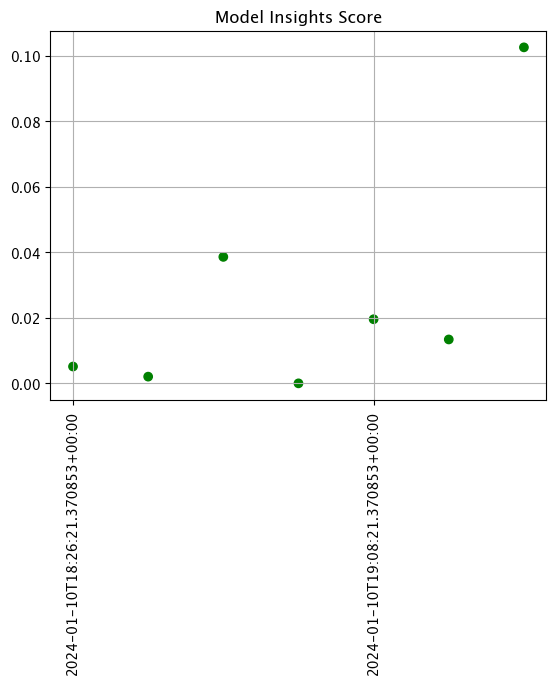
for i in range(5):
print(i)
for good_image in baseline_images_list:
ops_pipeline_inference(good_image)
for i in range(5):
print(i)
for bad_image in blurred_images_list:
edge_pipeline_inference(bad_image)
for i in range(4):
print(i)
for good_image in baseline_images_list:
ops_pipeline_inference(good_image)
for i in range(6):
print(i)
for bad_image in blurred_images_list:
edge_pipeline_inference(bad_image)
for i in range(3):
print(i)
for good_image in baseline_images_list:
ops_pipeline_inference(good_image)
for i in range(7):
print(i)
for bad_image in blurred_images_list:
edge_pipeline_inference(bad_image)
for i in range(2):
print(i)
for good_image in baseline_images_list:
ops_pipeline_inference(good_image)
for i in range(8):
print(i)
for bad_image in blurred_images_list:
edge_pipeline_inference(bad_image)
for i in range(1):
print(i)
for good_image in baseline_images_list:
ops_pipeline_inference(good_image)
for i in range(9):
print(i)
for bad_image in blurred_images_list:
edge_pipeline_inference(bad_image)
for i in range(10):
print(i)
for bad_image in blurred_images_list:
edge_pipeline_inference(bad_image)
# assay window from dates
assay_window_end = datetime.datetime.now(datetime.timezone.utc)
assay_builder_from_dates = assay_builder_from_dates.add_run_until(assay_window_end)
# just combined
(assay_builder_from_dates
.window_builder()
.add_width(minutes=6)
.add_interval(minutes=6)
.add_start(assay_window_start)
.add_location_filter([ops_partition, edge_name])
)
<wallaroo.assay_config.WindowBuilder at 0x14850dd00>
assay_config = assay_builder_from_dates.build()
assay_results = assay_config.interactive_run()
print(f"Generated {len(assay_results)} analyses")
assay_results.chart_scores()
Generated 16 analyses
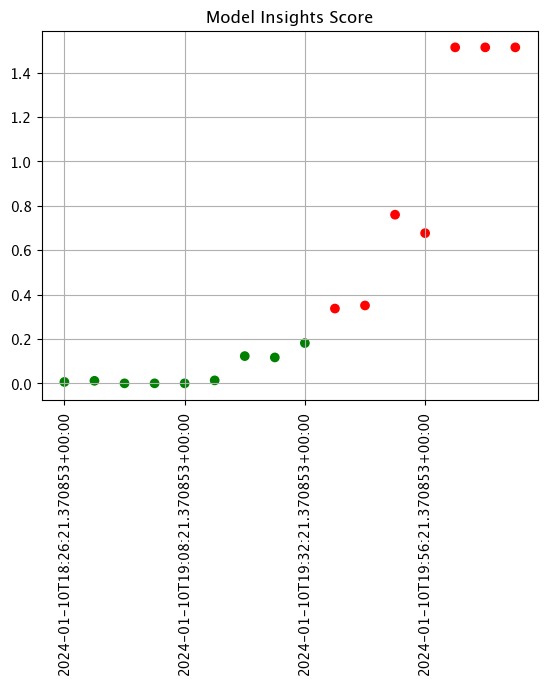
If we isolate to just the Ops center pipeline, we see a different result.
# assay window from dates
assay_window_end = datetime.datetime.now(datetime.timezone.utc)
assay_builder_from_dates = assay_builder_from_dates.add_run_until(assay_window_end)
# just combined
(assay_builder_from_dates
.window_builder()
.add_width(minutes=6)
.add_interval(minutes=6)
.add_start(assay_window_start)
.add_location_filter([ops_partition])
)
<wallaroo.assay_config.WindowBuilder at 0x14850dd00>
assay_config = assay_builder_from_dates.build()
assay_results = assay_config.interactive_run()
print(f"Generated {len(assay_results)} analyses")
assay_results.chart_scores()
Generated 13 analyses
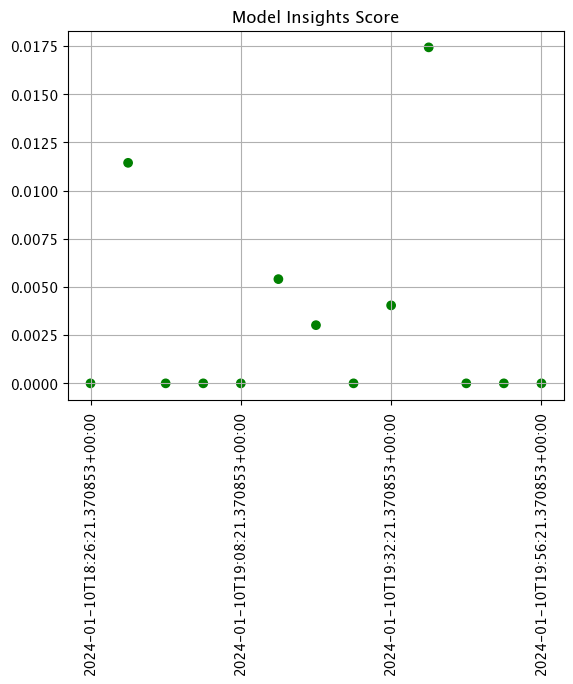
If we isolate to only the edge location, we see where the out of baseline scores are coming from.
# assay window from dates
assay_window_end = datetime.datetime.now(datetime.timezone.utc)
assay_builder_from_dates = assay_builder_from_dates.add_run_until(assay_window_end)
# just combined
(assay_builder_from_dates
.window_builder()
.add_width(minutes=6)
.add_interval(minutes=6)
.add_start(assay_window_start)
.add_location_filter([edge_name])
)
<wallaroo.assay_config.WindowBuilder at 0x14850dd00>
assay_config = assay_builder_from_dates.build()
assay_results = assay_config.interactive_run()
print(f"Generated {len(assay_results)} analyses")
assay_results.chart_scores()
Generated 12 analyses
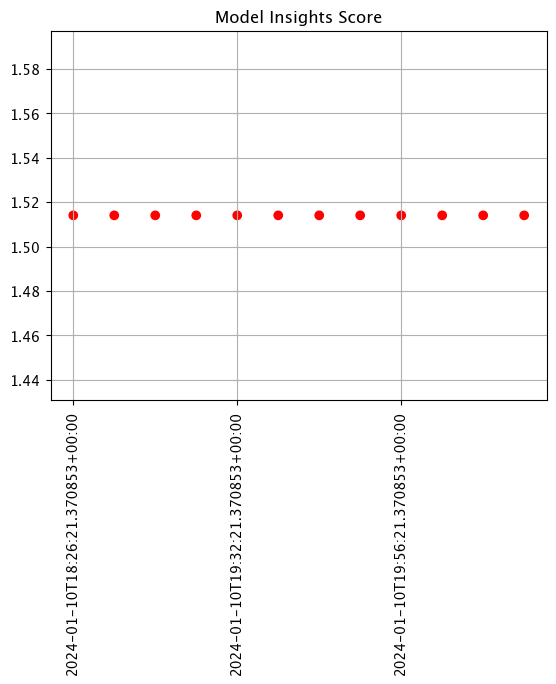
With the demonstration complete, we can shut down the edge deployed pipeline and undeploy the pipeline in the Ops center.
pipeline.undeploy()
name | retail-inv-tracker-edge-obs |
---|---|
created | 2024-01-10 17:50:59.035145+00:00 |
last_updated | 2024-01-10 18:09:22.128239+00:00 |
deployed | False |
arch | None |
tags | |
versions | c2dd2c7d-618d-497f-8515-3aa1469d7985, bee8f4f6-b1d3-40e7-9fc5-221db3ff1b87, 79321f4e-ca2d-4be1-8590-8c3be5d8953c, 64c06b04-d8fb-4651-ae5e-886c12a30e94, d0c2ed1c-3691-49f1-8fc8-e6510e4c39f8, 773099d3-6d64-4a92-b5a7-f614a916965d |
steps | resnet-with-intensity |
published | True |
2 - Wallaroo Model Observability: Anomaly Detection with CCFraud
The following tutorials are available from the Wallaroo Tutorials Repository.
Wallaroo Model Observability: Anomaly Detection with CCFraud
The following tutorial demonstrates the use case of detecting anomalies: inference input or output data that does not match typical validations.
Wallaroo provides validations to detect anomalous data from inference inputs and outputs. Validations are added to a Wallaroo pipeline with the wallaroo.pipeline.add_validations
method.
Adding validations takes the format:
pipeline.add_validations(
validation_name_01 = polars.col(in|out.{column_name}) EXPRESSION,
validation_name_02 = polars.col(in|out.{column_name}) EXPRESSION
...{additional rules}
)
validation_name
: The user provided name of the validation. The names must match Python variable naming requirements.- IMPORTANT NOTE: Using the name
count
as a validation name returns an error. Any validation rules namedcount
are dropped upon request and a warning returned.
- IMPORTANT NOTE: Using the name
polars.col(in|out.{column_name})
: Specifies the input or output for a specific field aka “column” in an inference result. Wallaroo inference requests are in the formatin.{field_name}
for inputs, andout.{field_name}
for outputs.- More than one field can be selected, as long as they follow the rules of the polars 0.18 Expressions library.
EXPRESSION
: The expression to validate. When the expression returns True, that indicates an anomaly detected.
The polars
library version 0.18.5 is used to create the validation rule. This is installed by default with the Wallaroo SDK. This provides a powerful range of comparisons to organizations tracking anomalous data from their ML models.
When validations are added to a pipeline, inference request outputs return the following fields:
Field | Type | Description |
---|---|---|
anomaly.count | Integer | The total of all validations that returned True. |
anomaly.{validation name} | Bool | The output of the validation {validation_name} . |
When validation returns True
, an anomaly is detected.
For example, adding the validation fraud
to the following pipeline returns anomaly.count
of 1
when the validation fraud
returns True
. The validation fraud
returns True
when the output field dense_1 at index 0 is greater than 0.9.
sample_pipeline = wallaroo.client.build_pipeline("sample-pipeline")
sample_pipeline.add_model_step(ccfraud_model)
# add the validation
sample_pipeline.add_validations(
fraud=pl.col("out.dense_1").list.get(0) > 0.9,
)
# deploy the pipeline
sample_pipeline.deploy()
# sample inference
display(sample_pipeline.infer_from_file("dev_high_fraud.json", data_format='pandas-records'))
 | time | in.tensor | out.dense_1 | anomaly.count | anomaly.fraud |
---|---|---|---|---|---|
0 | 2024-02-02 16:05:42.152 | [1.0678324729, 18.1555563975, -1.6589551058, 5…] | [0.981199] | 1 | True |
Detecting Anomalies from Inference Request Results
When an inference request is submitted to a Wallaroo pipeline with validations, the following fields are output:
Field | Type | Description |
---|---|---|
anomaly.count | Integer | The total of all validations that returned True. |
anomaly.{validation name} | Bool | The output of each pipeline validation {validation_name} . |
For example, adding the validation fraud
to the following pipeline returns anomaly.count
of 1
when the validation fraud
returns True
.
sample_pipeline = wallaroo.client.build_pipeline("sample-pipeline")
sample_pipeline.add_model_step(ccfraud_model)
# add the validation
sample_pipeline.add_validations(
fraud=pl.col("out.dense_1").list.get(0) > 0.9,
)
# deploy the pipeline
sample_pipeline.deploy()
# sample inference
display(sample_pipeline.infer_from_file("dev_high_fraud.json", data_format='pandas-records'))
 | time | in.tensor | out.dense_1 | anomaly.count | anomaly.fraud |
---|---|---|---|---|---|
0 | 2024-02-02 16:05:42.152 | [1.0678324729, 18.1555563975, -1.6589551058, 5…] | [0.981199] | 1 | True |
Anomaly Detection Demonstration
The following demonstrates how to:
- Upload a ccfraud ML model trained to detect the likelihood of a transaction being fraudulent. This outputs the field
dense_1
as an float where the closer to 1, the higher the likelihood of the transaction being fraudulent. - Add the ccfraud model as a pipeline step.
- Add the validation
fraud
to detect when the output ofdense_1
at index 0 when the values are greater than0.9
. - Deploy the pipeline and performing sample inferences on it.
- Perform sample inferences to show when the
fraud
validation returnsTrue
andFalse
. - Perform sample inference with different datasets to show enable or disable certain fields from displaying in the inference results.
Prerequisites
- Wallaroo version 2023.4.1 and above.
polars
version 0.18.5. This is installed by default with the Wallaroo SDK.
Tutorial Steps
Load Libraries
The first step is to import the libraries used in this notebook.
import wallaroo
wallaroo.__version__
'2023.4.1+379cb6b8a'
Connect to the Wallaroo Instance through the User Interface
The next step is to connect to Wallaroo through the Wallaroo client. The Python library is included in the Wallaroo install and available through the Jupyter Hub interface provided with your Wallaroo environment.
This is accomplished using the wallaroo.Client()
command, which provides a URL to grant the SDK permission to your specific Wallaroo environment. When displayed, enter the URL into a browser and confirm permissions. Store the connection into a variable that can be referenced later.
If logging into the Wallaroo instance through the internal JupyterHub service, use wl = wallaroo.Client()
. For more information on Wallaroo Client settings, see the Client Connection guide.
wl = wallaroo.Client()
Create a New Workspace
We’ll use the SDK below to create our workspace then assign as our current workspace. The current workspace is used by the Wallaroo SDK for where to upload models, create pipelines, etc. We’ll also set up variables for our models and pipelines down the road, so we have one spot to change names to whatever fits your organization’s standards best.
Before starting, verify that the workspace name is unique in your Wallaroo instance.
def get_workspace(name, client):
workspace = None
for ws in client.list_workspaces():
if ws.name() == name:
workspace= ws
if(workspace == None):
workspace = client.create_workspace(name)
return workspace
workspace_name = 'validation-ccfraud-demonstration-jch'
pipeline_name = 'ccfraud-validation-demo'
model_name = 'ccfraud'
model_file_name = './models/ccfraud.onnx'
workspace = get_workspace(workspace_name, wl)
wl.set_current_workspace(workspace)
{'name': 'validation-ccfraud-demonstration-jch', 'id': 19, 'archived': False, 'created_by': 'c97d480f-6064-4537-b18e-40fb1864b4cd', 'created_at': '2024-02-08T16:57:29.044902+00:00', 'models': [{'name': 'ccfraud', 'versions': 2, 'owner_id': '""', 'last_update_time': datetime.datetime(2024, 2, 8, 17, 12, 40, 341069, tzinfo=tzutc()), 'created_at': datetime.datetime(2024, 2, 8, 16, 57, 31, 612826, tzinfo=tzutc())}], 'pipelines': [{'name': 'ccfraud-validation', 'create_time': datetime.datetime(2024, 2, 8, 16, 57, 32, 340043, tzinfo=tzutc()), 'definition': '[]'}]}
Upload the Model
Upload the model to the Wallaroo workspace with the wallaroo.client.upload_model
method. Our ccfraud ML model is a Wallaroo Default Runtime of type ONNX
, so all we need is the model name, the model file path, and the framework type of wallaroo.framework.Framework.ONNX
.
ccfraud_model = (wl.upload_model(model_name,
model_file_name,
framework=wallaroo.framework.Framework.ONNX)
)
Build the Pipeline
Pipelines are build with the wallaroo.client.build_pipeline
method, which takes the pipeline name. This will create the pipeline in our default workspace. Note that if there are any existing pipelines with the same name in this workspace, this method will retrieve that pipeline for this SDK session.
Once the pipeline is created, we add the ccfraud model as our pipeline step.
sample_pipeline = wl.build_pipeline(pipeline_name)
sample_pipeline = sample_pipeline.add_model_step(ccfraud_model)
Add Validation
Now we add our validation to our new pipeline. We will give it the following configuration.
- Validation Name:
fraud
- Validation Field:
out.dense_1
- Validation Field Index:
0
- Validation Expression: Values greater than
0.9
.
The polars
library is required for creating the validation. We will import the polars library, then add our validation to the pipeline.
- IMPORTANT NOTE: Validation names must be unique per pipeline. If a validation of the same name is added, both are included in the pipeline validations, but only most recent validation with the same name is displayed with the inference results. Anomalies detected by multiple validations of the same name are added to the
anomaly.count
inference result field.
import polars as pl
sample_pipeline = sample_pipeline.add_validations(
fraud=pl.col("out.dense_1").list.get(0) > 0.9
)
Display Pipeline And Validation Steps
The method wallaroo.pipeline.steps()
shows the current pipeline steps. The added validations are in the Check
field. This is used for demonstration purposes to show the added validation to the pipeline.
sample_pipeline.steps()
[{'ModelInference': {'models': [{'name': 'ccfraud', 'version': 'f1f2ab86-a41d-4601-b14a-b594e3d86c6e', 'sha': 'bc85ce596945f876256f41515c7501c399fd97ebcb9ab3dd41bf03f8937b4507'}]}},
{'Check': {'tree': ['{"Alias":[{"BinaryExpr":{"left":{"Function":{"input":[{"Column":"out.dense_1"},{"Literal":{"Int32":0}}],"function":{"ListExpr":"Get"},"options":{"collect_groups":"ApplyFlat","fmt_str":"","input_wildcard_expansion":false,"auto_explode":true,"cast_to_supertypes":false,"allow_rename":false,"pass_name_to_apply":false,"changes_length":false,"check_lengths":true,"allow_group_aware":true}}},"op":"Gt","right":{"Literal":{"Float64":0.9}}}},"fraud"]}']}}]
Deploy Pipeline
With the pipeline steps set and the validations created, we deploy the pipeline. Because of it’s size, we will only allocate 0.1
cpu from the cluster for the pipeline’s use.
deploy_config = wallaroo.deployment_config.DeploymentConfigBuilder() \
.cpus(0.1)\
.build()
sample_pipeline.deploy(deployment_config=deploy_config)
Waiting for deployment - this will take up to 45s ......... ok
name | ccfraud-validation-demo |
---|---|
created | 2024-02-08 17:47:02.951799+00:00 |
last_updated | 2024-02-08 17:47:03.074392+00:00 |
deployed | True |
arch | None |
tags | |
versions | 5d5a2272-5c80-4eb1-9712-0a342febb775, 7baa8bc8-2218-4b09-9436-00a40407a14d |
steps | ccfraud |
published | False |
Sample Inferences
Two sample inferences are performed with the method wallaroo.pipeline.infer_from_file
that takes either a pandas Record JSON file or an Apache Arrow table as the input.
For our demonstration, we will use the following pandas Record JSON files with the following sample data:
./data/dev_smoke_test.pandas.json
: A sample inference that generates a low (lower than 0.01) likelihood of fraud../data/dev_high_fraud.pandas.json
: A sample inference that generates a high (higher than 0.90) likelihood of fraud.
The inference request returns a pandas DataFrame.
Each of the inference outputs will include the following fields:
Field | Type | Description |
---|---|---|
time | DateTime | The DateTime of the inference request. |
in.{input_field_name} | Input Dependent | Each input field submitted is labeled as in.{input_field_name} in the inference request result. For our example, this is tensor , so the input field in the returned inference request is in.tensor . |
out.{model_output_field_name} | Output Dependent | Each field output by the ML model is labeled as out.{model_output_field_name} in the inference request result. For our example, the ccfraud model returns dense_1 as its output field, so the output field in the returned inference request is out.dense_1 . |
anomaly.count | Integer | The total number of validations that returned True . |
**anomaly.{validation_name} | Bool | Each validation added to the pipeline is returned as anomaly.{validation_name} , and returns either True if the validation returns True , indicating an anomaly is found, or False for an anomaly for the validation is not found. For our example, we will have anomaly.fraud returned. |
sample_pipeline.infer_from_file("./data/dev_smoke_test.pandas.json")
time | in.dense_input | out.dense_1 | anomaly.count | anomaly.fraud | |
---|---|---|---|---|---|
0 | 2024-02-08 17:47:12.909 | [1.0678324729, 0.2177810266, -1.7115145262, 0.... | [0.0014974177] | 0 | False |
sample_pipeline.infer_from_file("./data/dev_high_fraud.json")
time | in.dense_input | out.dense_1 | anomaly.count | anomaly.fraud | |
---|---|---|---|---|---|
0 | 2024-02-08 17:47:12.962 | [1.0678324729, 18.1555563975, -1.6589551058, 5... | [0.981199] | 1 | True |
Other Validation Examples
The following are additional examples of validations.
Multiple Validations
The following uses multiple validations to check for anomalies. We still use fraud
which detects outputs that are greater than 0.9
. The second validation too_low
triggers an anomaly when the out.dense_1
is under 0.05.
After the validations are added, the pipeline is redeployed to “set” them.
sample_pipeline = sample_pipeline.add_validations(
too_low=pl.col("out.dense_1").list.get(0) < 0.001
)
deploy_config = wallaroo.deployment_config.DeploymentConfigBuilder() \
.cpus(0.1)\
.build()
sample_pipeline.undeploy()
sample_pipeline.deploy(deployment_config=deploy_config)
Waiting for undeployment - this will take up to 45s ..................................... ok
Waiting for deployment - this will take up to 45s ......... ok
name | ccfraud-validation-demo |
---|---|
created | 2024-02-08 17:47:02.951799+00:00 |
last_updated | 2024-02-08 17:47:51.243530+00:00 |
deployed | True |
arch | None |
tags | |
versions | 54982a2e-180b-4da6-ab50-e2940ec14ff5, 5d5a2272-5c80-4eb1-9712-0a342febb775, 7baa8bc8-2218-4b09-9436-00a40407a14d |
steps | ccfraud |
published | False |
sample_pipeline.infer_from_file("./data/dev_smoke_test.pandas.json")
time | in.dense_input | out.dense_1 | anomaly.count | anomaly.fraud | anomaly.too_low | |
---|---|---|---|---|---|---|
0 | 2024-02-08 17:48:00.996 | [1.0678324729, 0.2177810266, -1.7115145262, 0.... | [0.0014974177] | 0 | False | False |
sample_pipeline.infer_from_file("./data/dev_high_fraud.json")
time | in.dense_input | out.dense_1 | anomaly.count | anomaly.fraud | anomaly.too_low | |
---|---|---|---|---|---|---|
0 | 2024-02-08 17:48:01.041 | [1.0678324729, 18.1555563975, -1.6589551058, 5... | [0.981199] | 1 | True | False |
Compound Validations
The following combines multiple field checks into a single validation. For this, we will check for values of out.dense_1
that are between 0.05 and 0.9.
Each expression is separated by ()
. For example:
- Expression 1:
pl.col("out.dense_1").list.get(0) < 0.9
- Expression 2:
pl.col("out.dense_1").list.get(0) > 0.001
- Compound Expression:
(pl.col("out.dense_1").list.get(0) < 0.9) & (pl.col("out.dense_1").list.get(0) > 0.001)
sample_pipeline = sample_pipeline.add_validations(
in_between_2=(pl.col("out.dense_1").list.get(0) < 0.9) & (pl.col("out.dense_1").list.get(0) > 0.001)
)
deploy_config = wallaroo.deployment_config.DeploymentConfigBuilder() \
.cpus(0.1)\
.build()
sample_pipeline.undeploy()
sample_pipeline.deploy(deployment_config=deploy_config)
Waiting for undeployment - this will take up to 45s ..................................... ok
Waiting for deployment - this will take up to 45s ......... ok
name | ccfraud-validation-demo |
---|---|
created | 2024-02-08 17:47:02.951799+00:00 |
last_updated | 2024-02-08 17:48:39.387256+00:00 |
deployed | True |
arch | None |
tags | |
versions | 97fb284e-aa10-4192-a072-308a705b969c, 54982a2e-180b-4da6-ab50-e2940ec14ff5, 5d5a2272-5c80-4eb1-9712-0a342febb775, 7baa8bc8-2218-4b09-9436-00a40407a14d |
steps | ccfraud |
published | False |
results = sample_pipeline.infer_from_file("./data/cc_data_1k.df.json")
results.loc[results['anomaly.in_between_2'] == True]
time | in.dense_input | out.dense_1 | anomaly.count | anomaly.fraud | anomaly.in_between_2 | anomaly.too_low | |
---|---|---|---|---|---|---|---|
4 | 2024-02-08 17:48:49.305 | [0.5817662108, 0.097881551, 0.1546819424, 0.47... | [0.0010916889] | 1 | False | True | False |
7 | 2024-02-08 17:48:49.305 | [1.0379636346, -0.152987302, -1.0912561862, -0... | [0.0011294782] | 1 | False | True | False |
8 | 2024-02-08 17:48:49.305 | [0.1517283662, 0.6589966337, -0.3323713647, 0.... | [0.0018743575] | 1 | False | True | False |
9 | 2024-02-08 17:48:49.305 | [-0.1683100246, 0.7070470317, 0.1875234948, -0... | [0.0011520088] | 1 | False | True | False |
10 | 2024-02-08 17:48:49.305 | [0.6066235674, 0.0631839305, -0.0802961973, 0.... | [0.0016568303] | 1 | False | True | False |
... | ... | ... | ... | ... | ... | ... | ... |
982 | 2024-02-08 17:48:49.305 | [-0.0932906169, 0.2837744937, -0.061094265, 0.... | [0.0010192394] | 1 | False | True | False |
983 | 2024-02-08 17:48:49.305 | [0.0991458877, 0.5813808183, -0.3863062246, -0... | [0.0020678043] | 1 | False | True | False |
992 | 2024-02-08 17:48:49.305 | [1.0458395446, 0.2492453605, -1.5260449285, 0.... | [0.0013128221] | 1 | False | True | False |
998 | 2024-02-08 17:48:49.305 | [1.0046377125, 0.0343666504, -1.3512533246, 0.... | [0.0011070371] | 1 | False | True | False |
1000 | 2024-02-08 17:48:49.305 | [0.6118805301, 0.1726081102, 0.4310545502, 0.5... | [0.0012498498] | 1 | False | True | False |
179 rows × 7 columns
Specify Dataset
Wallaroo inference requests allow datasets to be excluded or included with the dataset_exclude
and dataset
parameters.
Parameter | Type | Description |
---|---|---|
dataset_exclude | List(String) | The list of datasets to exclude. Values include:
|
dataset | List(String) | The list of datasets and fields to include. |
For our example, we will exclude the anomaly
dataset, but include the datasets 'time'
, 'in'
, 'out'
, 'anomaly.count'
. Note that while we exclude anomaly
, we override that with by setting the anomaly field 'anomaly.count'
in our dataset
parameter.
sample_pipeline.infer_from_file("./data/dev_high_fraud.json",
dataset_exclude=['anomaly'],
dataset=['time', 'in', 'out', 'anomaly.count']
)
time | in.dense_input | out.dense_1 | anomaly.count | |
---|---|---|---|---|
0 | 2024-02-08 17:48:49.634 | [1.0678324729, 18.1555563975, -1.6589551058, 5... | [0.981199] | 1 |
Undeploy the Pipeline
With the demonstration complete, we undeploy the pipeline and return the resources back to the cluster.
sample_pipeline.undeploy()
Waiting for undeployment - this will take up to 45s ..................................... ok
name | ccfraud-validation-demo |
---|---|
created | 2024-02-08 17:47:02.951799+00:00 |
last_updated | 2024-02-08 17:48:39.387256+00:00 |
deployed | False |
arch | None |
tags | |
versions | 97fb284e-aa10-4192-a072-308a705b969c, 54982a2e-180b-4da6-ab50-e2940ec14ff5, 5d5a2272-5c80-4eb1-9712-0a342febb775, 7baa8bc8-2218-4b09-9436-00a40407a14d |
steps | ccfraud |
published | False |
3 - Wallaroo Model Observability: Anomaly Detection with House Price Prediction
The following tutorials are available from the Wallaroo Tutorials Repository.
Wallaroo Model Observability: Anomaly Detection with House Price Prediction
The following tutorial demonstrates the use case of detecting anomalies: inference input or output data that does not match typical validations.
Wallaroo provides validations to detect anomalous data from inference inputs and outputs. Validations are added to a Wallaroo pipeline with the wallaroo.pipeline.add_validations
method.
Adding validations takes the format:
pipeline.add_validations(
validation_name_01 = polars.col(in|out.{column_name}) EXPRESSION,
validation_name_02 = polars.col(in|out.{column_name}) EXPRESSION
...{additional rules}
)
validation_name
: The user provided name of the validation. The names must match Python variable naming requirements.- IMPORTANT NOTE: Using the name
count
as a validation name returns an error. Any validation rules namedcount
are dropped upon request and a warning returned.
- IMPORTANT NOTE: Using the name
polars.col(in|out.{column_name})
: Specifies the input or output for a specific field aka “column” in an inference result. Wallaroo inference requests are in the formatin.{field_name}
for inputs, andout.{field_name}
for outputs.- More than one field can be selected, as long as they follow the rules of the polars 0.18 Expressions library.
EXPRESSION
: The expression to validate. When the expression returns True, that indicates an anomaly detected.
The polars
library version 0.18.5 is used to create the validation rule. This is installed by default with the Wallaroo SDK. This provides a powerful range of comparisons to organizations tracking anomalous data from their ML models.
When validations are added to a pipeline, inference request outputs return the following fields:
Field | Type | Description |
---|---|---|
anomaly.count | Integer | The total of all validations that returned True. |
anomaly.{validation name} | Bool | The output of the validation {validation_name} . |
When validation returns True
, an anomaly is detected.
For example, adding the validation fraud
to the following pipeline returns anomaly.count
of 1
when the validation fraud
returns True
. The validation fraud
returns True
when the output field dense_1 at index 0 is greater than 0.9.
sample_pipeline = wallaroo.client.build_pipeline("sample-pipeline")
sample_pipeline.add_model_step(model)
# add the validation
sample_pipeline.add_validations(
fraud=pl.col("out.dense_1").list.get(0) > 0.9,
)
# deploy the pipeline
sample_pipeline.deploy()
# sample inference
display(sample_pipeline.infer_from_file("dev_high_fraud.json", data_format='pandas-records'))
 | time | in.tensor | out.dense_1 | anomaly.count | anomaly.fraud |
---|---|---|---|---|---|
0 | 2024-02-02 16:05:42.152 | [1.0678324729, 18.1555563975, -1.6589551058, 5…] | [0.981199] | 1 | True |
Detecting Anomalies from Inference Request Results
When an inference request is submitted to a Wallaroo pipeline with validations, the following fields are output:
Field | Type | Description |
---|---|---|
anomaly.count | Integer | The total of all validations that returned True. |
anomaly.{validation name} | Bool | The output of each pipeline validation {validation_name} . |
For example, adding the validation fraud
to the following pipeline returns anomaly.count
of 1
when the validation fraud
returns True
.
sample_pipeline = wallaroo.client.build_pipeline("sample-pipeline")
sample_pipeline.add_model_step(model)
# add the validation
sample_pipeline.add_validations(
fraud=pl.col("out.dense_1").list.get(0) > 0.9,
)
# deploy the pipeline
sample_pipeline.deploy()
# sample inference
display(sample_pipeline.infer_from_file("dev_high_fraud.json", data_format='pandas-records'))
 | time | in.tensor | out.dense_1 | anomaly.count | anomaly.fraud |
---|---|---|---|---|---|
0 | 2024-02-02 16:05:42.152 | [1.0678324729, 18.1555563975, -1.6589551058, 5…] | [0.981199] | 1 | True |
Anomaly Detection Demonstration
The following demonstrates how to:
- Upload a house price ML model trained to predict house prices based on a set of inputs. This outputs the field
variable
as an float which is the predicted house price. - Add the house price model as a pipeline step.
- Add the validation
too_high
to detect when a house price exceeds a certain value. - Deploy the pipeline and performing sample inferences on it.
- Perform sample inferences to show when the
too_high
validation returnsTrue
andFalse
. - Perform sample inference with different datasets to show enable or disable certain fields from displaying in the inference results.
Prerequisites
- Wallaroo version 2023.4.1 and above.
polars
version 0.18.5. This is installed by default with the Wallaroo SDK.
Tutorial Steps
Load Libraries
The first step is to import the libraries used in this notebook.
import wallaroo
wallaroo.__version__
'2023.4.1+379cb6b8a'
Connect to the Wallaroo Instance through the User Interface
The next step is to connect to Wallaroo through the Wallaroo client. The Python library is included in the Wallaroo install and available through the Jupyter Hub interface provided with your Wallaroo environment.
This is accomplished using the wallaroo.Client()
command, which provides a URL to grant the SDK permission to your specific Wallaroo environment. When displayed, enter the URL into a browser and confirm permissions. Store the connection into a variable that can be referenced later.
If logging into the Wallaroo instance through the internal JupyterHub service, use wl = wallaroo.Client()
. For more information on Wallaroo Client settings, see the Client Connection guide.
wl = wallaroo.Client()
Create a New Workspace
We’ll use the SDK below to create our workspace then assign as our current workspace. The current workspace is used by the Wallaroo SDK for where to upload models, create pipelines, etc. We’ll also set up variables for our models and pipelines down the road, so we have one spot to change names to whatever fits your organization’s standards best.
Before starting, verify that the workspace name is unique in your Wallaroo instance.
def get_workspace(name, client):
workspace = None
for ws in client.list_workspaces():
if ws.name() == name:
workspace= ws
if(workspace == None):
workspace = client.create_workspace(name)
return workspace
workspace_name = 'validation-house-price-demonstration'
pipeline_name = 'validation-demo'
model_name = 'anomaly-housing-model'
model_file_name = './models/rf_model.onnx'
workspace = get_workspace(workspace_name, wl)
wl.set_current_workspace(workspace)
{'name': 'validation-house-price-demonstration', 'id': 25, 'archived': False, 'created_by': 'c97d480f-6064-4537-b18e-40fb1864b4cd', 'created_at': '2024-02-08T21:52:50.354176+00:00', 'models': [{'name': 'anomaly-housing-model', 'versions': 1, 'owner_id': '""', 'last_update_time': datetime.datetime(2024, 2, 8, 21, 52, 51, 671284, tzinfo=tzutc()), 'created_at': datetime.datetime(2024, 2, 8, 21, 52, 51, 671284, tzinfo=tzutc())}], 'pipelines': [{'name': 'validation-demo', 'create_time': datetime.datetime(2024, 2, 8, 21, 52, 52, 879885, tzinfo=tzutc()), 'definition': '[]'}]}
Upload the Model
Upload the model to the Wallaroo workspace with the wallaroo.client.upload_model
method. Our house price ML model is a Wallaroo Default Runtime of type ONNX
, so all we need is the model name, the model file path, and the framework type of wallaroo.framework.Framework.ONNX
.
model = (wl.upload_model(model_name,
model_file_name,
framework=wallaroo.framework.Framework.ONNX)
)
Build the Pipeline
Pipelines are build with the wallaroo.client.build_pipeline
method, which takes the pipeline name. This will create the pipeline in our default workspace. Note that if there are any existing pipelines with the same name in this workspace, this method will retrieve that pipeline for this SDK session.
Once the pipeline is created, we add the ccfraud model as our pipeline step.
sample_pipeline = wl.build_pipeline(pipeline_name)
sample_pipeline.clear()
sample_pipeline = sample_pipeline.add_model_step(model)
import onnx
model = onnx.load(model_file_name)
output =[node.name for node in model.graph.output]
input_all = [node.name for node in model.graph.input]
input_initializer = [node.name for node in model.graph.initializer]
net_feed_input = list(set(input_all) - set(input_initializer))
print('Inputs: ', net_feed_input)
print('Outputs: ', output)
Inputs: ['float_input']
Outputs: ['variable']
Add Validation
Now we add our validation to our new pipeline. We will give it the following configuration.
- Validation Name:
too_high
- Validation Field:
out.variable
- Validation Field Index:
0
- Validation Expression: Values greater than
1000000.0
.
The polars
library is required for creating the validation. We will import the polars library, then add our validation to the pipeline.
- IMPORTANT NOTE: Validation names must be unique per pipeline. If a validation of the same name is added, both are included in the pipeline validations, but only most recent validation with the same name is displayed with the inference results. Anomalies detected by multiple validations of the same name are added to the
anomaly.count
inference result field.
import polars as pl
sample_pipeline = sample_pipeline.add_validations(
too_high=pl.col("out.variable").list.get(0) > 1000000.0
)
Display Pipeline And Validation Steps
The method wallaroo.pipeline.steps()
shows the current pipeline steps. The added validations are in the Check
field. This is used for demonstration purposes to show the added validation to the pipeline.
sample_pipeline.steps()
[{'ModelInference': {'models': [{'name': 'anomaly-housing-model', 'version': '9a76a2cf-9ea3-4978-8fd5-005d0280e661', 'sha': 'e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6'}]}},
{'Check': {'tree': ['{"Alias":[{"BinaryExpr":{"left":{"Function":{"input":[{"Column":"out.variable"},{"Literal":{"Int32":0}}],"function":{"ListExpr":"Get"},"options":{"collect_groups":"ApplyFlat","fmt_str":"","input_wildcard_expansion":false,"auto_explode":true,"cast_to_supertypes":false,"allow_rename":false,"pass_name_to_apply":false,"changes_length":false,"check_lengths":true,"allow_group_aware":true}}},"op":"Gt","right":{"Literal":{"Float64":1000000.0}}}},"too_high"]}']}}]
Deploy Pipeline
With the pipeline steps set and the validations created, we deploy the pipeline. Because of it’s size, we will only allocate 0.1
cpu from the cluster for the pipeline’s use.
deploy_config = wallaroo.deployment_config.DeploymentConfigBuilder() \
.cpus(0.25)\
.build()
sample_pipeline.deploy(deployment_config=deploy_config)
Waiting for undeployment - this will take up to 45s ..................................... ok
Waiting for deployment - this will take up to 45s ......... ok
name | validation-demo |
---|---|
created | 2024-02-08 21:52:52.879885+00:00 |
last_updated | 2024-02-08 22:14:13.217863+00:00 |
deployed | True |
arch | None |
tags | |
versions | 2a8d204a-f359-4f02-b558-950dbab28dc6, 424c7f24-ca65-45af-825f-64d3e9f8e8c8, 190226c9-a536-4457-9851-a68ef968b6fc, e10707fd-75b8-4386-8466-e58dc13d2828, 53b87a42-8498-475a-bf30-73fdebbf85cc |
steps | anomaly-housing-model |
published | False |
Sample Inferences
Two sample inferences are performed with the method wallaroo.pipeline.infer_from_file
that takes either a pandas Record JSON file or an Apache Arrow table as the input.
For our demonstration, we will use the following pandas Record JSON file with the following sample data:
./data/houseprice_5000_data.json
: A sample sets of 5000 houses to generates a range of predicted values.
The inference request returns a pandas DataFrame.
Each of the inference outputs will include the following fields:
Field | Type | Description |
---|---|---|
time | DateTime | The DateTime of the inference request. |
in.{input_field_name} | Input Dependent | Each input field submitted is labeled as in.{input_field_name} in the inference request result. For our example, this is tensor , so the input field in the returned inference request is in.tensor . |
out.{model_output_field_name} | Output Dependent | Each field output by the ML model is labeled as out.{model_output_field_name} in the inference request result. For our example, the ccfraud model returns dense_1 as its output field, so the output field in the returned inference request is out.dense_1 . |
anomaly.count | Integer | The total number of validations that returned True . |
**anomaly.{validation_name} | Bool | Each validation added to the pipeline is returned as anomaly.{validation_name} , and returns either True if the validation returns True , indicating an anomaly is found, or False for an anomaly for the validation is not found. For our example, we will have anomaly.fraud returned. |
results = sample_pipeline.infer_from_file('./data/test-1000.df.json')
# first 20 results
display(results.head(20))
# only results that trigger the anomaly too_high
results.loc[results['anomaly.too_high'] == True]
time | in.float_input | out.variable | anomaly.count | anomaly.too_high | |
---|---|---|---|---|---|
0 | 2024-02-08 22:14:47.075 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0,... | [718013.75] | 0 | False |
1 | 2024-02-08 22:14:47.075 | [2.0, 2.5, 2170.0, 6361.0, 1.0, 0.0, 2.0, 3.0,... | [615094.56] | 0 | False |
2 | 2024-02-08 22:14:47.075 | [3.0, 2.5, 1300.0, 812.0, 2.0, 0.0, 0.0, 3.0, ... | [448627.72] | 0 | False |
3 | 2024-02-08 22:14:47.075 | [4.0, 2.5, 2500.0, 8540.0, 2.0, 0.0, 0.0, 3.0,... | [758714.2] | 0 | False |
4 | 2024-02-08 22:14:47.075 | [3.0, 1.75, 2200.0, 11520.0, 1.0, 0.0, 0.0, 4.... | [513264.7] | 0 | False |
5 | 2024-02-08 22:14:47.075 | [3.0, 2.0, 2140.0, 4923.0, 1.0, 0.0, 0.0, 4.0,... | [668288.0] | 0 | False |
6 | 2024-02-08 22:14:47.075 | [4.0, 3.5, 3590.0, 5334.0, 2.0, 0.0, 2.0, 3.0,... | [1004846.5] | 1 | True |
7 | 2024-02-08 22:14:47.075 | [3.0, 2.0, 1280.0, 960.0, 2.0, 0.0, 0.0, 3.0, ... | [684577.2] | 0 | False |
8 | 2024-02-08 22:14:47.075 | [4.0, 2.5, 2820.0, 15000.0, 2.0, 0.0, 0.0, 4.0... | [727898.1] | 0 | False |
9 | 2024-02-08 22:14:47.075 | [3.0, 2.25, 1790.0, 11393.0, 1.0, 0.0, 0.0, 3.... | [559631.1] | 0 | False |
10 | 2024-02-08 22:14:47.075 | [3.0, 1.5, 1010.0, 7683.0, 1.5, 0.0, 0.0, 5.0,... | [340764.53] | 0 | False |
11 | 2024-02-08 22:14:47.075 | [3.0, 2.0, 1270.0, 1323.0, 3.0, 0.0, 0.0, 3.0,... | [442168.06] | 0 | False |
12 | 2024-02-08 22:14:47.075 | [4.0, 1.75, 2070.0, 9120.0, 1.0, 0.0, 0.0, 4.0... | [630865.6] | 0 | False |
13 | 2024-02-08 22:14:47.075 | [4.0, 1.0, 1620.0, 4080.0, 1.5, 0.0, 0.0, 3.0,... | [559631.1] | 0 | False |
14 | 2024-02-08 22:14:47.075 | [4.0, 3.25, 3990.0, 9786.0, 2.0, 0.0, 0.0, 3.0... | [909441.1] | 0 | False |
15 | 2024-02-08 22:14:47.075 | [4.0, 2.0, 1780.0, 19843.0, 1.0, 0.0, 0.0, 3.0... | [313096.0] | 0 | False |
16 | 2024-02-08 22:14:47.075 | [4.0, 2.5, 2130.0, 6003.0, 2.0, 0.0, 0.0, 3.0,... | [404040.8] | 0 | False |
17 | 2024-02-08 22:14:47.075 | [3.0, 1.75, 1660.0, 10440.0, 1.0, 0.0, 0.0, 3.... | [292859.5] | 0 | False |
18 | 2024-02-08 22:14:47.075 | [3.0, 2.5, 2110.0, 4118.0, 2.0, 0.0, 0.0, 3.0,... | [338357.88] | 0 | False |
19 | 2024-02-08 22:14:47.075 | [4.0, 2.25, 2200.0, 11250.0, 1.5, 0.0, 0.0, 5.... | [682284.6] | 0 | False |
time | in.float_input | out.variable | anomaly.count | anomaly.too_high | |
---|---|---|---|---|---|
6 | 2024-02-08 22:14:47.075 | [4.0, 3.5, 3590.0, 5334.0, 2.0, 0.0, 2.0, 3.0,... | [1004846.5] | 1 | True |
30 | 2024-02-08 22:14:47.075 | [4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0... | [1514079.8] | 1 | True |
40 | 2024-02-08 22:14:47.075 | [4.0, 4.5, 5120.0, 41327.0, 2.0, 0.0, 0.0, 3.0... | [1204324.8] | 1 | True |
63 | 2024-02-08 22:14:47.075 | [4.0, 3.0, 4040.0, 19700.0, 2.0, 0.0, 0.0, 3.0... | [1028923.06] | 1 | True |
110 | 2024-02-08 22:14:47.075 | [4.0, 2.5, 3470.0, 20445.0, 2.0, 0.0, 0.0, 4.0... | [1412215.3] | 1 | True |
130 | 2024-02-08 22:14:47.075 | [4.0, 2.75, 2620.0, 13777.0, 1.5, 0.0, 2.0, 4.... | [1223839.1] | 1 | True |
133 | 2024-02-08 22:14:47.075 | [5.0, 2.25, 3320.0, 13138.0, 1.0, 0.0, 2.0, 4.... | [1108000.1] | 1 | True |
154 | 2024-02-08 22:14:47.075 | [4.0, 2.75, 3800.0, 9606.0, 2.0, 0.0, 0.0, 3.0... | [1039781.25] | 1 | True |
160 | 2024-02-08 22:14:47.075 | [5.0, 3.5, 4150.0, 13232.0, 2.0, 0.0, 0.0, 3.0... | [1042119.1] | 1 | True |
210 | 2024-02-08 22:14:47.075 | [4.0, 3.5, 4300.0, 70407.0, 2.0, 0.0, 0.0, 3.0... | [1115275.0] | 1 | True |
239 | 2024-02-08 22:14:47.075 | [4.0, 3.25, 5010.0, 49222.0, 2.0, 0.0, 0.0, 5.... | [1092274.1] | 1 | True |
248 | 2024-02-08 22:14:47.075 | [4.0, 3.75, 4410.0, 8112.0, 3.0, 0.0, 4.0, 3.0... | [1967344.1] | 1 | True |
255 | 2024-02-08 22:14:47.075 | [4.0, 3.0, 4750.0, 21701.0, 1.5, 0.0, 0.0, 5.0... | [2002393.5] | 1 | True |
271 | 2024-02-08 22:14:47.075 | [5.0, 3.25, 5790.0, 13726.0, 2.0, 0.0, 3.0, 3.... | [1189654.4] | 1 | True |
281 | 2024-02-08 22:14:47.075 | [3.0, 3.0, 3570.0, 6250.0, 2.0, 0.0, 2.0, 3.0,... | [1124493.3] | 1 | True |
282 | 2024-02-08 22:14:47.075 | [3.0, 2.75, 3170.0, 34850.0, 1.0, 0.0, 0.0, 5.... | [1227073.8] | 1 | True |
283 | 2024-02-08 22:14:47.075 | [4.0, 2.75, 3260.0, 19542.0, 1.0, 0.0, 0.0, 4.... | [1364650.3] | 1 | True |
285 | 2024-02-08 22:14:47.075 | [4.0, 2.75, 4020.0, 18745.0, 2.0, 0.0, 4.0, 4.... | [1322835.9] | 1 | True |
323 | 2024-02-08 22:14:47.075 | [3.0, 3.0, 2480.0, 5500.0, 2.0, 0.0, 3.0, 3.0,... | [1100884.1] | 1 | True |
351 | 2024-02-08 22:14:47.075 | [5.0, 4.0, 4660.0, 9900.0, 2.0, 0.0, 2.0, 4.0,... | [1058105.0] | 1 | True |
360 | 2024-02-08 22:14:47.075 | [4.0, 3.5, 3770.0, 8501.0, 2.0, 0.0, 0.0, 3.0,... | [1169643.0] | 1 | True |
398 | 2024-02-08 22:14:47.075 | [3.0, 2.25, 2390.0, 7875.0, 1.0, 0.0, 1.0, 3.0... | [1364149.9] | 1 | True |
414 | 2024-02-08 22:14:47.075 | [5.0, 3.5, 5430.0, 10327.0, 2.0, 0.0, 2.0, 3.0... | [1207858.6] | 1 | True |
443 | 2024-02-08 22:14:47.075 | [5.0, 4.0, 4360.0, 8030.0, 2.0, 0.0, 0.0, 3.0,... | [1160512.8] | 1 | True |
497 | 2024-02-08 22:14:47.075 | [4.0, 2.5, 4090.0, 11225.0, 2.0, 0.0, 0.0, 3.0... | [1048372.4] | 1 | True |
513 | 2024-02-08 22:14:47.075 | [4.0, 3.25, 3320.0, 8587.0, 3.0, 0.0, 0.0, 3.0... | [1130661.0] | 1 | True |
520 | 2024-02-08 22:14:47.075 | [5.0, 3.75, 4170.0, 8142.0, 2.0, 0.0, 2.0, 3.0... | [1098628.8] | 1 | True |
530 | 2024-02-08 22:14:47.075 | [4.0, 4.25, 3500.0, 8750.0, 1.0, 0.0, 4.0, 5.0... | [1140733.8] | 1 | True |
535 | 2024-02-08 22:14:47.075 | [4.0, 3.5, 4460.0, 16271.0, 2.0, 0.0, 2.0, 3.0... | [1208638.0] | 1 | True |
556 | 2024-02-08 22:14:47.075 | [4.0, 3.5, 4285.0, 9567.0, 2.0, 0.0, 1.0, 5.0,... | [1886959.4] | 1 | True |
623 | 2024-02-08 22:14:47.075 | [4.0, 3.25, 4240.0, 25639.0, 2.0, 0.0, 3.0, 3.... | [1156651.3] | 1 | True |
624 | 2024-02-08 22:14:47.075 | [4.0, 3.5, 3440.0, 9776.0, 2.0, 0.0, 0.0, 3.0,... | [1124493.3] | 1 | True |
634 | 2024-02-08 22:14:47.075 | [4.0, 3.25, 4700.0, 38412.0, 2.0, 0.0, 0.0, 3.... | [1164589.4] | 1 | True |
651 | 2024-02-08 22:14:47.075 | [3.0, 3.0, 3920.0, 13085.0, 2.0, 1.0, 4.0, 4.0... | [1452224.5] | 1 | True |
658 | 2024-02-08 22:14:47.075 | [3.0, 3.25, 3230.0, 7800.0, 2.0, 0.0, 3.0, 3.0... | [1077279.3] | 1 | True |
671 | 2024-02-08 22:14:47.075 | [3.0, 3.5, 3080.0, 6495.0, 2.0, 0.0, 3.0, 3.0,... | [1122811.8] | 1 | True |
685 | 2024-02-08 22:14:47.075 | [4.0, 2.5, 4200.0, 35267.0, 2.0, 0.0, 0.0, 3.0... | [1181336.0] | 1 | True |
686 | 2024-02-08 22:14:47.075 | [4.0, 3.25, 4160.0, 47480.0, 2.0, 0.0, 0.0, 3.... | [1082353.3] | 1 | True |
698 | 2024-02-08 22:14:47.075 | [4.0, 4.5, 5770.0, 10050.0, 1.0, 0.0, 3.0, 5.0... | [1689843.3] | 1 | True |
711 | 2024-02-08 22:14:47.075 | [3.0, 2.5, 5403.0, 24069.0, 2.0, 1.0, 4.0, 4.0... | [1946437.3] | 1 | True |
720 | 2024-02-08 22:14:47.075 | [5.0, 3.0, 3420.0, 18129.0, 2.0, 0.0, 0.0, 3.0... | [1325961.0] | 1 | True |
722 | 2024-02-08 22:14:47.075 | [3.0, 3.25, 4560.0, 13363.0, 1.0, 0.0, 4.0, 3.... | [2005883.1] | 1 | True |
726 | 2024-02-08 22:14:47.075 | [5.0, 3.5, 4200.0, 5400.0, 2.0, 0.0, 0.0, 3.0,... | [1052898.0] | 1 | True |
737 | 2024-02-08 22:14:47.075 | [4.0, 3.25, 2980.0, 7000.0, 2.0, 0.0, 3.0, 3.0... | [1156206.5] | 1 | True |
740 | 2024-02-08 22:14:47.075 | [4.0, 4.5, 6380.0, 88714.0, 2.0, 0.0, 0.0, 3.0... | [1355747.1] | 1 | True |
782 | 2024-02-08 22:14:47.075 | [5.0, 4.25, 4860.0, 9453.0, 1.5, 0.0, 1.0, 5.0... | [1910823.8] | 1 | True |
798 | 2024-02-08 22:14:47.075 | [4.0, 2.5, 2790.0, 5450.0, 2.0, 0.0, 0.0, 3.0,... | [1097757.4] | 1 | True |
818 | 2024-02-08 22:14:47.075 | [4.0, 4.0, 4620.0, 130208.0, 2.0, 0.0, 0.0, 3.... | [1164589.4] | 1 | True |
827 | 2024-02-08 22:14:47.075 | [4.0, 2.5, 3340.0, 10422.0, 2.0, 0.0, 0.0, 3.0... | [1103101.4] | 1 | True |
828 | 2024-02-08 22:14:47.075 | [5.0, 3.5, 3760.0, 10207.0, 2.0, 0.0, 0.0, 3.0... | [1489624.5] | 1 | True |
901 | 2024-02-08 22:14:47.075 | [4.0, 2.25, 4470.0, 60373.0, 2.0, 0.0, 0.0, 3.... | [1208638.0] | 1 | True |
912 | 2024-02-08 22:14:47.075 | [3.0, 2.25, 2960.0, 8330.0, 1.0, 0.0, 3.0, 4.0... | [1178314.0] | 1 | True |
919 | 2024-02-08 22:14:47.075 | [4.0, 3.25, 5180.0, 19850.0, 2.0, 0.0, 3.0, 3.... | [1295531.3] | 1 | True |
941 | 2024-02-08 22:14:47.075 | [4.0, 3.75, 3770.0, 4000.0, 2.5, 0.0, 0.0, 5.0... | [1182821.0] | 1 | True |
965 | 2024-02-08 22:14:47.075 | [6.0, 4.0, 5310.0, 12741.0, 2.0, 0.0, 2.0, 3.0... | [2016006.0] | 1 | True |
973 | 2024-02-08 22:14:47.075 | [5.0, 2.0, 3540.0, 9970.0, 2.0, 0.0, 3.0, 3.0,... | [1085835.8] | 1 | True |
997 | 2024-02-08 22:14:47.075 | [4.0, 3.25, 2910.0, 1880.0, 2.0, 0.0, 3.0, 5.0... | [1060847.5] | 1 | True |
Other Validation Examples
The following are additional examples of validations.
Multiple Validations
The following uses multiple validations to check for anomalies. We still use fraud
which detects outputs that are greater than 1000000.0
. The second validation too_low
triggers an anomaly when the out.variable
is under 250000.0
.
After the validations are added, the pipeline is redeployed to “set” them.
sample_pipeline = sample_pipeline.add_validations(
too_low=pl.col("out.variable").list.get(0) < 250000.0
)
deploy_config = wallaroo.deployment_config.DeploymentConfigBuilder() \
.cpus(0.1)\
.build()
sample_pipeline.undeploy()
sample_pipeline.deploy(deployment_config=deploy_config)
Waiting for undeployment - this will take up to 45s ..................................... ok
Waiting for deployment - this will take up to 45s .............. ok
name | validation-demo |
---|---|
created | 2024-02-08 21:52:52.879885+00:00 |
last_updated | 2024-02-08 22:16:50.265231+00:00 |
deployed | True |
arch | None |
tags | |
versions | 053580e2-f73d-4b63-8c9c-0b5e06be96c2, 2a8d204a-f359-4f02-b558-950dbab28dc6, 424c7f24-ca65-45af-825f-64d3e9f8e8c8, 190226c9-a536-4457-9851-a68ef968b6fc, e10707fd-75b8-4386-8466-e58dc13d2828, 53b87a42-8498-475a-bf30-73fdebbf85cc |
steps | anomaly-housing-model |
published | False |
results = sample_pipeline.infer_from_file('./data/test-1000.df.json')
# first 20 results
display(results.head(20))
# only results that trigger the anomaly too_high
results.loc[results['anomaly.too_high'] == True]
# only results that trigger the anomaly too_low
results.loc[results['anomaly.too_low'] == True]
time | in.float_input | out.variable | anomaly.count | anomaly.too_high | anomaly.too_low | |
---|---|---|---|---|---|---|
0 | 2024-02-08 22:17:23.630 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0,... | [718013.75] | 0 | False | False |
1 | 2024-02-08 22:17:23.630 | [2.0, 2.5, 2170.0, 6361.0, 1.0, 0.0, 2.0, 3.0,... | [615094.56] | 0 | False | False |
2 | 2024-02-08 22:17:23.630 | [3.0, 2.5, 1300.0, 812.0, 2.0, 0.0, 0.0, 3.0, ... | [448627.72] | 0 | False | False |
3 | 2024-02-08 22:17:23.630 | [4.0, 2.5, 2500.0, 8540.0, 2.0, 0.0, 0.0, 3.0,... | [758714.2] | 0 | False | False |
4 | 2024-02-08 22:17:23.630 | [3.0, 1.75, 2200.0, 11520.0, 1.0, 0.0, 0.0, 4.... | [513264.7] | 0 | False | False |
5 | 2024-02-08 22:17:23.630 | [3.0, 2.0, 2140.0, 4923.0, 1.0, 0.0, 0.0, 4.0,... | [668288.0] | 0 | False | False |
6 | 2024-02-08 22:17:23.630 | [4.0, 3.5, 3590.0, 5334.0, 2.0, 0.0, 2.0, 3.0,... | [1004846.5] | 1 | True | False |
7 | 2024-02-08 22:17:23.630 | [3.0, 2.0, 1280.0, 960.0, 2.0, 0.0, 0.0, 3.0, ... | [684577.2] | 0 | False | False |
8 | 2024-02-08 22:17:23.630 | [4.0, 2.5, 2820.0, 15000.0, 2.0, 0.0, 0.0, 4.0... | [727898.1] | 0 | False | False |
9 | 2024-02-08 22:17:23.630 | [3.0, 2.25, 1790.0, 11393.0, 1.0, 0.0, 0.0, 3.... | [559631.1] | 0 | False | False |
10 | 2024-02-08 22:17:23.630 | [3.0, 1.5, 1010.0, 7683.0, 1.5, 0.0, 0.0, 5.0,... | [340764.53] | 0 | False | False |
11 | 2024-02-08 22:17:23.630 | [3.0, 2.0, 1270.0, 1323.0, 3.0, 0.0, 0.0, 3.0,... | [442168.06] | 0 | False | False |
12 | 2024-02-08 22:17:23.630 | [4.0, 1.75, 2070.0, 9120.0, 1.0, 0.0, 0.0, 4.0... | [630865.6] | 0 | False | False |
13 | 2024-02-08 22:17:23.630 | [4.0, 1.0, 1620.0, 4080.0, 1.5, 0.0, 0.0, 3.0,... | [559631.1] | 0 | False | False |
14 | 2024-02-08 22:17:23.630 | [4.0, 3.25, 3990.0, 9786.0, 2.0, 0.0, 0.0, 3.0... | [909441.1] | 0 | False | False |
15 | 2024-02-08 22:17:23.630 | [4.0, 2.0, 1780.0, 19843.0, 1.0, 0.0, 0.0, 3.0... | [313096.0] | 0 | False | False |
16 | 2024-02-08 22:17:23.630 | [4.0, 2.5, 2130.0, 6003.0, 2.0, 0.0, 0.0, 3.0,... | [404040.8] | 0 | False | False |
17 | 2024-02-08 22:17:23.630 | [3.0, 1.75, 1660.0, 10440.0, 1.0, 0.0, 0.0, 3.... | [292859.5] | 0 | False | False |
18 | 2024-02-08 22:17:23.630 | [3.0, 2.5, 2110.0, 4118.0, 2.0, 0.0, 0.0, 3.0,... | [338357.88] | 0 | False | False |
19 | 2024-02-08 22:17:23.630 | [4.0, 2.25, 2200.0, 11250.0, 1.5, 0.0, 0.0, 5.... | [682284.6] | 0 | False | False |
time | in.float_input | out.variable | anomaly.count | anomaly.too_high | anomaly.too_low | |
---|---|---|---|---|---|---|
21 | 2024-02-08 22:17:23.630 | [2.0, 2.0, 1390.0, 1302.0, 2.0, 0.0, 0.0, 3.0,... | [249227.8] | 1 | False | True |
69 | 2024-02-08 22:17:23.630 | [3.0, 1.75, 1050.0, 9871.0, 1.0, 0.0, 0.0, 5.0... | [236238.66] | 1 | False | True |
83 | 2024-02-08 22:17:23.630 | [3.0, 1.75, 1070.0, 8100.0, 1.0, 0.0, 0.0, 4.0... | [236238.66] | 1 | False | True |
95 | 2024-02-08 22:17:23.630 | [3.0, 2.5, 1340.0, 3011.0, 2.0, 0.0, 0.0, 3.0,... | [244380.27] | 1 | False | True |
124 | 2024-02-08 22:17:23.630 | [4.0, 1.5, 1200.0, 10890.0, 1.0, 0.0, 0.0, 5.0... | [241330.19] | 1 | False | True |
... | ... | ... | ... | ... | ... | ... |
939 | 2024-02-08 22:17:23.630 | [3.0, 1.0, 1150.0, 4800.0, 1.5, 0.0, 0.0, 4.0,... | [240834.92] | 1 | False | True |
946 | 2024-02-08 22:17:23.630 | [2.0, 1.0, 780.0, 6250.0, 1.0, 0.0, 0.0, 3.0, ... | [236815.78] | 1 | False | True |
948 | 2024-02-08 22:17:23.630 | [1.0, 1.0, 620.0, 8261.0, 1.0, 0.0, 0.0, 3.0, ... | [236815.78] | 1 | False | True |
962 | 2024-02-08 22:17:23.630 | [3.0, 1.0, 1190.0, 7500.0, 1.0, 0.0, 0.0, 5.0,... | [241330.19] | 1 | False | True |
991 | 2024-02-08 22:17:23.630 | [2.0, 1.0, 870.0, 8487.0, 1.0, 0.0, 0.0, 4.0, ... | [236238.66] | 1 | False | True |
62 rows × 6 columns
Compound Validations
The following combines multiple field checks into a single validation. For this, we will check for values of out.variable
that are between 500000 and 1000000.
Each expression is separated by ()
. For example:
- Expression 1:
pl.col("out.variable").list.get(0) < 1000000.0
- Expression 2:
pl.col("out.variable").list.get(0) > 500000.0
- Compound Expression:
(pl.col("out.variable").list.get(0) < 1000000.0) & (pl.col("out.variable").list.get(0) > 500000.0)
sample_pipeline = sample_pipeline.add_validations(
in_between=(pl.col("out.variable").list.get(0) < 1000000.0) & (pl.col("out.variable").list.get(0) > 500000.0)
)
deploy_config = wallaroo.deployment_config.DeploymentConfigBuilder() \
.cpus(0.1)\
.build()
sample_pipeline.undeploy()
sample_pipeline.deploy(deployment_config=deploy_config)
Waiting for undeployment - this will take up to 45s .................................... ok
Waiting for deployment - this will take up to 45s ............. ok
name | validation-demo |
---|---|
created | 2024-02-08 21:52:52.879885+00:00 |
last_updated | 2024-02-08 22:18:18.613710+00:00 |
deployed | True |
arch | None |
tags | |
versions | 016728d7-3948-467f-8ecc-e0594f406884, 053580e2-f73d-4b63-8c9c-0b5e06be96c2, 2a8d204a-f359-4f02-b558-950dbab28dc6, 424c7f24-ca65-45af-825f-64d3e9f8e8c8, 190226c9-a536-4457-9851-a68ef968b6fc, e10707fd-75b8-4386-8466-e58dc13d2828, 53b87a42-8498-475a-bf30-73fdebbf85cc |
steps | anomaly-housing-model |
published | False |
results = sample_pipeline.infer_from_file('./data/test-1000.df.json')
results.loc[results['anomaly.in_between'] == True]
time | in.float_input | out.variable | anomaly.count | anomaly.in_between | anomaly.too_high | anomaly.too_low | |
---|---|---|---|---|---|---|---|
0 | 2024-02-08 22:18:32.886 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0,... | [718013.75] | 1 | True | False | False |
1 | 2024-02-08 22:18:32.886 | [2.0, 2.5, 2170.0, 6361.0, 1.0, 0.0, 2.0, 3.0,... | [615094.56] | 1 | True | False | False |
3 | 2024-02-08 22:18:32.886 | [4.0, 2.5, 2500.0, 8540.0, 2.0, 0.0, 0.0, 3.0,... | [758714.2] | 1 | True | False | False |
4 | 2024-02-08 22:18:32.886 | [3.0, 1.75, 2200.0, 11520.0, 1.0, 0.0, 0.0, 4.... | [513264.7] | 1 | True | False | False |
5 | 2024-02-08 22:18:32.886 | [3.0, 2.0, 2140.0, 4923.0, 1.0, 0.0, 0.0, 4.0,... | [668288.0] | 1 | True | False | False |
... | ... | ... | ... | ... | ... | ... | ... |
989 | 2024-02-08 22:18:32.886 | [4.0, 2.75, 2500.0, 4950.0, 2.0, 0.0, 0.0, 3.0... | [700271.56] | 1 | True | False | False |
993 | 2024-02-08 22:18:32.886 | [3.0, 2.5, 2140.0, 8925.0, 2.0, 0.0, 0.0, 3.0,... | [669645.5] | 1 | True | False | False |
995 | 2024-02-08 22:18:32.886 | [3.0, 2.5, 2900.0, 23550.0, 1.0, 0.0, 0.0, 3.0... | [827411.0] | 1 | True | False | False |
998 | 2024-02-08 22:18:32.886 | [3.0, 1.75, 2910.0, 37461.0, 1.0, 0.0, 0.0, 4.... | [706823.56] | 1 | True | False | False |
999 | 2024-02-08 22:18:32.886 | [3.0, 2.0, 2005.0, 7000.0, 1.0, 0.0, 0.0, 3.0,... | [581003.0] | 1 | True | False | False |
395 rows × 7 columns
Specify Dataset
Wallaroo inference requests allow datasets to be excluded or included with the dataset_exclude
and dataset
parameters.
Parameter | Type | Description |
---|---|---|
dataset_exclude | List(String) | The list of datasets to exclude. Values include:
|
dataset | List(String) | The list of datasets and fields to include. |
For our example, we will exclude the anomaly
dataset, but include the datasets 'time'
, 'in'
, 'out'
, 'anomaly.count'
. Note that while we exclude anomaly
, we override that with by setting the anomaly field 'anomaly.count'
in our dataset
parameter.
sample_pipeline.infer_from_file('./data/test-1000.df.json',
dataset_exclude=['anomaly'],
dataset=['time', 'in', 'out', 'anomaly.count']
)
time | in.float_input | out.variable | anomaly.count | |
---|---|---|---|---|
0 | 2024-02-08 22:19:04.558 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0,... | [718013.75] | 1 |
1 | 2024-02-08 22:19:04.558 | [2.0, 2.5, 2170.0, 6361.0, 1.0, 0.0, 2.0, 3.0,... | [615094.56] | 1 |
2 | 2024-02-08 22:19:04.558 | [3.0, 2.5, 1300.0, 812.0, 2.0, 0.0, 0.0, 3.0, ... | [448627.72] | 0 |
3 | 2024-02-08 22:19:04.558 | [4.0, 2.5, 2500.0, 8540.0, 2.0, 0.0, 0.0, 3.0,... | [758714.2] | 1 |
4 | 2024-02-08 22:19:04.558 | [3.0, 1.75, 2200.0, 11520.0, 1.0, 0.0, 0.0, 4.... | [513264.7] | 1 |
... | ... | ... | ... | ... |
995 | 2024-02-08 22:19:04.558 | [3.0, 2.5, 2900.0, 23550.0, 1.0, 0.0, 0.0, 3.0... | [827411.0] | 1 |
996 | 2024-02-08 22:19:04.558 | [4.0, 1.75, 2700.0, 7875.0, 1.5, 0.0, 0.0, 4.0... | [441960.38] | 0 |
997 | 2024-02-08 22:19:04.558 | [4.0, 3.25, 2910.0, 1880.0, 2.0, 0.0, 3.0, 5.0... | [1060847.5] | 1 |
998 | 2024-02-08 22:19:04.558 | [3.0, 1.75, 2910.0, 37461.0, 1.0, 0.0, 0.0, 4.... | [706823.56] | 1 |
999 | 2024-02-08 22:19:04.558 | [3.0, 2.0, 2005.0, 7000.0, 1.0, 0.0, 0.0, 3.0,... | [581003.0] | 1 |
1000 rows × 4 columns
Undeploy the Pipeline
With the demonstration complete, we undeploy the pipeline and return the resources back to the cluster.
sample_pipeline.undeploy()
Waiting for undeployment - this will take up to 45s .................................... ok
name | validation-demo |
---|---|
created | 2024-02-08 21:52:52.879885+00:00 |
last_updated | 2024-02-08 22:18:18.613710+00:00 |
deployed | False |
arch | None |
tags | |
versions | 016728d7-3948-467f-8ecc-e0594f406884, 053580e2-f73d-4b63-8c9c-0b5e06be96c2, 2a8d204a-f359-4f02-b558-950dbab28dc6, 424c7f24-ca65-45af-825f-64d3e9f8e8c8, 190226c9-a536-4457-9851-a68ef968b6fc, e10707fd-75b8-4386-8466-e58dc13d2828, 53b87a42-8498-475a-bf30-73fdebbf85cc |
steps | anomaly-housing-model |
published | False |
4 - Wallaroo Edge Observability with Classification Financial Models
The following tutorial is available on the Wallaroo Github Repository.
Classification Financial Services Edge Deployment Demonstration
This notebook will walk through building Wallaroo pipeline with a a Classification model deployed to detect the likelihood of credit card fraud, then publishing that pipeline to an Open Container Initiative (OCI) Registry where it can be deployed in other Docker and Kubernetes environments.
This demonstration will focus on deployment to the edge. For further examples of using Wallaroo with this computer vision models, see Wallaroo 101.
This demonstration performs the following:
- In Wallaroo Ops:
- Setting up a workspace, pipeline, and model for deriving the price of a house based on inputs.
- Creating an assay from a sample of inferences.
- Display the inference result and upload the assay to the Wallaroo instance where it can be referenced later.
- In a remote aka edge location:
- Deploying the Wallaroo pipeline as a Wallaroo Inference Server deployed on an edge device with observability features.
- In Wallaroo Ops:
- Observe the Wallaroo Ops and remote Wallaroo Inference Server inference results as part of the pipeline logs.
Prerequisites
- A deployed Wallaroo Ops instance.
- A location with Docker or Kubernetes with
helm
for Wallaroo Inference server deployments. - The following Python libraries installed:
References
Data Scientist Pipeline Publish Steps
Load Libraries
The first step is to import the libraries used in this notebook.
import wallaroo
from wallaroo.object import EntityNotFoundError
import pyarrow as pa
import pandas as pd
import requests
# used to display dataframe information without truncating
from IPython.display import display
pd.set_option('display.max_colwidth', None)
Connect to the Wallaroo Instance through the User Interface
The next step is to connect to Wallaroo through the Wallaroo client. The Python library is included in the Wallaroo install and available through the Jupyter Hub interface provided with your Wallaroo environment.
This is accomplished using the wallaroo.Client()
command, which provides a URL to grant the SDK permission to your specific Wallaroo environment. When displayed, enter the URL into a browser and confirm permissions. Store the connection into a variable that can be referenced later.
If logging into the Wallaroo instance through the internal JupyterHub service, use wl = wallaroo.Client()
. For more information on Wallaroo Client settings, see the Client Connection guide.
wl = wallaroo.Client()
Create a New Workspace
We’ll use the SDK below to create our workspace , assign as our current workspace, then display all of the workspaces we have at the moment. We’ll also set up variables for our models and pipelines down the road, so we have one spot to change names to whatever fits your organization’s standards best.
To allow this tutorial to be run by multiple users in the same Wallaroo instance, a random 4 character prefix will be added to the workspace, pipeline, and model. Feel free to set suffix=''
if this is not required.
import string
import random
# make a random 4 character suffix to verify uniqueness in tutorials
random_suffix= ''.join(random.choice(string.ascii_lowercase) for i in range(4))
suffix='jch'
workspace_name = f'edge-observability-demo{suffix}'
pipeline_name = 'edge-observability-pipeline'
xgboost_model_name = 'ccfraud-xgboost'
xgboost_model_file_name = './models/xgboost_ccfraud.onnx'
def get_workspace(name):
workspace = None
for ws in wl.list_workspaces():
if ws.name() == name:
workspace= ws
if(workspace == None):
workspace = wl.create_workspace(name)
return workspace
def get_pipeline(name):
try:
pipeline = wl.pipelines_by_name(name)[0]
except EntityNotFoundError:
pipeline = wl.build_pipeline(name)
return pipeline
workspace = get_workspace(workspace_name)
wl.set_current_workspace(workspace)
{'name': 'edge-observability-demojch', 'id': 14, 'archived': False, 'created_by': 'b3deff28-04d0-41b8-a04f-b5cf610d6ce9', 'created_at': '2023-10-31T20:12:45.456363+00:00', 'models': [], 'pipelines': []}
Upload the Model
When a model is uploaded to a Wallaroo cluster, it is optimized and packaged to make it ready to run as part of a pipeline. In many times, the Wallaroo Server can natively run a model without any Python overhead. In other cases, such as a Python script, a custom Python environment will be automatically generated. This is comparable to the process of “containerizing” a model by adding a small HTTP server and other wrapping around it.
Our pretrained model is in ONNX format, which is specified in the framework
parameter.
xgboost_edge_demo_model = wl.upload_model(
xgboost_model_name,
xgboost_model_file_name,
framework=wallaroo.framework.Framework.ONNX,
).configure(tensor_fields=["tensor"])
Reserve Pipeline Resources
Before deploying an inference engine we need to tell wallaroo what resources it will need.
To do this we will use the wallaroo DeploymentConfigBuilder() and fill in the options listed below to determine what the properties of our inference engine will be.
We will be testing this deployment for an edge scenario, so the resource specifications are kept small – what’s the minimum needed to meet the expected load on the planned hardware.
- cpus - 1 => allow the engine to use 4 CPU cores when running the neural net
- memory - 900Mi => each inference engine will have 2 GB of memory, which is plenty for processing a single image at a time.
- arch - we will specify the X86 architecture.
deploy_config = (wallaroo
.DeploymentConfigBuilder()
.replica_count(1)
.cpus(1)
.memory("900Mi")
.build()
)
Simulated Edge Deployment
We will now deploy our pipeline into the current Kubernetes environment using the specified resource constraints. This is a “simulated edge” deploy in that we try to mimic the edge hardware as closely as possible.
pipeline = get_pipeline(pipeline_name)
display(pipeline)
# clear the pipeline if previously run
pipeline.clear()
pipeline.add_model_step(xgboost_edge_demo_model)
pipeline.deploy(deployment_config = deploy_config)
name | edge-observability-pipeline |
---|---|
created | 2023-10-31 20:12:45.895294+00:00 |
last_updated | 2023-10-31 20:12:45.895294+00:00 |
deployed | (none) |
arch | None |
tags | |
versions | 5d440e01-f2db-440c-a4f9-cd28bb491b6c |
steps | |
published | False |
Waiting for deployment - this will take up to 45s ....... ok
name | edge-observability-pipeline |
---|---|
created | 2023-10-31 20:12:45.895294+00:00 |
last_updated | 2023-10-31 20:12:46.009712+00:00 |
deployed | True |
arch | None |
tags | |
versions | 2b335efd-5593-422c-9ee9-52542b59601a, 5d440e01-f2db-440c-a4f9-cd28bb491b6c |
steps | ccfraud-xgboost |
published | False |
Run Single Image Inference
A single image, encoded using the Apache Arrow format, is sent to the deployed pipeline. Arrow is used here because, as a binary protocol, there is far lower network and compute overhead than using JSON. The Wallaroo Server engine accepts both JSON, pandas DataFrame, and Apache Arrow formats.
The sample DataFrames and arrow tables are in the ./data
directory. We’ll use the Apache Arrow table cc_data_10k.arrow
.
Once complete, we’ll display how long the inference request took.
import datetime
import time
deploy_url = pipeline._deployment._url()
headers = wl.auth.auth_header()
headers['Content-Type']='application/vnd.apache.arrow.file'
# headers['Content-Type']='application/json; format=pandas-records'
headers['Accept']='application/json; format=pandas-records'
dataFile = './data/cc_data_1k.arrow'
local_inference_start = datetime.datetime.now()
!curl -X POST {deploy_url} \
-H "Authorization:{headers['Authorization']}" \
-H "Content-Type:{headers['Content-Type']}" \
-H "Accept:{headers['Accept']}" \
--data-binary @{dataFile} > curl_response_xgboost.df.json
% Total % Received % Xferd Average Speed Time Time Time Current
Dload Upload Total Spent Left Speed
100 799k 100 685k 100 114k 39.3M 6733k --:--:-- --:--:-- --:--:-- 45.9M
We will import the inference output, and isolate the metadata partition
to store where the inference results are stored in the pipeline logs.
# display the first 20 results
df_results = pd.read_json('./curl_response_xgboost.df.json', orient="records")
# get just the partition
df_results['partition'] = df_results['metadata'].map(lambda x: x['partition'])
# display(df_results.head(20))
display(df_results.head(20).loc[:, ['time', 'out', 'partition']])
time | out | partition | |
---|---|---|---|
0 | 1698783174953 | {'variable': [1.0094898]} | engine-5d9b58dbd9-v5rvw |
1 | 1698783174953 | {'variable': [1.0094898]} | engine-5d9b58dbd9-v5rvw |
2 | 1698783174953 | {'variable': [1.0094898]} | engine-5d9b58dbd9-v5rvw |
3 | 1698783174953 | {'variable': [1.0094898]} | engine-5d9b58dbd9-v5rvw |
4 | 1698783174953 | {'variable': [-1.9073485999999998e-06]} | engine-5d9b58dbd9-v5rvw |
5 | 1698783174953 | {'variable': [-4.4882298e-05]} | engine-5d9b58dbd9-v5rvw |
6 | 1698783174953 | {'variable': [-9.36985e-05]} | engine-5d9b58dbd9-v5rvw |
7 | 1698783174953 | {'variable': [-8.3208084e-05]} | engine-5d9b58dbd9-v5rvw |
8 | 1698783174953 | {'variable': [-8.332728999999999e-05]} | engine-5d9b58dbd9-v5rvw |
9 | 1698783174953 | {'variable': [0.0004896521599999999]} | engine-5d9b58dbd9-v5rvw |
10 | 1698783174953 | {'variable': [0.0006609559]} | engine-5d9b58dbd9-v5rvw |
11 | 1698783174953 | {'variable': [7.57277e-05]} | engine-5d9b58dbd9-v5rvw |
12 | 1698783174953 | {'variable': [-0.000100553036]} | engine-5d9b58dbd9-v5rvw |
13 | 1698783174953 | {'variable': [-0.0005198717]} | engine-5d9b58dbd9-v5rvw |
14 | 1698783174953 | {'variable': [-3.695488e-06]} | engine-5d9b58dbd9-v5rvw |
15 | 1698783174953 | {'variable': [-0.00010883808]} | engine-5d9b58dbd9-v5rvw |
16 | 1698783174953 | {'variable': [-0.00017666817]} | engine-5d9b58dbd9-v5rvw |
17 | 1698783174953 | {'variable': [-2.8312206e-05]} | engine-5d9b58dbd9-v5rvw |
18 | 1698783174953 | {'variable': [2.1755695e-05]} | engine-5d9b58dbd9-v5rvw |
19 | 1698783174953 | {'variable': [-8.493661999999999e-05]} | engine-5d9b58dbd9-v5rvw |
Undeploy Pipeline
With our testing complete, we will undeploy the pipeline and return the resources back to the cluster.
pipeline.undeploy()
Waiting for undeployment - this will take up to 45s .................................... ok
name | edge-observability-pipeline |
---|---|
created | 2023-10-31 20:12:45.895294+00:00 |
last_updated | 2023-10-31 20:12:46.009712+00:00 |
deployed | False |
arch | None |
tags | |
versions | 2b335efd-5593-422c-9ee9-52542b59601a, 5d440e01-f2db-440c-a4f9-cd28bb491b6c |
steps | ccfraud-xgboost |
published | False |
Publish the Pipeline for Edge Deployment
It worked! For a demo, we’ll take working once as “tested”. So now that we’ve tested our pipeline, we are ready to publish it for edge deployment.
Publishing it means assembling all of the configuration files and model assets and pushing them to an Open Container Initiative (OCI) repository set in the Wallaroo instance as the Edge Registry service. DevOps engineers then retrieve that image and deploy it through Docker, Kubernetes, or similar deployments.
See Edge Deployment Registry Guide for details on adding an OCI Registry Service to Wallaroo as the Edge Deployment Registry.
This is done through the SDK command wallaroo.pipeline.publish(deployment_config)
which has the following parameters and returns.
Publish a Pipeline Parameters
The publish
method takes the following parameters. The containerized pipeline will be pushed to the Edge registry service with the model, pipeline configurations, and other artifacts needed to deploy the pipeline.
Parameter | Type | Description |
---|---|---|
deployment_config | wallaroo.deployment_config.DeploymentConfig (Optional) | Sets the pipeline deployment configuration. For example: For more information on pipeline deployment configuration, see the Wallaroo SDK Essentials Guide: Pipeline Deployment Configuration. |
Publish a Pipeline Returns
Field | Type | Description |
---|---|---|
id | integer | Numerical Wallaroo id of the published pipeline. |
pipeline version id | integer | Numerical Wallaroo id of the pipeline version published. |
status | string | The status of the pipeline publication. Values include:
|
Engine URL | string | The URL of the published pipeline engine in the edge registry. |
Pipeline URL | string | The URL of the published pipeline in the edge registry. |
Helm Chart URL | string | The URL of the helm chart for the published pipeline in the edge registry. |
Helm Chart Reference | string | The help chart reference. |
Helm Chart Version | string | The version of the Helm Chart of the published pipeline. This is also used as the Docker tag. |
Engine Config | wallaroo.deployment_config.DeploymentConfig | The pipeline configuration included with the published pipeline. |
Created At | DateTime | When the published pipeline was created. |
Updated At | DateTime | When the published pipeline was updated. |
Publish Example
We will now publish the pipeline to our Edge Deployment Registry with the pipeline.publish(deployment_config)
command. deployment_config
is an optional field that specifies the pipeline deployment. This can be overridden by the DevOps engineer during deployment.
pub=pipeline.publish(deploy_config)
display(pub)
Waiting for pipeline publish... It may take up to 600 sec.
Pipeline is Publishing....Published.
ID | 2 |
Pipeline Version | 1a5a21e2-f0e8-4148-9ee2-23c877c0ac90 |
Status | Published |
Engine URL | us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/engines/proxy/wallaroo/ghcr.io/wallaroolabs/standalone-mini:v2023.4.0-4092 |
Pipeline URL | us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/pipelines/edge-observability-pipeline:1a5a21e2-f0e8-4148-9ee2-23c877c0ac90 |
Helm Chart URL | oci://us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/charts/edge-observability-pipeline |
Helm Chart Reference | us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/charts@sha256:8bf628174b5ff87d913590d34a4c3d5eaa846b8b2a52bcf6a76295cd588cb6e8 |
Helm Chart Version | 0.0.1-1a5a21e2-f0e8-4148-9ee2-23c877c0ac90 |
Engine Config | {'engine': {'resources': {'limits': {'cpu': 1.0, 'memory': '512Mi'}, 'requests': {'cpu': 1.0, 'memory': '512Mi'}}}, 'engineAux': {'images': {}}, 'enginelb': {'resources': {'limits': {'cpu': 1.0, 'memory': '512Mi'}, 'requests': {'cpu': 1.0, 'memory': '512Mi'}}}} |
User Images | [] |
Created By | john.hummel@wallaroo.ai |
Created At | 2023-10-31 20:13:32.751657+00:00 |
Updated At | 2023-10-31 20:13:32.751657+00:00 |
Docker Run Variables | {} |
List Published Pipelines
The method wallaroo.client.list_pipelines()
shows a list of all pipelines in the Wallaroo instance, and includes the published
field that indicates whether the pipeline was published to the registry (True
), or has not yet been published (False
).
wl.list_pipelines()
name | created | last_updated | deployed | arch | tags | versions | steps | published |
---|---|---|---|---|---|---|---|---|
edge-observability-pipeline | 2023-31-Oct 20:12:45 | 2023-31-Oct 20:13:32 | False | None | 1a5a21e2-f0e8-4148-9ee2-23c877c0ac90, 2b335efd-5593-422c-9ee9-52542b59601a, 5d440e01-f2db-440c-a4f9-cd28bb491b6c | ccfraud-xgboost | True | |
housepricesagapipeline | 2023-31-Oct 20:04:58 | 2023-31-Oct 20:13:02 | True | None | 0056edf3-730f-452d-a6ed-2dfa47ff5567, 8bc714ea-8257-4512-a102-402baf3143b3, 76006480-b145-4d6a-9e95-9b2e7a4f8d8e | housepricesagacontrol | True |
List Publishes from a Pipeline
All publishes created from a pipeline are displayed with the wallaroo.pipeline.publishes
method. The pipeline_version_id
is used to know what version of the pipeline was used in that specific publish. This allows for pipelines to be updated over time, and newer versions to be sent and tracked to the Edge Deployment Registry service.
List Publishes Parameters
N/A
List Publishes Returns
A List of the following fields:
Field | Type | Description |
---|---|---|
id | integer | Numerical Wallaroo id of the published pipeline. |
pipeline_version_id | integer | Numerical Wallaroo id of the pipeline version published. |
engine_url | string | The URL of the published pipeline engine in the edge registry. |
pipeline_url | string | The URL of the published pipeline in the edge registry. |
created_by | string | The email address of the user that published the pipeline. |
Created At | DateTime | When the published pipeline was created. |
Updated At | DateTime | When the published pipeline was updated. |
pipeline.publishes()
id | pipeline_version_name | engine_url | pipeline_url | created_by | created_at | updated_at |
---|---|---|---|---|---|---|
2 | 1a5a21e2-f0e8-4148-9ee2-23c877c0ac90 | us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/engines/proxy/wallaroo/ghcr.io/wallaroolabs/standalone-mini:v2023.4.0-4092 | us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/pipelines/edge-observability-pipeline:1a5a21e2-f0e8-4148-9ee2-23c877c0ac90 | john.hummel@wallaroo.ai | 2023-31-Oct 20:13:32 | 2023-31-Oct 20:13:32 |
DevOps - Pipeline Edge Deployment
Once a pipeline is deployed to the Edge Registry service, it can be deployed in environments such as Docker, Kubernetes, or similar container running services by a DevOps engineer as a Wallaroo Server.
The following guides will demonstrate publishing a Wallaroo Pipeline as a Wallaroo Server.
Add Edge Location
Wallaroo Servers can optionally connect to the Wallaroo Ops instance and transmit their inference results. These are added to the pipeline logs for the published pipeline the Wallaroo Server is associated with.
Wallaroo Servers are added with the wallaroo.pipeline_publish.add_edge(name: string)
method. The name
is the unique primary key for each edge added to the pipeline publish and must be unique.
This returns a Publish Edge with the following fields:
Field | Type | Description |
---|---|---|
id | Integer | The integer ID of the pipeline publish. |
created_at | DateTime | The DateTime of the pipeline publish. |
docker_run_variables | String | The Docker variables in UUID format that include the following: The BUNDLE_VERSION , EDGE_NAME , JOIN_TOKEN_ , OPSCENTER_HOST , PIPELINE_URL , and WORKSPACE_ID . |
engine_config | String | The Wallaroo wallaroo.deployment_config.DeploymentConfig for the pipeline. |
pipeline_version_id | Integer | The integer identifier of the pipeline version published. |
status | String | The status of the publish. Published is a successful publish. |
updated_at | DateTime | The DateTime when the pipeline publish was updated. |
user_images | List[String] | User images used in the pipeline publish. |
created_by | String | The UUID of the Wallaroo user that created the pipeline publish. |
engine_url | String | The URL for the published pipeline’s Wallaroo engine in the OCI registry. |
error | String | Any errors logged. |
helm | String | The helm chart, helm reference and helm version. |
pipeline_url | String | The URL for the published pipeline’s container in the OCI registry. |
pipeline_version_name | String | The UUID identifier of the pipeline version published. |
additional_properties | String | Any other identities. |
Two edge publishes will be created so we can demonstrate removing an edge shortly.
edge_01_name = f'edge-ccfraud-observability{random_suffix}'
edge01 = pub.add_edge(edge_01_name)
display(edge01)
edge_02_name = f'edge-ccfraud-observability-02{random_suffix}'
edge02 = pub.add_edge(edge_02_name)
display(edge02)
ID | 2 |
Pipeline Version | 1a5a21e2-f0e8-4148-9ee2-23c877c0ac90 |
Status | Published |
Engine URL | us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/engines/proxy/wallaroo/ghcr.io/wallaroolabs/standalone-mini:v2023.4.0-4092 |
Pipeline URL | us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/pipelines/edge-observability-pipeline:1a5a21e2-f0e8-4148-9ee2-23c877c0ac90 |
Helm Chart URL | oci://us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/charts/edge-observability-pipeline |
Helm Chart Reference | us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/charts@sha256:8bf628174b5ff87d913590d34a4c3d5eaa846b8b2a52bcf6a76295cd588cb6e8 |
Helm Chart Version | 0.0.1-1a5a21e2-f0e8-4148-9ee2-23c877c0ac90 |
Engine Config | {'engine': {'resources': {'limits': {'cpu': 1.0, 'memory': '512Mi'}, 'requests': {'cpu': 1.0, 'memory': '512Mi'}}}, 'engineAux': {'images': {}}, 'enginelb': {'resources': {'limits': {'cpu': 1.0, 'memory': '512Mi'}, 'requests': {'cpu': 1.0, 'memory': '512Mi'}}}} |
User Images | [] |
Created By | john.hummel@wallaroo.ai |
Created At | 2023-10-31 20:13:32.751657+00:00 |
Updated At | 2023-10-31 20:13:32.751657+00:00 |
Docker Run Variables | {'EDGE_BUNDLE': 'abcde'} |
ID | 2 |
Pipeline Version | 1a5a21e2-f0e8-4148-9ee2-23c877c0ac90 |
Status | Published |
Engine URL | us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/engines/proxy/wallaroo/ghcr.io/wallaroolabs/standalone-mini:v2023.4.0-4092 |
Pipeline URL | us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/pipelines/edge-observability-pipeline:1a5a21e2-f0e8-4148-9ee2-23c877c0ac90 |
Helm Chart URL | oci://us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/charts/edge-observability-pipeline |
Helm Chart Reference | us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/charts@sha256:8bf628174b5ff87d913590d34a4c3d5eaa846b8b2a52bcf6a76295cd588cb6e8 |
Helm Chart Version | 0.0.1-1a5a21e2-f0e8-4148-9ee2-23c877c0ac90 |
Engine Config | {'engine': {'resources': {'limits': {'cpu': 1.0, 'memory': '512Mi'}, 'requests': {'cpu': 1.0, 'memory': '512Mi'}}}, 'engineAux': {'images': {}}, 'enginelb': {'resources': {'limits': {'cpu': 1.0, 'memory': '512Mi'}, 'requests': {'cpu': 1.0, 'memory': '512Mi'}}}} |
User Images | [] |
Created By | john.hummel@wallaroo.ai |
Created At | 2023-10-31 20:13:32.751657+00:00 |
Updated At | 2023-10-31 20:13:32.751657+00:00 |
Docker Run Variables | {'EDGE_BUNDLE': 'abcde'} |
pipeline.list_edges()
ID | Name | Tags | Pipeline Version | SPIFFE ID |
---|---|---|---|---|
898bb58c-77c2-4164-b6cc-f004dc39e125 | edge-ccfraud-observabilityymgy | [] | 6 | wallaroo.ai/ns/deployments/edge/898bb58c-77c2-4164-b6cc-f004dc39e125 |
1f35731a-f4f6-4cd0-a23a-c4a326b73277 | edge-ccfraud-observability-02ymgy | [] | 6 | wallaroo.ai/ns/deployments/edge/1f35731a-f4f6-4cd0-a23a-c4a326b73277 |
Remove Edge Location
Wallaroo Servers are removed with the wallaroo.pipeline_publish.remove_edge(name: string)
method.
This returns a Publish Edge with the following fields:
Field | Type | Description |
---|---|---|
id | Integer | The integer ID of the pipeline publish. |
created_at | DateTime | The DateTime of the pipeline publish. |
docker_run_variables | String | The Docker variables in UUID format that include the following: The BUNDLE_VERSION , EDGE_NAME , JOIN_TOKEN_ , OPSCENTER_HOST , PIPELINE_URL , and WORKSPACE_ID . |
engine_config | String | The Wallaroo wallaroo.deployment_config.DeploymentConfig for the pipeline. |
pipeline_version_id | Integer | The integer identifier of the pipeline version published. |
status | String | The status of the publish. Published is a successful publish. |
updated_at | DateTime | The DateTime when the pipeline publish was updated. |
user_images | List[String] | User images used in the pipeline publish. |
created_by | String | The UUID of the Wallaroo user that created the pipeline publish. |
engine_url | String | The URL for the published pipeline’s Wallaroo engine in the OCI registry. |
error | String | Any errors logged. |
helm | String | The helm chart, helm reference and helm version. |
pipeline_url | String | The URL for the published pipeline’s container in the OCI registry. |
pipeline_version_name | String | The UUID identifier of the pipeline version published. |
additional_properties | String | Any other identities. |
Two edge publishes will be created so we can demonstrate removing an edge shortly.
sample = pub.remove_edge(edge_02_name)
display(sample)
None
pipeline.list_edges()
ID | Name | Tags | Pipeline Version | SPIFFE ID |
---|---|---|---|---|
898bb58c-77c2-4164-b6cc-f004dc39e125 | edge-ccfraud-observabilityymgy | [] | 6 | wallaroo.ai/ns/deployments/edge/898bb58c-77c2-4164-b6cc-f004dc39e125 |
Docker Deployment
First, the DevOps engineer must authenticate to the same OCI Registry service used for the Wallaroo Edge Deployment registry.
For more details, check with the documentation on your artifact service. The following are provided for the three major cloud services:
- Set up authentication for Docker
- Authenticate with an Azure container registry
- Authenticating Amazon ECR Repositories for Docker CLI with Credential Helper
For the deployment, the engine URL is specified with the following environmental variables:
DEBUG
(true|false): Whether to include debug output.OCI_REGISTRY
: The URL of the registry service.CONFIG_CPUS
: The number of CPUs to use.OCI_USERNAME
: The edge registry username.OCI_PASSWORD
: The edge registry password or token.PIPELINE_URL
: The published pipeline URL.
Docker Deployment Example
Using our sample environment, here’s sample deployment using Docker with a computer vision ML model, the same used in the Wallaroo Use Case Tutorials Computer Vision: Retail tutorials.
docker run -p 8080:8080 \
-e DEBUG=true \
-e OCI_REGISTRY={your registry server} \
-e EDGE_BUNDLE={Your edge bundle['EDGE_BUNDLE']}
-e CONFIG_CPUS=1 \
-e OCI_USERNAME=oauth2accesstoken \
-e OCI_PASSWORD={registry token here} \
-e PIPELINE_URL={your registry server}{Your Wallaroo Server pipeline} \
{your registry server}/{Your Wallaroo Server engine}
Docker Compose Deployment
For users who prefer to use docker compose
, the following sample compose.yaml
file is used to launch the Wallaroo Edge pipeline. This is the same used in the Wallaroo Use Case Tutorials Computer Vision: Retail tutorials.
services:
engine:
image: {Your Engine URL}
ports:
- 8080:8080
environment:
EDGE_BUNDLE: {Your Edge Bundle['EDGE_BUNDLE']}
PIPELINE_URL: {Your Pipeline URL}
OCI_REGISTRY: {Your Edge Registry URL}
OCI_USERNAME: {Your Registry Username}
OCI_PASSWORD: {Your Token or Password}
CONFIG_CPUS: 1
For example:
services:
engine:
image: sample-registry.com/engine:v2023.3.0-main-3707
ports:
- 8080:8080
environment:
PIPELINE_URL: sample-registry.com/pipelines/edge-cv-retail:bf70eaf7-8c11-4b46-b751-916a43b1a555
OCI_REGISTRY: sample-registry.com
OCI_USERNAME: _json_key_base64
OCI_PASSWORD: abc123
CONFIG_CPUS: 1
Docker Compose Deployment Example
The deployment and undeployment is then just a simple docker compose up
and docker compose down
. The following shows an example of deploying the Wallaroo edge pipeline using docker compose
.
docker compose up
[+] Running 1/1
✔ Container cv_data-engine-1 Recreated 0.5s
Attaching to cv_data-engine-1
cv_data-engine-1 | Wallaroo Engine - Standalone mode
cv_data-engine-1 | Login Succeeded
cv_data-engine-1 | Fetching manifest and config for pipeline: sample-registry.com/pipelines/edge-cv-retail:bf70eaf7-8c11-4b46-b751-916a43b1a555
cv_data-engine-1 | Fetching model layers
cv_data-engine-1 | digest: sha256:c6c8869645962e7711132a7e17aced2ac0f60dcdc2c7faa79b2de73847a87984
cv_data-engine-1 | filename: c6c8869645962e7711132a7e17aced2ac0f60dcdc2c7faa79b2de73847a87984
cv_data-engine-1 | name: resnet-50
cv_data-engine-1 | type: model
cv_data-engine-1 | runtime: onnx
cv_data-engine-1 | version: 693e19b5-0dc7-4afb-9922-e3f7feefe66d
cv_data-engine-1 |
cv_data-engine-1 | Fetched
cv_data-engine-1 | Starting engine
cv_data-engine-1 | Looking for preexisting `yaml` files in //modelconfigs
cv_data-engine-1 | Looking for preexisting `yaml` files in //pipelines
Helm Deployment
Published pipelines can be deployed through the use of helm charts.
Helm deployments take up to two steps - the first step is in retrieving the required values.yaml
and making updates to override.
- Pull the helm charts from the published pipeline. The two fields are the Helm Chart URL and the Helm Chart version to specify the OCI . This typically takes the format of:
helm pull oci://{published.helm_chart_url} --version {published.helm_chart_version}
- Extract the
tgz
file and copy thevalues.yaml
and copy the values used to edit engine allocations, etc. The following are required for the deployment to run:
ociRegistry:
registry: {your registry service}
username: {registry username here}
password: {registry token here}
Store this into another file, suc as local-values.yaml
.
- Create the namespace to deploy the pipeline to. For example, the namespace
wallaroo-edge-pipeline
would be:
kubectl create -n wallaroo-edge-pipeline
Deploy the
helm
installation withhelm install
through one of the following options:Specify the
tgz
file that was downloaded and the local values file. For example:helm install --namespace {namespace} --values {local values file} {helm install name} {tgz path}
Specify the expended directory from the downloaded
tgz
file.helm install --namespace {namespace} --values {local values file} {helm install name} {helm directory path}
Specify the Helm Pipeline Helm Chart and the Pipeline Helm Version.
helm install --namespace {namespace} --values {local values file} {helm install name} oci://{published.helm_chart_url} --version {published.helm_chart_version}
Once deployed, the DevOps engineer will have to forward the appropriate ports to the
svc/engine-svc
service in the specific pipeline. For example, usingkubectl port-forward
to the namespaceccfraud
that would be:kubectl port-forward svc/engine-svc -n ccfraud01 8080 --address 0.0.0.0`
The following code segment generates a docker run
template based on the previously published pipeline. Replace the $REGISTRYURL
, $REGISTRYUSERNAME
and $REGISTRYPASSWORD
with your OCI registry values.
docker_command = f'''
docker run -p 8080:8080 \\
-e DEBUG=true \\
-e OCI_REGISTRY=$REGISTRYURL \\
-e EDGE_BUNDLE={edge01.docker_run_variables['EDGE_BUNDLE']} \\
-e CONFIG_CPUS=1 \\
-e OCI_USERNAME=$REGISTRYUSERNAME \\
-e OCI_PASSWORD=$REGISTRYPASSWORD \\
-e PIPELINE_URL={edge01.pipeline_url} \\
{edge01.engine_url}
'''
print(docker_command)
Edge Deployed Pipeline API Endpoints
Once deployed, we can check the pipelines and models available. We’ll use a curl
command, but any HTTP based request will work the same way.
The endpoint /pipelines
returns:
- id (String): The name of the pipeline.
- status (String): The status as either
Running
, orError
if there are any issues.
For this example, the deployment is made on a machine called localhost
. Replace this URL with the URL of you edge deployment.
!curl testboy.local:8080/pipelines
The endpoint /models
returns a List of models with the following fields:
- name (String): The model name.
- sha (String): The sha hash value of the ML model.
- status (String): The status of either Running or Error if there are any issues.
- version (String): The model version. This matches the version designation used by Wallaroo to track model versions in UUID format.
!curl testboy.local:8080/models
Edge Inference Endpoint
The inference endpoint takes the following pattern:
/pipelines/{pipeline-name}
: Thepipeline-name
is the same as returned from the/pipelines
endpoint asid
.
Wallaroo inference endpoint URLs accept the following data inputs through the Content-Type
header:
Content-Type: application/vnd.apache.arrow.file
: For Apache Arrow tables.Content-Type: application/json; format=pandas-records
: For pandas DataFrame in record format.
Once deployed, we can perform an inference through the deployment URL.
The endpoint returns Content-Type: application/json; format=pandas-records
by default with the following fields:
- check_failures (List[Integer]): Whether any validation checks were triggered. For more information, see Wallaroo SDK Essentials Guide: Pipeline Management: Anomaly Testing.
- elapsed (List[Integer]): A list of time in nanoseconds for:
- [0] The time to serialize the input.
- [1…n] How long each step took.
- model_name (String): The name of the model used.
- model_version (String): The version of the model in UUID format.
- original_data: The original input data. Returns
null
if the input may be too long for a proper return. - outputs (List): The outputs of the inference result separated by data type, where each data type includes:
- data: The returned values.
- dim (List[Integer]): The dimension shape returned.
- v (Integer): The vector shape of the data.
- pipeline_name (String): The name of the pipeline.
- shadow_data: Any shadow deployed data inferences in the same format as outputs.
- time (Integer): The time since UNIX epoch.
!curl -X POST testboy.local:8080/pipelines/edge-observability-pipeline \
-H "Content-Type: application/vnd.apache.arrow.file" \
--data-binary @./data/cc_data_1k.arrow > curl_response_edge.df.json
# display the first 20 results
df_results = pd.read_json('./curl_response_edge.df.json', orient="records")
# display(df_results.head(20))
display(df_results.head(20).loc[:, ['time', 'out', 'metadata']])
pipeline.export_logs(
limit=30000,
directory='partition-edge-observability',
file_prefix='edge-logs',
dataset=['time', 'out', 'metadata']
)
# display the head 20 results
df_logs = pd.read_json('./partition-edge-observability/edge-logs-1.json', orient="records", lines=True)
# get just the partition
# df_results['partition'] = df_results['metadata'].map(lambda x: x['partition'])
# display(df_results.head(20))
display(df_logs.head(20).loc[:, ['time', 'out.variable', 'metadata.partition']])
display(pd.unique(df_logs['metadata.partition']))
5 - Wallaroo Assays Model Insights Tutorial
This tutorial and the assets can be downloaded as part of the Wallaroo Tutorials repository.
Model Drift Observability with Assays
The Model Insights feature lets you monitor how the environment that your model operates within may be changing in ways that affect it’s predictions so that you can intervene (retrain) in an efficient and timely manner. Changes in the inputs, data drift, can occur due to errors in the data processing pipeline or due to changes in the environment such as user preference or behavior.
This notebook focuses on interactive exploration over historical data. After you are comfortable with how your data has behaved historically, you can schedule this same analysis (called an assay) to automatically run periodically, looking for indications of data drift or concept drift.
In this notebook, we will be running a drift assay on an ONNX model pre-trained to predict house prices.
Goal
Model insights monitors the output of the spam classifier model over a designated time window and compares it to an expected baseline distribution. We measure the difference between the window distribution and the baseline distribution; large differences indicate that the behavior of the model (or its inputs) has changed from what we expect. This possibly indicates a change that should be accounted for, possibly by retraining the models.
Resources
This tutorial provides the following:
- Models:
models/rf_model.onnx
: The champion model that has been used in this environment for some time.- Various inputs:
smallinputs.df.json
: A set of house inputs that tends to generate low house price values.biginputs.df.json
: A set of house inputs that tends to generate high house price values.
Prerequisites
- A deployed Wallaroo instance
- The following Python libraries installed:
Steps
- Deploying a sample ML model used to determine house prices based on a set of input parameters.
- Build an assay baseline from a set of baseline start and end dates, and an assay baseline from a numpy array.
- Preview the assay and show different assay configurations.
- Upload the assay.
- View assay results.
- Pause and resume the assay.
Import Libraries
The first step will be to import our libraries, and set variables used through this tutorial.
import wallaroo
from wallaroo.object import EntityNotFoundError
from wallaroo.framework import Framework
from IPython.display import display
# used to display DataFrame information without truncating
from IPython.display import display
import pandas as pd
pd.set_option('display.max_colwidth', None)
import datetime
import time
# used for unique connection names
import string
import random
suffix= ''.join(random.choice(string.ascii_lowercase) for i in range(4))
suffix=''
# used to make a unique workspace
suffix=''
workspace_name = f'assay-demonstration-tutorial{suffix}'
main_pipeline_name = f'assay-demonstration-tutorial'
model_name_control = f'house-price-estimator'
model_file_name_control = './models/rf_model.onnx'
# Set the name of the assay
assay_name=f"house price test{suffix}"
# ignoring warnings for demonstration
import warnings
warnings.filterwarnings('ignore')
# used to display DataFrame information without truncating
from IPython.display import display
import pandas as pd
pd.set_option('display.max_colwidth', None)
def get_workspace(name, client):
workspace = None
for ws in client.list_workspaces():
if ws.name() == name:
workspace= ws
if(workspace == None):
workspace = client.create_workspace(name)
return workspace
Connect to the Wallaroo Instance
The first step is to connect to Wallaroo through the Wallaroo client. The Python library is included in the Wallaroo install and available through the Jupyter Hub interface provided with your Wallaroo environment.
This is accomplished using the wallaroo.Client()
command, which provides a URL to grant the SDK permission to your specific Wallaroo environment. When displayed, enter the URL into a browser and confirm permissions. Store the connection into a variable that can be referenced later.
If logging into the Wallaroo instance through the internal JupyterHub service, use wl = wallaroo.Client()
. For more information on Wallaroo Client settings, see the Client Connection guide.
# Login through local Wallaroo instance
wl = wallaroo.Client()
wl = wallaroo.Client()
wallarooPrefix = "doc-test."
wallarooSuffix = "wallarooexample.ai"
wl = wallaroo.Client(api_endpoint=f"https://{wallarooPrefix}api.{wallarooSuffix}",
auth_endpoint=f"https://{wallarooPrefix}keycloak.{wallarooSuffix}",
auth_type="sso")
Create Workspace
We will create a workspace to manage our pipeline and models. The following variables will set the name of our sample workspace then set it as the current workspace.
Workspace, pipeline, and model names should be unique to each user, so we’ll add in a randomly generated suffix so multiple people can run this tutorial in a Wallaroo instance without effecting each other.
workspace = get_workspace(workspace_name, wl)
wl.set_current_workspace(workspace)
{'name': 'assay-demonstration-tutorial', 'id': 5, 'archived': False, 'created_by': '755d34dd-e562-454e-82ed-593d6f3244b7', 'created_at': '2024-02-15T16:18:22.083904+00:00', 'models': [], 'pipelines': []}
Upload The Champion Model
For our example, we will upload the champion model that has been trained to derive house prices from a variety of inputs. The model file is rf_model.onnx
, and is uploaded with the name house-price-estimator
.
housing_model_control = (wl.upload_model(model_name_control,
model_file_name_control,
framework=Framework.ONNX)
.configure(tensor_fields=["tensor"])
)
Build the Pipeline
This pipeline is made to be an example of an existing situation where a model is deployed and being used for inferences in a production environment. We’ll call it assay-demonstration-tutorial
, set housing_model_control
as a pipeline step, then run a few sample inferences.
This pipeline will be a simple one - just a single pipeline step.
mainpipeline = wl.build_pipeline(main_pipeline_name)
# clear the steps if used before
mainpipeline.clear()
mainpipeline.add_model_step(housing_model_control)
#minimum deployment config
deploy_config = wallaroo.DeploymentConfigBuilder().replica_count(1).cpus(0.5).memory("1Gi").build()
mainpipeline.deploy(deployment_config = deploy_config)
name | assay-demonstration-tutorial |
---|---|
created | 2024-02-15 16:18:25.122619+00:00 |
last_updated | 2024-02-15 16:18:26.007576+00:00 |
deployed | True |
arch | None |
tags | |
versions | 53ea84b7-21d0-4aad-9e20-5d8f368abc63, 8de360dd-a086-4b7f-a5ec-84bae69e13ac |
steps | house-price-estimator |
published | False |
Testing
We’ll use two inferences as a quick sample test - one that has a house that should be determined around $700k
, the other with a house determined to be around $1.5
million.
normal_input = pd.DataFrame.from_records({"tensor": [[4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0]]})
result = mainpipeline.infer(normal_input)
display(result)
time | in.tensor | out.variable | check_failures | |
---|---|---|---|---|
0 | 2024-02-15 16:18:42.466 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.7] | 0 |
large_house_input = pd.DataFrame.from_records({'tensor': [[4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0, 10.0, 2760.0, 950.0, 47.6696, -122.261, 3970.0, 20000.0, 79.0, 0.0, 0.0]]})
large_house_result = mainpipeline.infer(large_house_input)
display(large_house_result)
time | in.tensor | out.variable | check_failures | |
---|---|---|---|---|
0 | 2024-02-15 16:18:42.866 | [4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0, 10.0, 2760.0, 950.0, 47.6696, -122.261, 3970.0, 20000.0, 79.0, 0.0, 0.0] | [1514079.4] | 0 |
Generate Sample Data
Before creating the assays, we must generate data for the assays to build from.
For this example, we will:
- Perform sample inferences based on lower priced houses and use that as our baseline.
- Generate inferences from specific set of high priced houses create inference outputs that will be outside the baseline. This is used in later steps to demonstrate baseline comparison against assay analyses.
Inference Results History Generation
To start the demonstration, we’ll create a baseline of values from houses with small estimated prices and set that as our baseline.
We will save the beginning and end periods of our baseline data to the variables assay_baseline_start
and assay_baseline_end
.
small_houses_inputs = pd.read_json('./data/smallinputs.df.json')
baseline_size = 500
# Where the baseline data will start
assay_baseline_start = datetime.datetime.now()
# These inputs will be random samples of small priced houses. Around 30,000 is a good number
small_houses = small_houses_inputs.sample(baseline_size, replace=True).reset_index(drop=True)
# Wait 60 seconds to set this data apart from the rest
time.sleep(60)
small_results = mainpipeline.infer(small_houses)
# Set the baseline end
assay_baseline_end = datetime.datetime.now()
Generate Numpy Baseline Values
This process generates a numpy array of the inference results used as baseline data in later steps.
# get the numpy values
# set the results to a non-array value
small_results_baseline_df = small_results.copy()
small_results_baseline_df['variable']=small_results['out.variable'].map(lambda x: x[0])
small_results_baseline_df
# set the numpy array
small_results_baseline = small_results_baseline_df['variable'].to_numpy()
Assay Test Data
The following will generate inference data for us to test against the assay baseline. For this, we will add in house data that generate higher house prices than the baseline data we used earlier.
This process should take 6 minutes to generate the historical data we’ll later use in our assays. We store the DateTime assay_window_start
to determine where to start out assay analyses.
# Get a spread of house values
# # Set the start for our assay window period.
assay_window_start = datetime.datetime.now()
time.sleep(65)
inference_size = 1000
# And a spread of large house values
small_houses_inputs = pd.read_json('./data/smallinputs.df.json', orient="records")
small_houses = small_houses_inputs.sample(inference_size, replace=True).reset_index(drop=True)
mainpipeline.infer(small_houses)
time.sleep(65)
# Get a spread of large house values
time.sleep(65)
inference_size = 1000
# And a spread of large house values
big_houses_inputs = pd.read_json('./data/biginputs.df.json', orient="records")
big_houses = big_houses_inputs.sample(inference_size, replace=True).reset_index(drop=True)
mainpipeline.infer(big_houses)
time.sleep(65)
Model Insights via the Wallaroo Dashboard SDK
Assays generated through the Wallaroo SDK can be previewed, configured, and uploaded to the Wallaroo Ops instance. The following is a condensed version of this process. For full details see the Wallaroo SDK Essentials Guide: Assays Management guide.
Model drift detection with assays using the Wallaroo SDK follows this general process.
- Define the Baseline: From either historical inference data for a specific model in a pipeline, or from a pre-determine array of data, a baseline is formed.
- Assay Preview: Once the baseline is formed, we preview the assay and configure the different options until we have the the best method of detecting environment or model drift.
- Create Assay: With the previews and configuration complete, we upload the assay. The assay will perform an analysis on a regular scheduled based on the configuration.
- Get Assay Results: Retrieve the analyses and use them to detect model drift and possible sources.
- Pause/Resume Assay: Pause or restart an assay as needed.
Define the Baseline
Assay baselines are defined with the wallaroo.client.build_assay
method. Through this process we define the baseline from either a range of dates or pre-generated values.
wallaroo.client.build_assay
take the following parameters:
Parameter | Type | Description |
---|---|---|
assay_name | String (Required) - required | The name of the assay. Assay names must be unique across the Wallaroo instance. |
pipeline | wallaroo.pipeline.Pipeline (Required) | The pipeline the assay is monitoring. |
model_name | String (Required) | The name of the model to monitor. |
iopath | String (Required) | The input/output data for the model being tracked in the format input/output field index . Only one value is tracked for any assay. For example, to track the output of the model’s field house_value at index 0 , the iopath is 'output house_value 0 . |
baseline_start | datetime.datetime (Optional) | The start time for the inferences to use as the baseline. Must be included with baseline_end . Cannot be included with baseline_data . |
baseline_end | datetime.datetime (Optional) | The end time of the baseline window. the baseline. Windows start immediately after the baseline window and are run at regular intervals continuously until the assay is deactivated or deleted. Must be included with baseline_start . Cannot be included with baseline_data .. |
baseline_data | numpy.array (Optional) | The baseline data in numpy array format. Cannot be included with either baseline_start or baseline_data . |
Baselines are created in one of two ways:
- Date Range: The
baseline_start
andbaseline_end
retrieves the inference requests and results for the pipeline from the start and end period. This data is summarized and used to create the baseline. - Numpy Values: The
baseline_data
sets the baseline from a provided numpy array.
Define the Baseline Example
This example shows two methods of defining the baseline for an assay:
"assays from date baseline"
: This assay uses historical inference requests to define the baseline. This assay is saved to the variableassay_builder_from_dates
."assays from numpy"
: This assay uses a pre-generated numpy array to define the baseline. This assay is saved to the variableassay_builder_from_numpy
.
In both cases, the following parameters are used:
Parameter | Value |
---|---|
assay_name | "assays from date baseline" and "assays from numpy" |
pipeline | mainpipeline : A pipeline with a ML model that predicts house prices. The output field for this model is variable . |
model_name | "houseprice-predictor" - the model name set during model upload. |
iopath | These assays monitor the model’s output field variable at index 0. From this, the iopath setting is "output variable 0" . |
The difference between the two assays’ parameters determines how the baseline is generated.
"assays from date baseline"
: Uses thebaseline_start
andbaseline_end
to set the time period of inference requests and results to gather data from."assays from numpy"
: Uses a pre-generated numpy array as for the baseline data.
For each of our assays, we will set the time period of inference data to compare against the baseline data.
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
# assay builder by baseline
assay_builder_from_numpy = wl.build_assay(assay_name="assays from numpy",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_data = small_results_baseline)
# set the width, interval, and time period
assay_builder_from_numpy.add_run_until(datetime.datetime.now())
assay_builder_from_numpy.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_numpy = assay_builder_from_numpy.build()
assay_results_from_numpy = assay_config_from_numpy.interactive_run()
Baseline DataFrame
The method wallaroo.assay_config.AssayBuilder.baseline_dataframe
returns a DataFrame of the assay baseline generated from the provided parameters. This includes:
metadata
: The inference metadata with the model information, inference time, and other related factors.in
data: Each input field assigned with the labelin.{input field name}
.out
data: Each output field assigned with the labelout.{output field name}
Note that for assays generated from numpy values, there is only the out
data based on the supplied baseline data.
In the following example, the baseline DataFrame is retrieved.
display(assay_builder_from_dates.baseline_dataframe())
time | metadata | input_tensor_0 | input_tensor_1 | input_tensor_2 | input_tensor_3 | input_tensor_4 | input_tensor_5 | input_tensor_6 | input_tensor_7 | ... | input_tensor_9 | input_tensor_10 | input_tensor_11 | input_tensor_12 | input_tensor_13 | input_tensor_14 | input_tensor_15 | input_tensor_16 | input_tensor_17 | output_variable_0 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 1708013922866 | {'last_model': '{"model_name":"house-price-estimator","model_sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}', 'pipeline_version': '', 'elapsed': [49610, 341497], 'dropped': [], 'partition': 'engine-58b565bf45-29xnm'} | 4.0 | 3.00 | 3710.0 | 20000.0 | 2.0 | 0.0 | 2.0 | 5.0 | ... | 2760.0 | 950.0 | 47.669600 | -122.261000 | 3970.0 | 20000.0 | 79.0 | 0.0 | 0.0 | 1.514079e+06 |
1 | 1708013983808 | {'last_model': '{"model_name":"house-price-estimator","model_sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}', 'pipeline_version': '', 'elapsed': [2517407, 2287534], 'dropped': [], 'partition': 'engine-58b565bf45-29xnm'} | 3.0 | 2.50 | 1500.0 | 7420.0 | 1.0 | 0.0 | 0.0 | 3.0 | ... | 1000.0 | 500.0 | 47.723598 | -122.174004 | 1840.0 | 7272.0 | 42.0 | 0.0 | 0.0 | 4.196772e+05 |
2 | 1708013983808 | {'last_model': '{"model_name":"house-price-estimator","model_sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}', 'pipeline_version': '', 'elapsed': [2517407, 2287534], 'dropped': [], 'partition': 'engine-58b565bf45-29xnm'} | 4.0 | 2.50 | 2009.0 | 5000.0 | 2.0 | 0.0 | 0.0 | 3.0 | ... | 2009.0 | 0.0 | 47.257702 | -122.197998 | 2009.0 | 5182.0 | 0.0 | 0.0 | 0.0 | 3.208637e+05 |
3 | 1708013983808 | {'last_model': '{"model_name":"house-price-estimator","model_sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}', 'pipeline_version': '', 'elapsed': [2517407, 2287534], 'dropped': [], 'partition': 'engine-58b565bf45-29xnm'} | 3.0 | 1.75 | 1530.0 | 7245.0 | 1.0 | 0.0 | 0.0 | 4.0 | ... | 1530.0 | 0.0 | 47.730999 | -122.191002 | 1530.0 | 7490.0 | 31.0 | 0.0 | 0.0 | 4.319292e+05 |
4 | 1708013983808 | {'last_model': '{"model_name":"house-price-estimator","model_sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}', 'pipeline_version': '', 'elapsed': [2517407, 2287534], 'dropped': [], 'partition': 'engine-58b565bf45-29xnm'} | 3.0 | 1.75 | 1480.0 | 4800.0 | 2.0 | 0.0 | 0.0 | 4.0 | ... | 1140.0 | 340.0 | 47.656700 | -122.397003 | 1810.0 | 4800.0 | 70.0 | 0.0 | 0.0 | 5.361757e+05 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
496 | 1708013983808 | {'last_model': '{"model_name":"house-price-estimator","model_sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}', 'pipeline_version': '', 'elapsed': [2517407, 2287534], 'dropped': [], 'partition': 'engine-58b565bf45-29xnm'} | 4.0 | 2.25 | 2560.0 | 12100.0 | 1.0 | 0.0 | 0.0 | 4.0 | ... | 1760.0 | 800.0 | 47.631001 | -122.108002 | 2240.0 | 12100.0 | 38.0 | 0.0 | 0.0 | 7.019407e+05 |
497 | 1708013983808 | {'last_model': '{"model_name":"house-price-estimator","model_sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}', 'pipeline_version': '', 'elapsed': [2517407, 2287534], 'dropped': [], 'partition': 'engine-58b565bf45-29xnm'} | 2.0 | 1.00 | 1160.0 | 5000.0 | 1.0 | 0.0 | 0.0 | 4.0 | ... | 1160.0 | 0.0 | 47.686501 | -122.399002 | 1750.0 | 5000.0 | 77.0 | 0.0 | 0.0 | 4.508677e+05 |
498 | 1708013983808 | {'last_model': '{"model_name":"house-price-estimator","model_sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}', 'pipeline_version': '', 'elapsed': [2517407, 2287534], 'dropped': [], 'partition': 'engine-58b565bf45-29xnm'} | 4.0 | 2.50 | 1910.0 | 5000.0 | 2.0 | 0.0 | 0.0 | 3.0 | ... | 1910.0 | 0.0 | 47.360802 | -122.036003 | 2020.0 | 5000.0 | 9.0 | 0.0 | 0.0 | 2.962027e+05 |
499 | 1708013983808 | {'last_model': '{"model_name":"house-price-estimator","model_sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}', 'pipeline_version': '', 'elapsed': [2517407, 2287534], 'dropped': [], 'partition': 'engine-58b565bf45-29xnm'} | 3.0 | 1.50 | 1590.0 | 8911.0 | 1.0 | 0.0 | 0.0 | 3.0 | ... | 1590.0 | 0.0 | 47.739399 | -122.251999 | 1590.0 | 9625.0 | 58.0 | 0.0 | 0.0 | 4.371780e+05 |
500 | 1708013983808 | {'last_model': '{"model_name":"house-price-estimator","model_sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}', 'pipeline_version': '', 'elapsed': [2517407, 2287534], 'dropped': [], 'partition': 'engine-58b565bf45-29xnm'} | 4.0 | 2.75 | 2640.0 | 4000.0 | 2.0 | 0.0 | 0.0 | 5.0 | ... | 1730.0 | 910.0 | 47.672699 | -122.296997 | 1530.0 | 3740.0 | 89.0 | 0.0 | 0.0 | 7.184457e+05 |
501 rows × 21 columns
assay_builder_from_numpy.baseline_dataframe()
output_variable_0 | |
---|---|
0 | 419677.20 |
1 | 320863.72 |
2 | 431929.20 |
3 | 536175.70 |
4 | 343304.63 |
... | ... |
495 | 701940.70 |
496 | 450867.70 |
497 | 296202.70 |
498 | 437177.97 |
499 | 718445.70 |
500 rows × 1 columns
Baseline Stats
The method wallaroo.assay.AssayAnalysis.baseline_stats()
returns a pandas.core.frame.DataFrame
of the baseline stats.
The baseline stats for each assay are displayed in the examples below.
assay_results_from_dates[0].baseline_stats()
Baseline | |
---|---|
count | 501 |
min | 236238.671875 |
max | 1514079.375 |
mean | 495193.231786 |
median | 442168.125 |
std | 226075.814267 |
start | None |
end | None |
assay_results_from_numpy[0].baseline_stats()
Baseline | |
---|---|
count | 500 |
min | 236238.67 |
max | 1489624.3 |
mean | 493155.46054 |
median | 441840.425 |
std | 221657.583536 |
start | None |
end | None |
Baseline Bins
The method wallaroo.assay.AssayAnalysis.baseline_bins
a simple dataframe to with the edge/bin data for a baseline.
assay_results_from_dates[0].baseline_bins()
b_edges | b_edge_names | b_aggregated_values | b_aggregation | |
---|---|---|---|---|
0 | 2.362387e+05 | left_outlier | 0.000000 | Density |
1 | 2.962027e+05 | q_20 | 0.203593 | Density |
2 | 4.159643e+05 | q_40 | 0.195609 | Density |
3 | 4.640602e+05 | q_60 | 0.203593 | Density |
4 | 6.821819e+05 | q_80 | 0.197605 | Density |
5 | 1.514079e+06 | q_100 | 0.199601 | Density |
6 | inf | right_outlier | 0.000000 | Density |
assay_results_from_numpy[0].baseline_bins()
b_edges | b_edge_names | b_aggregated_values | b_aggregation | |
---|---|---|---|---|
0 | 236238.67 | left_outlier | 0.000 | Density |
1 | 296202.70 | q_20 | 0.204 | Density |
2 | 415964.30 | q_40 | 0.196 | Density |
3 | 464057.38 | q_60 | 0.200 | Density |
4 | 675545.44 | q_80 | 0.200 | Density |
5 | 1489624.30 | q_100 | 0.200 | Density |
6 | inf | right_outlier | 0.000 | Density |
Baseline Histogram Chart
The method wallaroo.assay_config.AssayBuilder.baseline_histogram
returns a histogram chart of the assay baseline generated from the provided parameters.
assay_builder_from_dates.baseline_histogram()
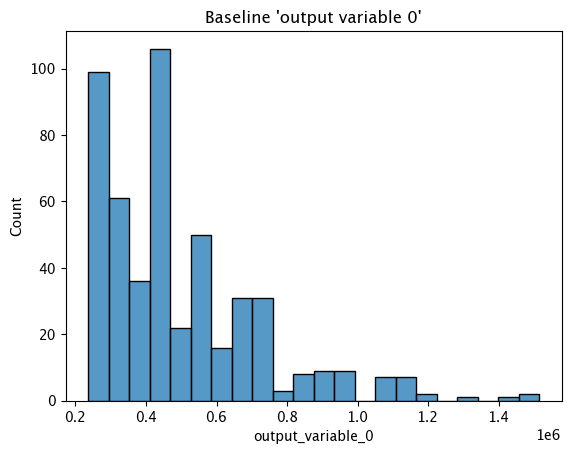
Baseline KDE Chart
The method wallaroo.assay_config.AssayBuilder.baseline_kde
returns a Kernel Density Estimation (KDE) chart of the assay baseline generated from the provided parameters.
assay_builder_from_dates.baseline_kde()
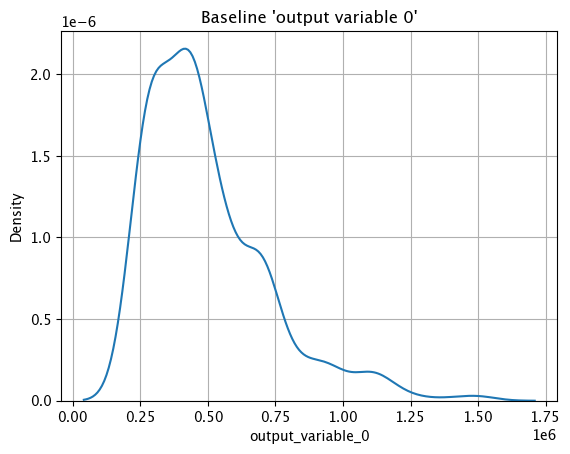
Baseline ECDF Chart
The method wallaroo.assay_config.AssayBuilder.baseline_ecdf
returns a Empirical Cumulative Distribution Function (CDF) chart of the assay baseline generated from the provided parameters.
assay_builder_from_dates.baseline_ecdf()
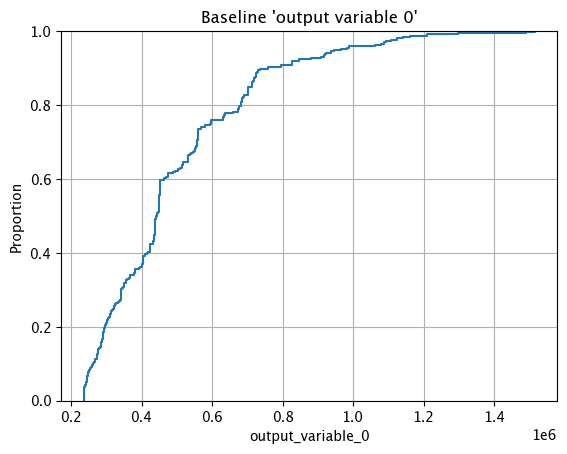
Assay Preview
Now that the baseline is defined, we look at different configuration options and view how the assay baseline and results changes. Once we determine what gives us the best method of determining model drift, we can create the assay.
Analysis List Chart Scores
Analysis List scores show the assay scores for each assay result interval in one chart. Values that are outside of the alert threshold are colored red, while scores within the alert threshold are green.
Assay chart scores are displayed with the method wallaroo.assay.AssayAnalysisList.chart_scores(title: Optional[str] = None)
, with ability to display an optional title with the chart.
The following example shows retrieving the assay results and displaying the chart scores. From our example, we have two windows - the first should be green, and the second is red showing that values were outside the alert threshold.
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates.chart_scores()
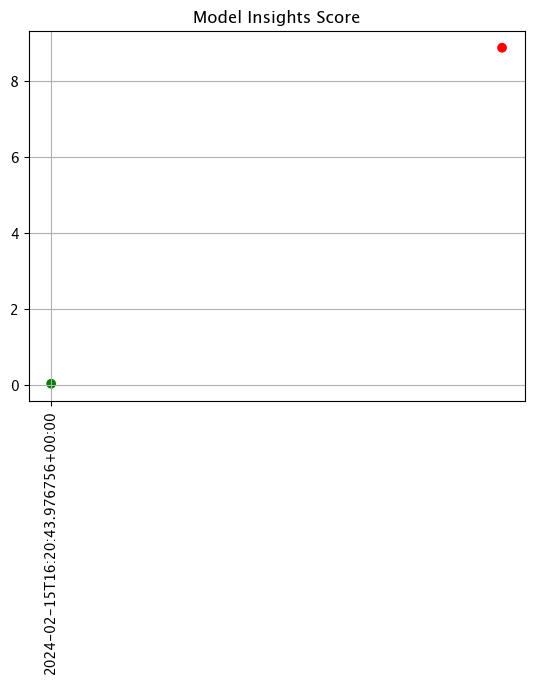
Analysis Chart
The method wallaroo.assay.AssayAnalysis.chart()
displays a comparison between the baseline and an interval of inference data.
This is compared to the Chart Scores, which is a list of all of the inference data split into intervals, while the Analysis Chart shows the breakdown of one set of inference data against the baseline.
Score from the Analysis List Chart Scores and each element from the Analysis List DataFrame generates
The following fields are included.
Field | Type | Description |
---|---|---|
baseline mean | Float | The mean of the baseline values. |
window mean | Float | The mean of the window values. |
baseline median | Float | The median of the baseline values. |
window median | Float | The median of the window values. |
bin_mode | String | The binning mode used for the assay. |
aggregation | String | The aggregation mode used for the assay. |
metric | String | The metric mode used for the assay. |
weighted | Bool | Whether the bins were manually weighted. |
score | Float | The score from the assay window. |
scores | List(Float) | The score from each assay window bin. |
index | Integer/None | The window index. Interactive assay runs are None . |
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates[0].chart()
baseline mean = 495193.23178642715
window mean = 517763.394625
baseline median = 442168.125
window median = 448627.8125
bin_mode = Quantile
aggregation = Density
metric = PSI
weighted = False
score = 0.0363497101644573
scores = [0.0, 0.027271477163285655, 0.003847844548034077, 0.000217023993714693, 0.002199485350158766, 0.0028138791092641195, 0.0]
index = None
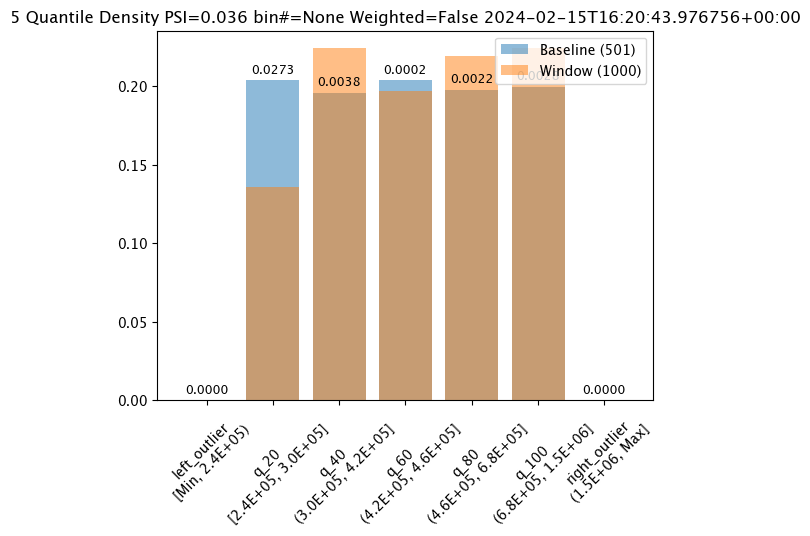
Analysis List DataFrame
wallaroo.assay.AssayAnalysisList.to_dataframe()
returns a DataFrame showing the assay results for each window aka individual analysis. This DataFrame contains the following fields:
Field | Type | Description |
---|---|---|
assay_id | Integer/None | The assay id. Only provided from uploaded and executed assays. |
name | String/None | The name of the assay. Only provided from uploaded and executed assays. |
iopath | String/None | The iopath of the assay. Only provided from uploaded and executed assays. |
score | Float | The assay score. |
start | DateTime | The DateTime start of the assay window. |
min | Float | The minimum value in the assay window. |
max | Float | The maximum value in the assay window. |
mean | Float | The mean value in the assay window. |
median | Float | The median value in the assay window. |
std | Float | The standard deviation value in the assay window. |
warning_threshold | Float/None | The warning threshold of the assay window. |
alert_threshold | Float/None | The alert threshold of the assay window. |
status | String | The assay window status. Values are:
|
For this example, the assay analysis list DataFrame is listed.
From this tutorial, we should have 2 windows of dta to look at, each one minute apart. The first window should show status: OK
, with the second window with the very large house prices will show status: alert
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates.to_dataframe()
assay_id | name | iopath | score | start | min | max | mean | median | std | warning_threshold | alert_threshold | status | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | None | 0.036350 | 2024-02-15T16:20:43.976756+00:00 | 2.362387e+05 | 1489624.250 | 5.177634e+05 | 4.486278e+05 | 227729.030050 | None | 0.25 | Ok | ||
1 | None | 8.868614 | 2024-02-15T16:22:43.976756+00:00 | 1.514079e+06 | 2016006.125 | 1.885772e+06 | 1.946438e+06 | 160046.727324 | None | 0.25 | Alert |
Analysis List Full DataFrame
wallaroo.assay.AssayAnalysisList.to_full_dataframe()
returns a DataFrame showing all values, including the inputs and outputs from the assay results for each window aka individual analysis. This DataFrame contains the following fields:
pipeline_id warning_threshold bin_index created_at
Field | Type | Description |
---|---|---|
window_start | DateTime | The date and time when the window period began. |
analyzed_at | DateTime | The date and time when the assay analysis was performed. |
elapsed_millis | Integer | How long the analysis took to perform in milliseconds. |
baseline_summary_count | Integer | The number of data elements from the baseline. |
baseline_summary_min | Float | The minimum value from the baseline summary. |
baseline_summary_max | Float | The maximum value from the baseline summary. |
baseline_summary_mean | Float | The mean value of the baseline summary. |
baseline_summary_median | Float | The median value of the baseline summary. |
baseline_summary_std | Float | The standard deviation value of the baseline summary. |
baseline_summary_edges_{0…n} | Float | The baseline summary edges for each baseline edge from 0 to number of edges. |
summarizer_type | String | The type of summarizer used for the baseline. See wallaroo.assay_config for other summarizer types. |
summarizer_bin_weights | List / None | If baseline bin weights were provided, the list of those weights. Otherwise, None . |
summarizer_provided_edges | List / None | If baseline bin edges were provided, the list of those edges. Otherwise, None . |
status | String | The assay window status. Values are:
|
id | Integer/None | The id for the window aka analysis. Only provided from uploaded and executed assays. |
assay_id | Integer/None | The assay id. Only provided from uploaded and executed assays. |
pipeline_id | Integer/None | The pipeline id. Only provided from uploaded and executed assays. |
warning_threshold | Float | The warning threshold set for the assay. |
warning_threshold | Float | The warning threshold set for the assay. |
bin_index | Integer/None | The bin index for the window aka analysis. |
created_at | Datetime/None | The date and time the window aka analysis was generated. Only provided from uploaded and executed assays. |
For this example, full DataFrame from an assay preview is generated.
From this tutorial, we should have 2 windows of dta to look at, each one minute apart. The first window should show status: OK
, with the second window with the very large house prices will show status: alert
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates.to_full_dataframe()
window_start | analyzed_at | elapsed_millis | baseline_summary_count | baseline_summary_min | baseline_summary_max | baseline_summary_mean | baseline_summary_median | baseline_summary_std | baseline_summary_edges_0 | ... | summarizer_type | summarizer_bin_weights | summarizer_provided_edges | status | id | assay_id | pipeline_id | warning_threshold | bin_index | created_at | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 2024-02-15T16:20:43.976756+00:00 | 2024-02-15T16:26:42.266029+00:00 | 82 | 501 | 236238.671875 | 1514079.375 | 495193.231786 | 442168.125 | 226075.814267 | 236238.671875 | ... | UnivariateContinuous | None | None | Ok | None | None | None | None | None | None |
1 | 2024-02-15T16:22:43.976756+00:00 | 2024-02-15T16:26:42.266134+00:00 | 83 | 501 | 236238.671875 | 1514079.375 | 495193.231786 | 442168.125 | 226075.814267 | 236238.671875 | ... | UnivariateContinuous | None | None | Alert | None | None | None | None | None | None |
2 rows × 86 columns
Analysis Compare Basic Stats
The method wallaroo.assay.AssayAnalysis.compare_basic_stats
returns a DataFrame comparing one set of inference data against the baseline.
This is compared to the Analysis List DataFrame, which is a list of all of the inference data split into intervals, while the Analysis Compare Basic Stats shows the breakdown of one set of inference data against the baseline.
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates[0].compare_basic_stats()
Baseline | Window | diff | pct_diff | |
---|---|---|---|---|
count | 501.0 | 1000.0 | 499.000000 | 99.600798 |
min | 236238.671875 | 236238.671875 | 0.000000 | 0.000000 |
max | 1514079.375 | 1489624.25 | -24455.125000 | -1.615181 |
mean | 495193.231786 | 517763.394625 | 22570.162839 | 4.557850 |
median | 442168.125 | 448627.8125 | 6459.687500 | 1.460912 |
std | 226075.814267 | 227729.03005 | 1653.215783 | 0.731266 |
start | None | 2024-02-15T16:20:43.976756+00:00 | NaN | NaN |
end | None | 2024-02-15T16:21:43.976756+00:00 | NaN | NaN |
Configure Assays
Before creating the assay, configure the assay and continue to preview it until the best method for detecting drift is set. The following options are available.
Score Metric
The score
is a distance between the baseline and the analysis window. The larger the score, the greater the difference between the baseline and the analysis window. The following methods are provided determining the score:
PSI
(Default) - Population Stability Index (PSI).MAXDIFF
: Maximum difference between corresponding bins.SUMDIFF
: Mum of differences between corresponding bins.
The metric type used is updated with the wallaroo.assay_config.AssayBuilder.add_metric(metric: wallaroo.assay_config.Metric)
method.
The following three charts use each of the metrics. Note how the scores change based on the score type used.
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# set metric PSI mode
assay_builder_from_dates.summarizer_builder.add_metric(wallaroo.assay_config.Metric.PSI)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates[0].chart()
baseline mean = 495193.23178642715
window mean = 517763.394625
baseline median = 442168.125
window median = 448627.8125
bin_mode = Quantile
aggregation = Density
metric = PSI
weighted = False
score = 0.0363497101644573
scores = [0.0, 0.027271477163285655, 0.003847844548034077, 0.000217023993714693, 0.002199485350158766, 0.0028138791092641195, 0.0]
index = None
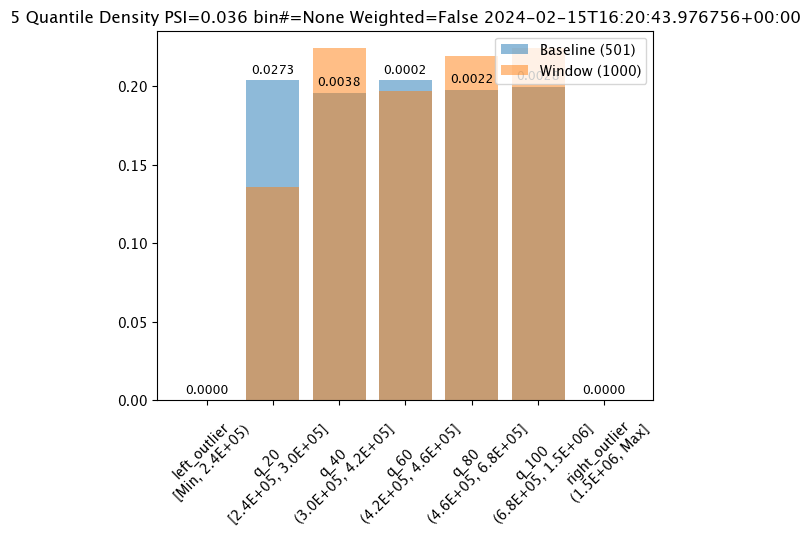
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# set metric MAXDIFF mode
assay_builder_from_dates.summarizer_builder.add_metric(wallaroo.assay_config.Metric.MAXDIFF)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates[0].chart()
baseline mean = 495193.23178642715
window mean = 517763.394625
baseline median = 442168.125
window median = 448627.8125
bin_mode = Quantile
aggregation = Density
metric = MaxDiff
weighted = False
score = 0.06759281437125747
scores = [0.0, 0.06759281437125747, 0.028391217564870255, 0.006592814371257472, 0.02139520958083832, 0.02439920159680639, 0.0]
index = 1
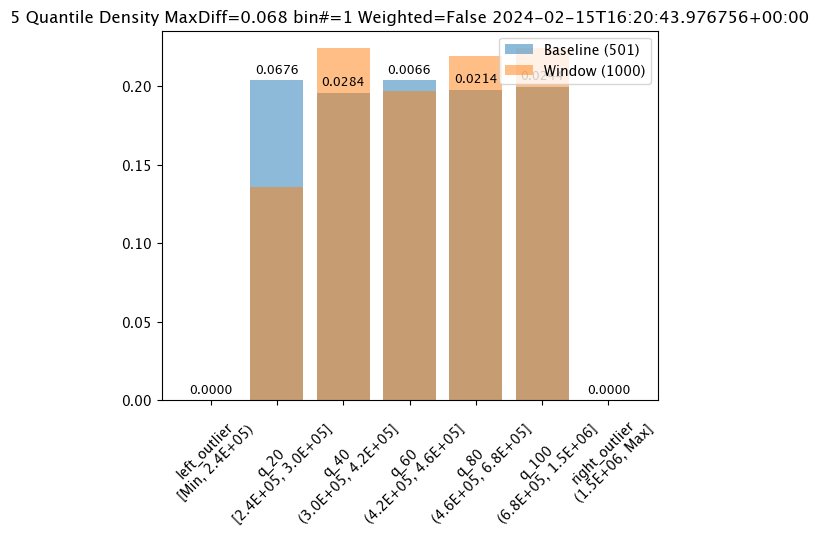
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# set metric SUMDIFF mode
assay_builder_from_dates.summarizer_builder.add_metric(wallaroo.assay_config.Metric.SUMDIFF)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates[0].chart()
baseline mean = 495193.23178642715
window mean = 517763.394625
baseline median = 442168.125
window median = 448627.8125
bin_mode = Quantile
aggregation = Density
metric = SumDiff
weighted = False
score = 0.07418562874251496
scores = [0.0, 0.06759281437125747, 0.028391217564870255, 0.006592814371257472, 0.02139520958083832, 0.02439920159680639, 0.0]
index = None
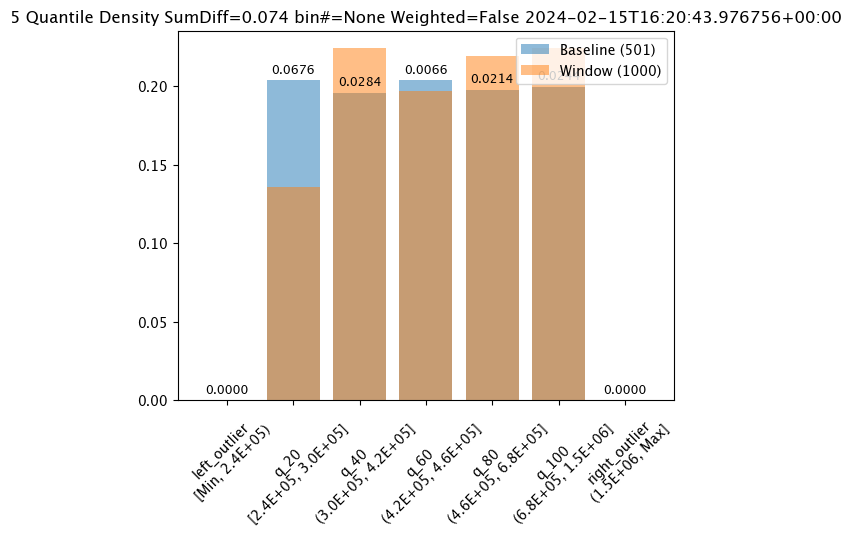
Alert Threshold
Assay alert thresholds are modified with the wallaroo.assay_config.AssayBuilder.add_alert_threshold(alert_threshold: float)
method. By default alert thresholds are 0.1
.
The following example updates the alert threshold to 0.5
.
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
assay_builder_from_dates.add_alert_threshold(0.5)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates.to_dataframe()
assay_id | name | iopath | score | start | min | max | mean | median | std | warning_threshold | alert_threshold | status | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | None | 0.036350 | 2024-02-15T16:20:43.976756+00:00 | 2.362387e+05 | 1489624.250 | 5.177634e+05 | 4.486278e+05 | 227729.030050 | None | 0.5 | Ok | ||
1 | None | 8.868614 | 2024-02-15T16:22:43.976756+00:00 | 1.514079e+06 | 2016006.125 | 1.885772e+06 | 1.946438e+06 | 160046.727324 | None | 0.5 | Alert |
Number of Bins
Number of bins sets how the baseline data is partitioned. The total number of bins includes the set number plus the left_outlier and the right_outlier, so the total number of bins will be the total set + 2.
The number of bins is set with the wallaroo.assay_config.UnivariateContinousSummarizerBuilder.add_num_bins(num_bins: int)
method.
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# Set the number of bins
# update number of bins here
assay_builder_from_dates.summarizer_builder.add_num_bins(10)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates[0].chart()
baseline mean = 495193.23178642715
window mean = 517763.394625
baseline median = 442168.125
window median = 448627.8125
bin_mode = Quantile
aggregation = Density
metric = PSI
weighted = False
score = 0.05250979748389363
scores = [0.0, 0.009076998929542533, 0.01924002322223739, 0.0021945246367443406, 0.0016700458183385653, 0.005779503770625584, 0.002393429678215835, 0.002942858220315506, 0.00010651192741915124, 0.00046961759334670583, 0.008636283687108028, 0.0]
index = None
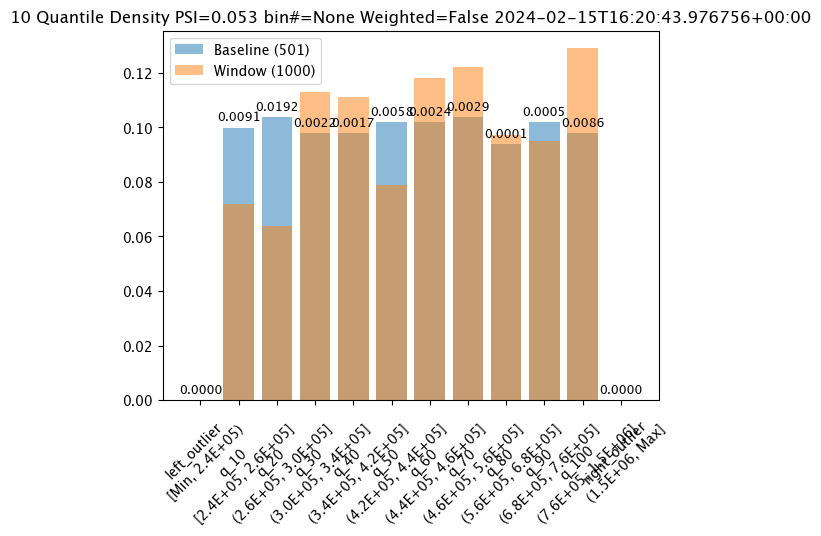
Binning Mode
Binning Mode defines how the bins are separated. Binning modes are modified through the wallaroo.assay_config.UnivariateContinousSummarizerBuilder.add_bin_mode(bin_mode: bin_mode: wallaroo.assay_config.BinMode, edges: Optional[List[float]] = None)
.
Available bin_mode
values from wallaroo.assay_config.Binmode
are the following:
QUANTILE
(Default): Based on percentages. Ifnum_bins
is 5 then quintiles so bins are created at the 20%, 40%, 60%, 80% and 100% points.EQUAL
: Evenly spaced bins where each bin is set with the formulamin - max / num_bins
PROVIDED
: The user provides the edge points for the bins.
If PROVIDED
is supplied, then a List of float values must be provided for the edges
parameter that matches the number of bins.
The following examples are used to show how each of the binning modes effects the bins.
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# update binning mode here
assay_builder_from_dates.summarizer_builder.add_bin_mode(wallaroo.assay_config.BinMode.QUANTILE)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates[0].chart()
baseline mean = 495193.23178642715
window mean = 517763.394625
baseline median = 442168.125
window median = 448627.8125
bin_mode = Quantile
aggregation = Density
metric = PSI
weighted = False
score = 0.0363497101644573
scores = [0.0, 0.027271477163285655, 0.003847844548034077, 0.000217023993714693, 0.002199485350158766, 0.0028138791092641195, 0.0]
index = None
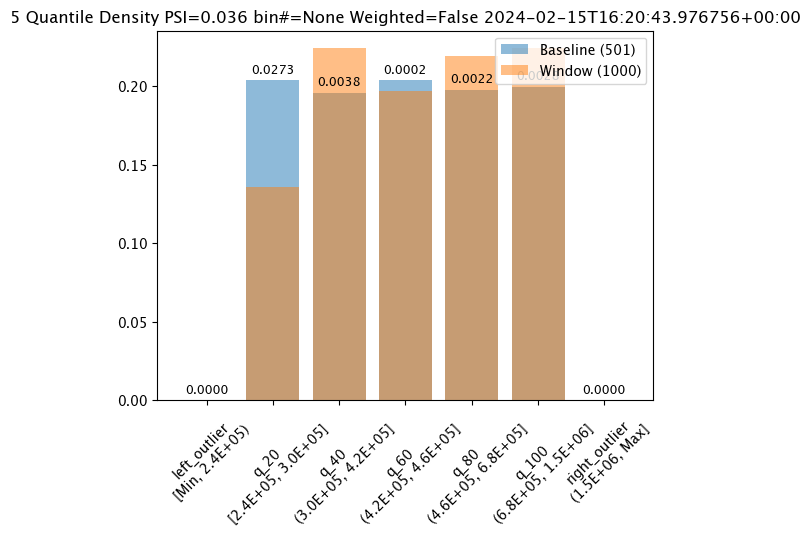
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# update binning mode here
assay_builder_from_dates.summarizer_builder.add_bin_mode(wallaroo.assay_config.BinMode.EQUAL)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates[0].chart()
baseline mean = 495193.23178642715
window mean = 517763.394625
baseline median = 442168.125
window median = 448627.8125
bin_mode = Equal
aggregation = Density
metric = PSI
weighted = False
score = 0.013362603453760629
scores = [0.0, 0.0016737762070682225, 1.1166481947075492e-06, 0.011233704798893194, 1.276169365380064e-07, 0.00045387818266796784, 0.0]
index = None
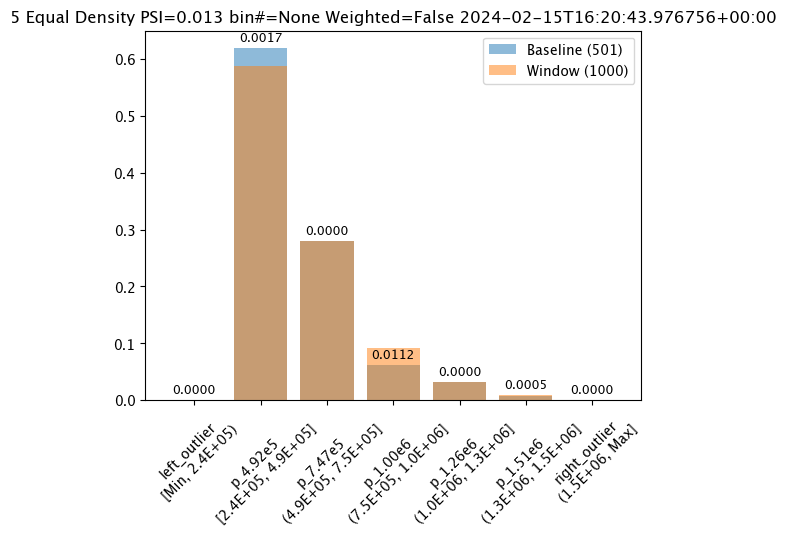
The following example manually sets the bin values.
The values in this dataset run from 200000 to 1500000. We can specify the bins with the BinMode.PROVIDED
and specifying a list of floats with the right hand / upper edge of each bin and optionally the lower edge of the smallest bin. If the lowest edge is not specified the threshold for left outliers is taken from the smallest value in the baseline dataset.
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
edges = [200000.0, 400000.0, 600000.0, 800000.0, 1500000.0, 2000000.0]
# update binning mode here
assay_builder_from_dates.summarizer_builder.add_bin_mode(wallaroo.assay_config.BinMode.PROVIDED, edges)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates[0].chart()
baseline mean = 495193.23178642715
window mean = 517763.394625
baseline median = 442168.125
window median = 448627.8125
bin_mode = Provided
aggregation = Density
metric = PSI
weighted = False
score = 0.01005936099521711
scores = [0.0, 0.0030207963288415803, 0.00011480201840874194, 0.00045327555974347976, 0.0037119550613212583, 0.0027585320269020493, 0.0]
index = None
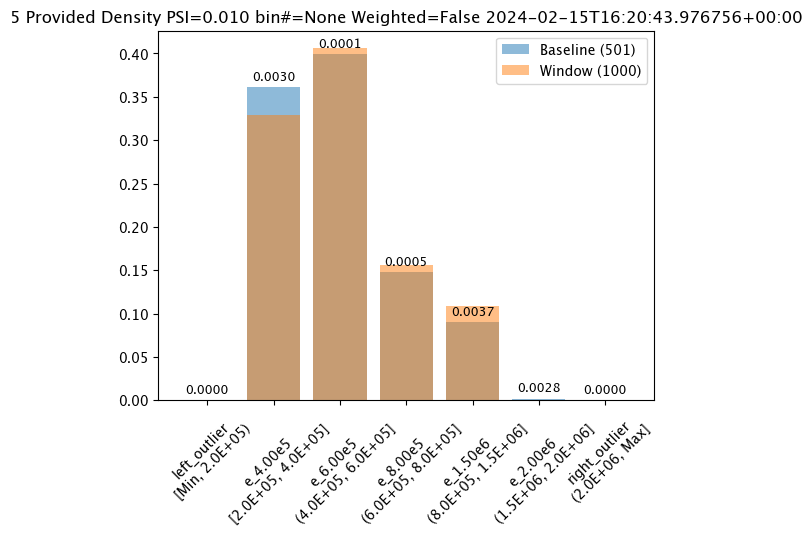
Aggregation Options
Assay aggregation options are modified with the wallaroo.assay_config.AssayBuilder.add_aggregation(aggregation: wallaroo.assay_config.Aggregation)
method. The following options are provided:
Aggregation.DENSITY
(Default): Count the number/percentage of values that fall in each bin.Aggregation.CUMULATIVE
: Empirical Cumulative Density Function style, which keeps a cumulative count of the values/percentages that fall in each bin.
The following example demonstrate the different results between the two.
#Aggregation.DENSITY - the default
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
assay_builder_from_dates.summarizer_builder.add_aggregation(wallaroo.assay_config.Aggregation.DENSITY)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates[0].chart()
baseline mean = 495193.23178642715
window mean = 517763.394625
baseline median = 442168.125
window median = 448627.8125
bin_mode = Quantile
aggregation = Density
metric = PSI
weighted = False
score = 0.0363497101644573
scores = [0.0, 0.027271477163285655, 0.003847844548034077, 0.000217023993714693, 0.002199485350158766, 0.0028138791092641195, 0.0]
index = None
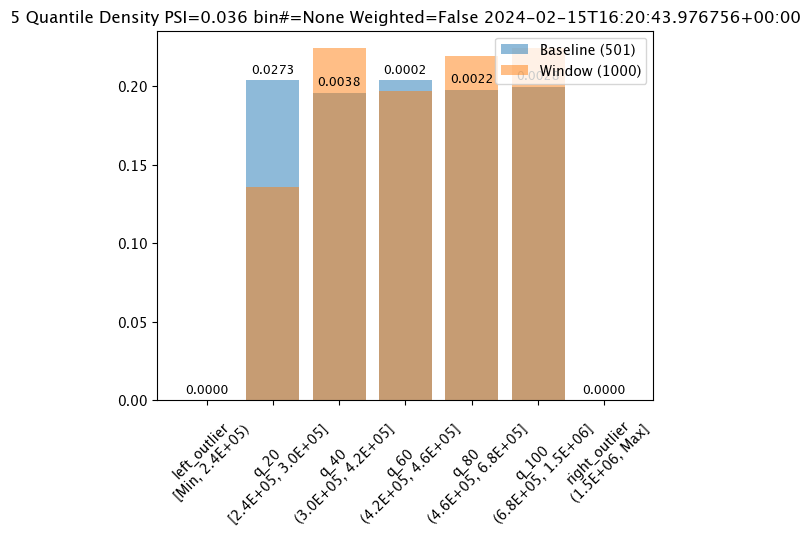
#Aggregation.CUMULATIVE
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
assay_builder_from_dates.summarizer_builder.add_aggregation(wallaroo.assay_config.Aggregation.CUMULATIVE)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates[0].chart()
baseline mean = 495193.23178642715
window mean = 517763.394625
baseline median = 442168.125
window median = 448627.8125
bin_mode = Quantile
aggregation = Cumulative
metric = PSI
weighted = False
score = 0.17698802395209584
scores = [0.0, 0.06759281437125747, 0.03920159680638724, 0.04579441117764471, 0.02439920159680642, 0.0, 0.0]
index = None
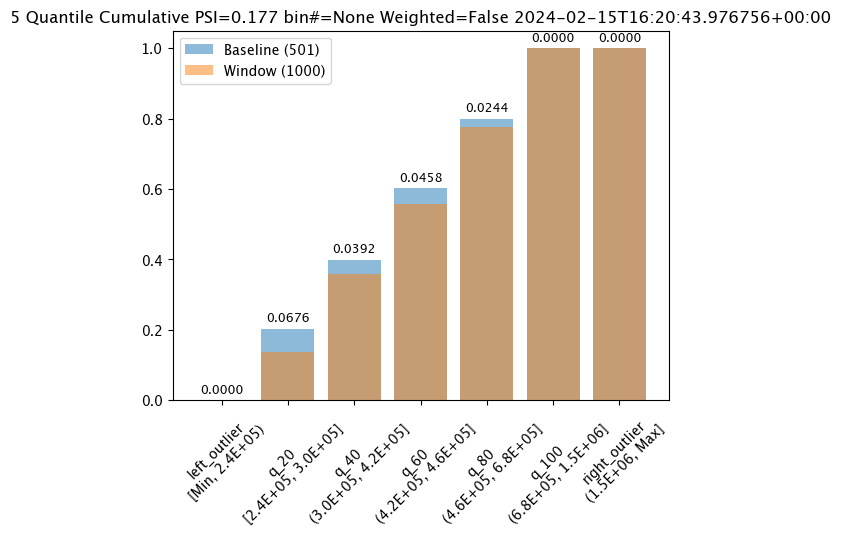
Inference Interval and Inference Width
The inference interval aka window interval sets how often to run the assay analysis. This is set from the wallaroo.assay_config.AssayBuilder.window_builder.add_interval
method to collect data expressed in time units: “hours=24”, “minutes=1”, etc.
For example, with an interval of 1 minute, the assay collects data every minute. Within an hour, 60 intervals of data is collected.
We can adjust the interval and see how the assays change based on how frequently they are run.
The width sets the time period from the wallaroo.assay_config.AssayBuilder.window_builder.add_width
method to collect data expressed in time units: “hours=24”, “minutes=1”, etc.
For example, an interval of 1 minute and a width of 1 minute collects 1 minutes worth of data every minute. An interval of 1 minute with a width of 5 minutes collects 5 minute of inference data every minute.
By default, the interval and width is 24 hours.
For this example, we’ll adjust the width and interval from 1 minute to 5 minutes and see how the number of analyses and their score changes.
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates.chart_scores()
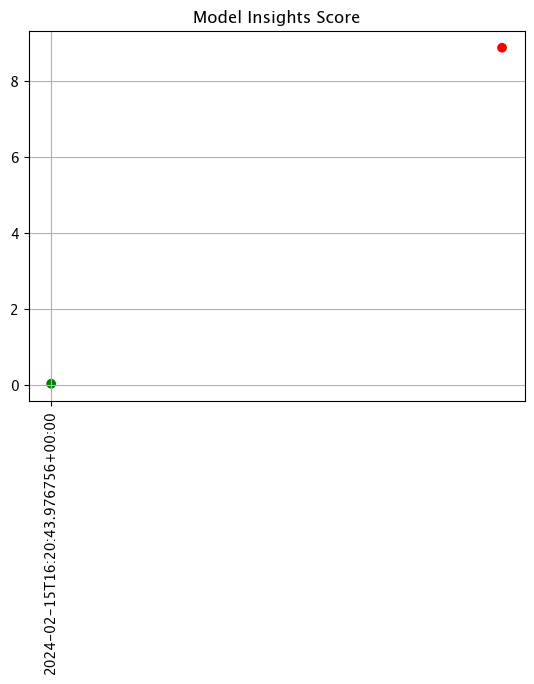
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=5).add_interval(minutes=5).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates.chart_scores()
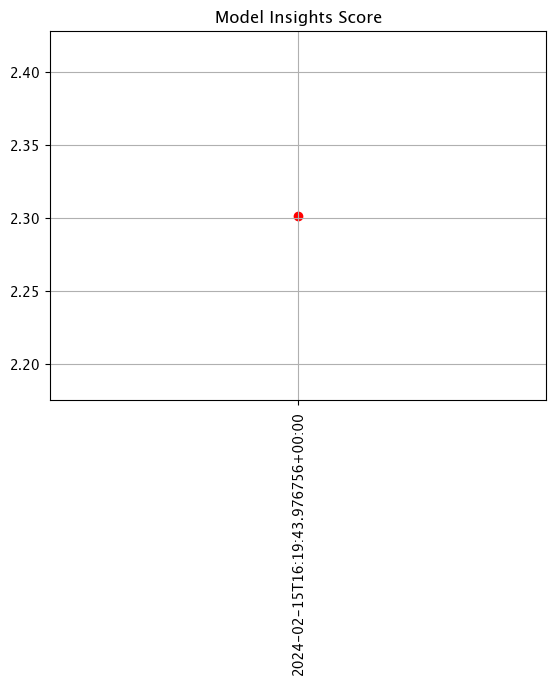
Add Run Until and Add Inference Start
For previewing assays, setting wallaroo.assay_config.AssayBuilder.add_run_until
sets the end date and time for collecting inference data. When an assay is uploaded, this setting is no longer valid - assays run at the Inference Interval until the assay is paused.
Setting the wallaroo.assay_config.WindowBuilder.add_start
sets the start date and time to collect inference data. When an assay is uploaded, this setting is included, and assay results will be displayed starting from that start date at the Inference Interval until the assay is paused. By default, add_start
begins 24 hours after the assay is uploaded unless set in the assay configuration manually.
For the following example, the add_run_until
setting is set to datetime.datetime.now()
to collect all inference data from assay_window_start
up until now, and the second example limits that example to only two minutes of data.
# inference data that includes all of the data until now
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(datetime.datetime.now())
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates.chart_scores()
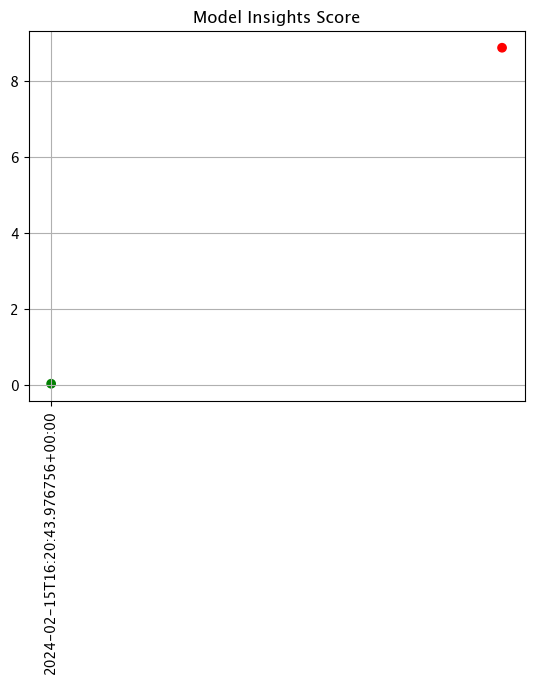
# inference data that includes all of the data until now
assay_builder_from_dates = wl.build_assay(assay_name="assays from date baseline",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# set the width, interval, and time period
assay_builder_from_dates.add_run_until(assay_window_start+datetime.timedelta(seconds=120))
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_dates.build()
assay_results_from_dates = assay_config_from_dates.interactive_run()
assay_results_from_dates.chart_scores()
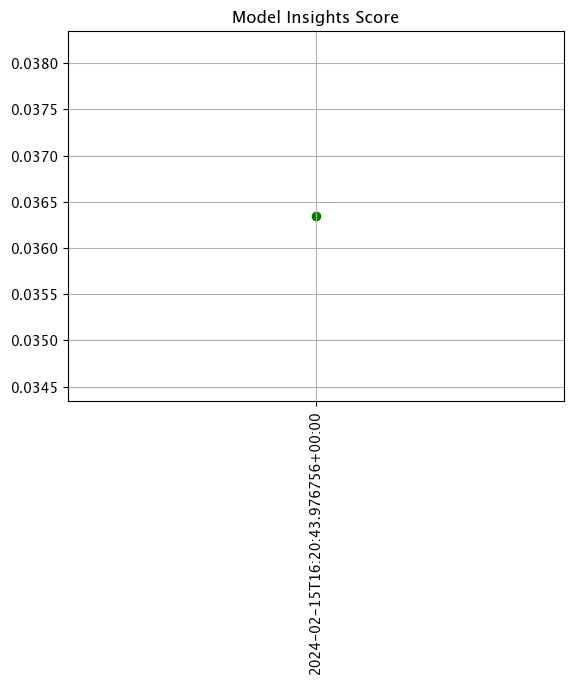
Create Assay
With the assay previewed and configuration options determined, we officially create it by uploading it to the Wallaroo instance.
Once it is uploaded, the assay runs an analysis based on the window width, interval, and the other settings configured.
Assays are uploaded with the wallaroo.assay_config.upload()
method. This uploads the assay into the Wallaroo database with the configurations applied and returns the assay id. Note that assay names must be unique across the Wallaroo instance; attempting to upload an assay with the same name as an existing one will return an error.
wallaroo.assay_config.upload()
returns the assay id for the assay.
Typically we would just call wallaroo.assay_config.upload()
after configuring the assay. For the example below, we will perform the complete configuration in one window to show all of the configuration steps at once before creating the assay.
# Build the assay, based on the start and end of our baseline time,
# and tracking the output variable index 0
assay_builder_from_dates = wl.build_assay(assay_name="assays creation example",
pipeline=mainpipeline,
model_name="house-price-estimator",
iopath="output variable 0",
baseline_start=assay_baseline_start,
baseline_end=assay_baseline_end)
# set the width, interval, and assay start date and time
assay_builder_from_dates.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
# add other options
assay_builder_from_dates.summarizer_builder.add_aggregation(wallaroo.assay_config.Aggregation.CUMULATIVE)
assay_builder_from_dates.summarizer_builder.add_metric(wallaroo.assay_config.Metric.MAXDIFF)
assay_builder_from_dates.add_alert_threshold(0.5)
assay_id = assay_builder_from_dates.upload()
# wait 65 seconds for the first analysis run performed
time.sleep(65)
The assay is now visible through the Wallaroo UI by selecting the workspace, then the pipeline, then Insights.
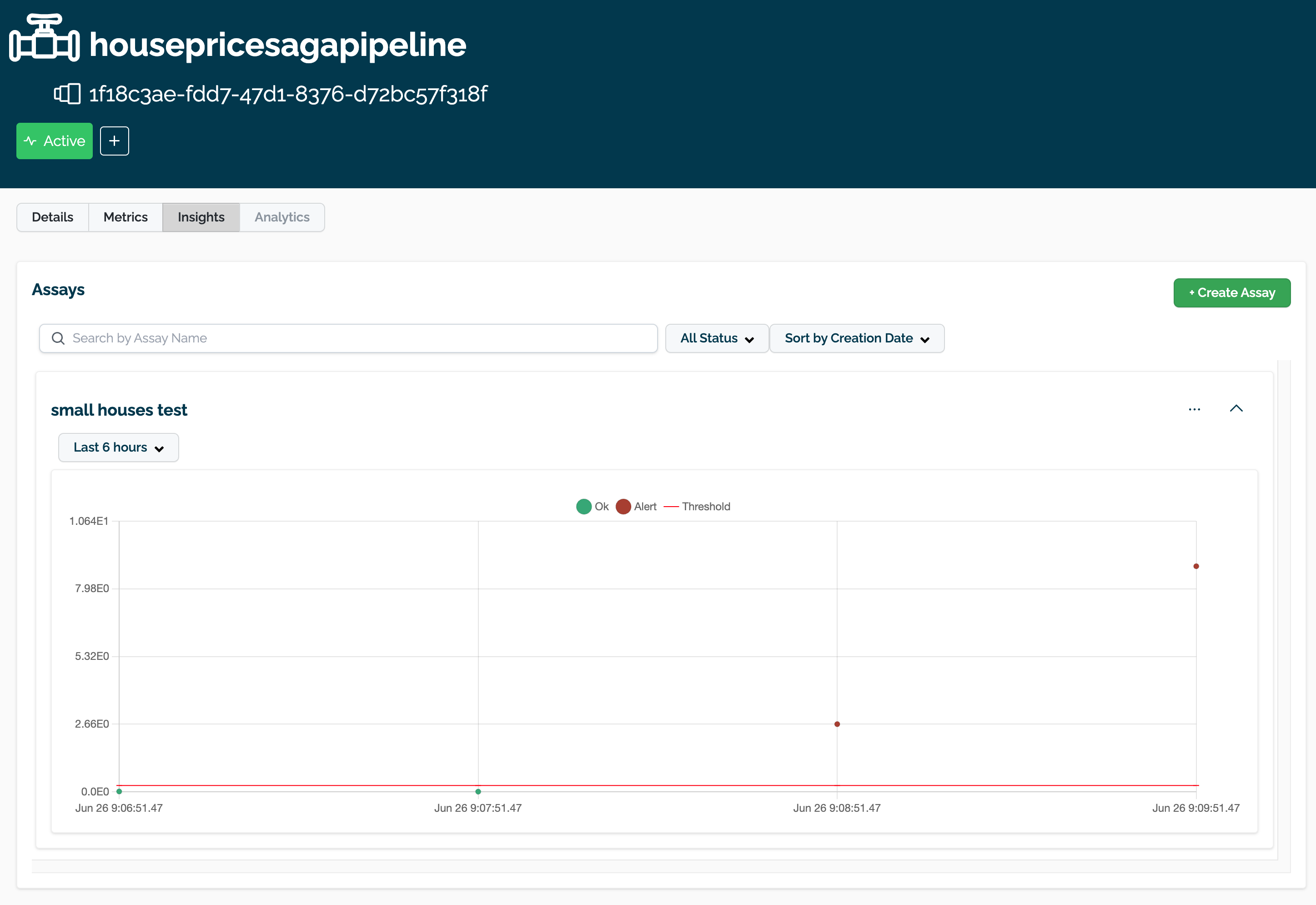
Get Assay Results
Once an assay is created the assay runs an analysis based on the window width, interval, and the other settings configured.
Assay results are retrieved with the wallaroo.client.get_assay_results
method, which takes the following parameters:
Parameter | Type | Description |
---|---|---|
assay_id | Integer (Required) | The numerical id of the assay. |
start | Datetime.Datetime (Required) | The start date and time of historical data from the pipeline to start analyses from. |
end | Datetime.Datetime (Required) | The end date and time of historical data from the pipeline to limit analyses to. |
- IMPORTANT NOTE: This process requires that additional historical data is generated from the time the assay is created to when the results are available. To add additional inference data, use the Assay Test Data section above.
assay_results = wl.get_assay_results(assay_id=assay_id,
start=assay_window_start,
end=datetime.datetime.now())
assay_results.chart_scores()
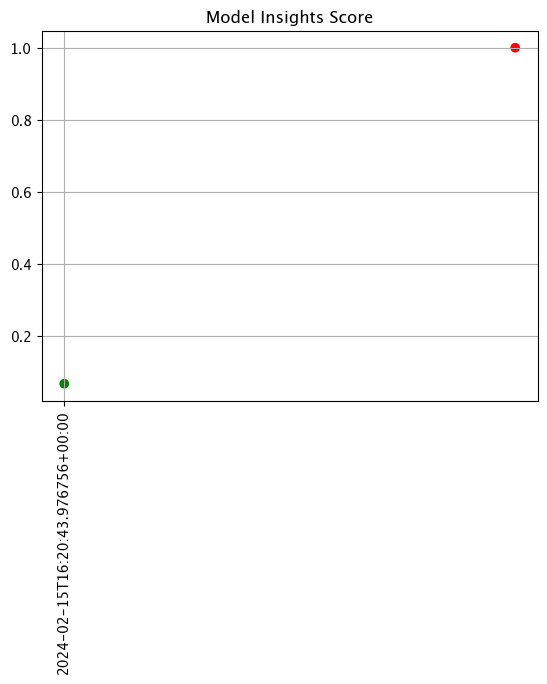
assay_results[0].chart()
baseline mean = 495193.23178642715
window mean = 517763.394625
baseline median = 442168.125
window median = 448627.8125
bin_mode = Quantile
aggregation = Cumulative
metric = MaxDiff
weighted = False
score = 0.067592815
scores = [0.0, 0.06759281437125747, 0.03920159680638724, 0.04579441117764471, 0.02439920159680642, 0.0, 0.0]
index = 1
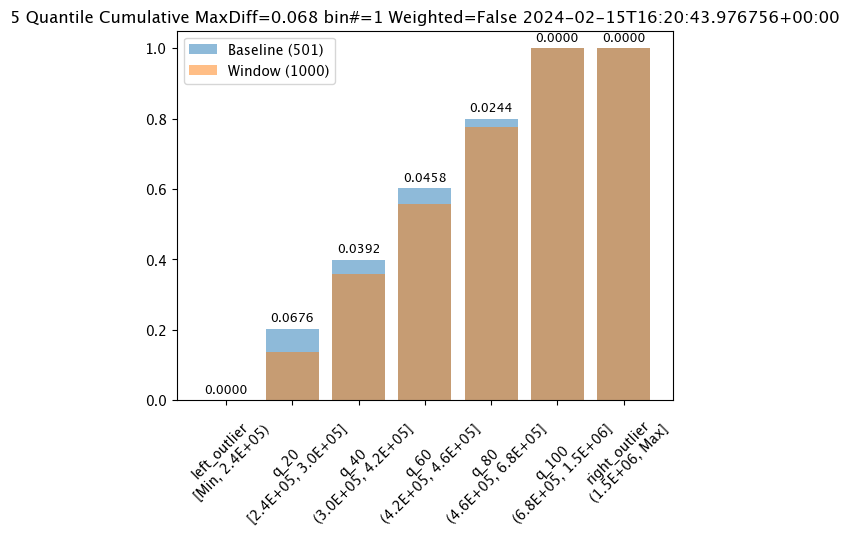
List and Retrieve Assay
If the assay id is not already know, it is retrieved from the wallaroo.client.list_assays()
method. Select the assay to retrieve data for and retrieve its id with wallaroo.assay.Assay._id
method.
wl.list_assays()
name | active | status | warning_threshold | alert_threshold | pipeline_name |
---|---|---|---|---|---|
assays creation example | True | {"run_at": "2024-02-15T16:40:53.212979206+00:00", "num_ok": 0, "num_warnings": 0, "num_alerts": 0} | None | 0.5 | assay-demonstration-tutorial |
retrieved_assay = wl.list_assays()[0]
live_assay_results = wl.get_assay_results(assay_id=retrieved_assay._id,
start=assay_window_start,
end=datetime.datetime.now())
live_assay_results.chart_scores()
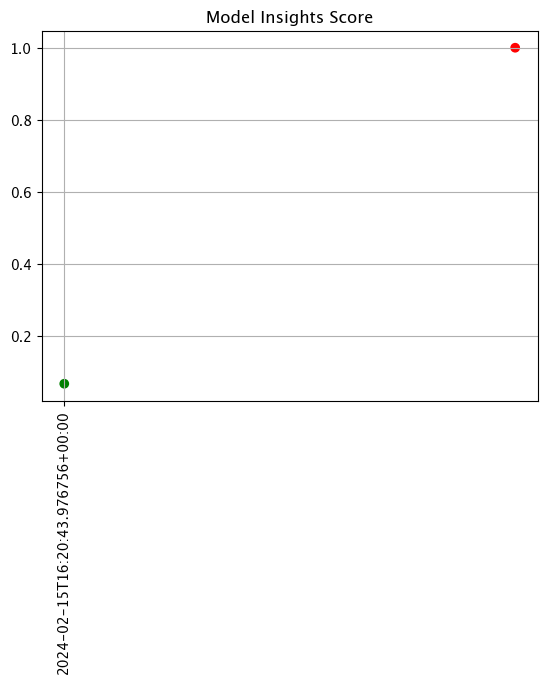
live_assay_results[0].chart()
baseline mean = 495193.23178642715
window mean = 517763.394625
baseline median = 442168.125
window median = 448627.8125
bin_mode = Quantile
aggregation = Cumulative
metric = MaxDiff
weighted = False
score = 0.067592815
scores = [0.0, 0.06759281437125747, 0.03920159680638724, 0.04579441117764471, 0.02439920159680642, 0.0, 0.0]
index = 1
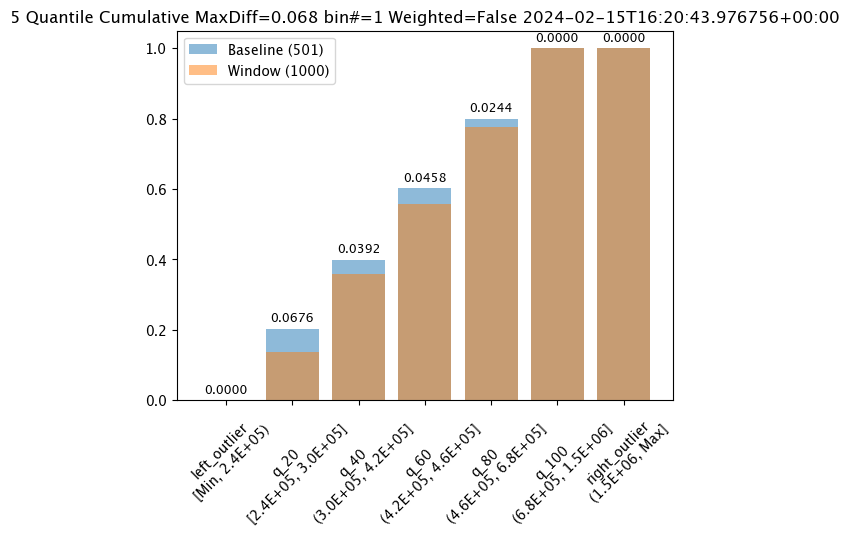
Pause and Resume Assay
Assays are paused and started with the wallaroo.assay.Assay.turn_off
and wallaroo.assay.Assay.turn_on
methods.
For the following, we retrieve an assay from the wallaroo instance and pause it, then list the assays to verify its setting Active
is False
.
display(wl.list_assays())
retrieved_assay = wl.list_assays()[0]
name | active | status | warning_threshold | alert_threshold | pipeline_name |
---|---|---|---|---|---|
assays creation example | True | {"run_at": "2024-02-15T16:40:53.212979206+00:00", "num_ok": 0, "num_warnings": 0, "num_alerts": 0} | None | 0.5 | assay-demonstration-tutorial |
Now we pause the assay, and show the assay list to verify it is no longer active.
retrieved_assay.turn_off()
display(wl.list_assays())
name | active | status | warning_threshold | alert_threshold | pipeline_name |
---|---|---|---|---|---|
assays creation example | False | {"run_at": "2024-02-15T16:40:53.212979206+00:00", "num_ok": 0, "num_warnings": 0, "num_alerts": 0} | None | 0.5 | assay-demonstration-tutorial |
We resume the assay and verify its setting Active
is True
.
retrieved_assay.turn_on()
display(wl.list_assays())
name | active | status | warning_threshold | alert_threshold | pipeline_name |
---|---|---|---|---|---|
assays creation example | True | {"run_at": "2024-02-15T16:40:53.212979206+00:00", "num_ok": 0, "num_warnings": 0, "num_alerts": 0} | None | 0.5 | assay-demonstration-tutorial |
Undeploy Main Pipeline
With the examples and tutorial complete, we will undeploy the main pipeline and return the resources back to the Wallaroo instance.
mainpipeline.undeploy()
6 - Computer Vision Pipeline Logs MLOps API Tutorial
This tutorial and the assets can be downloaded as part of the Wallaroo Tutorials repository.
Pipeline API Log Tutorial
This tutorial demonstrates Wallaroo Pipeline MLOps API for pipeline log retrieval for Computer Vision based models.
This tutorial will demonstrate how to:
- Select or create a workspace, pipeline and upload the control model, and additional testing models.
- Add a pipeline step with the champion model, then deploy the pipeline and perform sample inferences for computer vision detection.
- Retrieve the logs via the Wallaroo MLOps API. These steps will be simplified to only show the API log retrieval method. See the Wallaroo Documentation site for full details.
- Use the pipeline logs to display metadata data.
- Undeploy the pipeline.
This tutorial provides the following:
- Models:
models/yolov8n.onnx
: A pre-trained Yolov8n model.
- Data:
data/dogbike.png
: A PNG image with a dog and bicycle.data/dogbike.df.json
: A pandas Record format JSON file of the PNG image converted to numpy array values for inference requests.
Prerequisites
- A deployed Wallaroo instance
- The following Python libraries installed:
wallaroo
: The Wallaroo SDK. Included with the Wallaroo JupyterHub service by default.
Initial Steps
Import libraries
The first step is to import the libraries needed for this notebook.
import wallaroo
from wallaroo.object import EntityNotFoundError
import pyarrow as pa
from IPython.display import display
# used to display DataFrame information without truncating
from IPython.display import display
import pandas as pd
pd.set_option('display.max_colwidth', None)
import datetime
import requests
Connect to the Wallaroo Instance
The first step is to connect to Wallaroo through the Wallaroo client. The Python library is included in the Wallaroo install and available through the Jupyter Hub interface provided with your Wallaroo environment.
This is accomplished using the wallaroo.Client()
command, which provides a URL to grant the SDK permission to your specific Wallaroo environment. When displayed, enter the URL into a browser and confirm permissions. Store the connection into a variable that can be referenced later.
If logging into the Wallaroo instance through the internal JupyterHub service, use wl = wallaroo.Client()
. If logging in externally, update the wallarooPrefix
and wallarooSuffix
variables with the proper DNS information. For more information on Wallaroo Client settings, see the Client Connection guide.
# Login through local Wallaroo instance
wl = wallaroo.Client()
Wallaroo MLOps API URL
API URL
The variable APIURL
is used to specify the connection to the Wallaroo instance’s MLOps API URL, and is composed of the Wallaroo DNS prefix and suffix. For full details, see the Wallaroo API Connection Guide
.
For this demonstration, the following Wallaroo SDK methods are used to generate the API authentication Bearer token, and the MLOps API URL.
For full details on connecting to a Wallaroo instance via MLOps API calls, see the Wallaroo API Connection Guide.
These methods are:
wallaroo.client.auth_header()
: Returns the authorization Bearer token for a user authenticated through the Wallaroo SDK.wallaroo.client.api_endpoint
: Returns the Wallaroo instance’s api endpoint.
display(wl.auth.auth_header())
display(wl.api_endpoint)
{'Authorization': 'Bearer eyJhbGciOiJSUzI1NiIsInR5cCIgOiAiSldUIiwia2lkIiA6ICJzWThabWtGcm9VWDdDSzFiQjEyS1dwWGtaaUk2R01Gd1ZQUHduWmxxbkVRIn0.eyJleHAiOjE3MDMyNjYxOTIsImlhdCI6MTcwMzI2NjEzMiwiYXV0aF90aW1lIjoxNzAzMjY0OTgxLCJqdGkiOiI0NDdmNmU4YS02NTY0LTRhMDQtODBiYy03MDY1YjViMzM4NTQiLCJpc3MiOiJodHRwczovL2RvYy10ZXN0LmtleWNsb2FrLndhbGxhcm9vY29tbXVuaXR5Lm5pbmphL2F1dGgvcmVhbG1zL21hc3RlciIsImF1ZCI6WyJtYXN0ZXItcmVhbG0iLCJhY2NvdW50Il0sInN1YiI6ImNkOGZkMDYzLTYyZmItNDhkYy05NTg5LTFkZTFiMjlkOTZhNyIsInR5cCI6IkJlYXJlciIsImF6cCI6InNkay1jbGllbnQiLCJzZXNzaW9uX3N0YXRlIjoiMTIxNTg0M2UtMThhNy00NzgyLWE0YjgtODQ4YjIyYWQxYzdmIiwiYWNyIjoiMSIsInJlYWxtX2FjY2VzcyI6eyJyb2xlcyI6WyJkZWZhdWx0LXJvbGVzLW1hc3RlciIsIm9mZmxpbmVfYWNjZXNzIiwidW1hX2F1dGhvcml6YXRpb24iXX0sInJlc291cmNlX2FjY2VzcyI6eyJtYXN0ZXItcmVhbG0iOnsicm9sZXMiOlsibWFuYWdlLXVzZXJzIiwidmlldy11c2VycyIsInF1ZXJ5LWdyb3VwcyIsInF1ZXJ5LXVzZXJzIl19LCJhY2NvdW50Ijp7InJvbGVzIjpbIm1hbmFnZS1hY2NvdW50IiwibWFuYWdlLWFjY291bnQtbGlua3MiLCJ2aWV3LXByb2ZpbGUiXX19LCJzY29wZSI6InByb2ZpbGUgb3BlbmlkIGVtYWlsIiwic2lkIjoiMTIxNTg0M2UtMThhNy00NzgyLWE0YjgtODQ4YjIyYWQxYzdmIiwiZW1haWxfdmVyaWZpZWQiOmZhbHNlLCJodHRwczovL2hhc3VyYS5pby9qd3QvY2xhaW1zIjp7IngtaGFzdXJhLXVzZXItaWQiOiJjZDhmZDA2My02MmZiLTQ4ZGMtOTU4OS0xZGUxYjI5ZDk2YTciLCJ4LWhhc3VyYS1kZWZhdWx0LXJvbGUiOiJ1c2VyIiwieC1oYXN1cmEtYWxsb3dlZC1yb2xlcyI6WyJ1c2VyIl0sIngtaGFzdXJhLXVzZXItZ3JvdXBzIjoie30ifSwibmFtZSI6IkpvaG4gSGFuc2FyaWNrIiwicHJlZmVycmVkX3VzZXJuYW1lIjoiam9obi5odW1tZWxAd2FsbGFyb28uYWkiLCJnaXZlbl9uYW1lIjoiSm9obiIsImZhbWlseV9uYW1lIjoiSGFuc2FyaWNrIiwiZW1haWwiOiJqb2huLmh1bW1lbEB3YWxsYXJvby5haSJ9.W4rshsGTfdHrQnfP03zDcKUJSTEXVHb6HGmci5wDjCSUmqVibauaifoPl1b9mC9XR384-mt9CTEoA4k9JR9YntGzVgifQ2FgSvFtLHaTMMdl1BYulmJmnG_1jxheU7bc0XcgaBpTTQprkVn_UNvipIQuTxIwSsRyJzCmTXofQ8MQB5M1UVz2EQij-w9PzNWUbgDQ0K4IueYFK3idF3VWZCrO3ETEQV-xqySc-GoOmS8IQHWgNBb_WD11SjBYj1wrISYj3PgBAalyCc-hxZKDbdtuWGjjjeexqkGr_aTc4DfxQS3VUki4k9pCJEi5riLeOf0eJvRRYT69GrLrIe7IIA'}
Create Workspace
We will create a workspace to manage our pipeline and models. The following variables will set the name of our sample workspace then set it as the current workspace.
IMPORTANT NOTE: Workspace names must be unique across the Wallaroo instance. To verify unique names, the randomization code below is provided to allow the workspace name to be unique. If this is not required, set
suffix
to''
.References
import string
import random
# make a random 4 character suffix to prevent overwriting other user's workspaces
suffix= ''.join(random.choice(string.ascii_lowercase) for i in range(4))
suffix=''
workspace_name = f'log-api-cv-workspace{suffix}'
main_pipeline_name = 'log-api-cv'
model_name = 'yolov8n'
model_file_name = './models/yolov8n.onnx'
def get_workspace(name, client):
workspace = None
for ws in client.list_workspaces():
if ws.name() == name:
workspace= ws
if(workspace == None):
workspace = client.create_workspace(name)
return workspace
workspace = get_workspace(workspace_name, wl)
wl.set_current_workspace(workspace)
workspace_id = workspace.id()
Upload The Computer Vision Model
For our example, we will upload the Yolov8n model, and set the input field to images
.
# Upload Retrained Yolo8 Model
yolov8_model = (wl.upload_model(model_name,
model_file_name,
framework=wallaroo.framework.Framework.ONNX)
.configure(tensor_fields=['images'],
batch_config="single"
)
)
Build the Pipeline
This pipeline is made to be an example of an existing situation where a model is deployed and being used for inferences in a production environment. We’ll call it housepricepipeline
, set housingcontrol
as a pipeline step, then run a few sample inferences.
mainpipeline = wl.build_pipeline(main_pipeline_name)
# in case this pipeline was run before
mainpipeline.undeploy()
mainpipeline.clear()
mainpipeline.add_model_step(yolov8_model).deploy()
name | log-api-cv |
---|---|
created | 2023-12-22 17:10:06.563220+00:00 |
last_updated | 2023-12-22 17:55:34.460452+00:00 |
deployed | True |
arch | None |
tags | |
versions | b1c6d8ea-0259-4a45-aca5-c065a2d59d2d, a8f0adef-5ab9-4695-95eb-e09c256b221c, d174e395-66b5-40bb-bec0-a689e597749f, 4db1d862-0c8d-4394-9009-8aee1324503c, ce598566-83f7-4983-a8e5-4fc779e2c008, b016fb58-d52e-46e1-afc4-31268f471077, 0bd75ecf-1923-42cf-b7e5-6593699465c0 |
steps | api-sample-model |
published | False |
Testing
We’ll pass in our DataFrame reference file as an inference request, noting the start and end times for our log retrieval.
dataframe_start = datetime.datetime.now(datetime.timezone.utc)
# run as inference api
# Retrieve the token
headers = wl.auth.auth_header()
# set Content-Type type
headers['Content-Type']='application/json; format=pandas-records'
## Inference through external URL using dataframe
df = pd.read_json('./data/dogbike.df.json')
data = df.to_dict(orient="records")
# submit the request via POST, import as pandas DataFrame
response = pd.DataFrame.from_records(
requests.post(
mainpipeline._deployment._url(),
json=data,
headers=headers)
.json()
)
# just to account for any local versus server time discrepancy
import time
time.sleep(20)
dataframe_end = datetime.datetime.now(datetime.timezone.utc)
# run additional inferences outside the time frame
for i in range(10):
# submit the request via POST, import as pandas DataFrame
response = pd.DataFrame.from_records(
requests.post(
mainpipeline._deployment._url(),
json=data,
headers=headers)
.json()
)
Get Pipeline Logs
Pipeline logs are retrieved through the Wallaroo MLOps API with the following request.
- REQUEST URL
v1/api/pipelines/get_logs
- Headers
- Accept:
application/json; format=pandas-records
: For the logs returned as pandas DataFrameapplication/vnd.apache.arrow.file
: for the logs returned as Apache Arrow
- Accept:
- PARAMETERS
- pipeline_name (String Required): The name of the pipeline.
- workspace_id (Integer Required): The numerical identifier of the workspace.
- cursor (String Optional): Cursor returned with a previous page of results from a pipeline log request, used to retrieve the next page of information.
- order (String Optional Default:
Desc
): The order for log inserts returned. Valid values are:Asc
: In chronological order of inserts.Desc
: In reverse chronological order of inserts.
- page_size (Integer Optional Default:
1000
.): Max records per page. - start_time (String Optional): The start time of the period to retrieve logs for in RFC 3339 format for DateTime. Must be combined with
end_time
. - end_time (String Optional): The end time of the period to retrieve logs for in RFC 3339 format for DateTime. Must be combined with
start_time
.
- RETURNS
- The logs are returned by default as
'application/json; format=pandas-records'
format. To request the logs as Apache Arrow tables, set the submission headerAccept
toapplication/vnd.apache.arrow.file
. - Headers:
- x-iteration-cursor: Used to retrieve the next page of results. This is not included if
x-iteration-status
isAll
. - x-iteration-status: Informs whether there are more records available outside of this log request parameters.
- All: This page includes all logs available from this request. If
x-iteration-status
isAll
, thenx-iteration-cursor
is not provided. - SchemaChange: A change in the log schema caused by actions such as pipeline version, etc.
- RecordLimited: The number of records exceeded from the page size, more records can be requested as the next page. There may be more records available to retrieve OR the record limit was reached for this request even if no more records are available in next cursor request.
- ByteLimited: The number of records exceeded the pipeline log limit which is around 100K.
- All: This page includes all logs available from this request. If
- x-iteration-cursor: Used to retrieve the next page of results. This is not included if
- The logs are returned by default as
Get Pipeline Logs Example
For our example, we will retrieve the pipeline logs. FIrst by specifying the date and time, then we will request the logs and continue to show them as long as the cursor has another log to display. Because of the size of the input and outputs, most logs may be constrained by the x-iteration-status
as ByteLimited
.
# retrieve the authorization token
headers = wl.auth.auth_header()
url = f"{wl.api_endpoint}/v1/api/pipelines/get_logs"
# Standard log retrieval
data = {
'pipeline_name': main_pipeline_name,
'workspace_id': workspace_id,
'start_time': dataframe_start.isoformat(),
'end_time': dataframe_end.isoformat()
}
response = requests.post(url, headers=headers, json=data)
standard_logs = pd.DataFrame.from_records(response.json())
display(len(standard_logs))
display(standard_logs.loc[:, ["time", "out"]])
1
time | out | |
---|---|---|
0 | 1703267760538 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
# retrieve the authorization token
headers = wl.auth.auth_header()
url = f"{wl.api_endpoint}/v1/api/pipelines/get_logs"
# datetime set back one day to get more values
data = {
'pipeline_name': main_pipeline_name,
'workspace_id': workspace_id
}
response = requests.post(url, headers=headers, json=data)
standard_logs = pd.DataFrame.from_records(response.json())
display(standard_logs.loc[:, ["time", "out"]])
cursor = response.headers['x-iteration-cursor']
# if there's another record, get the next one
while 'x-iteration-cursor' in response.headers:
# retrieve the authorization token
headers = wl.auth.auth_header()
url = f"{wl.api_endpoint}/v1/api/pipelines/get_logs"
# datetime set back one day to get more values
data = {
'pipeline_name': main_pipeline_name,
'workspace_id': workspace_id,
'cursor': response.headers['x-iteration-cursor']
}
response = requests.post(url, headers=headers, json=data)
# if there's no response, the logs are done
if response.json() != []:
standard_logs = pd.DataFrame.from_records(response.json())
display(standard_logs.head(5).loc[:, ["time", "out"]])
time | out | |
---|---|---|
0 | 1703267304746 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
1 | 1703267414439 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
2 | 1703267420527 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
3 | 1703267426032 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
4 | 1703267432037 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
5 | 1703267437567 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
6 | 1703267442644 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
7 | 1703267449561 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
8 | 1703267455734 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
9 | 1703267462641 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
10 | 1703267468362 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
time | out | |
---|---|---|
0 | 1703267760538 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
1 | 1703267788432 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
2 | 1703267794241 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
3 | 1703267802339 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
4 | 1703267810346 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
time | out | |
---|---|---|
0 | 1703266176951 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
1 | 1703266192757 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
2 | 1703266198591 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
3 | 1703266205430 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
4 | 1703266212538 | {'output0': [17.09787, 16.459343, 17.259743, 19.960602, 43.600235, 59.986958, 62.826073, 68.24793, 77.43261, 80.82158, 89.44183, 96.168915, 99.22421, 112.584045, 126.75803, 131.9707, 137.1645, 141.93822, 146.29594, 152.00876, 155.94037, 165.20976, 175.27249, 184.05307, 193.66891, 201.51189, 215.04979, 223.80424, 227.24472, 234.19638, 244.9743, 248.5781, 252.42526, 264.95795, 278.48563, 285.758, 293.1897, 300.48227, 305.47742, 314.46085, 319.89404, 324.83658, 335.99536, 345.1116, 350.31964, 352.41107, 365.44934, 381.30008, 391.52316, 399.29163, 405.78503, 411.33804, 415.93207, 421.6868, 431.67108, 439.9069, 447.71542, 459.38522, 474.13187, 479.32642, 484.49884, 493.5153, 501.29932, 507.7967, 514.26044, 523.1473, 531.3479, 542.5094, 555.619, 557.7229, 564.6408, 571.5525, 572.8373, 587.95703, 604.2997, 609.452, 616.31714, 623.5797, 624.13153, 634.47266, 16.970057, 16.788723, 17.441803, 17.900642, 36.188023, 57.277973, 61.664352, 62.556896, 63.43486, 79.50621, 83.844, 95.983765, 106.166, 115.368454, 123.09253, 124.5821, 128.65866, 139.16113, 142.02315, 143.69855, ...]} |
Undeploy Main Pipeline
With the examples and tutorial complete, we will undeploy the main pipeline and return the resources back to the Wallaroo instance.
mainpipeline.undeploy()
name | log-api-cv |
---|---|
created | 2023-12-22 17:10:06.563220+00:00 |
last_updated | 2023-12-22 17:55:34.460452+00:00 |
deployed | False |
arch | None |
tags | |
versions | b1c6d8ea-0259-4a45-aca5-c065a2d59d2d, a8f0adef-5ab9-4695-95eb-e09c256b221c, d174e395-66b5-40bb-bec0-a689e597749f, 4db1d862-0c8d-4394-9009-8aee1324503c, ce598566-83f7-4983-a8e5-4fc779e2c008, b016fb58-d52e-46e1-afc4-31268f471077, 0bd75ecf-1923-42cf-b7e5-6593699465c0 |
steps | api-sample-model |
published | False |
7 - Wallaroo Edge Observability with Classification Financial Models through API
The following tutorial is available on the Wallaroo Github Repository.
Classification Financial Services Edge Deployment Demonstration via API
This notebook will walk through building Wallaroo pipeline with a a Classification model deployed to detect the likelihood of credit card fraud, then publishing that pipeline to an Open Container Initiative (OCI) Registry where it can be deployed in other Docker and Kubernetes environments. This example focuses on using the Wallaroo MLOps API to publish the pipeline and retrieve the relevant information.
This demonstration will focus on deployment to the edge. For further examples of using Wallaroo with this computer vision models, see Wallaroo 101.
For this demonstration, logging into the Wallaroo MLOps API will be done through the Wallaroo SDK. For more details on authenticating and connecting to the Wallaroo MLOps API, see the Wallaroo API Connection Guide.
This demonstration performs the following:
- In Wallaroo Ops:
- Setting up a workspace, pipeline, and model for deriving the price of a house based on inputs.
- Creating an assay from a sample of inferences.
- Display the inference result and upload the assay to the Wallaroo instance where it can be referenced later.
- In a remote aka edge location:
- Deploying the Wallaroo pipeline as a Wallaroo Inference Server deployed on an edge device with observability features.
- In Wallaroo Ops:
- Observe the Wallaroo Ops and remote Wallaroo Inference Server inference results as part of the pipeline logs.
Prerequisites
- A deployed Wallaroo Ops instance.
- A location with Docker or Kubernetes with
helm
for Wallaroo Inference server deployments. - The following Python libraries installed:
References
Data Scientist Pipeline Publish Steps
Load Libraries
The first step is to import the libraries used in this notebook.
import wallaroo
from wallaroo.object import EntityNotFoundError
import pyarrow as pa
import pandas as pd
import requests
# used to display dataframe information without truncating
from IPython.display import display
pd.set_option('display.max_colwidth', None)
Connect to the Wallaroo Instance through the User Interface
The next step is to connect to Wallaroo through the Wallaroo client. The Python library is included in the Wallaroo install and available through the Jupyter Hub interface provided with your Wallaroo environment.
This is accomplished using the wallaroo.Client()
command, which provides a URL to grant the SDK permission to your specific Wallaroo environment. When displayed, enter the URL into a browser and confirm permissions. Store the connection into a variable that can be referenced later.
If logging into the Wallaroo instance through the internal JupyterHub service, use wl = wallaroo.Client()
. For more information on Wallaroo Client settings, see the Client Connection guide.
wl = wallaroo.Client()
Create a New Workspace
We’ll use the SDK below to create our workspace , assign as our current workspace, then display all of the workspaces we have at the moment. We’ll also set up variables for our models and pipelines down the road, so we have one spot to change names to whatever fits your organization’s standards best.
To allow this tutorial to be run by multiple users in the same Wallaroo instance, a random 4 character prefix will be added to the workspace, pipeline, and model. Feel free to set suffix=''
if this is not required.
import string
import random
# make a random 4 character suffix to verify uniqueness in tutorials
suffix_random= ''.join(random.choice(string.ascii_lowercase) for i in range(4))
suffix=''
workspace_name = f'edge-publish-api-demo{suffix}'
pipeline_name = 'edge-pipeline'
model_name = 'ccfraud'
model_file_name = './models/xgboost_ccfraud.onnx'
def get_workspace(name):
workspace = None
for ws in wl.list_workspaces():
if ws.name() == name:
workspace= ws
if(workspace == None):
workspace = wl.create_workspace(name)
return workspace
def get_pipeline(name):
try:
pipeline = wl.pipelines_by_name(name)[0]
except EntityNotFoundError:
pipeline = wl.build_pipeline(name)
return pipeline
workspace = get_workspace(workspace_name)
wl.set_current_workspace(workspace)
{'name': 'edge-publish-api-demo', 'id': 21, 'archived': False, 'created_by': 'b3deff28-04d0-41b8-a04f-b5cf610d6ce9', 'created_at': '2023-10-31T20:36:33.093439+00:00', 'models': [], 'pipelines': []}
Upload the Model
When a model is uploaded to a Wallaroo cluster, it is optimized and packaged to make it ready to run as part of a pipeline. In many times, the Wallaroo Server can natively run a model without any Python overhead. In other cases, such as a Python script, a custom Python environment will be automatically generated. This is comparable to the process of “containerizing” a model by adding a small HTTP server and other wrapping around it.
Our pretrained model is in ONNX format, which is specified in the framework
parameter.
edge_demo_model = wl.upload_model(
model_name,
model_file_name,
framework=wallaroo.framework.Framework.ONNX,
).configure(tensor_fields=["tensor"])
Reserve Pipeline Resources
Before deploying an inference engine we need to tell wallaroo what resources it will need.
To do this we will use the wallaroo DeploymentConfigBuilder() and fill in the options listed below to determine what the properties of our inference engine will be.
We will be testing this deployment for an edge scenario, so the resource specifications are kept small – what’s the minimum needed to meet the expected load on the planned hardware.
- cpus - 0.5 => allow the engine to use 4 CPU cores when running the neural net
- memory - 900Mi => each inference engine will have 2 GB of memory, which is plenty for processing a single image at a time.
- arch - we will specify the X86 architecture.
deploy_config = (wallaroo
.DeploymentConfigBuilder()
.replica_count(1)
.cpus(1)
.memory("900Mi")
.build()
)
Simulated Edge Deployment
We will now deploy our pipeline into the current Kubernetes environment using the specified resource constraints. This is a “simulated edge” deploy in that we try to mimic the edge hardware as closely as possible.
pipeline = get_pipeline(pipeline_name)
display(pipeline)
pipeline.add_model_step(edge_demo_model)
pipeline.deploy(deployment_config = deploy_config)
name | edge-pipeline |
---|---|
created | 2023-10-31 20:36:33.486078+00:00 |
last_updated | 2023-10-31 20:36:33.486078+00:00 |
deployed | (none) |
arch | None |
tags | |
versions | a5752acc-9334-4cac-8b34-471979b55d61 |
steps | |
published | False |
Waiting for deployment - this will take up to 45s ............. ok
name | edge-pipeline |
---|---|
created | 2023-10-31 20:36:33.486078+00:00 |
last_updated | 2023-10-31 20:36:33.629569+00:00 |
deployed | True |
arch | None |
tags | |
versions | 932e9cd2-978e-40e6-ab84-2ed5e5d63408, a5752acc-9334-4cac-8b34-471979b55d61 |
steps | ccfraud |
published | False |
Run Sample Inference
A sample input will not be provided to test the inference.
The sample DataFrames and arrow tables are in the ./data
directory. We’ll use the Apache Arrow table cc_data_1k.arrow
with 1,000 records.
deploy_url = pipeline._deployment._url()
headers = wl.auth.auth_header()
headers['Content-Type']='application/vnd.apache.arrow.file'
# headers['Content-Type']='application/json; format=pandas-records'
headers['Accept']='application/json; format=pandas-records'
dataFile = './data/cc_data_1k.arrow'
!curl -X POST {deploy_url} \
-H "Authorization:{headers['Authorization']}" \
-H "Content-Type:{headers['Content-Type']}" \
-H "Accept:{headers['Accept']}" \
--data-binary @{dataFile} > curl_response.df.json
% Total % Received % Xferd Average Speed Time Time Time Current
Dload Upload Total Spent Left Speed
100 755k 100 641k 100 114k 44.7M 8176k --:--:-- --:--:-- --:--:-- 52.7M
# display the first 20 results
df_results = pd.read_json('./curl_response.df.json', orient="records")
# get just the partition
df_results['partition'] = df_results['metadata'].map(lambda x: x['partition'])
# display(df_results.head(20))
display(df_results.head(20).loc[:, ['time', 'out', 'partition']])
time | out | partition | |
---|---|---|---|
0 | 1698784607697 | {'variable': [1.0094898]} | engine-fc7d5c445-bw7hf |
1 | 1698784607697 | {'variable': [1.0094898]} | engine-fc7d5c445-bw7hf |
2 | 1698784607697 | {'variable': [1.0094898]} | engine-fc7d5c445-bw7hf |
3 | 1698784607697 | {'variable': [1.0094898]} | engine-fc7d5c445-bw7hf |
4 | 1698784607697 | {'variable': [-1.9073485999999998e-06]} | engine-fc7d5c445-bw7hf |
5 | 1698784607697 | {'variable': [-4.4882298e-05]} | engine-fc7d5c445-bw7hf |
6 | 1698784607697 | {'variable': [-9.36985e-05]} | engine-fc7d5c445-bw7hf |
7 | 1698784607697 | {'variable': [-8.3208084e-05]} | engine-fc7d5c445-bw7hf |
8 | 1698784607697 | {'variable': [-8.332728999999999e-05]} | engine-fc7d5c445-bw7hf |
9 | 1698784607697 | {'variable': [0.0004896521599999999]} | engine-fc7d5c445-bw7hf |
10 | 1698784607697 | {'variable': [0.0006609559]} | engine-fc7d5c445-bw7hf |
11 | 1698784607697 | {'variable': [7.57277e-05]} | engine-fc7d5c445-bw7hf |
12 | 1698784607697 | {'variable': [-0.000100553036]} | engine-fc7d5c445-bw7hf |
13 | 1698784607697 | {'variable': [-0.0005198717]} | engine-fc7d5c445-bw7hf |
14 | 1698784607697 | {'variable': [-3.695488e-06]} | engine-fc7d5c445-bw7hf |
15 | 1698784607697 | {'variable': [-0.00010883808]} | engine-fc7d5c445-bw7hf |
16 | 1698784607697 | {'variable': [-0.00017666817]} | engine-fc7d5c445-bw7hf |
17 | 1698784607697 | {'variable': [-2.8312206e-05]} | engine-fc7d5c445-bw7hf |
18 | 1698784607697 | {'variable': [2.1755695e-05]} | engine-fc7d5c445-bw7hf |
19 | 1698784607697 | {'variable': [-8.493661999999999e-05]} | engine-fc7d5c445-bw7hf |
Undeploy the Pipeline
With the testing complete, we can undeploy the pipeline. Note that deploying and undeploying a pipeline is not required for publishing a pipeline to the Edge Registry - this is done just for this demonstration.
pipeline.undeploy()
Waiting for undeployment - this will take up to 45s .................................... ok
name | edge-pipeline |
---|---|
created | 2023-10-31 20:36:33.486078+00:00 |
last_updated | 2023-10-31 20:36:33.629569+00:00 |
deployed | False |
arch | None |
tags | |
versions | 932e9cd2-978e-40e6-ab84-2ed5e5d63408, a5752acc-9334-4cac-8b34-471979b55d61 |
steps | ccfraud |
published | False |
Publish the Pipeline for Edge Deployment
It worked! For a demo, we’ll take working once as “tested”. So now that we’ve tested our pipeline, we are ready to publish it for edge deployment.
Publishing it means assembling all of the configuration files and model assets and pushing them to an Open Container Initiative (OCI) repository set in the Wallaroo instance as the Edge Registry service. DevOps engineers then retrieve that image and deploy it through Docker, Kubernetes, or similar deployments.
See Edge Deployment Registry Guide for details on adding an OCI Registry Service to Wallaroo as the Edge Deployment Registry.
This is done through the SDK command wallaroo.pipeline.publish(deployment_config)
which has the following parameters and returns.
Publish a Pipeline Endpoint
Pipelines are published as images to the edge registry set in the Enable Wallaroo Edge Registry through the following endpoint.
- Endpoint:
/v1/api/pipelines/publish
- Parameters
- pipeline_id (Integer Required): The numerical id of the pipeline to publish to the edge registry.
- pipeline_version_id (Integer Required): The numerical id of the pipeline’s version to publish to the edge registry.
- model_config_ids (List Optional): The list of model config ids to include.
- Returns
- id (Integer): Numerical Wallaroo id of the published pipeline.
- pipeline_version_id (Integer): Numerical Wallaroo id of the pipeline version published.
- status: The status of the pipeline publish. Values include:
- PendingPublish: The pipeline publication is about to be uploaded or is in the process of being uploaded.
- Published: The pipeline is published and ready for use.
- created_at (String): When the published pipeline was created.
- updated_at (String): When the published pipeline was updated.
- created_by (String): The email address of the Wallaroo user that created the pipeline publish.
- pipeline_url (String): The URL of the published pipeline in the edge registry. May be
null
until the status isPublished
) - engine_url (String): The URL of the published pipeline engine in the edge registry. May be
null
until the status isPublished
. - helm (String): The helm chart, helm reference and helm version.
- engine_config (*
wallaroo.deployment_config.DeploymentConfig
) | The pipeline configuration included with the published pipeline.
Publish Example
We will now publish the pipeline to our Edge Deployment Registry with the pipeline.publish(deployment_config)
command. deployment_config
is an optional field that specifies the pipeline deployment. This can be overridden by the DevOps engineer during deployment. For this demonstration, we will be retrieving the most recent pipeline version.
For this, we will require the pipeline version id, the workspace id, and the model config ids (which will be empty as not required).
# get the pipeline version
pipeline_version_id = pipeline.versions()[-1].id()
display(pipeline_version_id)
pipeline_id = pipeline.id()
display(pipeline_id)
headers = {
"Authorization": wl.auth._bearer_token_str(),
"Content-Type": "application/json",
}
url = f"{wl.api_endpoint}/v1/api/pipelines/publish"
response = requests.post(
url,
headers=headers,
json={"pipeline_id": 1,
"pipeline_version_id": pipeline_version_id,
"model_config_ids": [edge_demo_model.id()]
}
)
display(response.json())
7
7
{'id': 3,
'pipeline_version_id': 7,
'pipeline_version_name': 'a5752acc-9334-4cac-8b34-471979b55d61',
'status': 'PendingPublish',
'created_at': '2023-10-31T20:37:25.516351+00:00',
'updated_at': '2023-10-31T20:37:25.516351+00:00',
'created_by': 'b3deff28-04d0-41b8-a04f-b5cf610d6ce9',
'pipeline_url': None,
'engine_url': None,
'helm': None,
'engine_config': {'engine': {'resources': {'limits': {'cpu': 4.0,
'memory': '3Gi'},
'requests': {'cpu': 4.0, 'memory': '3Gi'}}},
'enginelb': {'resources': {'limits': {'cpu': 1.0, 'memory': '512Mi'},
'requests': {'cpu': 0.2, 'memory': '512Mi'}}},
'engineAux': {}},
'error': None,
'user_images': [],
'docker_run_variables': {}}
publish_id = response.json()['id']
display(publish_id)
3
Get Publish Status
The status of a created Wallaroo pipeline publish is available through the following endpoint.
- Endpoint:
/v1/api/pipelines/get_publish_status
- Parameters
- publish_id (Integer Required): The numerical id of the pipeline publish to retrieve.
- Returns
- id (Integer): Numerical Wallaroo id of the published pipeline.
- pipeline_version_id (Integer): Numerical Wallaroo id of the pipeline version published.
- status: The status of the pipeline publish. Values include:
- PendingPublish: The pipeline publication is about to be uploaded or is in the process of being uploaded.
- Published: The pipeline is published and ready for use.
- created_at (String): When the published pipeline was created.
- updated_at (String): When the published pipeline was updated.
- created_by (String): The email address of the Wallaroo user that created the pipeline publish.
- pipeline_url (String): The URL of the published pipeline in the edge registry. May be
null
until the status isPublished
) - engine_url (String): The URL of the published pipeline engine in the edge registry. May be
null
until the status isPublished
. - helm: The Helm chart information including the following fields:
- reference (String): The Helm reference.
- chart (String): The Helm chart URL.
- version (String): The Helm chart version.
- engine_config (*
wallaroo.deployment_config.DeploymentConfig
) | The pipeline configuration included with the published pipeline.
Get Publish Status Example
The following example shows retrieving the status of a recently created pipeline publish. Once the published status is Published
we know the pipeline is ready for deployment.
headers = {
"Authorization": wl.auth._bearer_token_str(),
"Content-Type": "application/json",
}
url = f"{wl.api_endpoint}/v1/api/pipelines/get_publish_status"
response = requests.post(
url,
headers=headers,
json={
"id": publish_id
},
)
display(response.json())
pub = response.json()
{'id': 3,
'pipeline_version_id': 7,
'pipeline_version_name': 'a5752acc-9334-4cac-8b34-471979b55d61',
'status': 'Publishing',
'created_at': '2023-10-31T20:37:25.516351+00:00',
'updated_at': '2023-10-31T20:37:25.516351+00:00',
'created_by': 'b3deff28-04d0-41b8-a04f-b5cf610d6ce9',
'pipeline_url': None,
'engine_url': None,
'helm': None,
'engine_config': {'engine': {'resources': {'limits': {'cpu': 4.0,
'memory': '3Gi'},
'requests': {'cpu': 4.0, 'memory': '3Gi'}}},
'enginelb': {'resources': {'limits': {'cpu': 1.0, 'memory': '512Mi'},
'requests': {'cpu': 0.2, 'memory': '512Mi'}}},
'engineAux': {}},
'error': None,
'user_images': [],
'docker_run_variables': {}}
List Publishes for a Pipeline
A list of publishes created for a specific pipeline is retrieved through the following endpoint.
- Endpoint:
/v1/api/pipelines/list_publishes_for_pipeline
- Parameters
- publish_id (Integer Required): The numerical id of the pipeline to retrieve all publishes for.
- Returns a List of pipeline publishes with the following fields:
- id (Integer): Numerical Wallaroo id of the published pipeline.
- pipeline_version_id (Integer): Numerical Wallaroo id of the pipeline version published.
- status: The status of the pipeline publish. Values include:
PendingPublish
: The pipeline publication is about to be uploaded or is in the process of being uploaded.Published
: The pipeline is published and ready for use.
- created_at (String): When the published pipeline was created.
- updated_at (String): When the published pipeline was updated.
- created_by (String): The email address of the Wallaroo user that created the pipeline publish.
- pipeline_url (String): The URL of the published pipeline in the edge registry. May be
null
until the status isPublished
) - engine_url (String): The URL of the published pipeline engine in the edge registry. May be
null
until the status isPublished
. - helm: The Helm chart information including the following fields:
- reference (String): The Helm reference.
- chart (String): The Helm chart URL.
- version (String): The Helm chart version.
- engine_config (*
wallaroo.deployment_config.DeploymentConfig
) | The pipeline configuration included with the published pipeline.
List Publishes for a Pipeline Example
headers = {
"Authorization": wl.auth._bearer_token_str(),
"Content-Type": "application/json",
}
url = f"{wl.api_endpoint}/v1/api/pipelines/list_publishes_for_pipeline"
response = requests.post(
url,
headers=headers,
json={"pipeline_id": pipeline_id},
)
display(response.json())
{'publishes': [{'id': 3,
'pipeline_version_id': 7,
'pipeline_version_name': 'a5752acc-9334-4cac-8b34-471979b55d61',
'status': 'Publishing',
'created_at': '2023-10-31T20:37:25.516351+00:00',
'updated_at': '2023-10-31T20:37:25.516351+00:00',
'created_by': 'b3deff28-04d0-41b8-a04f-b5cf610d6ce9',
'pipeline_url': None,
'engine_url': None,
'helm': None,
'engine_config': {'engine': {'resources': {'limits': {'cpu': 4.0,
'memory': '3Gi'},
'requests': {'cpu': 4.0, 'memory': '3Gi'}}},
'enginelb': {'resources': {'limits': {'cpu': 1.0, 'memory': '512Mi'},
'requests': {'cpu': 0.2, 'memory': '512Mi'}}},
'engineAux': {}},
'error': None,
'user_images': [],
'docker_run_variables': {}}],
'edges': []}
DevOps Deployment
We now have our pipeline published to our Edge Registry service. We can deploy this in a x86 environment running Docker that is logged into the same registry service that we deployed to.
For more details, check with the documentation on your artifact service. The following are provided for the three major cloud services:
- Set up authentication for Docker
- Authenticate with an Azure container registry
- Authenticating Amazon ECR Repositories for Docker CLI with Credential Helper
Add Edge Location
Wallaroo Servers can optionally connect to the Wallaroo Ops instance and transmit their inference results. These are added to the pipeline logs for the published pipeline the Wallaroo Server is associated with.
Add Publish Edge
Edges are added to an existing pipeline publish with the following endpoint.
- Endpoint
/v1/api/pipelines/add_edge_to_publish
- Parameters
- name (String Required): The name of the edge. This must be a unique value across all edges in the Wallaroo instance.
- pipeline_publish_id (Integer Required): The numerical identifier of the pipeline publish to add this edge to.
- tags (List[String] Required): A list of optional tags.
- Returns
- id (Integer): The integer ID of the pipeline publish.
- created_at: (String): The DateTime of the pipeline publish.
- docker_run_variables (String) The Docker variables in base64 encoded format that include the following: The
BUNDLE_VERSION
,EDGE_NAME
,JOIN_TOKEN_
,OPSCENTER_HOST
,PIPELINE_URL
, andWORKSPACE_ID
. - engine_config (String): The Wallaroo
wallaroo.deployment_config.DeploymentConfig
for the pipeline. - pipeline_version_id (Integer): The integer identifier of the pipeline version published.
- status (String): The status of the publish.
Published
is a successful publish. - updated_at (DateTime): The DateTime when the pipeline publish was updated.
- user_images (List[String]): User images used in the pipeline publish.
- created_by (String): The UUID of the Wallaroo user that created the pipeline publish.
- engine_url (String): The URL for the published pipeline’s Wallaroo engine in the OCI registry.
- error (String): Any errors logged.
- helm (String): The helm chart, helm reference and helm version.
- pipeline_url (String): The URL for the published pipeline’s container in the OCI registry.
- pipeline_version_name (String): The UUID identifier of the pipeline version published.
- additional_properties (String): Any additional properties.
headers = {
"Authorization": wl.auth._bearer_token_str(),
"Content-Type": "application/json",
}
edge_name = f"ccfraud-observability-api{suffix_random}"
url = f"{wl.api_endpoint}/v1/api/pipelines/add_edge_to_publish"
response = requests.post(
url,
headers=headers,
json={
"pipeline_publish_id": publish_id,
"name": edge_name,
"tags": []
}
)
display(response.json())
{'id': 3,
'pipeline_version_id': 7,
'pipeline_version_name': 'a5752acc-9334-4cac-8b34-471979b55d61',
'status': 'Published',
'created_at': '2023-10-31T20:37:25.516351+00:00',
'updated_at': '2023-10-31T20:37:25.516351+00:00',
'created_by': 'b3deff28-04d0-41b8-a04f-b5cf610d6ce9',
'pipeline_url': 'us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/pipelines/edge-pipeline:a5752acc-9334-4cac-8b34-471979b55d61',
'engine_url': 'us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/engines/proxy/wallaroo/ghcr.io/wallaroolabs/standalone-mini:v2023.4.0-4092',
'helm': {'reference': 'us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/charts@sha256:4197aaf0beccfc7f935e93c60338aa043d84dddaf4deaeadb000a5cdb4c7ab33',
'chart': 'us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/charts/edge-pipeline',
'version': '0.0.1-a5752acc-9334-4cac-8b34-471979b55d61',
'values': {'edgeBundle': 'ZXhwb3J0IEJVTkRMRV9WRVJTSU9OPTEKZXhwb3J0IEVER0VfTkFNRT1jY2ZyYXVkLW9ic2VydmFiaWxpdHktYXBpbnZ3aApleHBvcnQgSk9JTl9UT0tFTj1jMmFmNjgxNC0yZGI4LTRmNDgtOGJmMS03NzIwMTkxODhhMzEKZXhwb3J0IE9QU0NFTlRFUl9IT1NUPXByb2R1Y3QtdWF0LWVlLmVkZ2Uud2FsbGFyb29jb21tdW5pdHkubmluamEKZXhwb3J0IFBJUEVMSU5FX1VSTD11cy1jZW50cmFsMS1kb2NrZXIucGtnLmRldi93YWxsYXJvby1kZXYtMjUzODE2L3VhdC9waXBlbGluZXMvZWRnZS1waXBlbGluZTphNTc1MmFjYy05MzM0LTRjYWMtOGIzNC00NzE5NzliNTVkNjEKZXhwb3J0IFdPUktTUEFDRV9JRD0yMQ=='}},
'engine_config': {'engine': {'resources': {'limits': {'cpu': 4.0,
'memory': '3Gi'},
'requests': {'cpu': 4.0, 'memory': '3Gi'}}},
'enginelb': {'resources': {'limits': {'cpu': 1.0, 'memory': '512Mi'},
'requests': {'cpu': 0.2, 'memory': '512Mi'}}},
'engineAux': {}},
'error': None,
'user_images': [],
'docker_run_variables': {'EDGE_BUNDLE': 'abcde'}}
# used for deployment later
edge_location_publish=response.json()
Remove Edge from Publish
Edges are removed from an existing pipeline publish with the following endpoint.
- Endpoint
/v1/api/pipelines/remove_edge_from_publish
- Parameters
- name (String Required): The name of the edge. This must be a unique value across all edges in the Wallaroo instance.
- Returns
- null
# adding another edge to remove
headers = {
"Authorization": wl.auth._bearer_token_str(),
"Content-Type": "application/json",
}
edge_name2 = f"ccfraud-observability-api2{suffix_random}"
url = f"{wl.api_endpoint}/v1/api/pipelines/add_edge_to_publish"
response = requests.post(
url,
headers=headers,
json={
"pipeline_publish_id": publish_id,
"name": edge_name2,
"tags": []
}
)
display(response.json())
{'id': 3,
'pipeline_version_id': 7,
'pipeline_version_name': 'a5752acc-9334-4cac-8b34-471979b55d61',
'status': 'Published',
'created_at': '2023-10-31T20:37:25.516351+00:00',
'updated_at': '2023-10-31T20:37:25.516351+00:00',
'created_by': 'b3deff28-04d0-41b8-a04f-b5cf610d6ce9',
'pipeline_url': 'us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/pipelines/edge-pipeline:a5752acc-9334-4cac-8b34-471979b55d61',
'engine_url': 'us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/engines/proxy/wallaroo/ghcr.io/wallaroolabs/standalone-mini:v2023.4.0-4092',
'helm': {'reference': 'us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/charts@sha256:4197aaf0beccfc7f935e93c60338aa043d84dddaf4deaeadb000a5cdb4c7ab33',
'chart': 'us-central1-docker.pkg.dev/wallaroo-dev-253816/uat/charts/edge-pipeline',
'version': '0.0.1-a5752acc-9334-4cac-8b34-471979b55d61',
'values': {'edgeBundle': 'ZXhwb3J0IEJVTkRMRV9WRVJTSU9OPTEKZXhwb3J0IEVER0VfTkFNRT1jY2ZyYXVkLW9ic2VydmFiaWxpdHktYXBpMm52d2gKZXhwb3J0IEpPSU5fVE9LRU49NDZhODVmZGYtMzYwZC00MjUxLTk2NTEtNTBkYmRiNDFkNTA1CmV4cG9ydCBPUFNDRU5URVJfSE9TVD1wcm9kdWN0LXVhdC1lZS5lZGdlLndhbGxhcm9vY29tbXVuaXR5Lm5pbmphCmV4cG9ydCBQSVBFTElORV9VUkw9dXMtY2VudHJhbDEtZG9ja2VyLnBrZy5kZXYvd2FsbGFyb28tZGV2LTI1MzgxNi91YXQvcGlwZWxpbmVzL2VkZ2UtcGlwZWxpbmU6YTU3NTJhY2MtOTMzNC00Y2FjLThiMzQtNDcxOTc5YjU1ZDYxCmV4cG9ydCBXT1JLU1BBQ0VfSUQ9MjE='}},
'engine_config': {'engine': {'resources': {'limits': {'cpu': 4.0,
'memory': '3Gi'},
'requests': {'cpu': 4.0, 'memory': '3Gi'}}},
'enginelb': {'resources': {'limits': {'cpu': 1.0, 'memory': '512Mi'},
'requests': {'cpu': 0.2, 'memory': '512Mi'}}},
'engineAux': {}},
'error': None,
'user_images': [],
'docker_run_variables': {'EDGE_BUNDLE': 'abcde'}}
edge_name2
'ccfraud-observability-api2nvwh'
# remove the edge
headers = {
"Authorization": wl.auth._bearer_token_str(),
"Content-Type": "application/json",
}
edge_name2 = f"ccfraud-observability-api2{suffix_random}"
url = f"{wl.api_endpoint}/v1/api/pipelines/remove_edge"
response = requests.post(
url,
headers=headers,
json={
"name": "ccfraud-observability-api2nvwh",
}
)
display(response)
<Response [200]>
DevOps - Pipeline Edge Deployment
Once a pipeline is deployed to the Edge Registry service, it can be deployed in environments such as Docker, Kubernetes, or similar container running services by a DevOps engineer.
Docker Deployment
First, the DevOps engineer must authenticate to the same OCI Registry service used for the Wallaroo Edge Deployment registry.
For more details, check with the documentation on your artifact service. The following are provided for the three major cloud services:
- Set up authentication for Docker
- Authenticate with an Azure container registry
- Authenticating Amazon ECR Repositories for Docker CLI with Credential Helper
For the deployment, the engine URL is specified with the following environmental variables:
DEBUG
(true|false): Whether to include debug output.OCI_REGISTRY
: The URL of the registry service.CONFIG_CPUS
: The number of CPUs to use.OCI_USERNAME
: The edge registry username.OCI_PASSWORD
: The edge registry password or token.PIPELINE_URL
: The published pipeline URL.
Docker Deployment Example
Using our sample environment, here’s sample deployment using Docker with a computer vision ML model, the same used in the Wallaroo Use Case Tutorials Computer Vision: Retail tutorials.
docker run -p 8080:8080 \
-e DEBUG=true -e OCI_REGISTRY={your registry server} \
-e CONFIG_CPUS=1 \
-e OCI_USERNAME=oauth2accesstoken \
-e OCI_PASSWORD={registry token here} \
-e PIPELINE_URL={your registry server}/pipelines/edge-cv-retail:bf70eaf7-8c11-4b46-b751-916a43b1a555 \
{your registry server}/engine:v2023.3.0-main-3707
Docker Compose Deployment
For users who prefer to use docker compose
, the following sample compose.yaml
file is used to launch the Wallaroo Edge pipeline. This is the same used in the Wallaroo Use Case Tutorials Computer Vision: Retail tutorials.
services:
engine:
image: {Your Engine URL}
ports:
- 8080:8080
environment:
PIPELINE_URL: {Your Pipeline URL}
OCI_REGISTRY: {Your Edge Registry URL}
OCI_USERNAME: {Your Registry Username}
OCI_PASSWORD: {Your Token or Password}
CONFIG_CPUS: 1
For example:
services:
engine:
image: sample-registry.com/engine:v2023.3.0-main-3707
ports:
- 8080:8080
environment:
PIPELINE_URL: sample-registry.com/pipelines/edge-cv-retail:bf70eaf7-8c11-4b46-b751-916a43b1a555
OCI_REGISTRY: sample-registry.com
OCI_USERNAME: _json_key_base64
OCI_PASSWORD: abc123
CONFIG_CPUS: 1
Docker Compose Deployment Example
The deployment and undeployment is then just a simple docker compose up
and docker compose down
. The following shows an example of deploying the Wallaroo edge pipeline using docker compose
.
docker compose up
[+] Running 1/1
✔ Container cv_data-engine-1 Recreated 0.5s
Attaching to cv_data-engine-1
cv_data-engine-1 | Wallaroo Engine - Standalone mode
cv_data-engine-1 | Login Succeeded
cv_data-engine-1 | Fetching manifest and config for pipeline: sample-registry.com/pipelines/edge-cv-retail:bf70eaf7-8c11-4b46-b751-916a43b1a555
cv_data-engine-1 | Fetching model layers
cv_data-engine-1 | digest: sha256:c6c8869645962e7711132a7e17aced2ac0f60dcdc2c7faa79b2de73847a87984
cv_data-engine-1 | filename: c6c8869645962e7711132a7e17aced2ac0f60dcdc2c7faa79b2de73847a87984
cv_data-engine-1 | name: resnet-50
cv_data-engine-1 | type: model
cv_data-engine-1 | runtime: onnx
cv_data-engine-1 | version: 693e19b5-0dc7-4afb-9922-e3f7feefe66d
cv_data-engine-1 |
cv_data-engine-1 | Fetched
cv_data-engine-1 | Starting engine
cv_data-engine-1 | Looking for preexisting `yaml` files in //modelconfigs
cv_data-engine-1 | Looking for preexisting `yaml` files in //pipelines
Helm Deployment
Published pipelines can be deployed through the use of helm charts.
Helm deployments take up to two steps - the first step is in retrieving the required values.yaml
and making updates to override.
- Pull the helm charts from the published pipeline. The two fields are the Helm Chart URL and the Helm Chart version to specify the OCI . This typically takes the format of:
helm pull oci://{published.helm_chart_url} --version {published.helm_chart_version}
- Extract the
tgz
file and copy thevalues.yaml
and copy the values used to edit engine allocations, etc. The following are required for the deployment to run:
ociRegistry:
registry: {your registry service}
username: {registry username here}
password: {registry token here}
Store this into another file, suc as local-values.yaml
.
- Create the namespace to deploy the pipeline to. For example, the namespace
wallaroo-edge-pipeline
would be:
kubectl create -n wallaroo-edge-pipeline
Deploy the
helm
installation withhelm install
through one of the following options:Specify the
tgz
file that was downloaded and the local values file. For example:helm install --namespace {namespace} --values {local values file} {helm install name} {tgz path}
Specify the expended directory from the downloaded
tgz
file.helm install --namespace {namespace} --values {local values file} {helm install name} {helm directory path}
Specify the Helm Pipeline Helm Chart and the Pipeline Helm Version.
helm install --namespace {namespace} --values {local values file} {helm install name} oci://{published.helm_chart_url} --version {published.helm_chart_version}
Once deployed, the DevOps engineer will have to forward the appropriate ports to the
svc/engine-svc
service in the specific pipeline. For example, usingkubectl port-forward
to the namespaceccfraud
that would be:kubectl port-forward svc/engine-svc -n ccfraud01 8080 --address 0.0.0.0`
The following generates a docker run
command based on the added edge location example above. Replace $REGISTRYURL
, $REGISTRYUSERNAME
and $REGISTRYPASSWORD
with the appropriate values.
docker_command = f'''
docker run -p 8080:8080 \\
-e DEBUG=true \\
-e OCI_REGISTRY=$REGISTRYURL \\
-e EDGE_BUNDLE={edge_location_publish['docker_run_variables']['EDGE_BUNDLE']} \\
-e CONFIG_CPUS=1 \\
-e OCI_USERNAME=$REGISTRYUSERNAME \\
-e OCI_PASSWORD=$REGISTRYPASSWORD \\
-e PIPELINE_URL={edge_location_publish['pipeline_url']} \\
{edge_location_publish['engine_url']}
'''
print(docker_command)
Edge Deployed Pipeline API Endpoints
Once deployed, we can check the pipelines and models available. We’ll use a curl
command, but any HTTP based request will work the same way.
The endpoint /pipelines
returns:
- id (String): The name of the pipeline.
- status (String): The status as either
Running
, orError
if there are any issues.
For this example, the deployment is made on a machine called testboy.local
. Replace this URL with the URL of you edge deployment.
!curl testboy.local:8080/pipelines
The endpoint /models
returns a List of models with the following fields:
- name (String): The model name.
- sha (String): The sha hash value of the ML model.
- status (String): The status of either Running or Error if there are any issues.
- version (String): The model version. This matches the version designation used by Wallaroo to track model versions in UUID format.
!curl testboy.local:8080/models
Edge Inference Endpoint
The inference endpoint takes the following pattern:
/pipelines/{pipeline-name}
: Thepipeline-name
is the same as returned from the/pipelines
endpoint asid
.
Wallaroo inference endpoint URLs accept the following data inputs through the Content-Type
header:
Content-Type: application/vnd.apache.arrow.file
: For Apache Arrow tables.Content-Type: application/json; format=pandas-records
: For pandas DataFrame in record format.
Once deployed, we can perform an inference through the deployment URL.
The endpoint returns Content-Type: application/json; format=pandas-records
by default with the following fields:
- check_failures (List[Integer]): Whether any validation checks were triggered. For more information, see Wallaroo SDK Essentials Guide: Pipeline Management: Anomaly Testing.
- elapsed (List[Integer]): A list of time in nanoseconds for:
- [0] The time to serialize the input.
- [1…n] How long each step took.
- model_name (String): The name of the model used.
- model_version (String): The version of the model in UUID format.
- original_data: The original input data. Returns
null
if the input may be too long for a proper return. - outputs (List): The outputs of the inference result separated by data type, where each data type includes:
- data: The returned values.
- dim (List[Integer]): The dimension shape returned.
- v (Integer): The vector shape of the data.
- pipeline_name (String): The name of the pipeline.
- shadow_data: Any shadow deployed data inferences in the same format as outputs.
- time (Integer): The time since UNIX epoch.
!curl -X POST testboy.local:8080/pipelines/edge-pipeline \
-H "Content-Type: application/vnd.apache.arrow.file" \
--data-binary @./data/cc_data_1k.arrow > curl_response_edge.df.json
# display the first 20 results
df_results = pd.read_json('./curl_response_edge.df.json', orient="records")
# display(df_results.head(20))
display(df_results.head(20).loc[:, ['time', 'out', 'metadata']])
pipeline.export_logs(
limit=30000,
directory='partition-edge-observability',
file_prefix='edge-logs-api',
dataset=['time', 'out', 'metadata']
)
# display the head 20 results
df_logs = pd.read_json('./partition-edge-observability/edge-logs-api-1.json', orient="records", lines=True)
# get just the partition
# df_results['partition'] = df_results['metadata'].map(lambda x: x['partition'])
# display(df_results.head(20))
display(df_logs.head(20).loc[:, ['time', 'out.variable', 'metadata.partition']])
display(pd.unique(df_logs['metadata.partition']))
8 - Pipeline Logs MLOps API Tutorial
This tutorial and the assets can be downloaded as part of the Wallaroo Tutorials repository.
Pipeline API Log Tutorial
This tutorial demonstrates Wallaroo Pipeline MLOps API for pipeline log retrieval.
This tutorial will demonstrate how to:
- Select or create a workspace, pipeline and upload the control model, and additional testing models.
- Add a pipeline step with the champion model, then deploy the pipeline and perform sample inferences.
- Retrieve the logs via the Wallaroo MLOps API. These steps will be simplified to only show the API log retrieval method. See the Wallaroo Documentation site for full details.
- Swap out the pipeline step with the champion model with a shadow deploy step that compares the champion model against two competitors.
- Perform sample inferences with a shadow deployed step, then display the log files through the MLOps API for a shadow deployed pipeline.
- Swap out the shadow deployed pipeline step with an A/B pipeline step.
- Perform sample inferences with a A/B pipeline step, then display the log files through the MLOps API for an A/B pipeline step.
- Undeploy the pipeline.
This tutorial provides the following:
- Models:
models/rf_model.onnx
: The champion model that has been used in this environment for some time.models/xgb_model.onnx
andmodels/gbr_model.onnx
: Rival models that will be tested against the champion.
- Data:
data/xtest-1.df.json
anddata/xtest-1k.df.json
: DataFrame JSON inference inputs with 1 input and 1,000 inputs.data/xtest-1k.arrow
: Apache Arrow inference inputs with 1 input and 1,000 inputs.
Prerequisites
- A deployed Wallaroo instance
- The following Python libraries installed:
Initial Steps
Import libraries
The first step is to import the libraries needed for this notebook.
import wallaroo
from wallaroo.object import EntityNotFoundError
import pyarrow as pa
from IPython.display import display
# used to display DataFrame information without truncating
from IPython.display import display
import pandas as pd
pd.set_option('display.max_colwidth', None)
import datetime
import requests
Connect to the Wallaroo Instance
The first step is to connect to Wallaroo through the Wallaroo client. The Python library is included in the Wallaroo install and available through the Jupyter Hub interface provided with your Wallaroo environment.
This is accomplished using the wallaroo.Client()
command, which provides a URL to grant the SDK permission to your specific Wallaroo environment. When displayed, enter the URL into a browser and confirm permissions. Store the connection into a variable that can be referenced later.
If logging into the Wallaroo instance through the internal JupyterHub service, use wl = wallaroo.Client()
. If logging in externally, update the wallarooPrefix
and wallarooSuffix
variables with the proper DNS information. For more information on Wallaroo Client settings, see the Client Connection guide.
# Login through local Wallaroo instance
wl = wallaroo.Client()
Wallaroo MLOps API URL
API URL
The variable APIURL
is used to specify the connection to the Wallaroo instance’s MLOps API URL, and is composed of the Wallaroo DNS prefix and suffix. For full details, see the Wallaroo API Connection Guide
.
For our examples, we will use the Wallaroo SDK to retrieve the API endpoint via the wl.api_endpoint()
method.
display(wl.api_endpoint)
'https://doc-test.api.wallarooexample.ai'
Create Workspace
We will create a workspace to manage our pipeline and models. The following variables will set the name of our sample workspace then set it as the current workspace.
workspace_name = 'logapiworkspace'
main_pipeline_name = 'logapipipeline'
model_name_control = 'logapicontrol'
model_file_name_control = './models/rf_model.onnx'
def get_workspace(name, client):
workspace = None
for ws in client.list_workspaces():
if ws.name() == name:
workspace= ws
if(workspace == None):
workspace = client.create_workspace(name)
return workspace
workspace = get_workspace(workspace_name, wl)
wl.set_current_workspace(workspace)
workspace_id = workspace.id()
Standard Pipeline
Upload The Champion Model
For our example, we will upload the champion model that has been trained to derive house prices from a variety of inputs. The model file is rf_model.onnx
, and is uploaded with the name housingcontrol
.
housing_model_control = (wl.upload_model(model_name_control,
model_file_name_control,
framework=wallaroo.framework.Framework.ONNX)
.configure(tensor_fields=["tensor"])
)
Build the Pipeline
This pipeline is made to be an example of an existing situation where a model is deployed and being used for inferences in a production environment. We’ll call it housepricepipeline
, set housingcontrol
as a pipeline step, then run a few sample inferences.
mainpipeline = wl.build_pipeline(main_pipeline_name)
mainpipeline.undeploy()
# in case this pipeline was run before
mainpipeline.clear()
mainpipeline.add_model_step(housing_model_control).deploy()
name | logapipipeline |
---|---|
created | 2024-03-07 17:14:00.354660+00:00 |
last_updated | 2024-03-07 17:14:01.362335+00:00 |
deployed | True |
arch | None |
accel | None |
tags | |
versions | c6c4e074-9525-4c51-8496-d2ed4c0ec714, 9739a581-ea94-4ba6-bcef-169e076253d2 |
steps | logapicontrol |
published | False |
Testing
We’ll pass in two DataFrame formatted inference requests which are returned as a pandas DataFrame. Then roughly 1,000 inferences as a batch as an Apache Arrow table, which is returned as an arrow table, which we’ll convert into a pandas DataFrame to display the first 20 results.
dataframe_start = datetime.datetime.now(datetime.timezone.utc)
normal_input = pd.DataFrame.from_records({"tensor": [[4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0]]})
result = mainpipeline.infer(normal_input)
display(result)
large_house_input = pd.DataFrame.from_records({'tensor': [[4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0, 10.0, 2760.0, 950.0, 47.6696, -122.261, 3970.0, 20000.0, 79.0, 0.0, 0.0]]})
large_house_result = mainpipeline.infer(large_house_input)
display(large_house_result)
import time
time.sleep(10)
dataframe_end = datetime.datetime.now(datetime.timezone.utc)
# generating multiple log entries
batch_inferences = mainpipeline.infer_from_file('./data/xtest-1k.arrow')
batch_inferences = mainpipeline.infer_from_file('./data/xtest-1k.arrow')
batch_inferences = mainpipeline.infer_from_file('./data/xtest-1k.arrow')
large_inference_result = batch_inferences.to_pandas()
display(large_inference_result.head(20))
time | in.tensor | out.variable | anomaly.count | |
---|---|---|---|---|
0 | 2024-03-07 17:14:17.592 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.7] | 0 |
time | in.tensor | out.variable | anomaly.count | |
---|---|---|---|---|
0 | 2024-03-07 17:14:17.811 | [4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0, 10.0, 2760.0, 950.0, 47.6696, -122.261, 3970.0, 20000.0, 79.0, 0.0, 0.0] | [1514079.4] | 0 |
time | in.tensor | out.variable | anomaly.count | |
---|---|---|---|---|
0 | 2024-03-07 17:14:28.829 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.75] | 0 |
1 | 2024-03-07 17:14:28.829 | [2.0, 2.5, 2170.0, 6361.0, 1.0, 0.0, 2.0, 3.0, 8.0, 2170.0, 0.0, 47.7109, -122.017, 2310.0, 7419.0, 6.0, 0.0, 0.0] | [615094.56] | 0 |
2 | 2024-03-07 17:14:28.829 | [3.0, 2.5, 1300.0, 812.0, 2.0, 0.0, 0.0, 3.0, 8.0, 880.0, 420.0, 47.5893, -122.317, 1300.0, 824.0, 6.0, 0.0, 0.0] | [448627.72] | 0 |
3 | 2024-03-07 17:14:28.829 | [4.0, 2.5, 2500.0, 8540.0, 2.0, 0.0, 0.0, 3.0, 9.0, 2500.0, 0.0, 47.5759, -121.994, 2560.0, 8475.0, 24.0, 0.0, 0.0] | [758714.2] | 0 |
4 | 2024-03-07 17:14:28.829 | [3.0, 1.75, 2200.0, 11520.0, 1.0, 0.0, 0.0, 4.0, 7.0, 2200.0, 0.0, 47.7659, -122.341, 1690.0, 8038.0, 62.0, 0.0, 0.0] | [513264.7] | 0 |
5 | 2024-03-07 17:14:28.829 | [3.0, 2.0, 2140.0, 4923.0, 1.0, 0.0, 0.0, 4.0, 8.0, 1070.0, 1070.0, 47.6902, -122.339, 1470.0, 4923.0, 86.0, 0.0, 0.0] | [668288.0] | 0 |
6 | 2024-03-07 17:14:28.829 | [4.0, 3.5, 3590.0, 5334.0, 2.0, 0.0, 2.0, 3.0, 9.0, 3140.0, 450.0, 47.6763, -122.267, 2100.0, 6250.0, 9.0, 0.0, 0.0] | [1004846.5] | 0 |
7 | 2024-03-07 17:14:28.829 | [3.0, 2.0, 1280.0, 960.0, 2.0, 0.0, 0.0, 3.0, 9.0, 1040.0, 240.0, 47.602, -122.311, 1280.0, 1173.0, 0.0, 0.0, 0.0] | [684577.2] | 0 |
8 | 2024-03-07 17:14:28.829 | [4.0, 2.5, 2820.0, 15000.0, 2.0, 0.0, 0.0, 4.0, 9.0, 2820.0, 0.0, 47.7255, -122.101, 2440.0, 15000.0, 29.0, 0.0, 0.0] | [727898.1] | 0 |
9 | 2024-03-07 17:14:28.829 | [3.0, 2.25, 1790.0, 11393.0, 1.0, 0.0, 0.0, 3.0, 8.0, 1790.0, 0.0, 47.6297, -122.099, 2290.0, 11894.0, 36.0, 0.0, 0.0] | [559631.1] | 0 |
10 | 2024-03-07 17:14:28.829 | [3.0, 1.5, 1010.0, 7683.0, 1.5, 0.0, 0.0, 5.0, 7.0, 1010.0, 0.0, 47.72, -122.318, 1550.0, 7271.0, 61.0, 0.0, 0.0] | [340764.53] | 0 |
11 | 2024-03-07 17:14:28.829 | [3.0, 2.0, 1270.0, 1323.0, 3.0, 0.0, 0.0, 3.0, 8.0, 1270.0, 0.0, 47.6934, -122.342, 1330.0, 1323.0, 8.0, 0.0, 0.0] | [442168.06] | 0 |
12 | 2024-03-07 17:14:28.829 | [4.0, 1.75, 2070.0, 9120.0, 1.0, 0.0, 0.0, 4.0, 7.0, 1250.0, 820.0, 47.6045, -122.123, 1650.0, 8400.0, 57.0, 0.0, 0.0] | [630865.6] | 0 |
13 | 2024-03-07 17:14:28.829 | [4.0, 1.0, 1620.0, 4080.0, 1.5, 0.0, 0.0, 3.0, 7.0, 1620.0, 0.0, 47.6696, -122.324, 1760.0, 4080.0, 91.0, 0.0, 0.0] | [559631.1] | 0 |
14 | 2024-03-07 17:14:28.829 | [4.0, 3.25, 3990.0, 9786.0, 2.0, 0.0, 0.0, 3.0, 9.0, 3990.0, 0.0, 47.6784, -122.026, 3920.0, 8200.0, 10.0, 0.0, 0.0] | [909441.1] | 0 |
15 | 2024-03-07 17:14:28.829 | [4.0, 2.0, 1780.0, 19843.0, 1.0, 0.0, 0.0, 3.0, 7.0, 1780.0, 0.0, 47.4414, -122.154, 2210.0, 13500.0, 52.0, 0.0, 0.0] | [313096.0] | 0 |
16 | 2024-03-07 17:14:28.829 | [4.0, 2.5, 2130.0, 6003.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2130.0, 0.0, 47.4518, -122.12, 1940.0, 4529.0, 11.0, 0.0, 0.0] | [404040.8] | 0 |
17 | 2024-03-07 17:14:28.829 | [3.0, 1.75, 1660.0, 10440.0, 1.0, 0.0, 0.0, 3.0, 7.0, 1040.0, 620.0, 47.4448, -121.77, 1240.0, 10380.0, 36.0, 0.0, 0.0] | [292859.5] | 0 |
18 | 2024-03-07 17:14:28.829 | [3.0, 2.5, 2110.0, 4118.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2110.0, 0.0, 47.3878, -122.153, 2110.0, 4044.0, 25.0, 0.0, 0.0] | [338357.88] | 0 |
19 | 2024-03-07 17:14:28.829 | [4.0, 2.25, 2200.0, 11250.0, 1.5, 0.0, 0.0, 5.0, 7.0, 1300.0, 900.0, 47.6845, -122.201, 2320.0, 10814.0, 94.0, 0.0, 0.0] | [682284.6] | 0 |
Standard Pipeline Logs
Pipeline logs are retrieved through the Wallaroo MLOps API with the following request.
- REQUEST URL
v1/api/pipelines/get_logs
- Headers
- Accept:
application/json; format=pandas-records
: For the logs returned as pandas DataFrameapplication/vnd.apache.arrow.file
: for the logs returned as Apache Arrow
- Accept:
- PARAMETERS
- pipeline_name (String Required): The name of the pipeline.
- workspace_id (Integer Required): The numerical identifier of the workspace.
- cursor (String Optional): Cursor returned with a previous page of results from a pipeline log request, used to retrieve the next page of information.
- order (String Optional Default:
Desc
): The order for log inserts returned. Valid values are:Asc
: In chronological order of inserts.Desc
: In reverse chronological order of inserts.
- page_size (Integer Optional Default:
1000
.): Max records per page. - start_time (String Optional): The start time of the period to retrieve logs for in RFC 3339 format for DateTime. Must be combined with
end_time
. - end_time (String Optional): The end time of the period to retrieve logs for in RFC 3339 format for DateTime. Must be combined with
start_time
.
- RETURNS
- The logs are returned by default as
'application/json; format=pandas-records'
format. To request the logs as Apache Arrow tables, set the submission headerAccept
toapplication/vnd.apache.arrow.file
. - Headers:
- x-iteration-cursor: Used to retrieve the next page of results. This is not included if
x-iteration-status
isAll
. - x-iteration-status: Informs whether there are more records available outside of this log request parameters.
- All: This page includes all logs available from this request. If
x-iteration-status
isAll
, thenx-iteration-cursor
is not provided. - SchemaChange: A change in the log schema caused by actions such as pipeline version, etc.
- RecordLimited: The number of records exceeded from the page size, more records can be requested as the next page. There may be more records available to retrieve OR the record limit was reached for this request even if no more records are available in next cursor request.
- ByteLimited: The number of records exceeded the pipeline log limit which is around 100K.
- All: This page includes all logs available from this request. If
- x-iteration-cursor: Used to retrieve the next page of results. This is not included if
- The logs are returned by default as
# retrieve the authorization token
headers = wl.auth.auth_header()
url = f"{wl.api_endpoint}/v1/api/pipelines/get_logs"
# Standard log retrieval
data = {
'pipeline_name': main_pipeline_name,
'workspace_id': workspace_id
}
response = requests.post(url, headers=headers, json=data)
standard_logs = pd.DataFrame.from_records(response.json())
display(len(standard_logs))
display(standard_logs.head(5).loc[:, ["time", "in", "out"]])
cursor = response.headers['x-iteration-cursor']
2
time | in | out | |
---|---|---|---|
0 | 1709831657592 | {'tensor': [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0]} | {'variable': [718013.7]} |
1 | 1709831657811 | {'tensor': [4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0, 10.0, 2760.0, 950.0, 47.6696, -122.261, 3970.0, 20000.0, 79.0, 0.0, 0.0]} | {'variable': [1514079.4]} |
# Get next page of results as an arrow table
# retrieve the authorization token
headers = wl.auth.auth_header()
headers['Accept']="application/vnd.apache.arrow.file"
url = f"{wl.api_endpoint}/v1/api/pipelines/get_logs"
# Standard log retrieval
data = {
'pipeline_name': main_pipeline_name,
'workspace_id': workspace_id,
'cursor': cursor
}
response = requests.post(url, headers=headers, json=data)
# Arrow table is retrieved
with pa.ipc.open_file(response.content) as reader:
arrow_table = reader.read_all()
# convert to Polars DataFrame and display the first 5 rows
display(arrow_table.to_pandas().head(5).loc[:,["time", "out"]])
time | out | |
---|---|---|
0 | 1709831668232 | {'variable': [718013.75]} |
1 | 1709831668232 | {'variable': [615094.56]} |
2 | 1709831668232 | {'variable': [448627.72]} |
3 | 1709831668232 | {'variable': [758714.2]} |
4 | 1709831668232 | {'variable': [513264.7]} |
# Retrieve logs from specific date/time to only get the two DataFrame input inferences in ascending format
# retrieve the authorization token
headers = wl.auth.auth_header()
url = f"{wl.api_endpoint}/v1/api/pipelines/get_logs"
# Standard log retrieval
data = {
'pipeline_name': main_pipeline_name,
'workspace_id': workspace_id,
'order': 'Asc',
'start_time': f'{dataframe_start.isoformat()}',
'end_time': f'{dataframe_end.isoformat()}'
}
response = requests.post(url, headers=headers, json=data)
standard_logs = pd.DataFrame.from_records(response.json())
display(standard_logs.head(5).loc[:, ["time", "in", "out"]])
display(response.headers)
time | in | out | |
---|---|---|---|
0 | 1709831657592 | {'tensor': [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0]} | {'variable': [718013.7]} |
1 | 1709831657811 | {'tensor': [4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0, 10.0, 2760.0, 950.0, 47.6696, -122.261, 3970.0, 20000.0, 79.0, 0.0, 0.0]} | {'variable': [1514079.4]} |
{'content-type': 'application/json; format=pandas-records', 'x-iteration-status': 'All', 'content-length': '877', 'date': 'Thu, 07 Mar 2024 17:14:29 GMT', 'x-envoy-upstream-service-time': '4', 'server': 'envoy'}
Shadow Deploy Pipelines
Let’s assume that after analyzing the assay information we want to test two challenger models to our control. We do that with the Shadow Deploy pipeline step.
In Shadow Deploy, the pipeline step is added with the add_shadow_deploy
method, with the champion model listed first, then an array of challenger models after. All inference data is fed to all models, with the champion results displayed in the out.variable
column, and the shadow results in the format out_{model name}.variable
. For example, since we named our challenger models housingchallenger01
and housingchallenger02
, the columns out_housingchallenger01.variable
and out_housingchallenger02.variable
have the shadow deployed model results.
For this example, we will remove the previous pipeline step, then replace it with a shadow deploy step with rf_model.onnx
as our champion, and models xgb_model.onnx
and gbr_model.onnx
as the challengers. We’ll deploy the pipeline and prepare it for sample inferences.
# Upload the challenger models
model_name_challenger01 = 'logcontrolchallenger01'
model_file_name_challenger01 = './models/xgb_model.onnx'
model_name_challenger02 = 'logcontrolchallenger02'
model_file_name_challenger02 = './models/gbr_model.onnx'
housing_model_challenger01 = (wl.upload_model(model_name_challenger01,
model_file_name_challenger01,
framework=wallaroo.framework.Framework.ONNX)
.configure(tensor_fields=["tensor"])
)
housing_model_challenger02 = (wl.upload_model(model_name_challenger02,
model_file_name_challenger02,
framework=wallaroo.framework.Framework.ONNX).configure(tensor_fields=["tensor"])
)
# Undeploy the pipeline
mainpipeline.undeploy()
mainpipeline.clear()
# Add the new shadow deploy step with our challenger models
mainpipeline.add_shadow_deploy(housing_model_control, [housing_model_challenger01, housing_model_challenger02])
# Deploy the pipeline with the new shadow step
mainpipeline.deploy()
name | logapipipeline |
---|---|
created | 2024-03-07 17:14:00.354660+00:00 |
last_updated | 2024-03-07 17:16:26.023654+00:00 |
deployed | True |
arch | None |
accel | None |
tags | |
versions | 3690d26a-d9d8-4b48-b0a7-afcac1d7c6b9, c6c4e074-9525-4c51-8496-d2ed4c0ec714, 9739a581-ea94-4ba6-bcef-169e076253d2 |
steps | logapicontrol |
published | False |
Shadow Deploy Sample Inference
We’ll now use our same sample data for an inference to our shadow deployed pipeline, then display the first 20 results with just the comparative outputs.
shadow_date_start = datetime.datetime.now(datetime.timezone.utc)
shadow_result = mainpipeline.infer_from_file('./data/xtest-1k.arrow')
shadow_outputs = shadow_result.to_pandas()
display(shadow_outputs.loc[0:20,['out.variable','out_logcontrolchallenger01.variable','out_logcontrolchallenger02.variable']])
shadow_date_end = datetime.datetime.now(datetime.timezone.utc)
out.variable | out_logcontrolchallenger01.variable | out_logcontrolchallenger02.variable | |
---|---|---|---|
0 | [718013.75] | [659806.0] | [704901.9] |
1 | [615094.56] | [732883.5] | [695994.44] |
2 | [448627.72] | [419508.84] | [416164.8] |
3 | [758714.2] | [634028.8] | [655277.2] |
4 | [513264.7] | [427209.44] | [426854.66] |
5 | [668288.0] | [615501.9] | [632556.1] |
6 | [1004846.5] | [1139732.5] | [1100465.2] |
7 | [684577.2] | [498328.88] | [528278.06] |
8 | [727898.1] | [722664.4] | [659439.94] |
9 | [559631.1] | [525746.44] | [534331.44] |
10 | [340764.53] | [376337.1] | [377187.2] |
11 | [442168.06] | [382053.12] | [403964.3] |
12 | [630865.6] | [505608.97] | [528991.3] |
13 | [559631.1] | [603260.5] | [612201.75] |
14 | [909441.1] | [969585.4] | [893874.7] |
15 | [313096.0] | [313633.75] | [318054.94] |
16 | [404040.8] | [360413.56] | [357816.75] |
17 | [292859.5] | [316674.94] | [294034.7] |
18 | [338357.88] | [299907.44] | [323254.3] |
19 | [682284.6] | [811896.75] | [770916.7] |
20 | [583765.94] | [573618.5] | [549141.4] |
Shadow Deploy Logs
Pipelines with a shadow deployed step include the shadow inference result in the same format as the inference result: inference results from shadow deployed models are displayed as out_{model name}.{output variable}
.
# Retrieve logs from specific date/time to only get the two DataFrame input inferences in ascending format
# retrieve the authorization token
headers = wl.auth.auth_header()
url = f"{wl.api_endpoint}/v1/api/pipelines/get_logs"
# Standard log retrieval
data = {
'pipeline_name': main_pipeline_name,
'workspace_id': workspace_id,
'order': 'Asc',
'start_time': f'{shadow_date_start.isoformat()}',
'end_time': f'{shadow_date_end.isoformat()}'
}
response = requests.post(url, headers=headers, json=data)
standard_logs = pd.DataFrame.from_records(response.json())
display(standard_logs.head(5).loc[:, ["time", "out", "out_logcontrolchallenger01", "out_logcontrolchallenger02"]])
time | out | out_logcontrolchallenger01 | out_logcontrolchallenger02 | |
---|---|---|---|---|
0 | 1709831801106 | {'variable': [718013.75]} | {'variable': [659806.0]} | {'variable': [704901.9]} |
1 | 1709831801106 | {'variable': [615094.56]} | {'variable': [732883.5]} | {'variable': [695994.44]} |
2 | 1709831801106 | {'variable': [448627.72]} | {'variable': [419508.84]} | {'variable': [416164.8]} |
3 | 1709831801106 | {'variable': [758714.2]} | {'variable': [634028.8]} | {'variable': [655277.2]} |
4 | 1709831801106 | {'variable': [513264.7]} | {'variable': [427209.44]} | {'variable': [426854.66]} |
A/B Testing Pipeline
A/B testing allows inference requests to be split between a control model and one or more challenger models. For full details, see the Pipeline Management Guide: A/B Testing.
When the inference results and log entries are displayed, they include the column out._model_split
which displays:
Field | Type | Description |
---|---|---|
name | String | The model name used for the inference. |
version | String | The version of the model. |
sha | String | The sha hash of the model version. |
For this example, the shadow deployed step will be removed and replaced with an A/B Testing step with the ratio 1:1:1, so the control and each of the challenger models will be split randomly between inference requests. A set of sample inferences will be run, then the pipeline logs displayed.
pipeline = (wl.build_pipeline(“randomsplitpipeline-demo”)
.add_random_split([(2, control), (1, challenger)], “session_id”))
ab_date_start = datetime.datetime.now(datetime.timezone.utc)
mainpipeline.undeploy()
# remove the shadow deploy steps
mainpipeline.clear()
# Add the a/b test step to the pipeline
mainpipeline.add_random_split([(1, housing_model_control), (1, housing_model_challenger01), (1, housing_model_challenger02)], "session_id")
mainpipeline.deploy()
# Perform sample inferences of 20 rows and display the results
abtesting_inputs = pd.read_json('./data/xtest-1k.df.json')
for index, row in abtesting_inputs.sample(20).iterrows():
display(mainpipeline.infer(row.to_frame('tensor').reset_index()).loc[:,["out._model_split", "out.variable"]])
ab_date_end = datetime.datetime.now(datetime.timezone.utc)
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger01","version":"1ec4c975-95bf-4f83-8ef8-37c76fd2f861","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}] | [224316.13] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logapicontrol","version":"3a4d6449-64d8-482d-b497-a8c9d9092a8c","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [241330.17] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logapicontrol","version":"3a4d6449-64d8-482d-b497-a8c9d9092a8c","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [675545.44] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger01","version":"1ec4c975-95bf-4f83-8ef8-37c76fd2f861","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}] | [391424.6] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger01","version":"1ec4c975-95bf-4f83-8ef8-37c76fd2f861","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}] | [767853.5] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger02","version":"5a205da3-a31f-4758-9626-74da277f060e","sha":"ed6065a79d841f7e96307bb20d5ef22840f15da0b587efb51425c7ad60589d6a"}] | [327108.25] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logapicontrol","version":"3a4d6449-64d8-482d-b497-a8c9d9092a8c","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [338138.0] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger01","version":"1ec4c975-95bf-4f83-8ef8-37c76fd2f861","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}] | [220148.0] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logapicontrol","version":"3a4d6449-64d8-482d-b497-a8c9d9092a8c","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [291799.84] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logapicontrol","version":"3a4d6449-64d8-482d-b497-a8c9d9092a8c","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [706407.4] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger02","version":"5a205da3-a31f-4758-9626-74da277f060e","sha":"ed6065a79d841f7e96307bb20d5ef22840f15da0b587efb51425c7ad60589d6a"}] | [231846.72] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger01","version":"1ec4c975-95bf-4f83-8ef8-37c76fd2f861","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}] | [428174.94] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger02","version":"5a205da3-a31f-4758-9626-74da277f060e","sha":"ed6065a79d841f7e96307bb20d5ef22840f15da0b587efb51425c7ad60589d6a"}] | [728114.44] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger02","version":"5a205da3-a31f-4758-9626-74da277f060e","sha":"ed6065a79d841f7e96307bb20d5ef22840f15da0b587efb51425c7ad60589d6a"}] | [416164.8] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logapicontrol","version":"3a4d6449-64d8-482d-b497-a8c9d9092a8c","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [879092.9] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logapicontrol","version":"3a4d6449-64d8-482d-b497-a8c9d9092a8c","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [441465.72] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger02","version":"5a205da3-a31f-4758-9626-74da277f060e","sha":"ed6065a79d841f7e96307bb20d5ef22840f15da0b587efb51425c7ad60589d6a"}] | [1532461.0] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logapicontrol","version":"3a4d6449-64d8-482d-b497-a8c9d9092a8c","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [448627.8] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logapicontrol","version":"3a4d6449-64d8-482d-b497-a8c9d9092a8c","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [713485.7] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger01","version":"1ec4c975-95bf-4f83-8ef8-37c76fd2f861","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}] | [1720818.0] |
Retrieve A/B Testing Log Files through API
The log files for A/B Testing pipeline inference results contain the model information with the model outputs in the out
field.
# Retrieve logs from specific date/time to only get the two DataFrame input inferences in ascending format
# retrieve the authorization token
headers = wl.auth.auth_header()
url = f"{wl.api_endpoint}/v1/api/pipelines/get_logs"
# Standard log retrieval
data = {
'pipeline_name': main_pipeline_name,
'workspace_id': workspace_id,
'order': 'Asc',
'start_time': f'{ab_date_start.isoformat()}',
'end_time': f'{ab_date_end.isoformat()}'
}
response = requests.post(url, headers=headers, json=data)
standard_logs = pd.DataFrame.from_records(response.json())
display(standard_logs.head(5).loc[:, ["time", "out"]])
time | out | |
---|---|---|
0 | 1709831883585 | {'_model_split': ['{"name":"logcontrolchallenger01","version":"1ec4c975-95bf-4f83-8ef8-37c76fd2f861","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}'], 'variable': [224316.13]} |
1 | 1709831883828 | {'_model_split': ['{"name":"logapicontrol","version":"3a4d6449-64d8-482d-b497-a8c9d9092a8c","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}'], 'variable': [241330.17]} |
2 | 1709831884044 | {'_model_split': ['{"name":"logapicontrol","version":"3a4d6449-64d8-482d-b497-a8c9d9092a8c","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}'], 'variable': [675545.44]} |
3 | 1709831884273 | {'_model_split': ['{"name":"logcontrolchallenger01","version":"1ec4c975-95bf-4f83-8ef8-37c76fd2f861","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}'], 'variable': [391424.6]} |
4 | 1709831884465 | {'_model_split': ['{"name":"logcontrolchallenger01","version":"1ec4c975-95bf-4f83-8ef8-37c76fd2f861","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}'], 'variable': [767853.5]} |
Undeploy Main Pipeline
With the examples and tutorial complete, we will undeploy the main pipeline and return the resources back to the Wallaroo instance.
mainpipeline.undeploy()
name | logapipipeline |
---|---|
created | 2024-03-07 17:14:00.354660+00:00 |
last_updated | 2024-03-07 17:17:33.498224+00:00 |
deployed | False |
arch | None |
accel | None |
tags | |
versions | 7fba4700-0076-48d8-924c-bbc18d030f47, 3690d26a-d9d8-4b48-b0a7-afcac1d7c6b9, c6c4e074-9525-4c51-8496-d2ed4c0ec714, 9739a581-ea94-4ba6-bcef-169e076253d2 |
steps | logapicontrol |
published | False |
9 - Pipeline Logs Tutorial
This tutorial and the assets can be downloaded as part of the Wallaroo Tutorials repository.
Pipeline Log Tutorial
This tutorial demonstrates Wallaroo Pipeline logs and
This tutorial will demonstrate how to:
- Select or create a workspace, pipeline and upload the control model, then additional models for A/B Testing and Shadow Deploy.
- Add a pipeline step with the champion model, then deploy the pipeline and perform sample inferences.
- Display the various log types for a standard deployed pipeline.
- Swap out the pipeline step with the champion model with a shadow deploy step that compares the champion model against two competitors.
- Perform sample inferences with a shadow deployed step, then display the log files for a shadow deployed pipeline.
- Swap out the shadow deployed pipeline step with an A/B pipeline step.
- Perform sample inferences with a A/B pipeline step, then display the log files for an A/B pipeline step.
- Undeploy the pipeline.
This tutorial provides the following:
- Models:
models/rf_model.onnx
: The champion model that has been used in this environment for some time.models/xgb_model.onnx
andmodels/gbr_model.onnx
: Rival models that will be tested against the champion.
- Data:
data/xtest-1.df.json
anddata/xtest-1k.df.json
: DataFrame JSON inference inputs with 1 input and 1,000 inputs.data/xtest-1k.arrow
: Apache Arrow inference inputs with 1 input and 1,000 inputs.
Prerequisites
- A deployed Wallaroo instance
- The following Python libraries installed:
Initial Steps
Import libraries
The first step is to import the libraries needed for this notebook.
import wallaroo
from wallaroo.object import EntityNotFoundError
import pyarrow as pa
from IPython.display import display
# used to display DataFrame information without truncating
from IPython.display import display
import pandas as pd
pd.set_option('display.max_colwidth', None)
import datetime
import os
Connect to the Wallaroo Instance
The first step is to connect to Wallaroo through the Wallaroo client. The Python library is included in the Wallaroo install and available through the Jupyter Hub interface provided with your Wallaroo environment.
This is accomplished using the wallaroo.Client()
command, which provides a URL to grant the SDK permission to your specific Wallaroo environment. When displayed, enter the URL into a browser and confirm permissions. Store the connection into a variable that can be referenced later.
If logging into the Wallaroo instance through the internal JupyterHub service, use wl = wallaroo.Client()
. For more information on Wallaroo Client settings, see the Client Connection guide.
# Login through local Wallaroo instance
wl = wallaroo.Client()
Create Workspace
We will create a workspace to manage our pipeline and models. The following variables will set the name of our sample workspace then set it as the current workspace.
workspace_name = 'logworkspace'
main_pipeline_name = 'logpipeline-test'
model_name_control = 'logcontrol'
model_file_name_control = './models/rf_model.onnx'
def get_workspace(name):
workspace = None
for ws in wl.list_workspaces():
if ws.name() == name:
workspace= ws
if(workspace == None):
workspace = wl.create_workspace(name)
return workspace
workspace = get_workspace(workspace_name)
wl.set_current_workspace(workspace)
{'name': 'logworkspace', 'id': 27, 'archived': False, 'created_by': 'c97d480f-6064-4537-b18e-40fb1864b4cd', 'created_at': '2024-02-09T16:21:07.131681+00:00', 'models': [], 'pipelines': []}
Standard Pipeline
Upload The Champion Model
For our example, we will upload the champion model that has been trained to derive house prices from a variety of inputs. The model file is rf_model.onnx
, and is uploaded with the name housingcontrol
.
housing_model_control = (wl.upload_model(model_name_control,
model_file_name_control,
framework=wallaroo.framework.Framework.ONNX)
.configure(tensor_fields=["tensor"])
)
Build the Pipeline
This pipeline is made to be an example of an existing situation where a model is deployed and being used for inferences in a production environment. We’ll call it housepricepipeline
, set housingcontrol
as a pipeline step, then run a few sample inferences.
mainpipeline = wl.build_pipeline(main_pipeline_name)
# in case this pipeline was run before
mainpipeline.clear()
mainpipeline.add_model_step(housing_model_control)
deploy_config = wallaroo.deployment_config.DeploymentConfigBuilder() \
.cpus(0.25)\
.build()
mainpipeline.deploy(deployment_config=deploy_config)
Waiting for deployment - this will take up to 45s .......... ok
name | logpipeline-test |
---|---|
created | 2024-02-09 16:21:09.406182+00:00 |
last_updated | 2024-02-09 16:30:53.067304+00:00 |
deployed | True |
arch | None |
tags | |
versions | e2b9d903-4015-4d09-902b-9150a7196cea, 9df38be1-d2f4-4be1-9022-8f0570a238b9, 3078b49f-3eff-48d1-8d9b-a8780b329ecc, 21bff9df-828f-40e7-8a22-449a2e636b44, f78a7030-bd25-4bf7-ba0d-a18cfe3790e0, 10c1ac25-d626-4413-8d5d-1bed42d0e65c, b179b693-b6b6-4ff9-b2a4-2a639d88bc9b, da7b9cf0-81e8-452b-8b70-689406dc9548, a9a9b62c-9d37-427f-99af-67725558bf9b, 1c14591a-96b4-4059-bb63-2d2bc4e308d5, add660ac-0ebf-4a24-bb6d-6cdc875866c8 |
steps | logcontrol |
published | False |
Testing
We’ll use two inferences as a quick sample test - one that has a house that should be determined around \$700k, the other with a house determined to be around \$1.5 million. We’ll also save the start and end periods for these events to for later log functionality.
dataframe_start = datetime.datetime.now()
normal_input = pd.DataFrame.from_records({"tensor": [
[
4.0,
2.5,
2900.0,
5505.0,
2.0,
0.0,
0.0,
3.0,
8.0,
2900.0,
0.0,
47.6063,
-122.02,
2970.0,
5251.0,
12.0,
0.0,
0.0
]
]
}
)
result = mainpipeline.infer(normal_input)
display(result)
time | in.tensor | out.variable | anomaly.count | |
---|---|---|---|---|
0 | 2024-02-09 16:31:04.817 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.7] | 0 |
large_house_input = pd.DataFrame.from_records(
{
'tensor': [
[
4.0,
3.0,
3710.0,
20000.0,
2.0,
0.0,
2.0,
5.0,
10.0,
2760.0,
950.0,
47.6696,
-122.261,
3970.0,
20000.0,
79.0,
0.0,
0.0
]
]
}
)
large_house_result = mainpipeline.infer(large_house_input)
display(large_house_result)
import time
time.sleep(10)
dataframe_end = datetime.datetime.now()
time | in.tensor | out.variable | anomaly.count | |
---|---|---|---|---|
0 | 2024-02-09 16:31:04.917 | [4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0, 10.0, 2760.0, 950.0, 47.6696, -122.261, 3970.0, 20000.0, 79.0, 0.0, 0.0] | [1514079.4] | 0 |
As one last sample, we’ll run through roughly 1,000 inferences at once and show a few of the results. For this example we’ll use an Apache Arrow table, which has a smaller file size compared to uploading a pandas DataFrame JSON file. The inference result is returned as an arrow table, which we’ll convert into a pandas DataFrame to display the first 20 results.
batch_inferences = mainpipeline.infer_from_file('./data/xtest-1k.arrow')
large_inference_result = batch_inferences.to_pandas()
display(large_inference_result.head(20))
time | in.tensor | out.variable | anomaly.count | |
---|---|---|---|---|
0 | 2024-02-09 16:31:15.018 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.75] | 0 |
1 | 2024-02-09 16:31:15.018 | [2.0, 2.5, 2170.0, 6361.0, 1.0, 0.0, 2.0, 3.0, 8.0, 2170.0, 0.0, 47.7109, -122.017, 2310.0, 7419.0, 6.0, 0.0, 0.0] | [615094.56] | 0 |
2 | 2024-02-09 16:31:15.018 | [3.0, 2.5, 1300.0, 812.0, 2.0, 0.0, 0.0, 3.0, 8.0, 880.0, 420.0, 47.5893, -122.317, 1300.0, 824.0, 6.0, 0.0, 0.0] | [448627.72] | 0 |
3 | 2024-02-09 16:31:15.018 | [4.0, 2.5, 2500.0, 8540.0, 2.0, 0.0, 0.0, 3.0, 9.0, 2500.0, 0.0, 47.5759, -121.994, 2560.0, 8475.0, 24.0, 0.0, 0.0] | [758714.2] | 0 |
4 | 2024-02-09 16:31:15.018 | [3.0, 1.75, 2200.0, 11520.0, 1.0, 0.0, 0.0, 4.0, 7.0, 2200.0, 0.0, 47.7659, -122.341, 1690.0, 8038.0, 62.0, 0.0, 0.0] | [513264.7] | 0 |
5 | 2024-02-09 16:31:15.018 | [3.0, 2.0, 2140.0, 4923.0, 1.0, 0.0, 0.0, 4.0, 8.0, 1070.0, 1070.0, 47.6902, -122.339, 1470.0, 4923.0, 86.0, 0.0, 0.0] | [668288.0] | 0 |
6 | 2024-02-09 16:31:15.018 | [4.0, 3.5, 3590.0, 5334.0, 2.0, 0.0, 2.0, 3.0, 9.0, 3140.0, 450.0, 47.6763, -122.267, 2100.0, 6250.0, 9.0, 0.0, 0.0] | [1004846.5] | 0 |
7 | 2024-02-09 16:31:15.018 | [3.0, 2.0, 1280.0, 960.0, 2.0, 0.0, 0.0, 3.0, 9.0, 1040.0, 240.0, 47.602, -122.311, 1280.0, 1173.0, 0.0, 0.0, 0.0] | [684577.2] | 0 |
8 | 2024-02-09 16:31:15.018 | [4.0, 2.5, 2820.0, 15000.0, 2.0, 0.0, 0.0, 4.0, 9.0, 2820.0, 0.0, 47.7255, -122.101, 2440.0, 15000.0, 29.0, 0.0, 0.0] | [727898.1] | 0 |
9 | 2024-02-09 16:31:15.018 | [3.0, 2.25, 1790.0, 11393.0, 1.0, 0.0, 0.0, 3.0, 8.0, 1790.0, 0.0, 47.6297, -122.099, 2290.0, 11894.0, 36.0, 0.0, 0.0] | [559631.1] | 0 |
10 | 2024-02-09 16:31:15.018 | [3.0, 1.5, 1010.0, 7683.0, 1.5, 0.0, 0.0, 5.0, 7.0, 1010.0, 0.0, 47.72, -122.318, 1550.0, 7271.0, 61.0, 0.0, 0.0] | [340764.53] | 0 |
11 | 2024-02-09 16:31:15.018 | [3.0, 2.0, 1270.0, 1323.0, 3.0, 0.0, 0.0, 3.0, 8.0, 1270.0, 0.0, 47.6934, -122.342, 1330.0, 1323.0, 8.0, 0.0, 0.0] | [442168.06] | 0 |
12 | 2024-02-09 16:31:15.018 | [4.0, 1.75, 2070.0, 9120.0, 1.0, 0.0, 0.0, 4.0, 7.0, 1250.0, 820.0, 47.6045, -122.123, 1650.0, 8400.0, 57.0, 0.0, 0.0] | [630865.6] | 0 |
13 | 2024-02-09 16:31:15.018 | [4.0, 1.0, 1620.0, 4080.0, 1.5, 0.0, 0.0, 3.0, 7.0, 1620.0, 0.0, 47.6696, -122.324, 1760.0, 4080.0, 91.0, 0.0, 0.0] | [559631.1] | 0 |
14 | 2024-02-09 16:31:15.018 | [4.0, 3.25, 3990.0, 9786.0, 2.0, 0.0, 0.0, 3.0, 9.0, 3990.0, 0.0, 47.6784, -122.026, 3920.0, 8200.0, 10.0, 0.0, 0.0] | [909441.1] | 0 |
15 | 2024-02-09 16:31:15.018 | [4.0, 2.0, 1780.0, 19843.0, 1.0, 0.0, 0.0, 3.0, 7.0, 1780.0, 0.0, 47.4414, -122.154, 2210.0, 13500.0, 52.0, 0.0, 0.0] | [313096.0] | 0 |
16 | 2024-02-09 16:31:15.018 | [4.0, 2.5, 2130.0, 6003.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2130.0, 0.0, 47.4518, -122.12, 1940.0, 4529.0, 11.0, 0.0, 0.0] | [404040.8] | 0 |
17 | 2024-02-09 16:31:15.018 | [3.0, 1.75, 1660.0, 10440.0, 1.0, 0.0, 0.0, 3.0, 7.0, 1040.0, 620.0, 47.4448, -121.77, 1240.0, 10380.0, 36.0, 0.0, 0.0] | [292859.5] | 0 |
18 | 2024-02-09 16:31:15.018 | [3.0, 2.5, 2110.0, 4118.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2110.0, 0.0, 47.3878, -122.153, 2110.0, 4044.0, 25.0, 0.0, 0.0] | [338357.88] | 0 |
19 | 2024-02-09 16:31:15.018 | [4.0, 2.25, 2200.0, 11250.0, 1.5, 0.0, 0.0, 5.0, 7.0, 1300.0, 900.0, 47.6845, -122.201, 2320.0, 10814.0, 94.0, 0.0, 0.0] | [682284.6] | 0 |
Standard Pipeline Logs
Pipeline logs with standard pipeline steps are retrieved either with:
- Pipeline
logs
which returns either a pandas DataFrame or Apache Arrow table. - Pipeline
export_logs
which saves the logs either a pandas DataFrame JSON file or Apache Arrow table.
For full details, see the Wallaroo Documentation Pipeline Log Management guide.
Pipeline Log Method
The Pipeline logs
method includes the following parameters. For a complete list, see the Wallaroo SDK Essentials Guide: Pipeline Log Management.
Parameter | Type | Description |
---|---|---|
limit | Int (Optional) | Limits how many log records to display. Defaults to 100 . If there are more pipeline logs than are being displayed, the Warning message Pipeline log record limit exceeded will be displayed. For example, if 100 log files were requested and there are a total of 1,000, the warning message will be displayed. |
start_datetime and end_datetime | DateTime (Optional) | Limits logs to all logs between the start and end DateTime parameters. Both parameters must be provided. Submitting a logs() request with only start_datetime or end_datetime will generate an exception.If start_datetime and end_datetime are provided as parameters, then the records are returned in chronological order, with the oldest record displayed first. |
dataset | List (OPTIONAL) | The datasets to be returned. The datasets available are:
metadata.elapsed : IMPORTANT NOTE: See Metadata Requests Restrictionsfor specifications on how this dataset can be used with other datasets.
|
arrow | Boolean (Optional) | Defaults to False. If arrow is set to True , then the logs are returned as an Apache Arrow table. If arrow=False , then the logs are returned as a pandas DataFrame. |
Pipeline Log Warnings
If the total number of logs the either the set limit or 10 MB in file size, the following warning is returned:
Warning: There are more logs available. Please set a larger limit or request a file using export_logs.
If the total number of logs requested either through the limit or through the start_datetime
and end_datetime
request is greater than 10 MB in size, the following error is displayed:
Warning: Pipeline log size limit exceeded. Only displaying 509 log messages. Please request a file using export_logs.
The following examples demonstrate displaying the logs, then displaying the logs between the control_model_start
and control_model_end
periods, then again retrieved as an Arrow table with the logs limited to only 5 entries.
# pipeline log retrieval - reverse chronological order
regular_logs = mainpipeline.logs()
display("Standard Logs")
display(len(regular_logs))
display(regular_logs)
# Display metadata
metadatalogs = mainpipeline.logs(dataset=["time", "out.variable", "metadata"])
display("Metadata Logs")
# Only showing the pipeline version for space reasons
display(metadatalogs.loc[:, ["time", "out.variable", "metadata.pipeline_version"]])
# Display logs restricted by date and limit
display("Logs restricted by date")
arrow_logs = mainpipeline.logs(start_datetime=dataframe_start, end_datetime=dataframe_end, limit=50)
display(len(arrow_logs))
display(arrow_logs)
# # pipeline log retrieval limited to arrow tables
display(mainpipeline.logs(arrow=True))
Pipeline log schema has changed over the logs requested 1 newest records retrieved successfully, newest record seen was at <datetime>. Please request additional records separately
'Standard Logs'
1
time | in.tensor | out.variable | anomaly.count | |
---|---|---|---|---|
0 | 2024-02-09 16:28:44.753 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.7] | 0 |
Pipeline log schema has changed over the logs requested 1 newest records retrieved successfully, newest record seen was at <datetime>. Please request additional records separately
'Metadata Logs'
time | out.variable | metadata.pipeline_version | |
---|---|---|---|
0 | 2024-02-09 16:28:44.753 | [718013.7] | 21bff9df-828f-40e7-8a22-449a2e636b44 |
'Logs restricted by date'
2
time | in.tensor | out.variable | anomaly.count | |
---|---|---|---|---|
0 | 2024-02-09 16:31:04.817 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.7] | 0 |
1 | 2024-02-09 16:31:04.917 | [4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0, 10.0, 2760.0, 950.0, 47.6696, -122.261, 3970.0, 20000.0, 79.0, 0.0, 0.0] | [1514079.4] | 0 |
Pipeline log schema has changed over the logs requested 1 newest records retrieved successfully, newest record seen was at <datetime>. Please request additional records separately
pyarrow.Table
time: timestamp[ms]
in.tensor: list<item: double> not null
child 0, item: double
out.variable: list<inner: float not null> not null
child 0, inner: float not null
anomaly.count: uint32 not null
----
time: [[2024-02-09 16:28:44.753]]
in.tensor: [[[4,2.5,2900,5505,2,...,2970,5251,12,0,0]]]
out.variable: [[[718013.7]]]
anomaly.count: [[0]]
result = mainpipeline.infer(normal_input, dataset=["*", "metadata.pipeline_version"])
display(result)
time | in.tensor | out.variable | anomaly.count | metadata.pipeline_version | |
---|---|---|---|---|---|
0 | 2024-02-09 16:31:30.617 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.7] | 0 |
The following displays the pipeline metadata logs.
Standard Pipeline Steps Log Requests
Effected pipeline steps:
add_model_step
replace_with_model_step
For log file requests, the following metadata dataset requests for standard pipeline steps are available:
metadata
These must be paired with specific columns. *
is not available when paired with metadata
.
in
: All input fields.out
: All output fields.time
: The DateTime the inference request was made.in.{input_fields}
: Any input fields (tensor
, etc.)out.{output_fields}
: Any output fields (out.house_price
,out.variable
, etc.)anomaly.count
: Any anomalies detected from validations.anomaly.{validation}
: The validation that triggered the anomaly detection and whether it isTrue
(indicating an anomaly was detected) orFalse
.
The following requests the metadata, and displays the output variable and last model from the metadata.
# Display metadata
metadatalogs = mainpipeline.logs(dataset=['time', "out","metadata"])
display("Metadata Logs")
display(metadatalogs.loc[:, ['time', 'out.variable', 'metadata.last_model']])
Pipeline log schema has changed over the logs requested 2 newest records retrieved successfully, newest record seen was at <datetime>. Please request additional records separately
'Metadata Logs'
time | out.variable | metadata.last_model | |
---|---|---|---|
0 | 2024-02-09 16:28:44.753 | [718013.7] | {"model_name":"logcontrol","model_sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"} |
1 | 2024-02-09 16:31:30.617 | [718013.7] | {"model_name":"logcontrol","model_sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"} |
Pipeline Limits
In a previous step we performed 10,000 inferences at once. If we attempt to pull them at once, we’ll likely run into the size limit for this pipeline and receive the following warning message indicating that the pipeline size limits were exceeded and we should use export_logs
instead.
Warning: Pipeline log size limit exceeded. Only displaying 1000 log messages (of 10000 requested). Please request a file using export_logs.
logs = mainpipeline.logs(limit=10000)
display(logs)
Pipeline log schema has changed over the logs requested 2 newest records retrieved successfully, newest record seen was at <datetime>. Please request additional records separately
time | in.tensor | out.variable | anomaly.count | |
---|---|---|---|---|
0 | 2024-02-09 16:28:44.753 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.7] | 0 |
1 | 2024-02-09 16:31:30.617 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.7] | 0 |
Pipeline export_logs Method
The Pipeline method export_logs
returns the Pipeline records as either a DataFrame JSON file, or an Apache Arrow table file. For a complete list, see the Wallaroo SDK Essentials Guide: Pipeline Log Management.
The export_logs
method takes the following parameters:
Parameter | Type | Description |
---|---|---|
directory | String (Optional) (Default: logs ) | Logs are exported to a file from current working directory to directory . |
data_size_limit | String (Optional) ((Default: 100MB ) | The maximum size for the exported data in bytes. Note that file size is approximate to the request; a request of 10MiB may return 10.3MB of data. The fields are in the format “{size as number} {unit value}”, and can include a space so “10 MiB” and “10MiB” are the same. The accepted unit values are:
|
file_prefix | String (Optional) (Default: The name of the pipeline) | The name of the exported files. By default, this will the name of the pipeline and is segmented by pipeline version between the limits or the start and end period. For example: ’logpipeline-1.json`, etc. |
limit | Int (Optional) | Limits how many log records to display. Defaults to 100 . If there are more pipeline logs than are being displayed, the Warning message Pipeline log record limit exceeded will be displayed. For example, if 100 log files were requested and there are a total of 1,000, the warning message will be displayed. |
start and end | DateTime (Optional) | Limits logs to all logs between the start and end DateTime parameters. Both parameters must be provided. Submitting a logs() request with only start or end will generate an exception.If start and end are provided as parameters, then the records are returned in chronological order, with the oldest record displayed first. |
dataset | List (OPTIONAL) | The datasets to be returned. The datasets available are:
metadata.elapsed : IMPORTANT NOTE: See Metadata Requests Restrictionsfor specifications on how this dataset can be used with other datasets.
|
arrow | Boolean (Optional) | Defaults to False. If arrow is set to True , then the logs are returned as an Apache Arrow table. If arrow=False , then the logs are returned as JSON in pandas DataFrame format. |
The following examples demonstrate saving a DataFrame version of the mainpipeline
logs, then an Arrow version.
# Save the DataFrame version of the log file
mainpipeline.export_logs()
display(os.listdir('./logs'))
mainpipeline.export_logs(arrow=True)
display(os.listdir('./logs'))
Warning: There are more logs available. Please set a larger limit to export more data.
Note: The logs with different schemas are written to separate files in the provided directory.
[’logpipeline-test-1.arrow’,
’logpipeline-test-2.arrow’,
’logpipeline-test-2.json’,
’logpipeline-1.json’,
’logpipeline-test-1.json’,
’logpipeline-1.arrow']
Warning: There are more logs available. Please set a larger limit to export more data.
Note: The logs with different schemas are written to separate files in the provided directory.
[’logpipeline-test-1.arrow’,
’logpipeline-test-2.arrow’,
’logpipeline-test-2.json’,
’logpipeline-1.json’,
’logpipeline-test-1.json’,
’logpipeline-1.arrow’]
Shadow Deploy Pipelines
Let’s assume that after analyzing the assay information we want to test two challenger models to our control. We do that with the Shadow Deploy pipeline step.
In Shadow Deploy, the pipeline step is added with the add_shadow_deploy
method, with the champion model listed first, then an array of challenger models after. All inference data is fed to all models, with the champion results displayed in the out.variable
column, and the shadow results in the format out_{model name}.variable
. For example, since we named our challenger models housingchallenger01
and housingchallenger02
, the columns out_housingchallenger01.variable
and out_housingchallenger02.variable
have the shadow deployed model results.
For this example, we will remove the previous pipeline step, then replace it with a shadow deploy step with rf_model.onnx
as our champion, and models xgb_model.onnx
and gbr_model.onnx
as the challengers. We’ll deploy the pipeline and prepare it for sample inferences.
# Upload the challenger models
model_name_challenger01 = 'logcontrolchallenger01'
model_file_name_challenger01 = './models/xgb_model.onnx'
model_name_challenger02 = 'logcontrolchallenger02'
model_file_name_challenger02 = './models/gbr_model.onnx'
housing_model_challenger01 = (wl.upload_model(model_name_challenger01,
model_file_name_challenger01,
framework=wallaroo.framework.Framework.ONNX)
.configure(tensor_fields=["tensor"])
)
housing_model_challenger02 = (wl.upload_model(model_name_challenger02,
model_file_name_challenger02,
framework=wallaroo.framework.Framework.ONNX)
.configure(tensor_fields=["tensor"])
)
# Undeploy the pipeline
mainpipeline.undeploy()
mainpipeline.clear()
# Add the new shadow deploy step with our challenger models
mainpipeline.add_shadow_deploy(housing_model_control, [housing_model_challenger01, housing_model_challenger02])
# Deploy the pipeline with the new shadow step
deploy_config = wallaroo.deployment_config.DeploymentConfigBuilder() \
.cpus(0.25)\
.build()
mainpipeline.deploy(deployment_config=deploy_config)
Waiting for undeployment - this will take up to 45s ................................... ok
Waiting for deployment - this will take up to 45s ........ ok
name | logpipeline-test |
---|---|
created | 2024-02-09 16:21:09.406182+00:00 |
last_updated | 2024-02-09 16:33:08.547068+00:00 |
deployed | True |
arch | None |
tags | |
versions | e143a2d5-5641-4dcc-8ae4-786fd777a30a, e2b9d903-4015-4d09-902b-9150a7196cea, 9df38be1-d2f4-4be1-9022-8f0570a238b9, 3078b49f-3eff-48d1-8d9b-a8780b329ecc, 21bff9df-828f-40e7-8a22-449a2e636b44, f78a7030-bd25-4bf7-ba0d-a18cfe3790e0, 10c1ac25-d626-4413-8d5d-1bed42d0e65c, b179b693-b6b6-4ff9-b2a4-2a639d88bc9b, da7b9cf0-81e8-452b-8b70-689406dc9548, a9a9b62c-9d37-427f-99af-67725558bf9b, 1c14591a-96b4-4059-bb63-2d2bc4e308d5, add660ac-0ebf-4a24-bb6d-6cdc875866c8 |
steps | logcontrol |
published | False |
Shadow Deploy Sample Inference
We’ll now use our same sample data for an inference to our shadow deployed pipeline, then display the first 20 results with just the comparative outputs.
shadow_date_start = datetime.datetime.now()
shadow_result = mainpipeline.infer_from_file('./data/xtest-1k.arrow')
shadow_outputs = shadow_result.to_pandas()
display(shadow_outputs.loc[0:20,['out.variable','out_logcontrolchallenger01.variable','out_logcontrolchallenger02.variable']])
shadow_date_end = datetime.datetime.now()
out.variable | out_logcontrolchallenger01.variable | out_logcontrolchallenger02.variable | |
---|---|---|---|
0 | [718013.75] | [659806.0] | [704901.9] |
1 | [615094.56] | [732883.5] | [695994.44] |
2 | [448627.72] | [419508.84] | [416164.8] |
3 | [758714.2] | [634028.8] | [655277.2] |
4 | [513264.7] | [427209.44] | [426854.66] |
5 | [668288.0] | [615501.9] | [632556.1] |
6 | [1004846.5] | [1139732.5] | [1100465.2] |
7 | [684577.2] | [498328.88] | [528278.06] |
8 | [727898.1] | [722664.4] | [659439.94] |
9 | [559631.1] | [525746.44] | [534331.44] |
10 | [340764.53] | [376337.1] | [377187.2] |
11 | [442168.06] | [382053.12] | [403964.3] |
12 | [630865.6] | [505608.97] | [528991.3] |
13 | [559631.1] | [603260.5] | [612201.75] |
14 | [909441.1] | [969585.4] | [893874.7] |
15 | [313096.0] | [313633.75] | [318054.94] |
16 | [404040.8] | [360413.56] | [357816.75] |
17 | [292859.5] | [316674.94] | [294034.7] |
18 | [338357.88] | [299907.44] | [323254.3] |
19 | [682284.6] | [811896.75] | [770916.7] |
20 | [583765.94] | [573618.5] | [549141.4] |
Shadow Deploy Logs
Pipelines with a shadow deployed step include the shadow inference result in the same format as the inference result: inference results from shadow deployed models are displayed as out_{model name}.{output variable}
.
# display logs with shadow deployed steps
display(mainpipeline.logs(start_datetime=shadow_date_start, end_datetime=shadow_date_end).loc[:, ["time", "out.variable", "out_logcontrolchallenger01.variable", "out_logcontrolchallenger02.variable"]])
Warning: Pipeline log size limit exceeded. Please request logs using export_logs
time | out.variable | out_logcontrolchallenger01.variable | out_logcontrolchallenger02.variable | |
---|---|---|---|---|
0 | 2024-02-09 16:33:18.093 | [718013.75] | [659806.0] | [704901.9] |
1 | 2024-02-09 16:33:18.093 | [615094.56] | [732883.5] | [695994.44] |
2 | 2024-02-09 16:33:18.093 | [448627.72] | [419508.84] | [416164.8] |
3 | 2024-02-09 16:33:18.093 | [758714.2] | [634028.8] | [655277.2] |
4 | 2024-02-09 16:33:18.093 | [513264.7] | [427209.44] | [426854.66] |
... | ... | ... | ... | ... |
495 | 2024-02-09 16:33:18.093 | [873315.0] | [779848.6] | [771244.75] |
496 | 2024-02-09 16:33:18.093 | [721143.6] | [607252.1] | [610430.56] |
497 | 2024-02-09 16:33:18.093 | [1048372.4] | [844343.56] | [900959.4] |
498 | 2024-02-09 16:33:18.093 | [244566.38] | [251694.84] | [246188.81] |
499 | 2024-02-09 16:33:18.093 | [518869.0] | [482136.66] | [547725.56] |
500 rows × 4 columns
For log file requests, the following metadata dataset requests for testing pipeline steps are available:
metadata
These must be paired with specific columns. *
is not available when paired with metadata
.
in
: All input fields.out
: All output fields.time
: The DateTime the inference request was made.in.{input_fields}
: Any input fields (tensor
, etc.).out.{output_fields}
: Any output fields matching the specificoutput_field
(out.house_price
,out.variable
, etc.).out_
: All shadow deployed challenger steps Any output fields matching the specificoutput_field
(out.house_price
,out.variable
, etc.).anomaly.count
: Any anomalies detected from validations.anomaly.{validation}
: The validation that triggered the anomaly detection and whether it isTrue
(indicating an anomaly was detected) orFalse
.
The following example retrieves the logs from a pipeline with shadow deployed models, and displays the specific shadow deployed model outputs and the metadata.elasped
field.
# display logs with shadow deployed steps
display(mainpipeline.logs(start_datetime=shadow_date_start, end_datetime=shadow_date_end).loc[:, ["time",
"out.variable",
"out_logcontrolchallenger01.variable",
"out_logcontrolchallenger02.variable"
]
])
Warning: Pipeline log size limit exceeded. Please request logs using export_logs
time | out.variable | out_logcontrolchallenger01.variable | out_logcontrolchallenger02.variable | |
---|---|---|---|---|
0 | 2024-02-09 16:33:18.093 | [718013.75] | [659806.0] | [704901.9] |
1 | 2024-02-09 16:33:18.093 | [615094.56] | [732883.5] | [695994.44] |
2 | 2024-02-09 16:33:18.093 | [448627.72] | [419508.84] | [416164.8] |
3 | 2024-02-09 16:33:18.093 | [758714.2] | [634028.8] | [655277.2] |
4 | 2024-02-09 16:33:18.093 | [513264.7] | [427209.44] | [426854.66] |
... | ... | ... | ... | ... |
495 | 2024-02-09 16:33:18.093 | [873315.0] | [779848.6] | [771244.75] |
496 | 2024-02-09 16:33:18.093 | [721143.6] | [607252.1] | [610430.56] |
497 | 2024-02-09 16:33:18.093 | [1048372.4] | [844343.56] | [900959.4] |
498 | 2024-02-09 16:33:18.093 | [244566.38] | [251694.84] | [246188.81] |
499 | 2024-02-09 16:33:18.093 | [518869.0] | [482136.66] | [547725.56] |
500 rows × 4 columns
metadatalogs = mainpipeline.logs(dataset=["time",
"out_logcontrolchallenger01.variable",
"out_logcontrolchallenger02.variable",
"metadata",
'anomaly.count'
],
start_datetime=shadow_date_start,
end_datetime=shadow_date_end
)
display(metadatalogs.loc[:, ['out_logcontrolchallenger01.variable',
'out_logcontrolchallenger02.variable',
'metadata.elapsed',
'anomaly.count'
]
])
Warning: Pipeline log size limit exceeded. Please request logs using export_logs
out_logcontrolchallenger01.variable | out_logcontrolchallenger02.variable | metadata.elapsed | anomaly.count | |
---|---|---|---|---|
0 | [659806.0] | [704901.9] | [325472, 124071] | 0 |
1 | [732883.5] | [695994.44] | [325472, 124071] | 0 |
2 | [419508.84] | [416164.8] | [325472, 124071] | 0 |
3 | [634028.8] | [655277.2] | [325472, 124071] | 0 |
4 | [427209.44] | [426854.66] | [325472, 124071] | 0 |
... | ... | ... | ... | ... |
495 | [779848.6] | [771244.75] | [325472, 124071] | 0 |
496 | [607252.1] | [610430.56] | [325472, 124071] | 0 |
497 | [844343.56] | [900959.4] | [325472, 124071] | 0 |
498 | [251694.84] | [246188.81] | [325472, 124071] | 0 |
499 | [482136.66] | [547725.56] | [325472, 124071] | 0 |
500 rows × 4 columns
The following demonstrates exporting the shadow deployed logs to the directory shadow
.
# Save shadow deployed log files as pandas DataFrame
mainpipeline.export_logs(directory="shadow", file_prefix="shadowdeploylogs")
display(os.listdir('./shadow'))
Warning: There are more logs available. Please set a larger limit to export more data.
Note: The logs with different schemas are written to separate files in the provided directory.
[‘shadowdeploylogs-2.json’, ‘shadowdeploylogs-1.json’]
A/B Testing Pipeline
A/B testing allows inference requests to be split between a control model and one or more challenger models. For full details, see the Pipeline Management Guide: A/B Testing.
When the inference results and log entries are displayed, they include the column out._model_split
which displays:
Field | Type | Description |
---|---|---|
name | String | The model name used for the inference. |
version | String | The version of the model. |
sha | String | The sha hash of the model version. |
For this example, the shadow deployed step will be removed and replaced with an A/B Testing step with the ratio 1:1:1, so the control and each of the challenger models will be split randomly between inference requests. A set of sample inferences will be run, then the pipeline logs displayed.
pipeline = (wl.build_pipeline(“randomsplitpipeline-demo”)
.add_random_split([(2, control), (1, challenger)], “session_id”))
mainpipeline.undeploy()
# remove the shadow deploy steps
mainpipeline.clear()
# Add the a/b test step to the pipeline
mainpipeline.add_random_split([(1, housing_model_control), (1, housing_model_challenger01), (1, housing_model_challenger02)], "session_id")
deploy_config = wallaroo.deployment_config.DeploymentConfigBuilder() \
.cpus(0.25)\
.build()
mainpipeline.deploy(deployment_config=deploy_config)
# Perform sample inferences of 20 rows and display the results
ab_date_start = datetime.datetime.now()
abtesting_inputs = pd.read_json('./data/xtest-1k.df.json')
for index, row in abtesting_inputs.sample(20).iterrows():
display(mainpipeline.infer(row.to_frame('tensor').reset_index()).loc[:,["out._model_split", "out.variable"]])
ab_date_end = datetime.datetime.now()
Waiting for undeployment - this will take up to 45s ..................................... ok
Waiting for deployment - this will take up to 45s ......... ok
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger01","version":"5b63884e-3f09-4e90-9f09-213350b9c445","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}] | [300542.5] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger01","version":"5b63884e-3f09-4e90-9f09-213350b9c445","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}] | [580584.3] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrol","version":"1f93edce-3f3e-4d29-be29-6a4e9303da05","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [447162.84] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrol","version":"1f93edce-3f3e-4d29-be29-6a4e9303da05","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [581002.94] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger01","version":"5b63884e-3f09-4e90-9f09-213350b9c445","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}] | [944906.25] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger02","version":"6fc54099-7151-48d7-9e57-6d989fb9bb1c","sha":"ed6065a79d841f7e96307bb20d5ef22840f15da0b587efb51425c7ad60589d6a"}] | [488997.9] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrol","version":"1f93edce-3f3e-4d29-be29-6a4e9303da05","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [373955.94] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger01","version":"5b63884e-3f09-4e90-9f09-213350b9c445","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}] | [868765.4] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger02","version":"6fc54099-7151-48d7-9e57-6d989fb9bb1c","sha":"ed6065a79d841f7e96307bb20d5ef22840f15da0b587efb51425c7ad60589d6a"}] | [499459.2] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrol","version":"1f93edce-3f3e-4d29-be29-6a4e9303da05","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [559631.06] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger01","version":"5b63884e-3f09-4e90-9f09-213350b9c445","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}] | [344156.25] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger02","version":"6fc54099-7151-48d7-9e57-6d989fb9bb1c","sha":"ed6065a79d841f7e96307bb20d5ef22840f15da0b587efb51425c7ad60589d6a"}] | [296829.75] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger02","version":"6fc54099-7151-48d7-9e57-6d989fb9bb1c","sha":"ed6065a79d841f7e96307bb20d5ef22840f15da0b587efb51425c7ad60589d6a"}] | [532923.94] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger02","version":"6fc54099-7151-48d7-9e57-6d989fb9bb1c","sha":"ed6065a79d841f7e96307bb20d5ef22840f15da0b587efb51425c7ad60589d6a"}] | [878232.2] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger02","version":"6fc54099-7151-48d7-9e57-6d989fb9bb1c","sha":"ed6065a79d841f7e96307bb20d5ef22840f15da0b587efb51425c7ad60589d6a"}] | [996693.6] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger02","version":"6fc54099-7151-48d7-9e57-6d989fb9bb1c","sha":"ed6065a79d841f7e96307bb20d5ef22840f15da0b587efb51425c7ad60589d6a"}] | [544343.3] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrol","version":"1f93edce-3f3e-4d29-be29-6a4e9303da05","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [379076.28] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger01","version":"5b63884e-3f09-4e90-9f09-213350b9c445","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}] | [585684.3] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrolchallenger01","version":"5b63884e-3f09-4e90-9f09-213350b9c445","sha":"31e92d6ccb27b041a324a7ac22cf95d9d6cc3aa7e8263a229f7c4aec4938657c"}] | [573976.44] |
out._model_split | out.variable | |
---|---|---|
0 | [{"name":"logcontrol","version":"1f93edce-3f3e-4d29-be29-6a4e9303da05","sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"}] | [310164.06] |
## Get the logs with the a/b testing information
metadatalogs = mainpipeline.logs(dataset=["time",
"out",
"metadata"
]
)
display(metadatalogs.loc[:, ['out.variable', 'metadata.last_model']])
Pipeline log schema has changed over the logs requested 2 newest records retrieved successfully, newest record seen was at <datetime>. Please request additional records separately
out.variable | metadata.last_model | |
---|---|---|
0 | [718013.7] | {"model_name":"logcontrol","model_sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"} |
1 | [718013.7] | {"model_name":"logcontrol","model_sha":"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6"} |
# Save a/b testing log files as DataFrame
mainpipeline.export_logs(directory="abtesting",
file_prefix="abtests",
start_datetime=ab_date_start,
end_datetime=ab_date_end)
display(os.listdir('./abtesting'))
['abtests-1.json']
The following exports the metadata with the log files.
# Save a/b testing log files as DataFrame
mainpipeline.export_logs(directory="abtesting-metadata",
file_prefix="abtests",
start_datetime=ab_date_start,
end_datetime=ab_date_end,
dataset=["time", "out", "metadata"])
display(os.listdir('./abtesting-metadata'))
['abtests-1.json']
Anomaly Detection Logs
Wallaroo provides validations to detect anomalous data from inference inputs and outputs. Validations are added to a Wallaroo pipeline with the wallaroo.pipeline.add_validations
method.
Adding validations takes the format:
pipeline.add_validations(
validation_name_01 = polars.col(in|out.{column_name}) EXPRESSION,
validation_name_02 = polars.col(in|out.{column_name}) EXPRESSION
...{additional rules}
)
validation_name
: The user provided name of the validation. The names must match Python variable naming requirements.- IMPORTANT NOTE: Using the name
count
as a validation name returns an error. Any validation rules namedcount
are dropped upon request and an error returned.
- IMPORTANT NOTE: Using the name
polars.col(in|out.{column_name})
: Specifies the input or output for a specific field aka “column” in an inference result. Wallaroo inference requests are in the formatin.{field_name}
for inputs, andout.{field_name}
for outputs.- More than one field can be selected, as long as they follow the rules of the polars 0.18 Expressions library.
EXPRESSION
: The expression to validate. When the expression returns True, that indicates an anomaly detected.
The polars
library version 0.18.5 is used to create the validation rule. This is installed by default with the Wallaroo SDK. This provides a powerful range of comparisons to organizations tracking anomalous data from their ML models.
When validations are added to a pipeline, inference request outputs return the following fields:
Field | Type | Description |
---|---|---|
anomaly.count | Integer | The total of all validations that returned True. |
anomaly.{validation name} | Bool | The output of the validation {validation_name} . |
When validation returns True
, an anomaly is detected.
For example, adding the validation fraud
to the following pipeline returns anomaly.count
of 1
when the validation fraud
returns True
. The validation fraud
returns True
when the output field dense_1 at index 0 is greater than 0.9.
sample_pipeline = wallaroo.client.build_pipeline("sample-pipeline")
sample_pipeline.add_model_step(model)
# add the validation
sample_pipeline.add_validations(
fraud=pl.col("out.dense_1").list.get(0) > 0.9,
)
# deploy the pipeline
sample_pipeline.deploy()
# sample inference
display(sample_pipeline.infer_from_file("dev_high_fraud.json", data_format='pandas-records'))
 | time | in.tensor | out.dense_1 | anomaly.count | anomaly.fraud |
---|---|---|---|---|---|
0 | 2024-02-02 16:05:42.152 | [1.0678324729, 18.1555563975, -1.6589551058, 5…] | [0.981199] | 1 | True |
Anomaly Detection Inference Requests Example
For this example, we create the validation rule too_high
which detects houses with a value greater than 1,000,000 and show the output for houses that trigger that validation.
For these examples we’ll create a new pipeline to ensure the logs are “clean” for the samples.
import polars as pl
mainpipeline.undeploy()
mainpipeline.clear()
mainpipeline.add_model_step(housing_model_control)
mainpipeline.add_validations(
too_high=pl.col("out.variable").list.get(0) > 1000000.0
)
deploy_config = wallaroo.deployment_config.DeploymentConfigBuilder() \
.cpus(0.25)\
.build()
mainpipeline.deploy(deployment_config=deploy_config)
Waiting for undeployment - this will take up to 45s ...................................... ok
Waiting for deployment - this will take up to 45s ......... ok
name | logpipeline-test |
---|---|
created | 2024-02-09 16:21:09.406182+00:00 |
last_updated | 2024-02-09 16:53:37.061953+00:00 |
deployed | True |
arch | None |
tags | |
versions | 764c7706-c996-42e9-90ff-87b1b496f98d, 05c46dbc-9d72-40d5-bc4c-7fee7bc3e971, 9a4d76f5-9905-4063-8bf8-47e103987515, d5e4882a-3c17-4965-b059-66432a50a3cd, 00b3d5e7-4644-4138-b73d-b0511b3c9e2a, e143a2d5-5641-4dcc-8ae4-786fd777a30a, e2b9d903-4015-4d09-902b-9150a7196cea, 9df38be1-d2f4-4be1-9022-8f0570a238b9, 3078b49f-3eff-48d1-8d9b-a8780b329ecc, 21bff9df-828f-40e7-8a22-449a2e636b44, f78a7030-bd25-4bf7-ba0d-a18cfe3790e0, 10c1ac25-d626-4413-8d5d-1bed42d0e65c, b179b693-b6b6-4ff9-b2a4-2a639d88bc9b, da7b9cf0-81e8-452b-8b70-689406dc9548, a9a9b62c-9d37-427f-99af-67725558bf9b, 1c14591a-96b4-4059-bb63-2d2bc4e308d5, add660ac-0ebf-4a24-bb6d-6cdc875866c8 |
steps | logcontrol |
published | False |
import datetime
import time
import pytz
inference_start = datetime.datetime.now(pytz.utc)
# adding sleep to ensure log distinction
time.sleep(15)
results = mainpipeline.infer_from_file('./data/test-1000.df.json')
inference_end = datetime.datetime.now(pytz.utc)
# first 20 results
display(results.head(20))
# only results that trigger the anomaly too_high
results.loc[results['anomaly.too_high'] == True]
time | in.tensor | out.variable | anomaly.count | anomaly.too_high | |
---|---|---|---|---|---|
0 | 2024-02-09 16:54:02.507 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.75] | 0 | False |
1 | 2024-02-09 16:54:02.507 | [2.0, 2.5, 2170.0, 6361.0, 1.0, 0.0, 2.0, 3.0, 8.0, 2170.0, 0.0, 47.7109, -122.017, 2310.0, 7419.0, 6.0, 0.0, 0.0] | [615094.56] | 0 | False |
2 | 2024-02-09 16:54:02.507 | [3.0, 2.5, 1300.0, 812.0, 2.0, 0.0, 0.0, 3.0, 8.0, 880.0, 420.0, 47.5893, -122.317, 1300.0, 824.0, 6.0, 0.0, 0.0] | [448627.72] | 0 | False |
3 | 2024-02-09 16:54:02.507 | [4.0, 2.5, 2500.0, 8540.0, 2.0, 0.0, 0.0, 3.0, 9.0, 2500.0, 0.0, 47.5759, -121.994, 2560.0, 8475.0, 24.0, 0.0, 0.0] | [758714.2] | 0 | False |
4 | 2024-02-09 16:54:02.507 | [3.0, 1.75, 2200.0, 11520.0, 1.0, 0.0, 0.0, 4.0, 7.0, 2200.0, 0.0, 47.7659, -122.341, 1690.0, 8038.0, 62.0, 0.0, 0.0] | [513264.7] | 0 | False |
5 | 2024-02-09 16:54:02.507 | [3.0, 2.0, 2140.0, 4923.0, 1.0, 0.0, 0.0, 4.0, 8.0, 1070.0, 1070.0, 47.6902, -122.339, 1470.0, 4923.0, 86.0, 0.0, 0.0] | [668288.0] | 0 | False |
6 | 2024-02-09 16:54:02.507 | [4.0, 3.5, 3590.0, 5334.0, 2.0, 0.0, 2.0, 3.0, 9.0, 3140.0, 450.0, 47.6763, -122.267, 2100.0, 6250.0, 9.0, 0.0, 0.0] | [1004846.5] | 1 | True |
7 | 2024-02-09 16:54:02.507 | [3.0, 2.0, 1280.0, 960.0, 2.0, 0.0, 0.0, 3.0, 9.0, 1040.0, 240.0, 47.602, -122.311, 1280.0, 1173.0, 0.0, 0.0, 0.0] | [684577.2] | 0 | False |
8 | 2024-02-09 16:54:02.507 | [4.0, 2.5, 2820.0, 15000.0, 2.0, 0.0, 0.0, 4.0, 9.0, 2820.0, 0.0, 47.7255, -122.101, 2440.0, 15000.0, 29.0, 0.0, 0.0] | [727898.1] | 0 | False |
9 | 2024-02-09 16:54:02.507 | [3.0, 2.25, 1790.0, 11393.0, 1.0, 0.0, 0.0, 3.0, 8.0, 1790.0, 0.0, 47.6297, -122.099, 2290.0, 11894.0, 36.0, 0.0, 0.0] | [559631.1] | 0 | False |
10 | 2024-02-09 16:54:02.507 | [3.0, 1.5, 1010.0, 7683.0, 1.5, 0.0, 0.0, 5.0, 7.0, 1010.0, 0.0, 47.72, -122.318, 1550.0, 7271.0, 61.0, 0.0, 0.0] | [340764.53] | 0 | False |
11 | 2024-02-09 16:54:02.507 | [3.0, 2.0, 1270.0, 1323.0, 3.0, 0.0, 0.0, 3.0, 8.0, 1270.0, 0.0, 47.6934, -122.342, 1330.0, 1323.0, 8.0, 0.0, 0.0] | [442168.06] | 0 | False |
12 | 2024-02-09 16:54:02.507 | [4.0, 1.75, 2070.0, 9120.0, 1.0, 0.0, 0.0, 4.0, 7.0, 1250.0, 820.0, 47.6045, -122.123, 1650.0, 8400.0, 57.0, 0.0, 0.0] | [630865.6] | 0 | False |
13 | 2024-02-09 16:54:02.507 | [4.0, 1.0, 1620.0, 4080.0, 1.5, 0.0, 0.0, 3.0, 7.0, 1620.0, 0.0, 47.6696, -122.324, 1760.0, 4080.0, 91.0, 0.0, 0.0] | [559631.1] | 0 | False |
14 | 2024-02-09 16:54:02.507 | [4.0, 3.25, 3990.0, 9786.0, 2.0, 0.0, 0.0, 3.0, 9.0, 3990.0, 0.0, 47.6784, -122.026, 3920.0, 8200.0, 10.0, 0.0, 0.0] | [909441.1] | 0 | False |
15 | 2024-02-09 16:54:02.507 | [4.0, 2.0, 1780.0, 19843.0, 1.0, 0.0, 0.0, 3.0, 7.0, 1780.0, 0.0, 47.4414, -122.154, 2210.0, 13500.0, 52.0, 0.0, 0.0] | [313096.0] | 0 | False |
16 | 2024-02-09 16:54:02.507 | [4.0, 2.5, 2130.0, 6003.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2130.0, 0.0, 47.4518, -122.12, 1940.0, 4529.0, 11.0, 0.0, 0.0] | [404040.8] | 0 | False |
17 | 2024-02-09 16:54:02.507 | [3.0, 1.75, 1660.0, 10440.0, 1.0, 0.0, 0.0, 3.0, 7.0, 1040.0, 620.0, 47.4448, -121.77, 1240.0, 10380.0, 36.0, 0.0, 0.0] | [292859.5] | 0 | False |
18 | 2024-02-09 16:54:02.507 | [3.0, 2.5, 2110.0, 4118.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2110.0, 0.0, 47.3878, -122.153, 2110.0, 4044.0, 25.0, 0.0, 0.0] | [338357.88] | 0 | False |
19 | 2024-02-09 16:54:02.507 | [4.0, 2.25, 2200.0, 11250.0, 1.5, 0.0, 0.0, 5.0, 7.0, 1300.0, 900.0, 47.6845, -122.201, 2320.0, 10814.0, 94.0, 0.0, 0.0] | [682284.6] | 0 | False |
time | in.tensor | out.variable | anomaly.count | anomaly.too_high | |
---|---|---|---|---|---|
6 | 2024-02-09 16:54:02.507 | [4.0, 3.5, 3590.0, 5334.0, 2.0, 0.0, 2.0, 3.0, 9.0, 3140.0, 450.0, 47.6763, -122.267, 2100.0, 6250.0, 9.0, 0.0, 0.0] | [1004846.5] | 1 | True |
30 | 2024-02-09 16:54:02.507 | [4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0, 10.0, 2760.0, 950.0, 47.6696, -122.261, 3970.0, 20000.0, 79.0, 0.0, 0.0] | [1514079.8] | 1 | True |
40 | 2024-02-09 16:54:02.507 | [4.0, 4.5, 5120.0, 41327.0, 2.0, 0.0, 0.0, 3.0, 10.0, 3290.0, 1830.0, 47.7009, -122.059, 3360.0, 82764.0, 6.0, 0.0, 0.0] | [1204324.8] | 1 | True |
63 | 2024-02-09 16:54:02.507 | [4.0, 3.0, 4040.0, 19700.0, 2.0, 0.0, 0.0, 3.0, 11.0, 4040.0, 0.0, 47.7205, -122.127, 3930.0, 21887.0, 27.0, 0.0, 0.0] | [1028923.06] | 1 | True |
110 | 2024-02-09 16:54:02.507 | [4.0, 2.5, 3470.0, 20445.0, 2.0, 0.0, 0.0, 4.0, 10.0, 3470.0, 0.0, 47.547, -122.219, 3360.0, 21950.0, 51.0, 0.0, 0.0] | [1412215.3] | 1 | True |
130 | 2024-02-09 16:54:02.507 | [4.0, 2.75, 2620.0, 13777.0, 1.5, 0.0, 2.0, 4.0, 9.0, 1720.0, 900.0, 47.58, -122.285, 3530.0, 9287.0, 88.0, 0.0, 0.0] | [1223839.1] | 1 | True |
133 | 2024-02-09 16:54:02.507 | [5.0, 2.25, 3320.0, 13138.0, 1.0, 0.0, 2.0, 4.0, 9.0, 1900.0, 1420.0, 47.759, -122.269, 2820.0, 13138.0, 51.0, 0.0, 0.0] | [1108000.1] | 1 | True |
154 | 2024-02-09 16:54:02.507 | [4.0, 2.75, 3800.0, 9606.0, 2.0, 0.0, 0.0, 3.0, 9.0, 3800.0, 0.0, 47.7368, -122.208, 3400.0, 9677.0, 6.0, 0.0, 0.0] | [1039781.25] | 1 | True |
160 | 2024-02-09 16:54:02.507 | [5.0, 3.5, 4150.0, 13232.0, 2.0, 0.0, 0.0, 3.0, 11.0, 4150.0, 0.0, 47.3417, -122.182, 3840.0, 15121.0, 9.0, 0.0, 0.0] | [1042119.1] | 1 | True |
210 | 2024-02-09 16:54:02.507 | [4.0, 3.5, 4300.0, 70407.0, 2.0, 0.0, 0.0, 3.0, 10.0, 2710.0, 1590.0, 47.4472, -122.092, 3520.0, 26727.0, 22.0, 0.0, 0.0] | [1115275.0] | 1 | True |
239 | 2024-02-09 16:54:02.507 | [4.0, 3.25, 5010.0, 49222.0, 2.0, 0.0, 0.0, 5.0, 9.0, 3710.0, 1300.0, 47.5489, -122.092, 3140.0, 54014.0, 36.0, 0.0, 0.0] | [1092274.1] | 1 | True |
248 | 2024-02-09 16:54:02.507 | [4.0, 3.75, 4410.0, 8112.0, 3.0, 0.0, 4.0, 3.0, 11.0, 3570.0, 840.0, 47.5888, -122.392, 2770.0, 5750.0, 12.0, 0.0, 0.0] | [1967344.1] | 1 | True |
255 | 2024-02-09 16:54:02.507 | [4.0, 3.0, 4750.0, 21701.0, 1.5, 0.0, 0.0, 5.0, 11.0, 4750.0, 0.0, 47.6454, -122.218, 3120.0, 18551.0, 38.0, 0.0, 0.0] | [2002393.5] | 1 | True |
271 | 2024-02-09 16:54:02.507 | [5.0, 3.25, 5790.0, 13726.0, 2.0, 0.0, 3.0, 3.0, 10.0, 4430.0, 1360.0, 47.5388, -122.114, 5790.0, 13726.0, 0.0, 0.0, 0.0] | [1189654.4] | 1 | True |
281 | 2024-02-09 16:54:02.507 | [3.0, 3.0, 3570.0, 6250.0, 2.0, 0.0, 2.0, 3.0, 10.0, 2710.0, 860.0, 47.5624, -122.399, 2550.0, 7596.0, 30.0, 0.0, 0.0] | [1124493.3] | 1 | True |
282 | 2024-02-09 16:54:02.507 | [3.0, 2.75, 3170.0, 34850.0, 1.0, 0.0, 0.0, 5.0, 9.0, 3170.0, 0.0, 47.6611, -122.169, 3920.0, 36740.0, 58.0, 0.0, 0.0] | [1227073.8] | 1 | True |
283 | 2024-02-09 16:54:02.507 | [4.0, 2.75, 3260.0, 19542.0, 1.0, 0.0, 0.0, 4.0, 10.0, 2170.0, 1090.0, 47.6245, -122.236, 3480.0, 19863.0, 46.0, 0.0, 0.0] | [1364650.3] | 1 | True |
285 | 2024-02-09 16:54:02.507 | [4.0, 2.75, 4020.0, 18745.0, 2.0, 0.0, 4.0, 4.0, 10.0, 2830.0, 1190.0, 47.6042, -122.21, 3150.0, 20897.0, 26.0, 0.0, 0.0] | [1322835.9] | 1 | True |
323 | 2024-02-09 16:54:02.507 | [3.0, 3.0, 2480.0, 5500.0, 2.0, 0.0, 3.0, 3.0, 10.0, 1730.0, 750.0, 47.6466, -122.404, 2950.0, 5670.0, 64.0, 1.0, 55.0] | [1100884.1] | 1 | True |
351 | 2024-02-09 16:54:02.507 | [5.0, 4.0, 4660.0, 9900.0, 2.0, 0.0, 2.0, 4.0, 9.0, 2600.0, 2060.0, 47.5135, -122.2, 3380.0, 9900.0, 35.0, 0.0, 0.0] | [1058105.0] | 1 | True |
360 | 2024-02-09 16:54:02.507 | [4.0, 3.5, 3770.0, 8501.0, 2.0, 0.0, 0.0, 3.0, 10.0, 3770.0, 0.0, 47.6744, -122.196, 1520.0, 9660.0, 6.0, 0.0, 0.0] | [1169643.0] | 1 | True |
398 | 2024-02-09 16:54:02.507 | [3.0, 2.25, 2390.0, 7875.0, 1.0, 0.0, 1.0, 3.0, 10.0, 1980.0, 410.0, 47.6515, -122.278, 3720.0, 9075.0, 66.0, 0.0, 0.0] | [1364149.9] | 1 | True |
414 | 2024-02-09 16:54:02.507 | [5.0, 3.5, 5430.0, 10327.0, 2.0, 0.0, 2.0, 3.0, 10.0, 4010.0, 1420.0, 47.5476, -122.116, 4340.0, 10324.0, 7.0, 0.0, 0.0] | [1207858.6] | 1 | True |
443 | 2024-02-09 16:54:02.507 | [5.0, 4.0, 4360.0, 8030.0, 2.0, 0.0, 0.0, 3.0, 10.0, 4360.0, 0.0, 47.5923, -121.973, 3570.0, 6185.0, 0.0, 0.0, 0.0] | [1160512.8] | 1 | True |
497 | 2024-02-09 16:54:02.507 | [4.0, 2.5, 4090.0, 11225.0, 2.0, 0.0, 0.0, 3.0, 10.0, 4090.0, 0.0, 47.581, -121.971, 3510.0, 8762.0, 9.0, 0.0, 0.0] | [1048372.4] | 1 | True |
513 | 2024-02-09 16:54:02.507 | [4.0, 3.25, 3320.0, 8587.0, 3.0, 0.0, 0.0, 3.0, 11.0, 2950.0, 370.0, 47.691, -122.337, 1860.0, 5668.0, 6.0, 0.0, 0.0] | [1130661.0] | 1 | True |
520 | 2024-02-09 16:54:02.507 | [5.0, 3.75, 4170.0, 8142.0, 2.0, 0.0, 2.0, 3.0, 10.0, 4170.0, 0.0, 47.5354, -122.181, 3030.0, 7980.0, 9.0, 0.0, 0.0] | [1098628.8] | 1 | True |
530 | 2024-02-09 16:54:02.507 | [4.0, 4.25, 3500.0, 8750.0, 1.0, 0.0, 4.0, 5.0, 9.0, 2140.0, 1360.0, 47.7222, -122.367, 3110.0, 8750.0, 63.0, 0.0, 0.0] | [1140733.8] | 1 | True |
535 | 2024-02-09 16:54:02.507 | [4.0, 3.5, 4460.0, 16271.0, 2.0, 0.0, 2.0, 3.0, 11.0, 4460.0, 0.0, 47.5862, -121.97, 4540.0, 17122.0, 13.0, 0.0, 0.0] | [1208638.0] | 1 | True |
556 | 2024-02-09 16:54:02.507 | [4.0, 3.5, 4285.0, 9567.0, 2.0, 0.0, 1.0, 5.0, 10.0, 3485.0, 800.0, 47.6434, -122.409, 2960.0, 6902.0, 68.0, 0.0, 0.0] | [1886959.4] | 1 | True |
623 | 2024-02-09 16:54:02.507 | [4.0, 3.25, 4240.0, 25639.0, 2.0, 0.0, 3.0, 3.0, 10.0, 3550.0, 690.0, 47.3241, -122.378, 3590.0, 24967.0, 25.0, 0.0, 0.0] | [1156651.3] | 1 | True |
624 | 2024-02-09 16:54:02.507 | [4.0, 3.5, 3440.0, 9776.0, 2.0, 0.0, 0.0, 3.0, 10.0, 3440.0, 0.0, 47.5374, -122.216, 2400.0, 11000.0, 9.0, 0.0, 0.0] | [1124493.3] | 1 | True |
634 | 2024-02-09 16:54:02.507 | [4.0, 3.25, 4700.0, 38412.0, 2.0, 0.0, 0.0, 3.0, 10.0, 3420.0, 1280.0, 47.6445, -122.167, 3640.0, 35571.0, 36.0, 0.0, 0.0] | [1164589.4] | 1 | True |
651 | 2024-02-09 16:54:02.507 | [3.0, 3.0, 3920.0, 13085.0, 2.0, 1.0, 4.0, 4.0, 11.0, 3920.0, 0.0, 47.5716, -122.204, 3450.0, 13287.0, 18.0, 0.0, 0.0] | [1452224.5] | 1 | True |
658 | 2024-02-09 16:54:02.507 | [3.0, 3.25, 3230.0, 7800.0, 2.0, 0.0, 3.0, 3.0, 10.0, 3230.0, 0.0, 47.6348, -122.403, 3030.0, 6600.0, 9.0, 0.0, 0.0] | [1077279.3] | 1 | True |
671 | 2024-02-09 16:54:02.507 | [3.0, 3.5, 3080.0, 6495.0, 2.0, 0.0, 3.0, 3.0, 11.0, 2530.0, 550.0, 47.6321, -122.393, 4120.0, 8620.0, 18.0, 1.0, 10.0] | [1122811.8] | 1 | True |
685 | 2024-02-09 16:54:02.507 | [4.0, 2.5, 4200.0, 35267.0, 2.0, 0.0, 0.0, 3.0, 11.0, 4200.0, 0.0, 47.7108, -122.071, 3540.0, 22234.0, 24.0, 0.0, 0.0] | [1181336.0] | 1 | True |
686 | 2024-02-09 16:54:02.507 | [4.0, 3.25, 4160.0, 47480.0, 2.0, 0.0, 0.0, 3.0, 10.0, 4160.0, 0.0, 47.7266, -122.115, 3400.0, 40428.0, 19.0, 0.0, 0.0] | [1082353.3] | 1 | True |
698 | 2024-02-09 16:54:02.507 | [4.0, 4.5, 5770.0, 10050.0, 1.0, 0.0, 3.0, 5.0, 9.0, 3160.0, 2610.0, 47.677, -122.275, 2950.0, 6700.0, 65.0, 0.0, 0.0] | [1689843.3] | 1 | True |
711 | 2024-02-09 16:54:02.507 | [3.0, 2.5, 5403.0, 24069.0, 2.0, 1.0, 4.0, 4.0, 12.0, 5403.0, 0.0, 47.4169, -122.348, 3980.0, 104374.0, 39.0, 0.0, 0.0] | [1946437.3] | 1 | True |
720 | 2024-02-09 16:54:02.507 | [5.0, 3.0, 3420.0, 18129.0, 2.0, 0.0, 0.0, 3.0, 9.0, 2540.0, 880.0, 47.5333, -122.217, 3750.0, 16316.0, 62.0, 1.0, 53.0] | [1325961.0] | 1 | True |
722 | 2024-02-09 16:54:02.507 | [3.0, 3.25, 4560.0, 13363.0, 1.0, 0.0, 4.0, 3.0, 11.0, 2760.0, 1800.0, 47.6205, -122.214, 4060.0, 13362.0, 20.0, 0.0, 0.0] | [2005883.1] | 1 | True |
726 | 2024-02-09 16:54:02.507 | [5.0, 3.5, 4200.0, 5400.0, 2.0, 0.0, 0.0, 3.0, 9.0, 3140.0, 1060.0, 47.7077, -122.12, 3300.0, 5564.0, 2.0, 0.0, 0.0] | [1052898.0] | 1 | True |
737 | 2024-02-09 16:54:02.507 | [4.0, 3.25, 2980.0, 7000.0, 2.0, 0.0, 3.0, 3.0, 10.0, 2140.0, 840.0, 47.5933, -122.292, 2200.0, 4800.0, 114.0, 1.0, 114.0] | [1156206.5] | 1 | True |
740 | 2024-02-09 16:54:02.507 | [4.0, 4.5, 6380.0, 88714.0, 2.0, 0.0, 0.0, 3.0, 12.0, 6380.0, 0.0, 47.5592, -122.015, 3040.0, 7113.0, 8.0, 0.0, 0.0] | [1355747.1] | 1 | True |
782 | 2024-02-09 16:54:02.507 | [5.0, 4.25, 4860.0, 9453.0, 1.5, 0.0, 1.0, 5.0, 10.0, 3100.0, 1760.0, 47.6196, -122.286, 3150.0, 8557.0, 109.0, 0.0, 0.0] | [1910823.8] | 1 | True |
798 | 2024-02-09 16:54:02.507 | [4.0, 2.5, 2790.0, 5450.0, 2.0, 0.0, 0.0, 3.0, 10.0, 1930.0, 860.0, 47.6453, -122.303, 2320.0, 5450.0, 89.0, 1.0, 75.0] | [1097757.4] | 1 | True |
818 | 2024-02-09 16:54:02.507 | [4.0, 4.0, 4620.0, 130208.0, 2.0, 0.0, 0.0, 3.0, 10.0, 4620.0, 0.0, 47.5885, -121.939, 4620.0, 131007.0, 1.0, 0.0, 0.0] | [1164589.4] | 1 | True |
827 | 2024-02-09 16:54:02.507 | [4.0, 2.5, 3340.0, 10422.0, 2.0, 0.0, 0.0, 3.0, 10.0, 3340.0, 0.0, 47.6515, -122.197, 1770.0, 9490.0, 18.0, 0.0, 0.0] | [1103101.4] | 1 | True |
828 | 2024-02-09 16:54:02.507 | [5.0, 3.5, 3760.0, 10207.0, 2.0, 0.0, 0.0, 3.0, 10.0, 3150.0, 610.0, 47.5605, -122.225, 3550.0, 12118.0, 46.0, 0.0, 0.0] | [1489624.5] | 1 | True |
901 | 2024-02-09 16:54:02.507 | [4.0, 2.25, 4470.0, 60373.0, 2.0, 0.0, 0.0, 3.0, 11.0, 4470.0, 0.0, 47.7289, -122.127, 3210.0, 40450.0, 26.0, 0.0, 0.0] | [1208638.0] | 1 | True |
912 | 2024-02-09 16:54:02.507 | [3.0, 2.25, 2960.0, 8330.0, 1.0, 0.0, 3.0, 4.0, 10.0, 2260.0, 700.0, 47.7035, -122.385, 2960.0, 8840.0, 62.0, 0.0, 0.0] | [1178314.0] | 1 | True |
919 | 2024-02-09 16:54:02.507 | [4.0, 3.25, 5180.0, 19850.0, 2.0, 0.0, 3.0, 3.0, 12.0, 3540.0, 1640.0, 47.562, -122.162, 3160.0, 9750.0, 9.0, 0.0, 0.0] | [1295531.3] | 1 | True |
941 | 2024-02-09 16:54:02.507 | [4.0, 3.75, 3770.0, 4000.0, 2.5, 0.0, 0.0, 5.0, 9.0, 2890.0, 880.0, 47.6157, -122.287, 2800.0, 5000.0, 98.0, 0.0, 0.0] | [1182821.0] | 1 | True |
965 | 2024-02-09 16:54:02.507 | [6.0, 4.0, 5310.0, 12741.0, 2.0, 0.0, 2.0, 3.0, 10.0, 3600.0, 1710.0, 47.5696, -122.213, 4190.0, 12632.0, 48.0, 0.0, 0.0] | [2016006.0] | 1 | True |
973 | 2024-02-09 16:54:02.507 | [5.0, 2.0, 3540.0, 9970.0, 2.0, 0.0, 3.0, 3.0, 9.0, 3540.0, 0.0, 47.7108, -122.277, 2280.0, 7195.0, 44.0, 0.0, 0.0] | [1085835.8] | 1 | True |
997 | 2024-02-09 16:54:02.507 | [4.0, 3.25, 2910.0, 1880.0, 2.0, 0.0, 3.0, 5.0, 9.0, 1830.0, 1080.0, 47.616, -122.282, 3100.0, 8200.0, 100.0, 0.0, 0.0] | [1060847.5] | 1 | True |
### Anomaly Detection Logs
Pipeline logs retrieves with `wallaroo.pipeline.logs` include the `anomaly` dataset.
logs = mainpipeline.logs(limit=1000)
display(logs)
display(logs.loc[logs['anomaly.too_high'] == True])
Warning: There are more logs available. Please set a larger limit or request a file using export_logs.
time | in.tensor | out.variable | anomaly.count | anomaly.too_high | |
---|---|---|---|---|---|
0 | 2024-02-09 16:49:26.521 | [3.0, 2.0, 2005.0, 7000.0, 1.0, 0.0, 0.0, 3.0, 7.0, 1605.0, 400.0, 47.6039, -122.298, 1750.0, 4500.0, 34.0, 0.0, 0.0] | [581003.0] | 0 | False |
1 | 2024-02-09 16:49:26.521 | [3.0, 1.75, 2910.0, 37461.0, 1.0, 0.0, 0.0, 4.0, 7.0, 1530.0, 1380.0, 47.7015, -122.164, 2520.0, 18295.0, 47.0, 0.0, 0.0] | [706823.56] | 0 | False |
2 | 2024-02-09 16:49:26.521 | [4.0, 3.25, 2910.0, 1880.0, 2.0, 0.0, 3.0, 5.0, 9.0, 1830.0, 1080.0, 47.616, -122.282, 3100.0, 8200.0, 100.0, 0.0, 0.0] | [1060847.5] | 1 | True |
3 | 2024-02-09 16:49:26.521 | [4.0, 1.75, 2700.0, 7875.0, 1.5, 0.0, 0.0, 4.0, 8.0, 2700.0, 0.0, 47.454, -122.144, 2220.0, 7875.0, 46.0, 0.0, 0.0] | [441960.38] | 0 | False |
4 | 2024-02-09 16:49:26.521 | [3.0, 2.5, 2900.0, 23550.0, 1.0, 0.0, 0.0, 3.0, 10.0, 1490.0, 1410.0, 47.5708, -122.153, 2900.0, 19604.0, 27.0, 0.0, 0.0] | [827411.0] | 0 | False |
... | ... | ... | ... | ... | ... |
995 | 2024-02-09 16:49:26.521 | [3.0, 1.75, 2200.0, 11520.0, 1.0, 0.0, 0.0, 4.0, 7.0, 2200.0, 0.0, 47.7659, -122.341, 1690.0, 8038.0, 62.0, 0.0, 0.0] | [513264.7] | 0 | False |
996 | 2024-02-09 16:49:26.521 | [4.0, 2.5, 2500.0, 8540.0, 2.0, 0.0, 0.0, 3.0, 9.0, 2500.0, 0.0, 47.5759, -121.994, 2560.0, 8475.0, 24.0, 0.0, 0.0] | [758714.2] | 0 | False |
997 | 2024-02-09 16:49:26.521 | [3.0, 2.5, 1300.0, 812.0, 2.0, 0.0, 0.0, 3.0, 8.0, 880.0, 420.0, 47.5893, -122.317, 1300.0, 824.0, 6.0, 0.0, 0.0] | [448627.72] | 0 | False |
998 | 2024-02-09 16:49:26.521 | [2.0, 2.5, 2170.0, 6361.0, 1.0, 0.0, 2.0, 3.0, 8.0, 2170.0, 0.0, 47.7109, -122.017, 2310.0, 7419.0, 6.0, 0.0, 0.0] | [615094.56] | 0 | False |
999 | 2024-02-09 16:49:26.521 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.75] | 0 | False |
1000 rows × 5 columns
time | in.tensor | out.variable | anomaly.count | anomaly.too_high | |
---|---|---|---|---|---|
2 | 2024-02-09 16:49:26.521 | [4.0, 3.25, 2910.0, 1880.0, 2.0, 0.0, 3.0, 5.0, 9.0, 1830.0, 1080.0, 47.616, -122.282, 3100.0, 8200.0, 100.0, 0.0, 0.0] | [1060847.5] | 1 | True |
26 | 2024-02-09 16:49:26.521 | [5.0, 2.0, 3540.0, 9970.0, 2.0, 0.0, 3.0, 3.0, 9.0, 3540.0, 0.0, 47.7108, -122.277, 2280.0, 7195.0, 44.0, 0.0, 0.0] | [1085835.8] | 1 | True |
34 | 2024-02-09 16:49:26.521 | [6.0, 4.0, 5310.0, 12741.0, 2.0, 0.0, 2.0, 3.0, 10.0, 3600.0, 1710.0, 47.5696, -122.213, 4190.0, 12632.0, 48.0, 0.0, 0.0] | [2016006.0] | 1 | True |
58 | 2024-02-09 16:49:26.521 | [4.0, 3.75, 3770.0, 4000.0, 2.5, 0.0, 0.0, 5.0, 9.0, 2890.0, 880.0, 47.6157, -122.287, 2800.0, 5000.0, 98.0, 0.0, 0.0] | [1182821.0] | 1 | True |
80 | 2024-02-09 16:49:26.521 | [4.0, 3.25, 5180.0, 19850.0, 2.0, 0.0, 3.0, 3.0, 12.0, 3540.0, 1640.0, 47.562, -122.162, 3160.0, 9750.0, 9.0, 0.0, 0.0] | [1295531.2] | 1 | True |
87 | 2024-02-09 16:49:26.521 | [3.0, 2.25, 2960.0, 8330.0, 1.0, 0.0, 3.0, 4.0, 10.0, 2260.0, 700.0, 47.7035, -122.385, 2960.0, 8840.0, 62.0, 0.0, 0.0] | [1178314.0] | 1 | True |
98 | 2024-02-09 16:49:26.521 | [4.0, 2.25, 4470.0, 60373.0, 2.0, 0.0, 0.0, 3.0, 11.0, 4470.0, 0.0, 47.7289, -122.127, 3210.0, 40450.0, 26.0, 0.0, 0.0] | [1208638.0] | 1 | True |
171 | 2024-02-09 16:49:26.521 | [5.0, 3.5, 3760.0, 10207.0, 2.0, 0.0, 0.0, 3.0, 10.0, 3150.0, 610.0, 47.5605, -122.225, 3550.0, 12118.0, 46.0, 0.0, 0.0] | [1489624.5] | 1 | True |
172 | 2024-02-09 16:49:26.521 | [4.0, 2.5, 3340.0, 10422.0, 2.0, 0.0, 0.0, 3.0, 10.0, 3340.0, 0.0, 47.6515, -122.197, 1770.0, 9490.0, 18.0, 0.0, 0.0] | [1103101.4] | 1 | True |
181 | 2024-02-09 16:49:26.521 | [4.0, 4.0, 4620.0, 130208.0, 2.0, 0.0, 0.0, 3.0, 10.0, 4620.0, 0.0, 47.5885, -121.939, 4620.0, 131007.0, 1.0, 0.0, 0.0] | [1164589.4] | 1 | True |
201 | 2024-02-09 16:49:26.521 | [4.0, 2.5, 2790.0, 5450.0, 2.0, 0.0, 0.0, 3.0, 10.0, 1930.0, 860.0, 47.6453, -122.303, 2320.0, 5450.0, 89.0, 1.0, 75.0] | [1097757.4] | 1 | True |
217 | 2024-02-09 16:49:26.521 | [5.0, 4.25, 4860.0, 9453.0, 1.5, 0.0, 1.0, 5.0, 10.0, 3100.0, 1760.0, 47.6196, -122.286, 3150.0, 8557.0, 109.0, 0.0, 0.0] | [1910823.8] | 1 | True |
259 | 2024-02-09 16:49:26.521 | [4.0, 4.5, 6380.0, 88714.0, 2.0, 0.0, 0.0, 3.0, 12.0, 6380.0, 0.0, 47.5592, -122.015, 3040.0, 7113.0, 8.0, 0.0, 0.0] | [1355747.1] | 1 | True |
262 | 2024-02-09 16:49:26.521 | [4.0, 3.25, 2980.0, 7000.0, 2.0, 0.0, 3.0, 3.0, 10.0, 2140.0, 840.0, 47.5933, -122.292, 2200.0, 4800.0, 114.0, 1.0, 114.0] | [1156206.5] | 1 | True |
273 | 2024-02-09 16:49:26.521 | [5.0, 3.5, 4200.0, 5400.0, 2.0, 0.0, 0.0, 3.0, 9.0, 3140.0, 1060.0, 47.7077, -122.12, 3300.0, 5564.0, 2.0, 0.0, 0.0] | [1052898.0] | 1 | True |
277 | 2024-02-09 16:49:26.521 | [3.0, 3.25, 4560.0, 13363.0, 1.0, 0.0, 4.0, 3.0, 11.0, 2760.0, 1800.0, 47.6205, -122.214, 4060.0, 13362.0, 20.0, 0.0, 0.0] | [2005883.1] | 1 | True |
279 | 2024-02-09 16:49:26.521 | [5.0, 3.0, 3420.0, 18129.0, 2.0, 0.0, 0.0, 3.0, 9.0, 2540.0, 880.0, 47.5333, -122.217, 3750.0, 16316.0, 62.0, 1.0, 53.0] | [1325961.0] | 1 | True |
288 | 2024-02-09 16:49:26.521 | [3.0, 2.5, 5403.0, 24069.0, 2.0, 1.0, 4.0, 4.0, 12.0, 5403.0, 0.0, 47.4169, -122.348, 3980.0, 104374.0, 39.0, 0.0, 0.0] | [1946437.2] | 1 | True |
301 | 2024-02-09 16:49:26.521 | [4.0, 4.5, 5770.0, 10050.0, 1.0, 0.0, 3.0, 5.0, 9.0, 3160.0, 2610.0, 47.677, -122.275, 2950.0, 6700.0, 65.0, 0.0, 0.0] | [1689843.2] | 1 | True |
313 | 2024-02-09 16:49:26.521 | [4.0, 3.25, 4160.0, 47480.0, 2.0, 0.0, 0.0, 3.0, 10.0, 4160.0, 0.0, 47.7266, -122.115, 3400.0, 40428.0, 19.0, 0.0, 0.0] | [1082353.2] | 1 | True |
314 | 2024-02-09 16:49:26.521 | [4.0, 2.5, 4200.0, 35267.0, 2.0, 0.0, 0.0, 3.0, 11.0, 4200.0, 0.0, 47.7108, -122.071, 3540.0, 22234.0, 24.0, 0.0, 0.0] | [1181336.0] | 1 | True |
328 | 2024-02-09 16:49:26.521 | [3.0, 3.5, 3080.0, 6495.0, 2.0, 0.0, 3.0, 3.0, 11.0, 2530.0, 550.0, 47.6321, -122.393, 4120.0, 8620.0, 18.0, 1.0, 10.0] | [1122811.8] | 1 | True |
341 | 2024-02-09 16:49:26.521 | [3.0, 3.25, 3230.0, 7800.0, 2.0, 0.0, 3.0, 3.0, 10.0, 3230.0, 0.0, 47.6348, -122.403, 3030.0, 6600.0, 9.0, 0.0, 0.0] | [1077279.2] | 1 | True |
348 | 2024-02-09 16:49:26.521 | [3.0, 3.0, 3920.0, 13085.0, 2.0, 1.0, 4.0, 4.0, 11.0, 3920.0, 0.0, 47.5716, -122.204, 3450.0, 13287.0, 18.0, 0.0, 0.0] | [1452224.5] | 1 | True |
365 | 2024-02-09 16:49:26.521 | [4.0, 3.25, 4700.0, 38412.0, 2.0, 0.0, 0.0, 3.0, 10.0, 3420.0, 1280.0, 47.6445, -122.167, 3640.0, 35571.0, 36.0, 0.0, 0.0] | [1164589.4] | 1 | True |
375 | 2024-02-09 16:49:26.521 | [4.0, 3.5, 3440.0, 9776.0, 2.0, 0.0, 0.0, 3.0, 10.0, 3440.0, 0.0, 47.5374, -122.216, 2400.0, 11000.0, 9.0, 0.0, 0.0] | [1124493.2] | 1 | True |
376 | 2024-02-09 16:49:26.521 | [4.0, 3.25, 4240.0, 25639.0, 2.0, 0.0, 3.0, 3.0, 10.0, 3550.0, 690.0, 47.3241, -122.378, 3590.0, 24967.0, 25.0, 0.0, 0.0] | [1156651.2] | 1 | True |
443 | 2024-02-09 16:49:26.521 | [4.0, 3.5, 4285.0, 9567.0, 2.0, 0.0, 1.0, 5.0, 10.0, 3485.0, 800.0, 47.6434, -122.409, 2960.0, 6902.0, 68.0, 0.0, 0.0] | [1886959.4] | 1 | True |
464 | 2024-02-09 16:49:26.521 | [4.0, 3.5, 4460.0, 16271.0, 2.0, 0.0, 2.0, 3.0, 11.0, 4460.0, 0.0, 47.5862, -121.97, 4540.0, 17122.0, 13.0, 0.0, 0.0] | [1208638.0] | 1 | True |
469 | 2024-02-09 16:49:26.521 | [4.0, 4.25, 3500.0, 8750.0, 1.0, 0.0, 4.0, 5.0, 9.0, 2140.0, 1360.0, 47.7222, -122.367, 3110.0, 8750.0, 63.0, 0.0, 0.0] | [1140733.8] | 1 | True |
479 | 2024-02-09 16:49:26.521 | [5.0, 3.75, 4170.0, 8142.0, 2.0, 0.0, 2.0, 3.0, 10.0, 4170.0, 0.0, 47.5354, -122.181, 3030.0, 7980.0, 9.0, 0.0, 0.0] | [1098628.8] | 1 | True |
486 | 2024-02-09 16:49:26.521 | [4.0, 3.25, 3320.0, 8587.0, 3.0, 0.0, 0.0, 3.0, 11.0, 2950.0, 370.0, 47.691, -122.337, 1860.0, 5668.0, 6.0, 0.0, 0.0] | [1130661.0] | 1 | True |
502 | 2024-02-09 16:49:26.521 | [4.0, 2.5, 4090.0, 11225.0, 2.0, 0.0, 0.0, 3.0, 10.0, 4090.0, 0.0, 47.581, -121.971, 3510.0, 8762.0, 9.0, 0.0, 0.0] | [1048372.4] | 1 | True |
556 | 2024-02-09 16:49:26.521 | [5.0, 4.0, 4360.0, 8030.0, 2.0, 0.0, 0.0, 3.0, 10.0, 4360.0, 0.0, 47.5923, -121.973, 3570.0, 6185.0, 0.0, 0.0, 0.0] | [1160512.8] | 1 | True |
585 | 2024-02-09 16:49:26.521 | [5.0, 3.5, 5430.0, 10327.0, 2.0, 0.0, 2.0, 3.0, 10.0, 4010.0, 1420.0, 47.5476, -122.116, 4340.0, 10324.0, 7.0, 0.0, 0.0] | [1207858.6] | 1 | True |
601 | 2024-02-09 16:49:26.521 | [3.0, 2.25, 2390.0, 7875.0, 1.0, 0.0, 1.0, 3.0, 10.0, 1980.0, 410.0, 47.6515, -122.278, 3720.0, 9075.0, 66.0, 0.0, 0.0] | [1364149.9] | 1 | True |
639 | 2024-02-09 16:49:26.521 | [4.0, 3.5, 3770.0, 8501.0, 2.0, 0.0, 0.0, 3.0, 10.0, 3770.0, 0.0, 47.6744, -122.196, 1520.0, 9660.0, 6.0, 0.0, 0.0] | [1169643.0] | 1 | True |
648 | 2024-02-09 16:49:26.521 | [5.0, 4.0, 4660.0, 9900.0, 2.0, 0.0, 2.0, 4.0, 9.0, 2600.0, 2060.0, 47.5135, -122.2, 3380.0, 9900.0, 35.0, 0.0, 0.0] | [1058105.0] | 1 | True |
676 | 2024-02-09 16:49:26.521 | [3.0, 3.0, 2480.0, 5500.0, 2.0, 0.0, 3.0, 3.0, 10.0, 1730.0, 750.0, 47.6466, -122.404, 2950.0, 5670.0, 64.0, 1.0, 55.0] | [1100884.1] | 1 | True |
714 | 2024-02-09 16:49:26.521 | [4.0, 2.75, 4020.0, 18745.0, 2.0, 0.0, 4.0, 4.0, 10.0, 2830.0, 1190.0, 47.6042, -122.21, 3150.0, 20897.0, 26.0, 0.0, 0.0] | [1322835.9] | 1 | True |
716 | 2024-02-09 16:49:26.521 | [4.0, 2.75, 3260.0, 19542.0, 1.0, 0.0, 0.0, 4.0, 10.0, 2170.0, 1090.0, 47.6245, -122.236, 3480.0, 19863.0, 46.0, 0.0, 0.0] | [1364650.2] | 1 | True |
717 | 2024-02-09 16:49:26.521 | [3.0, 2.75, 3170.0, 34850.0, 1.0, 0.0, 0.0, 5.0, 9.0, 3170.0, 0.0, 47.6611, -122.169, 3920.0, 36740.0, 58.0, 0.0, 0.0] | [1227073.8] | 1 | True |
718 | 2024-02-09 16:49:26.521 | [3.0, 3.0, 3570.0, 6250.0, 2.0, 0.0, 2.0, 3.0, 10.0, 2710.0, 860.0, 47.5624, -122.399, 2550.0, 7596.0, 30.0, 0.0, 0.0] | [1124493.2] | 1 | True |
728 | 2024-02-09 16:49:26.521 | [5.0, 3.25, 5790.0, 13726.0, 2.0, 0.0, 3.0, 3.0, 10.0, 4430.0, 1360.0, 47.5388, -122.114, 5790.0, 13726.0, 0.0, 0.0, 0.0] | [1189654.4] | 1 | True |
744 | 2024-02-09 16:49:26.521 | [4.0, 3.0, 4750.0, 21701.0, 1.5, 0.0, 0.0, 5.0, 11.0, 4750.0, 0.0, 47.6454, -122.218, 3120.0, 18551.0, 38.0, 0.0, 0.0] | [2002393.5] | 1 | True |
751 | 2024-02-09 16:49:26.521 | [4.0, 3.75, 4410.0, 8112.0, 3.0, 0.0, 4.0, 3.0, 11.0, 3570.0, 840.0, 47.5888, -122.392, 2770.0, 5750.0, 12.0, 0.0, 0.0] | [1967344.1] | 1 | True |
760 | 2024-02-09 16:49:26.521 | [4.0, 3.25, 5010.0, 49222.0, 2.0, 0.0, 0.0, 5.0, 9.0, 3710.0, 1300.0, 47.5489, -122.092, 3140.0, 54014.0, 36.0, 0.0, 0.0] | [1092274.1] | 1 | True |
789 | 2024-02-09 16:49:26.521 | [4.0, 3.5, 4300.0, 70407.0, 2.0, 0.0, 0.0, 3.0, 10.0, 2710.0, 1590.0, 47.4472, -122.092, 3520.0, 26727.0, 22.0, 0.0, 0.0] | [1115275.0] | 1 | True |
839 | 2024-02-09 16:49:26.521 | [5.0, 3.5, 4150.0, 13232.0, 2.0, 0.0, 0.0, 3.0, 11.0, 4150.0, 0.0, 47.3417, -122.182, 3840.0, 15121.0, 9.0, 0.0, 0.0] | [1042119.1] | 1 | True |
845 | 2024-02-09 16:49:26.521 | [4.0, 2.75, 3800.0, 9606.0, 2.0, 0.0, 0.0, 3.0, 9.0, 3800.0, 0.0, 47.7368, -122.208, 3400.0, 9677.0, 6.0, 0.0, 0.0] | [1039781.25] | 1 | True |
866 | 2024-02-09 16:49:26.521 | [5.0, 2.25, 3320.0, 13138.0, 1.0, 0.0, 2.0, 4.0, 9.0, 1900.0, 1420.0, 47.759, -122.269, 2820.0, 13138.0, 51.0, 0.0, 0.0] | [1108000.1] | 1 | True |
869 | 2024-02-09 16:49:26.521 | [4.0, 2.75, 2620.0, 13777.0, 1.5, 0.0, 2.0, 4.0, 9.0, 1720.0, 900.0, 47.58, -122.285, 3530.0, 9287.0, 88.0, 0.0, 0.0] | [1223839.1] | 1 | True |
889 | 2024-02-09 16:49:26.521 | [4.0, 2.5, 3470.0, 20445.0, 2.0, 0.0, 0.0, 4.0, 10.0, 3470.0, 0.0, 47.547, -122.219, 3360.0, 21950.0, 51.0, 0.0, 0.0] | [1412215.2] | 1 | True |
936 | 2024-02-09 16:49:26.521 | [4.0, 3.0, 4040.0, 19700.0, 2.0, 0.0, 0.0, 3.0, 11.0, 4040.0, 0.0, 47.7205, -122.127, 3930.0, 21887.0, 27.0, 0.0, 0.0] | [1028923.06] | 1 | True |
959 | 2024-02-09 16:49:26.521 | [4.0, 4.5, 5120.0, 41327.0, 2.0, 0.0, 0.0, 3.0, 10.0, 3290.0, 1830.0, 47.7009, -122.059, 3360.0, 82764.0, 6.0, 0.0, 0.0] | [1204324.8] | 1 | True |
969 | 2024-02-09 16:49:26.521 | [4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0, 10.0, 2760.0, 950.0, 47.6696, -122.261, 3970.0, 20000.0, 79.0, 0.0, 0.0] | [1514079.8] | 1 | True |
993 | 2024-02-09 16:49:26.521 | [4.0, 3.5, 3590.0, 5334.0, 2.0, 0.0, 2.0, 3.0, 9.0, 3140.0, 450.0, 47.6763, -122.267, 2100.0, 6250.0, 9.0, 0.0, 0.0] | [1004846.5] | 1 | True |
Undeploy Main Pipeline
With the examples and tutorial complete, we will undeploy the main pipeline and return the resources back to the Wallaroo instance.
mainpipeline.undeploy()
Waiting for undeployment - this will take up to 45s ..................................... ok
name | logpipeline-test |
---|---|
created | 2024-02-09 16:21:09.406182+00:00 |
last_updated | 2024-02-09 16:53:37.061953+00:00 |
deployed | False |
arch | None |
tags | |
versions | 764c7706-c996-42e9-90ff-87b1b496f98d, 05c46dbc-9d72-40d5-bc4c-7fee7bc3e971, 9a4d76f5-9905-4063-8bf8-47e103987515, d5e4882a-3c17-4965-b059-66432a50a3cd, 00b3d5e7-4644-4138-b73d-b0511b3c9e2a, e143a2d5-5641-4dcc-8ae4-786fd777a30a, e2b9d903-4015-4d09-902b-9150a7196cea, 9df38be1-d2f4-4be1-9022-8f0570a238b9, 3078b49f-3eff-48d1-8d9b-a8780b329ecc, 21bff9df-828f-40e7-8a22-449a2e636b44, f78a7030-bd25-4bf7-ba0d-a18cfe3790e0, 10c1ac25-d626-4413-8d5d-1bed42d0e65c, b179b693-b6b6-4ff9-b2a4-2a639d88bc9b, da7b9cf0-81e8-452b-8b70-689406dc9548, a9a9b62c-9d37-427f-99af-67725558bf9b, 1c14591a-96b4-4059-bb63-2d2bc4e308d5, add660ac-0ebf-4a24-bb6d-6cdc875866c8 |
steps | logcontrol |
published | False |
10 - Wallaroo Edge Observability with Wallaroo Assays
This tutorial and the assets can be downloaded as part of the Wallaroo Tutorials repository.
Wallaroo Edge Observability with Assays Tutorial
This notebook is designed to demonstrate the Wallaroo Edge Observability with Wallaroo Assays. This notebook will walk through the process of:
- In Wallaroo Ops:
- Setting up a workspace, pipeline, and model for deriving the price of a house based on inputs.
- Creating an assay from a sample of inferences.
- Display the inference result and upload the assay to the Wallaroo instance where it can be referenced later.
- In a remote aka edge location:
- Deploying the Wallaroo pipeline as a Wallaroo Inference Server deployed on an edge device with observability features.
- In Wallaroo Ops:
- Updating the assay to use the edge location’s inferences for assays.
This tutorial provides the following:
- Models:
models/rf_model.onnx
: The champion model that has been used in this environment for some time.models/xgb_model.onnx
andmodels/gbr_model.onnx
: Rival models that will be tested against the champion.
- Data:
data/xtest-1.df.json
anddata/xtest-1k.df.json
: DataFrame JSON inference inputs with 1 input and 1,000 inputs.data/xtest-1k.arrow
: Apache Arrow inference inputs with 1 input and 1,000 inputs.
Prerequisites
- A deployed Wallaroo Ops instance.
- A location with Docker or Kubernetes with
helm
for Wallaroo Inference server deployments. - The following Python libraries installed:
References
# used for unique workspace names
import string
import random
suffix= ''.join(random.choice(string.ascii_lowercase) for i in range(4))
suffix='jch'
workspace_name = f'edge-observability-{suffix}'
main_pipeline_name = 'houseprice-estimation-edge'
model_name_control = 'housepricesagacontrol'
model_file_name_control = './models/rf_model.onnx'
# Set the name of the assay
edge_name=f"house-price-edge-{suffix}"
def get_workspace(name, client):
workspace = None
for ws in wl.list_workspaces():
if ws.name() == name:
workspace= ws
if(workspace == None):
workspace = wl.create_workspace(name)
return workspace
def get_pipeline(name, workspace):
pipelines = workspace.pipelines()
pipe_filter = filter(lambda x: x.name() == name, pipelines)
pipes = list(pipe_filter)
# we can't have a pipe in the workspace with the same name, so it's always the first
if pipes:
pipeline = pipes[0]
else:
pipeline = wl.build_pipeline(name)
return pipeline
Initial Steps
Import libraries
The first step is to import the libraries needed for this notebook.
import wallaroo
from wallaroo.object import EntityNotFoundError
from wallaroo.framework import Framework
from IPython.display import display
# used to display DataFrame information without truncating
from IPython.display import display
import pandas as pd
pd.set_option('display.max_colwidth', None)
import datetime
import time
Connect to the Wallaroo Instance
The first step is to connect to Wallaroo through the Wallaroo client. The Python library is included in the Wallaroo install and available through the Jupyter Hub interface provided with your Wallaroo environment.
This is accomplished using the wallaroo.Client()
command, which provides a URL to grant the SDK permission to your specific Wallaroo environment. When displayed, enter the URL into a browser and confirm permissions. Store the connection into a variable that can be referenced later.
If logging into the Wallaroo instance through the internal JupyterHub service, use wl = wallaroo.Client()
. For more information on Wallaroo Client settings, see the Client Connection guide.
# Login through local Wallaroo instance
wl = wallaroo.Client()
Create Workspace
We will create a workspace to manage our pipeline and models. The following variables will set the name of our sample workspace then set it as the current workspace.
Workspace, pipeline, and model names should be unique to each user, so we’ll add in a randomly generated suffix so multiple people can run this tutorial in a Wallaroo instance without effecting each other.
workspace = get_workspace(workspace_name, wl)
wl.set_current_workspace(workspace)
{'name': 'edge-observability-jch', 'id': 11, 'archived': False, 'created_by': '93dc3434-cf57-47b4-a3e4-b2168b1fd7e1', 'created_at': '2023-12-12T18:03:20.250521+00:00', 'models': [], 'pipelines': []}
Upload The Champion Model
For our example, we will upload the champion model that has been trained to derive house prices from a variety of inputs. The model file is rf_model.onnx
, and is uploaded with the name housingcontrol
.
housing_model_control = (wl.upload_model(model_name_control,
model_file_name_control,
framework=Framework.ONNX)
.configure(tensor_fields=["tensor"])
)
Standard Pipeline Steps
Build the Pipeline
This pipeline is made to be an example of an existing situation where a model is deployed and being used for inferences in a production environment. We’ll call it housepricepipeline
, set housingcontrol
as a pipeline step, then run a few sample inferences.
This pipeline will be a simple one - just a single pipeline step.
mainpipeline = get_pipeline(main_pipeline_name, workspace)
mainpipeline.clear()
mainpipeline.add_model_step(housing_model_control)
#minimum deployment config
deploy_config = wallaroo.DeploymentConfigBuilder().replica_count(1).cpus(0.5).memory("1Gi").build()
mainpipeline.deploy(deployment_config = deploy_config)
name | houseprice-estimation-edge |
---|---|
created | 2023-12-12 18:03:28.275490+00:00 |
last_updated | 2023-12-12 18:03:29.206241+00:00 |
deployed | True |
arch | None |
tags | |
versions | 66d73202-0c8c-4468-b589-67286a2f5dd6, be11e14c-1565-4d68-8bf5-f9eebd7cd458 |
steps | housepricesagacontrol |
published | False |
Testing
We’ll use two inferences as a quick sample test - one that has a house that should be determined around $700k
, the other with a house determined to be around $1.5
million. We’ll also save the start and end periods for these events to for later log functionality.
normal_input = pd.DataFrame.from_records({"tensor": [[4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0]]})
result = mainpipeline.infer(normal_input)
display(result)
time | in.tensor | out.variable | check_failures | |
---|---|---|---|---|
0 | 2023-12-12 18:03:46.251 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.7] | 0 |
large_house_input = pd.DataFrame.from_records({'tensor': [[4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0, 10.0, 2760.0, 950.0, 47.6696, -122.261, 3970.0, 20000.0, 79.0, 0.0, 0.0]]})
large_house_result = mainpipeline.infer(large_house_input)
display(large_house_result)
time | in.tensor | out.variable | check_failures | |
---|---|---|---|---|
0 | 2023-12-12 18:03:46.644 | [4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0, 10.0, 2760.0, 950.0, 47.6696, -122.261, 3970.0, 20000.0, 79.0, 0.0, 0.0] | [1514079.4] | 0 |
Assays
Wallaroo assays provide a method for detecting input or model drift. These can be triggered either when unexpected input is provided for the inference, or when the model needs to be retrained from changing environment conditions.
Wallaroo assays can track either an input field and its index, or an output field and its index. For full details, see the Wallaroo Assays Management Guide.
For this example, we will:
- Perform sample inferences based on lower priced houses.
- Create an assay with the baseline set off those lower priced houses.
- Generate inferences spread across all house values, plus specific set of high priced houses to trigger the assay alert.
- Run an interactive assay to show the detection of values outside the established baseline.
Assay Generation
To start the demonstration, we’ll create a baseline of values from houses with small estimated prices and set that as our baseline. Assays are typically run on a 24 hours interval based on a 24 hour window of data, but we’ll bypass that by setting our baseline time even shorter.
small_houses_inputs = pd.read_json('./data/smallinputs.df.json')
baseline_size = 500
# Where the baseline data will start
assay_baseline_start = datetime.datetime.now()
# These inputs will be random samples of small priced houses. Around 30,000 is a good number
small_houses = small_houses_inputs.sample(baseline_size, replace=True).reset_index(drop=True)
# Wait 30 seconds to set this data apart from the rest
time.sleep(30)
small_results = mainpipeline.infer(small_houses)
# Set the baseline end
assay_baseline_end = datetime.datetime.now()
display(small_results)
time | in.tensor | out.variable | check_failures | |
---|---|---|---|---|
0 | 2023-12-12 18:04:57.444 | [2.0, 1.0, 830.0, 26329.0, 1.0, 1.0, 3.0, 4.0, 6.0, 830.0, 0.0, 47.4011993408, -122.4250030518, 2030.0, 27338.0, 86.0, 0.0, 0.0] | [379398.28] | 0 |
1 | 2023-12-12 18:04:57.444 | [5.0, 1.75, 2660.0, 10637.0, 1.5, 0.0, 0.0, 5.0, 7.0, 1670.0, 990.0, 47.6944999695, -122.2919998169, 1570.0, 6825.0, 92.0, 0.0, 0.0] | [716776.56] | 0 |
2 | 2023-12-12 18:04:57.444 | [3.0, 1.75, 1630.0, 15600.0, 1.0, 0.0, 0.0, 3.0, 7.0, 1630.0, 0.0, 47.6800003052, -122.1650009155, 1830.0, 10850.0, 56.0, 0.0, 0.0] | [559631.06] | 0 |
3 | 2023-12-12 18:04:57.444 | [3.0, 1.0, 1980.0, 3243.0, 1.5, 0.0, 0.0, 3.0, 8.0, 1980.0, 0.0, 47.6428985596, -122.327003479, 1380.0, 1249.0, 102.0, 0.0, 0.0] | [577149.0] | 0 |
4 | 2023-12-12 18:04:57.444 | [4.0, 2.5, 2230.0, 4372.0, 2.0, 0.0, 0.0, 5.0, 8.0, 1540.0, 690.0, 47.6697998047, -122.3339996338, 2020.0, 4372.0, 79.0, 0.0, 0.0] | [682284.56] | 0 |
... | ... | ... | ... | ... |
495 | 2023-12-12 18:04:57.444 | [3.0, 2.75, 2050.0, 3320.0, 1.5, 0.0, 0.0, 4.0, 7.0, 1580.0, 470.0, 47.6719017029, -122.3010025024, 1760.0, 4150.0, 87.0, 0.0, 0.0] | [630865.5] | 0 |
496 | 2023-12-12 18:04:57.444 | [3.0, 2.5, 1520.0, 4170.0, 2.0, 0.0, 0.0, 3.0, 7.0, 1520.0, 0.0, 47.3842010498, -122.0400009155, 1560.0, 4237.0, 10.0, 0.0, 0.0] | [261886.94] | 0 |
497 | 2023-12-12 18:04:57.444 | [3.0, 1.75, 1460.0, 7800.0, 1.0, 0.0, 0.0, 2.0, 7.0, 1040.0, 420.0, 47.3035011292, -122.3820037842, 1310.0, 7865.0, 36.0, 0.0, 0.0] | [300480.3] | 0 |
498 | 2023-12-12 18:04:57.444 | [4.0, 1.75, 2320.0, 9240.0, 1.0, 0.0, 0.0, 3.0, 7.0, 1160.0, 1160.0, 47.4908981323, -122.2570037842, 2130.0, 7320.0, 55.0, 1.0, 55.0] | [404676.1] | 0 |
499 | 2023-12-12 18:04:57.444 | [5.0, 3.0, 2170.0, 2440.0, 1.5, 0.0, 0.0, 4.0, 8.0, 1450.0, 720.0, 47.6724014282, -122.3170013428, 2070.0, 4000.0, 103.0, 0.0, 0.0] | [675545.44] | 0 |
500 rows × 4 columns
Derive Numpy Values
For our assay, we will create it using the inference results and collect them into one numpy array.
# get the numpy values
# set the results to a non-array value
small_results_baseline_df = small_results.copy()
small_results_baseline_df['variable']=small_results['out.variable'].map(lambda x: x[0])
small_results_baseline_df
time | in.tensor | out.variable | check_failures | variable | |
---|---|---|---|---|---|
0 | 2023-12-12 18:04:57.444 | [2.0, 1.0, 830.0, 26329.0, 1.0, 1.0, 3.0, 4.0, 6.0, 830.0, 0.0, 47.4011993408, -122.4250030518, 2030.0, 27338.0, 86.0, 0.0, 0.0] | [379398.28] | 0 | 379398.28 |
1 | 2023-12-12 18:04:57.444 | [5.0, 1.75, 2660.0, 10637.0, 1.5, 0.0, 0.0, 5.0, 7.0, 1670.0, 990.0, 47.6944999695, -122.2919998169, 1570.0, 6825.0, 92.0, 0.0, 0.0] | [716776.56] | 0 | 716776.56 |
2 | 2023-12-12 18:04:57.444 | [3.0, 1.75, 1630.0, 15600.0, 1.0, 0.0, 0.0, 3.0, 7.0, 1630.0, 0.0, 47.6800003052, -122.1650009155, 1830.0, 10850.0, 56.0, 0.0, 0.0] | [559631.06] | 0 | 559631.06 |
3 | 2023-12-12 18:04:57.444 | [3.0, 1.0, 1980.0, 3243.0, 1.5, 0.0, 0.0, 3.0, 8.0, 1980.0, 0.0, 47.6428985596, -122.327003479, 1380.0, 1249.0, 102.0, 0.0, 0.0] | [577149.0] | 0 | 577149.00 |
4 | 2023-12-12 18:04:57.444 | [4.0, 2.5, 2230.0, 4372.0, 2.0, 0.0, 0.0, 5.0, 8.0, 1540.0, 690.0, 47.6697998047, -122.3339996338, 2020.0, 4372.0, 79.0, 0.0, 0.0] | [682284.56] | 0 | 682284.56 |
... | ... | ... | ... | ... | ... |
495 | 2023-12-12 18:04:57.444 | [3.0, 2.75, 2050.0, 3320.0, 1.5, 0.0, 0.0, 4.0, 7.0, 1580.0, 470.0, 47.6719017029, -122.3010025024, 1760.0, 4150.0, 87.0, 0.0, 0.0] | [630865.5] | 0 | 630865.50 |
496 | 2023-12-12 18:04:57.444 | [3.0, 2.5, 1520.0, 4170.0, 2.0, 0.0, 0.0, 3.0, 7.0, 1520.0, 0.0, 47.3842010498, -122.0400009155, 1560.0, 4237.0, 10.0, 0.0, 0.0] | [261886.94] | 0 | 261886.94 |
497 | 2023-12-12 18:04:57.444 | [3.0, 1.75, 1460.0, 7800.0, 1.0, 0.0, 0.0, 2.0, 7.0, 1040.0, 420.0, 47.3035011292, -122.3820037842, 1310.0, 7865.0, 36.0, 0.0, 0.0] | [300480.3] | 0 | 300480.30 |
498 | 2023-12-12 18:04:57.444 | [4.0, 1.75, 2320.0, 9240.0, 1.0, 0.0, 0.0, 3.0, 7.0, 1160.0, 1160.0, 47.4908981323, -122.2570037842, 2130.0, 7320.0, 55.0, 1.0, 55.0] | [404676.1] | 0 | 404676.10 |
499 | 2023-12-12 18:04:57.444 | [5.0, 3.0, 2170.0, 2440.0, 1.5, 0.0, 0.0, 4.0, 8.0, 1450.0, 720.0, 47.6724014282, -122.3170013428, 2070.0, 4000.0, 103.0, 0.0, 0.0] | [675545.44] | 0 | 675545.44 |
500 rows × 5 columns
# get the numpy values
small_results_baseline = small_results_baseline_df['variable'].to_numpy()
small_results_baseline
array([ 379398.28, 716776.56, 559631.06, 577149. , 682284.56,
950176.7 , 675545.44, 559631.06, 256630.36, 340764.53,
559631.06, 246088.6 , 241852.33, 1322835.6 , 317132.63,
435628.72, 519346.94, 252192.9 , 536371.2 , 559631.06,
575724.7 , 559452.94, 725572.56, 252192.9 , 274207.16,
703282.7 , 758714.3 , 827411.25, 736751.3 , 657905.75,
548006.06, 557391.25, 448180.78, 451058.3 , 381737.6 ,
502022.94, 411000.13, 488736.22, 716558.56, 430252.3 ,
450867.7 , 416774.6 , 559631.06, 682181.9 , 879092.9 ,
555231.94, 391459.97, 244380.27, 236238.67, 328513.6 ,
252539.6 , 879092.9 , 358668.2 , 291903.97, 365436.22,
320863.72, 713485.7 , 284336.47, 448627.8 , 439977.53,
758714.3 , 721518.44, 701940.7 , 879092.9 , 559631.06,
435628.72, 303002.25, 551223.44, 447162.84, 536371.2 ,
244380.27, 873848.44, 725572.56, 557391.25, 725184.1 ,
1077279.1 , 554921.94, 706407.4 , 673288.6 , 448627.8 ,
557391.25, 581002.94, 380009.28, 435628.72, 513264.66,
572709.94, 637377. , 448627.8 , 557391.25, 284336.47,
283759.94, 292859.44, 703282.7 , 258377. , 450867.7 ,
700294.25, 557391.25, 448627.8 , 380009.28, 287576.4 ,
701940.7 , 981676.7 , 557391.25, 341649.34, 450867.7 ,
1100884.3 , 365436.22, 448627.8 , 519346.94, 293808.03,
368504.3 , 437177.97, 423382.72, 448627.8 , 241330.17,
712309.9 , 244380.27, 236238.67, 987157.25, 713979. ,
288798.1 , 904378.7 , 400561.2 , 448627.8 , 904378.7 ,
424966.6 , 758714.3 , 450867.7 , 641162.2 , 473287.25,
557391.25, 278475.66, 559631.06, 736751.3 , 404676.1 ,
313096. , 447951.56, 275408.03, 431992.22, 450996.34,
718445.7 , 296411.7 , 450867.7 , 435628.72, 682284.56,
450867.7 , 1295531.8 , 757403.4 , 559631.06, 435628.72,
363491.63, 713358.8 , 404676.1 , 447162.84, 450867.7 ,
559631.06, 630865.5 , 597475.75, 999203.06, 236238.67,
544392.06, 365436.22, 559631.06, 437177.97, 1052897.9 ,
700294.25, 266405.63, 559452.94, 448627.8 , 268856.88,
448627.8 , 713979. , 435628.72, 391459.97, 276709.06,
765468.9 , 637377. , 846775.06, 536371.2 , 437177.97,
581002.94, 435628.72, 700271.56, 415964.3 , 513083.8 ,
431929.2 , 236238.67, 291857.06, 448627.8 , 457449.06,
442168.13, 236238.67, 711565.44, 291239.75, 725875.94,
831483.56, 448627.8 , 426066.38, 701940.7 , 829775.3 ,
431992.22, 308049.63, 553463.25, 300446.66, 291239.75,
553463.25, 439977.53, 873848.44, 713979. , 448627.8 ,
919031.5 , 1052897.9 , 765468.9 , 431992.22, 437177.97,
536371.2 , 379752.2 , 713485.7 , 446769. , 837085.5 ,
536371.2 , 837085.5 , 552992.06, 450867.7 , 642519.7 ,
381737.6 , 536371.2 , 713485.7 , 442168.13, 303002.25,
363491.63, 263051.63, 437177.97, 448627.8 , 846775.06,
553463.25, 523152.63, 379752.2 , 665791.5 , 475270.97,
758714.3 , 725184.1 , 448627.8 , 437177.97, 236238.67,
383833.88, 559631.06, 244351.92, 713485.7 , 450867.7 ,
416774.6 , 575285.1 , 630865.5 , 701940.7 , 290323.1 ,
284845.34, 554921.94, 581002.94, 519346.94, 340764.53,
338357.88, 340764.53, 421402.1 , 453195.8 , 999203.06,
448627.8 , 236238.67, 274207.16, 266405.63, 536371.2 ,
251194.58, 917346.2 , 349102.75, 947561.9 , 431992.22,
291239.75, 252192.9 , 448627.8 , 385561.78, 1122811.6 ,
317765.63, 725875.94, 784103.56, 422481.5 , 447951.56,
241657.14, 267013.97, 597475.75, 458858.44, 322015.03,
536371.2 , 725572.56, 448627.8 , 538436.75, 538436.75,
349102.75, 288798.1 , 448627.8 , 557391.25, 448627.8 ,
431992.22, 544392.06, 1160512.8 , 416774.6 , 300988.8 ,
310992.94, 555231.94, 421306.6 , 630865.5 , 278475.66,
656923.44, 921695.4 , 442168.13, 680620.7 , 236238.67,
324875.06, 628260.9 , 274207.16, 759983.6 , 252192.9 ,
290323.1 , 424966.6 , 450867.7 , 448627.8 , 450867.7 ,
400561.2 , 557391.25, 921695.4 , 303002.25, 274207.16,
450867.7 , 1100884.3 , 475270.97, 886958.6 , 573403.2 ,
557391.25, 873848.44, 379076.28, 464057.38, 261201.22,
246525.2 , 437177.97, 252539.6 , 1060847.5 , 532234.1 ,
947561.9 , 713485.7 , 424966.6 , 400561.2 , 1295531.8 ,
435628.72, 333878.22, 573403.2 , 517771.6 , 450996.34,
434534.22, 682284.56, 831483.56, 554921.94, 544392.06,
937359.6 , 1077279.1 , 332134.97, 391459.97, 718013.7 ,
448627.8 , 303002.25, 424966.6 , 348616.63, 758714.3 ,
532234.1 , 469038.13, 435628.72, 334257.7 , 309800.8 ,
274207.16, 516278.63, 559631.06, 276709.06, 718445.7 ,
437177.97, 437177.97, 675545.44, 559923.9 , 303002.25,
404551.03, 432908.6 , 687786.44, 292859.44, 411000.13,
252192.9 , 1108000. , 921695.4 , 1124493. , 542342. ,
293808.03, 448627.8 , 453195.8 , 383833.88, 630865.5 ,
380009.28, 340764.53, 758714.3 , 450867.7 , 435628.72,
276709.06, 421402.1 , 340764.53, 886958.6 , 447162.84,
475270.97, 442856.4 , 1082353.1 , 416774.6 , 785664.75,
383833.88, 403520.16, 404040.78, 921561.56, 437177.97,
482485.6 , 244380.27, 340764.53, 266405.63, 718588.94,
450867.7 , 332134.97, 563844.44, 701940.7 , 784103.56,
277145.63, 418823.38, 784103.56, 450996.34, 426066.38,
758714.3 , 1227073.8 , 332134.97, 290323.1 , 559631.06,
277145.63, 701940.7 , 291857.06, 404484.53, 418823.38,
236238.67, 380009.28, 342604.47, 542342. , 243585.28,
516278.63, 448627.8 , 594678.75, 431929.2 , 291239.75,
349102.75, 723934.9 , 559631.06, 517121.56, 423382.72,
296202.7 , 435628.72, 252192.9 , 1039781.2 , 450867.7 ,
303936.78, 393833.97, 403520.16, 276709.06, 284845.34,
320863.72, 308049.63, 267013.97, 701940.7 , 291239.75,
919031.5 , 461279.1 , 450867.7 , 557391.25, 726181.75,
700271.56, 400561.2 , 542342. , 788215.2 , 450996.34,
675545.44, 340764.53, 765468.9 , 536371.2 , 435628.72,
846775.06, 765468.9 , 641162.2 , 779810.06, 683845.75,
630865.5 , 261886.94, 300480.3 , 404676.1 , 675545.44])
Create Assay from Numpy Values
Assays are created with the wallaroo.client.build_assay
method. We provide the following parameters:
- The unique name of the assay
- The pipeline
- The name of the model to track inference data from
- The model name
- The iopath (the fields output by the model to track)
- The baseline data - our derived numpy array.
assay_baseline_from_numpy_name = "assays from numpy for edge"
# assay builder by baseline
assay_builder_from_numpy = wl.build_assay(assay_name=assay_baseline_from_numpy_name,
pipeline=mainpipeline,
model_name=model_name_control,
iopath="output variable 0",
baseline_data = small_results_baseline)
We’ll display the histogram of the current baseline, which came directly from the previous inference results.
# assay_builder_from_numpy.window_builder().add_width(minutes=1)
# assay_builder_from_numpy.window_builder().add_start(assay_baseline_start)
<wallaroo.assay_config.WindowBuilder at 0x177b80c40>
# assay_config_from_numpy = assay_builder_from_numpy.build()
# assay_analysis_from_numpy = assay_config_from_numpy.interactive_run()
# get the histogram from the numpy baseline
assay_builder_from_numpy.baseline_histogram()
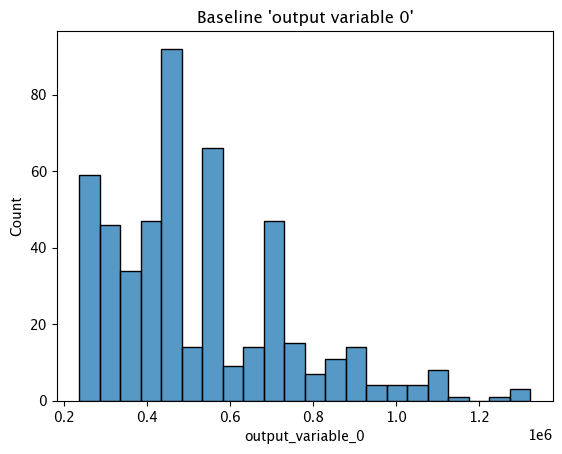
Assay Testing
Now we’ll perform some inferences with a spread of values, then a larger set with a set of larger house values to trigger our assay alert. We’ll use our assay created from the numpy baseline values to demonstrate.
Because our assay windows are 1 minutes, we’ll need to stagger our inference values to be set into the proper windows. This will take about 4 minutes.
# Get a spread of house values
# Set the start for our assay window period.
assay_window_start = datetime.datetime.now()
time.sleep(35)
regular_houses_inputs = pd.read_json('./data/xtest-1k.df.json', orient="records")
inference_size = 1000
regular_houses = regular_houses_inputs.sample(inference_size, replace=True).reset_index(drop=True)
# And a spread of large house values
time.sleep(65)
big_houses_inputs = pd.read_json('./data/biginputs.df.json', orient="records")
big_houses = big_houses_inputs.sample(inference_size, replace=True).reset_index(drop=True)
mainpipeline.infer(big_houses)
# End our assay window period
assay_window_end = datetime.datetime.now()
Assay Configuration
Because we are using a short amount of data, we’ll shorten the window and interval to just 1 minute each, and narrow the window to when we started our set of inferences to when we finish.
assay_builder_from_numpy.add_run_until(assay_window_end)
assay_builder_from_numpy.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_numpy.build()
assay_analysis_from_numpy = assay_config_from_numpy.interactive_run()
# Show how many assay windows were analyzed, then show the chart
print(f"Generated {len(assay_analysis_from_numpy)} analyses")
assay_analysis_from_numpy.chart_scores()
Generated 2 analyses
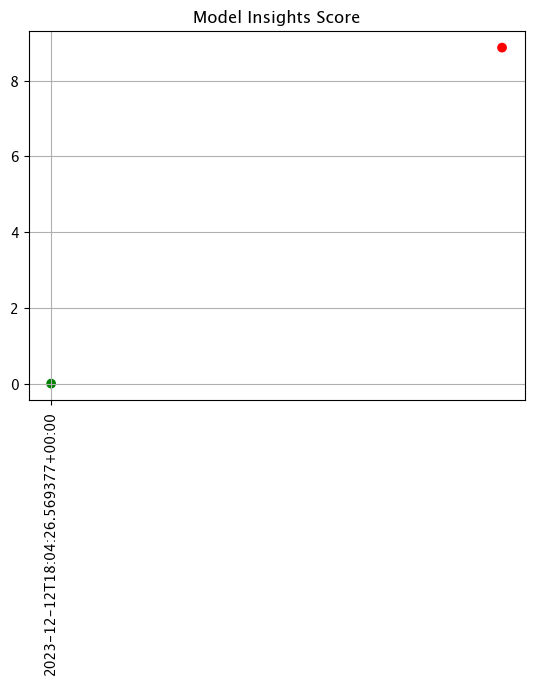
# Display the results as a DataFrame - we're mainly interested in the score and whether the
# alert threshold was triggered
display(assay_analysis_from_numpy.to_dataframe().loc[:, ["score", "start", "alert_threshold", "status"]])
score | start | alert_threshold | status | |
---|---|---|---|---|
0 | 0.002769 | 2023-12-12T18:04:26.569377+00:00 | 0.25 | Ok |
1 | 8.869974 | 2023-12-12T18:05:26.569377+00:00 | 0.25 | Alert |
Undeploy Main Pipeline
With the examples and tutorial complete, we will undeploy the main pipeline and return the resources back to the Wallaroo instance.
mainpipeline.undeploy()
name | houseprice-estimation-edge |
---|---|
created | 2023-12-12 18:03:28.275490+00:00 |
last_updated | 2023-12-12 18:03:29.206241+00:00 |
deployed | False |
arch | None |
tags | |
versions | 66d73202-0c8c-4468-b589-67286a2f5dd6, be11e14c-1565-4d68-8bf5-f9eebd7cd458 |
steps | housepricesagacontrol |
published | False |
Edge Deployment
We can now deploy the pipeline to an edge device. This will require the following steps:
- Publish the pipeline: Publishes the pipeline to the OCI registry.
- Add Edge: Add the edge location to the pipeline publish.
- Deploy Edge: Deploy the edge device with the edge location settings.
Publish Pipeline
Publishing the pipeline uses the pipeline publish()
command, which returns the OCI registry information.
assay_pub = mainpipeline.publish()
Waiting for pipeline publish... It may take up to 600 sec.
Pipeline is Publishing....Published.
Add Edge
The edge location is added with the publish.add_edge(name)
method. This returns the OCI registration information, and the EDGE_BUNDLE
information. The EDGE_BUNDLE
data is a base64 encoded set of parameters for the pipeline that the edge device is associated with.
display(edge_name)
edge_publish = assay_pub.add_edge(edge_name)
display(edge_publish)
'house-price-edge-jch'
ID | 3 |
Pipeline Version | 210d54bf-2788-4e61-be63-31a57738ce15 |
Status | Published |
Engine URL | ghcr.io/wallaroolabs/doc-samples/engines/proxy/wallaroo/ghcr.io/wallaroolabs/standalone-mini:v2023.4.0-4103 |
Pipeline URL | ghcr.io/wallaroolabs/doc-samples/pipelines/houseprice-estimation-edge:210d54bf-2788-4e61-be63-31a57738ce15 |
Helm Chart URL | oci://ghcr.io/wallaroolabs/doc-samples/charts/houseprice-estimation-edge |
Helm Chart Reference | ghcr.io/wallaroolabs/doc-samples/charts@sha256:a075005a0aca85006392b49edcb0066f17261f32ace3796e87e2a753d78f42d3 |
Helm Chart Version | 0.0.1-210d54bf-2788-4e61-be63-31a57738ce15 |
Engine Config | {'engine': {'resources': {'limits': {'cpu': 4.0, 'memory': '3Gi'}, 'requests': {'cpu': 4.0, 'memory': '3Gi'}}}, 'engineAux': {}, 'enginelb': {'resources': {'limits': {'cpu': 1.0, 'memory': '512Mi'}, 'requests': {'cpu': 0.2, 'memory': '512Mi'}}}} |
User Images | [] |
Created By | john.hansarick@wallaroo.ai |
Created At | 2023-12-12 18:16:53.829858+00:00 |
Updated At | 2023-12-12 18:16:53.829858+00:00 |
Docker Run Variables | {'EDGE_BUNDLE': 'abcde'} |
DevOps Deployment
The edge deployment is performed with docker run
, docker compose
, or helm
installations. The following command generates the docker run
command, with the following values provided by the DevOps Engineer:
$REGISTRYURL
$REGISTRYUSERNAME
$REGISTRYPASSWORD
Before deploying, create the ./data
directory that is used to store the authentication credentials.
# create docker run
docker_command = f'''
docker run -p 8080:8080 \\
-v ./data:/persist \\
-e DEBUG=true \\
-e OCI_REGISTRY=$REGISTRYURL \\
-e EDGE_BUNDLE={edge_publish.docker_run_variables['EDGE_BUNDLE']} \\
-e CONFIG_CPUS=1 \\
-e OCI_USERNAME=$REGISTRYUSERNAME \\
-e OCI_PASSWORD=$REGISTRYPASSWORD \\
-e PIPELINE_URL={edge_publish.pipeline_url} \\
{edge_publish.engine_url}
'''
print(docker_command)
docker run -p 8080:8080 \
-v ./data:/persist \
-e DEBUG=true \
-e OCI_REGISTRY=$REGISTRYURL \
-e EDGE_BUNDLE=ZXhwb3J0IEJVTkRMRV9WRVJTSU9OPTEKZXhwb3J0IEVER0VfTkFNRT1ob3VzZS1wcmljZS1lZGdlLWpjaApleHBvcnQgSk9JTl9UT0tFTj05MzNjNDIyNy0wZWYxLTQxODQtYTkyZS1lMWFiYzNkM2Y0M2YKZXhwb3J0IE9QU0NFTlRFUl9IT1NUPWRvYy10ZXN0LmVkZ2Uud2FsbGFyb29jb21tdW5pdHkubmluamEKZXhwb3J0IFBJUEVMSU5FX1VSTD1naGNyLmlvL3dhbGxhcm9vbGFicy9kb2Mtc2FtcGxlcy9waXBlbGluZXMvaG91c2VwcmljZS1lc3RpbWF0aW9uLWVkZ2U6MjEwZDU0YmYtMjc4OC00ZTYxLWJlNjMtMzFhNTc3MzhjZTE1CmV4cG9ydCBXT1JLU1BBQ0VfSUQ9MTE= \
-e CONFIG_CPUS=1 \
-e OCI_USERNAME=$REGISTRYUSERNAME \
-e OCI_PASSWORD=$REGISTRYPASSWORD \
-e PIPELINE_URL=ghcr.io/wallaroolabs/doc-samples/pipelines/houseprice-estimation-edge:210d54bf-2788-4e61-be63-31a57738ce15 \
ghcr.io/wallaroolabs/doc-samples/engines/proxy/wallaroo/ghcr.io/wallaroolabs/standalone-mini:v2023.4.0-4103
Verify Logs
Before we perform inferences on the edge deployment, we’ll collect the pipeline logs and display the current partitions. These should only include the Wallaroo Ops pipeline.
logs = mainpipeline.logs(dataset=['time', 'out.variable', 'metadata'])
ops_locations = [pd.unique(logs['metadata.partition']).tolist()][0]
display(ops_locations)
ops_location = ops_locations[0]
Warning: There are more logs available. Please set a larger limit or request a file using export_logs.
['engine-6c9f97965b-pgjrw']
Edge Inferences
We will perform sample inference on our edge location, then verify that the inference results are added to our pipeline logs with the name of our edge location. For these example, the edge location is on the hostname testboy.local
.
edge_datetime_start = datetime.datetime.now()
!curl testboy.local:8080/pipelines
{"pipelines":[{"id":"houseprice-estimation-edge","status":"Running"}]}
!curl -X POST testboy.local:8080/pipelines/{main_pipeline_name} \
-H "Content-Type: Content-Type: application/json; format=pandas-records" \
--data @./data/xtest-1k.df.json
[{"time":1702405553622,"in":{"tensor":[4.0,2.5,2900.0,5505.0,2.0,0.0,0.0,3.0,8.0,2900.0,0.0,47.6063,-122.02,2970.0,5251.0,12.0,0.0,0.0]},"out":{"variable":[718013.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.5,2170.0,6361.0,1.0,0.0,2.0,3.0,8.0,2170.0,0.0,47.7109,-122.017,2310.0,7419.0,6.0,0.0,0.0]},"out":{"variable":[615094.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1300.0,812.0,2.0,0.0,0.0,3.0,8.0,880.0,420.0,47.5893,-122.317,1300.0,824.0,6.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2500.0,8540.0,2.0,0.0,0.0,3.0,9.0,2500.0,0.0,47.5759,-121.994,2560.0,8475.0,24.0,0.0,0.0]},"out":{"variable":[758714.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2200.0,11520.0,1.0,0.0,0.0,4.0,7.0,2200.0,0.0,47.7659,-122.341,1690.0,8038.0,62.0,0.0,0.0]},"out":{"variable":[513264.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,2140.0,4923.0,1.0,0.0,0.0,4.0,8.0,1070.0,1070.0,47.6902,-122.339,1470.0,4923.0,86.0,0.0,0.0]},"out":{"variable":[668287.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.5,3590.0,5334.0,2.0,0.0,2.0,3.0,9.0,3140.0,450.0,47.6763,-122.267,2100.0,6250.0,9.0,0.0,0.0]},"out":{"variable":[1004846.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1280.0,960.0,2.0,0.0,0.0,3.0,9.0,1040.0,240.0,47.602,-122.311,1280.0,1173.0,0.0,0.0,0.0]},"out":{"variable":[684577.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2820.0,15000.0,2.0,0.0,0.0,4.0,9.0,2820.0,0.0,47.7255,-122.101,2440.0,15000.0,29.0,0.0,0.0]},"out":{"variable":[727898.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1790.0,11393.0,1.0,0.0,0.0,3.0,8.0,1790.0,0.0,47.6297,-122.099,2290.0,11894.0,36.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1010.0,7683.0,1.5,0.0,0.0,5.0,7.0,1010.0,0.0,47.72,-122.318,1550.0,7271.0,61.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1270.0,1323.0,3.0,0.0,0.0,3.0,8.0,1270.0,0.0,47.6934,-122.342,1330.0,1323.0,8.0,0.0,0.0]},"out":{"variable":[442168.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2070.0,9120.0,1.0,0.0,0.0,4.0,7.0,1250.0,820.0,47.6045,-122.123,1650.0,8400.0,57.0,0.0,0.0]},"out":{"variable":[630865.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.0,1620.0,4080.0,1.5,0.0,0.0,3.0,7.0,1620.0,0.0,47.6696,-122.324,1760.0,4080.0,91.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,3990.0,9786.0,2.0,0.0,0.0,3.0,9.0,3990.0,0.0,47.6784,-122.026,3920.0,8200.0,10.0,0.0,0.0]},"out":{"variable":[909441.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,1780.0,19843.0,1.0,0.0,0.0,3.0,7.0,1780.0,0.0,47.4414,-122.154,2210.0,13500.0,52.0,0.0,0.0]},"out":{"variable":[313096.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2130.0,6003.0,2.0,0.0,0.0,3.0,8.0,2130.0,0.0,47.4518,-122.12,1940.0,4529.0,11.0,0.0,0.0]},"out":{"variable":[404040.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1660.0,10440.0,1.0,0.0,0.0,3.0,7.0,1040.0,620.0,47.4448,-121.77,1240.0,10380.0,36.0,0.0,0.0]},"out":{"variable":[292859.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2110.0,4118.0,2.0,0.0,0.0,3.0,8.0,2110.0,0.0,47.3878,-122.153,2110.0,4044.0,25.0,0.0,0.0]},"out":{"variable":[338357.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2200.0,11250.0,1.5,0.0,0.0,5.0,7.0,1300.0,900.0,47.6845,-122.201,2320.0,10814.0,94.0,0.0,0.0]},"out":{"variable":[682284.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2020.0,10260.0,2.0,0.0,0.0,4.0,7.0,2020.0,0.0,47.6801,-122.114,2020.0,10311.0,31.0,0.0,0.0]},"out":{"variable":[583765.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.0,1390.0,1302.0,2.0,0.0,0.0,3.0,7.0,1390.0,0.0,47.3089,-122.33,1390.0,1302.0,28.0,0.0,0.0]},"out":{"variable":[249227.78]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,1400.0,7920.0,1.0,0.0,0.0,3.0,7.0,1400.0,0.0,47.4658,-122.184,1910.0,7700.0,52.0,0.0,0.0]},"out":{"variable":[267013.97]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.5,1370.0,1140.0,2.0,0.0,0.0,3.0,8.0,1080.0,290.0,47.7055,-122.342,1340.0,1050.0,5.0,0.0,0.0]},"out":{"variable":[342604.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2390.0,6976.0,2.0,0.0,0.0,3.0,7.0,2390.0,0.0,47.4807,-122.182,2390.0,6346.0,11.0,0.0,0.0]},"out":{"variable":[410291.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.0,2170.0,2440.0,1.5,0.0,0.0,4.0,8.0,1450.0,720.0,47.6724,-122.317,2070.0,4000.0,103.0,0.0,0.0]},"out":{"variable":[675545.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1370.0,9460.0,1.0,0.0,0.0,3.0,6.0,1370.0,0.0,47.6238,-122.191,1690.0,9930.0,65.0,0.0,0.0]},"out":{"variable":[449699.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2270.0,5000.0,2.0,0.0,0.0,3.0,9.0,2270.0,0.0,47.6916,-122.37,1210.0,5000.0,0.0,0.0,0.0]},"out":{"variable":[756046.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.0,1330.0,1379.0,2.0,0.0,0.0,4.0,8.0,1120.0,210.0,47.6126,-122.313,1810.0,1770.0,9.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,1.75,2490.0,24969.0,1.0,0.0,2.0,4.0,8.0,1540.0,950.0,47.336,-122.35,2790.0,15600.0,55.0,0.0,0.0]},"out":{"variable":[392724.16]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.0,3710.0,20000.0,2.0,0.0,2.0,5.0,10.0,2760.0,950.0,47.6696,-122.261,3970.0,20000.0,79.0,0.0,0.0]},"out":{"variable":[1514079.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2800.0,17300.0,1.0,0.0,0.0,4.0,8.0,1420.0,1380.0,47.5716,-122.128,2140.0,12650.0,44.0,0.0,0.0]},"out":{"variable":[706407.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1790.0,50529.0,1.0,0.0,0.0,5.0,7.0,1090.0,700.0,47.3511,-122.073,1940.0,50529.0,50.0,0.0,0.0]},"out":{"variable":[291904.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1340.0,11744.0,1.0,0.0,0.0,2.0,7.0,1340.0,0.0,47.4947,-122.36,2020.0,13673.0,64.0,0.0,0.0]},"out":{"variable":[259657.52]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2110.0,7434.0,2.0,0.0,0.0,4.0,7.0,2110.0,0.0,47.3935,-122.169,2100.0,7749.0,36.0,0.0,0.0]},"out":{"variable":[335398.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2440.0,3600.0,2.5,0.0,0.0,4.0,8.0,2440.0,0.0,47.6298,-122.362,2440.0,5440.0,112.0,0.0,0.0]},"out":{"variable":[713978.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2100.0,11894.0,1.0,0.0,0.0,4.0,8.0,1720.0,380.0,47.6006,-122.194,2390.0,9450.0,46.0,0.0,0.0]},"out":{"variable":[642519.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1280.0,959.0,3.0,0.0,0.0,3.0,8.0,1280.0,0.0,47.6914,-122.343,1130.0,1126.0,9.0,0.0,0.0]},"out":{"variable":[446768.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1740.0,9038.0,1.0,0.0,0.0,4.0,7.0,1740.0,0.0,47.5897,-122.136,1390.0,9770.0,59.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.5,1860.0,3504.0,2.0,0.0,0.0,3.0,7.0,1860.0,0.0,47.776,-122.239,1860.0,4246.0,14.0,0.0,0.0]},"out":{"variable":[438514.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,4.5,5120.0,41327.0,2.0,0.0,0.0,3.0,10.0,3290.0,1830.0,47.7009,-122.059,3360.0,82764.0,6.0,0.0,0.0]},"out":{"variable":[1204324.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,670.0,11505.0,1.0,0.0,0.0,3.0,5.0,670.0,0.0,47.499,-122.157,2180.0,11505.0,12.0,0.0,0.0]},"out":{"variable":[251194.61]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2370.0,4200.0,2.0,0.0,0.0,3.0,8.0,2370.0,0.0,47.3699,-122.019,2370.0,4370.0,1.0,0.0,0.0]},"out":{"variable":[349102.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.75,2580.0,7865.0,1.0,0.0,0.0,4.0,8.0,1480.0,1100.0,47.6208,-122.139,2140.0,8400.0,51.0,0.0,0.0]},"out":{"variable":[701940.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,998.0,904.0,2.0,0.0,0.0,3.0,7.0,798.0,200.0,47.6983,-122.367,998.0,1110.0,7.0,0.0,0.0]},"out":{"variable":[400561.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2930.0,7806.0,2.0,0.0,0.0,3.0,9.0,2930.0,0.0,47.6219,-122.024,2600.0,6051.0,9.0,0.0,0.0]},"out":{"variable":[779809.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1120.0,4000.0,1.0,0.0,0.0,4.0,7.0,870.0,250.0,47.6684,-122.368,1470.0,4000.0,98.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.0,2000.0,4800.0,2.0,0.0,0.0,4.0,7.0,2000.0,0.0,47.6583,-122.351,1260.0,1452.0,104.0,0.0,0.0]},"out":{"variable":[581002.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1520.0,3370.0,2.0,0.0,0.0,3.0,8.0,1520.0,0.0,47.5696,-122.004,1860.0,4486.0,21.0,0.0,0.0]},"out":{"variable":[535229.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2920.0,9904.0,2.0,0.0,0.0,4.0,9.0,2920.0,0.0,47.5759,-121.995,1810.0,5617.0,24.0,0.0,0.0]},"out":{"variable":[778197.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1850.0,8208.0,1.0,0.0,0.0,4.0,7.0,1180.0,670.0,47.3109,-122.362,1790.0,8174.0,44.0,0.0,0.0]},"out":{"variable":[291261.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3430.0,35120.0,2.0,0.0,0.0,3.0,10.0,3430.0,0.0,47.6484,-122.182,3920.0,35230.0,31.0,0.0,0.0]},"out":{"variable":[950176.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,1530.0,6375.0,2.0,0.0,0.0,3.0,7.0,1530.0,0.0,47.4692,-122.162,1500.0,8712.0,72.0,1.0,41.0]},"out":{"variable":[289359.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1640.0,8400.0,1.0,0.0,0.0,3.0,8.0,1180.0,460.0,47.3733,-122.289,1600.0,8120.0,46.0,0.0,0.0]},"out":{"variable":[277145.72]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1540.0,8400.0,1.0,0.0,0.0,3.0,7.0,1180.0,360.0,47.6554,-122.129,1550.0,8760.0,46.0,0.0,0.0]},"out":{"variable":[552992.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1850.0,8310.0,1.0,0.0,0.0,3.0,7.0,1200.0,650.0,47.7717,-122.29,1840.0,10080.0,53.0,0.0,0.0]},"out":{"variable":[437753.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1530.0,7245.0,1.0,0.0,0.0,4.0,7.0,1530.0,0.0,47.731,-122.191,1530.0,7490.0,31.0,0.0,0.0]},"out":{"variable":[431929.03]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2610.0,5866.0,2.0,0.0,0.0,3.0,8.0,2610.0,0.0,47.3441,-122.04,2480.0,5188.0,9.0,0.0,0.0]},"out":{"variable":[358668.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1710.0,5000.0,1.0,0.0,0.0,3.0,8.0,1110.0,600.0,47.6772,-122.285,1750.0,5304.0,36.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.5,3470.0,12003.0,2.0,0.0,0.0,3.0,8.0,3470.0,0.0,47.624,-122.048,2220.0,12283.0,37.0,0.0,0.0]},"out":{"variable":[732736.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1020.0,1204.0,2.0,0.0,0.0,3.0,7.0,720.0,300.0,47.5445,-122.376,1360.0,1506.0,10.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.75,2870.0,6600.0,2.0,0.0,2.0,3.0,8.0,2870.0,0.0,47.5745,-122.113,2570.0,7925.0,30.0,0.0,0.0]},"out":{"variable":[710023.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.0,1440.0,4666.0,1.0,0.0,0.0,3.0,8.0,1440.0,0.0,47.709,-122.019,1510.0,4595.0,5.0,0.0,0.0]},"out":{"variable":[359614.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.0,4040.0,19700.0,2.0,0.0,0.0,3.0,11.0,4040.0,0.0,47.7205,-122.127,3930.0,21887.0,27.0,0.0,0.0]},"out":{"variable":[1028923.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[6.0,4.5,3300.0,7480.0,2.0,0.0,0.0,3.0,8.0,3300.0,0.0,47.6796,-122.104,2470.0,7561.0,34.0,0.0,0.0]},"out":{"variable":[725572.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1670.0,3852.0,2.0,0.0,3.0,4.0,8.0,1670.0,0.0,47.6411,-122.371,2320.0,4572.0,87.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1700.0,6031.0,2.0,0.0,0.0,3.0,8.0,1700.0,0.0,47.3582,-122.191,1930.0,6035.0,20.0,0.0,0.0]},"out":{"variable":[290323.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3220.0,7873.0,2.0,0.0,0.0,3.0,10.0,3220.0,0.0,47.5849,-122.03,2610.0,8023.0,21.0,0.0,0.0]},"out":{"variable":[886958.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.25,3030.0,20446.0,2.0,0.0,2.0,3.0,9.0,2130.0,900.0,47.6133,-122.106,2890.0,20908.0,38.0,0.0,0.0]},"out":{"variable":[811666.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1050.0,9871.0,1.0,0.0,0.0,5.0,7.0,1050.0,0.0,47.3816,-122.087,1300.0,10794.0,46.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,2230.0,6000.0,1.0,0.0,1.0,3.0,8.0,1260.0,970.0,47.5373,-122.395,2120.0,7200.0,47.0,0.0,0.0]},"out":{"variable":[683845.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2710.0,6474.0,2.0,0.0,0.0,3.0,8.0,2710.0,0.0,47.5383,-121.878,2870.0,6968.0,11.0,0.0,0.0]},"out":{"variable":[717470.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3490.0,18521.0,2.0,0.0,0.0,4.0,9.0,3490.0,0.0,47.7406,-122.07,2850.0,18521.0,24.0,0.0,0.0]},"out":{"variable":[873314.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,720.0,5820.0,1.0,0.0,1.0,5.0,6.0,720.0,0.0,47.7598,-122.255,952.0,5820.0,65.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2100.0,11942.0,1.0,0.0,0.0,3.0,7.0,1030.0,1070.0,47.55,-122.356,1170.0,6986.0,50.0,0.0,0.0]},"out":{"variable":[642519.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.5,3240.0,6919.0,2.0,0.0,0.0,3.0,8.0,2760.0,480.0,47.4779,-122.122,2970.0,5690.0,0.0,0.0,0.0]},"out":{"variable":[464811.22]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1410.0,5000.0,1.0,0.0,2.0,4.0,7.0,940.0,470.0,47.5531,-122.379,1450.0,5000.0,97.0,0.0,0.0]},"out":{"variable":[437929.72]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.75,2300.0,5090.0,2.0,0.0,0.0,3.0,8.0,1700.0,600.0,47.545,-122.36,1530.0,9100.0,7.0,0.0,0.0]},"out":{"variable":[686890.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2410.0,7140.0,2.0,0.0,0.0,3.0,8.0,2410.0,0.0,47.6329,-122.021,2350.0,7140.0,21.0,0.0,0.0]},"out":{"variable":[701940.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,1920.0,7455.0,1.0,0.0,0.0,4.0,7.0,960.0,960.0,47.7106,-122.286,1920.0,7455.0,75.0,1.0,25.0]},"out":{"variable":[447162.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,1670.0,9886.0,1.0,0.0,0.0,5.0,7.0,1670.0,0.0,47.7249,-122.287,2590.0,9997.0,67.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1880.0,5500.0,2.0,0.0,0.0,4.0,7.0,1880.0,0.0,47.657,-122.393,1230.0,5500.0,67.0,1.0,60.0]},"out":{"variable":[561604.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1270.0,10790.0,1.0,0.0,0.0,3.0,7.0,1270.0,0.0,47.6647,-122.177,1270.0,10790.0,58.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1070.0,8100.0,1.0,0.0,0.0,4.0,6.0,1070.0,0.0,47.2853,-122.22,1260.0,8100.0,57.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2480.0,4950.0,2.0,0.0,0.0,3.0,7.0,2480.0,0.0,47.4389,-122.116,2230.0,5298.0,11.0,0.0,0.0]},"out":{"variable":[397183.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1400.0,4914.0,1.5,0.0,0.0,3.0,7.0,1400.0,0.0,47.681,-122.364,1400.0,3744.0,85.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3190.0,4980.0,2.0,0.0,0.0,3.0,9.0,3190.0,0.0,47.3657,-122.034,2830.0,6720.0,10.0,0.0,0.0]},"out":{"variable":[511200.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1700.0,4400.0,1.5,0.0,0.0,4.0,8.0,1700.0,0.0,47.612,-122.292,1610.0,4180.0,108.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.5,1620.0,14467.0,2.0,0.0,0.0,3.0,7.0,1620.0,0.0,47.6306,-122.035,1470.0,13615.0,33.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2160.0,8809.0,1.0,0.0,0.0,3.0,9.0,1540.0,620.0,47.6994,-122.349,930.0,5420.0,0.0,0.0,0.0]},"out":{"variable":[726181.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1060.0,7868.0,1.0,0.0,0.0,3.0,7.0,1060.0,0.0,47.7414,-122.295,1530.0,10728.0,63.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.25,2320.0,18919.0,2.0,1.0,4.0,4.0,8.0,2320.0,0.0,47.3905,-122.462,1610.0,18919.0,39.0,0.0,0.0]},"out":{"variable":[491385.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[6.0,3.75,3190.0,4700.0,2.0,0.0,0.0,3.0,8.0,3190.0,0.0,47.3724,-122.105,2680.0,5640.0,12.0,0.0,0.0]},"out":{"variable":[383833.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[6.0,2.0,1900.0,8240.0,1.0,0.0,0.0,2.0,7.0,1200.0,700.0,47.7037,-122.296,1900.0,8240.0,51.0,0.0,0.0]},"out":{"variable":[458858.38]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,3940.0,27591.0,2.0,0.0,3.0,3.0,9.0,3440.0,500.0,47.5157,-122.116,3420.0,29170.0,14.0,0.0,0.0]},"out":{"variable":[736751.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1340.0,3011.0,2.0,0.0,0.0,3.0,7.0,1340.0,0.0,47.3839,-122.038,1060.0,3232.0,19.0,0.0,0.0]},"out":{"variable":[244380.27]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,2280.0,8339.0,1.0,0.0,0.0,4.0,7.0,1220.0,1060.0,47.6986,-122.297,1970.0,8340.0,61.0,0.0,0.0]},"out":{"variable":[665791.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2510.0,12013.0,2.0,0.0,0.0,3.0,8.0,2510.0,0.0,47.3473,-122.314,1870.0,8017.0,27.0,0.0,0.0]},"out":{"variable":[352864.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,1.5,1750.0,12491.0,1.0,0.0,0.0,3.0,6.0,1390.0,360.0,47.6995,-122.174,1560.0,12473.0,54.0,0.0,0.0]},"out":{"variable":[467742.97]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1590.0,4600.0,1.5,0.0,0.0,4.0,7.0,1290.0,300.0,47.6807,-122.399,1770.0,4350.0,88.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1740.0,3060.0,1.0,0.0,0.0,5.0,8.0,950.0,790.0,47.6816,-122.31,1800.0,3960.0,84.0,1.0,84.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2570.0,11070.0,2.0,0.0,0.0,4.0,8.0,2570.0,0.0,47.4507,-122.152,2210.0,9600.0,49.0,0.0,0.0]},"out":{"variable":[427942.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2420.0,11120.0,1.0,0.0,2.0,4.0,8.0,1620.0,800.0,47.4954,-122.366,2210.0,8497.0,63.0,0.0,0.0]},"out":{"variable":[447951.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2632.0,4117.0,2.0,0.0,0.0,3.0,8.0,2632.0,0.0,47.3428,-122.278,2040.0,5195.0,1.0,0.0,0.0]},"out":{"variable":[368504.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1440.0,11330.0,1.0,0.0,0.0,3.0,7.0,1440.0,0.0,47.4742,-122.265,1580.0,10100.0,50.0,0.0,0.0]},"out":{"variable":[263051.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.25,1630.0,1686.0,2.0,0.0,0.0,3.0,10.0,1330.0,300.0,47.6113,-122.314,1570.0,2580.0,0.0,0.0,0.0]},"out":{"variable":[917346.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.5,2600.0,3839.0,2.0,0.0,0.0,3.0,7.0,2600.0,0.0,47.4324,-122.145,2180.0,4800.0,9.0,0.0,0.0]},"out":{"variable":[400676.47]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,3160.0,8429.0,1.0,0.0,3.0,4.0,7.0,1620.0,1540.0,47.511,-122.251,1760.0,6780.0,33.0,0.0,0.0]},"out":{"variable":[480989.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1480.0,5400.0,2.0,0.0,0.0,4.0,8.0,1480.0,0.0,47.6095,-122.296,1280.0,3600.0,100.0,0.0,0.0]},"out":{"variable":[533935.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2040.0,12065.0,1.0,0.0,0.0,3.0,8.0,2040.0,0.0,47.3756,-122.044,2010.0,11717.0,27.0,0.0,0.0]},"out":{"variable":[328513.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3470.0,20445.0,2.0,0.0,0.0,4.0,10.0,3470.0,0.0,47.547,-122.219,3360.0,21950.0,51.0,0.0,0.0]},"out":{"variable":[1412215.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1400.0,6380.0,1.0,0.0,0.0,4.0,7.0,700.0,700.0,47.7015,-122.316,1690.0,5800.0,91.0,0.0,0.0]},"out":{"variable":[394707.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,1610.0,8976.0,1.0,0.0,0.0,4.0,8.0,1610.0,0.0,47.6011,-122.192,1930.0,8976.0,48.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1850.0,8770.0,2.0,0.0,0.0,3.0,8.0,1850.0,0.0,47.2091,-122.009,2350.0,8606.0,18.0,0.0,0.0]},"out":{"variable":[291857.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,2170.0,9948.0,2.0,0.0,0.0,3.0,7.0,2170.0,0.0,47.4263,-122.331,1500.0,9750.0,62.0,0.0,0.0]},"out":{"variable":[371795.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.25,1950.0,8086.0,1.0,0.0,0.0,3.0,7.0,1130.0,820.0,47.3179,-122.331,1670.0,8550.0,35.0,0.0,0.0]},"out":{"variable":[312429.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2080.0,87991.0,1.0,0.0,0.0,3.0,6.0,1040.0,1040.0,47.6724,-121.865,2080.0,84300.0,44.0,0.0,0.0]},"out":{"variable":[632114.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,2580.0,4500.0,2.0,0.0,0.0,4.0,9.0,1850.0,730.0,47.6245,-122.316,2590.0,4100.0,110.0,0.0,0.0]},"out":{"variable":[964052.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,2240.0,7725.0,1.0,0.0,0.0,5.0,7.0,1120.0,1120.0,47.5331,-122.365,1340.0,6300.0,58.0,0.0,0.0]},"out":{"variable":[423382.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,800.0,1200.0,2.0,0.0,0.0,3.0,7.0,800.0,0.0,47.6969,-122.347,806.0,1200.0,15.0,0.0,0.0]},"out":{"variable":[404484.38]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1980.0,3243.0,1.5,0.0,0.0,3.0,8.0,1980.0,0.0,47.6429,-122.327,1380.0,1249.0,102.0,0.0,0.0]},"out":{"variable":[577149.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.5,1280.0,1257.0,2.0,0.0,0.0,3.0,8.0,1040.0,240.0,47.6721,-122.374,1280.0,1249.0,14.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2000.0,14733.0,1.0,0.0,0.0,4.0,8.0,2000.0,0.0,47.6001,-122.178,2620.0,14733.0,57.0,0.0,0.0]},"out":{"variable":[581002.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.25,1350.0,1493.0,2.0,0.0,0.0,3.0,8.0,1050.0,300.0,47.5421,-122.388,1250.0,1202.0,7.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1200.0,10890.0,1.0,0.0,0.0,5.0,7.0,1200.0,0.0,47.3423,-122.088,1250.0,10139.0,42.0,0.0,0.0]},"out":{"variable":[241330.19]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1850.0,7684.0,1.0,0.0,0.0,3.0,8.0,1320.0,530.0,47.2975,-122.37,1940.0,7630.0,35.0,0.0,0.0]},"out":{"variable":[291857.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,1440.0,725.0,2.0,0.0,0.0,3.0,8.0,1100.0,340.0,47.5607,-122.378,1440.0,4255.0,3.0,0.0,0.0]},"out":{"variable":[447192.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1040.0,4240.0,1.0,0.0,0.0,4.0,7.0,860.0,180.0,47.6768,-122.367,1170.0,4240.0,90.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,1088.0,1360.0,2.0,0.0,0.0,3.0,7.0,1088.0,0.0,47.7094,-122.213,1098.0,1469.0,31.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1260.0,15000.0,1.5,0.0,0.0,4.0,7.0,1260.0,0.0,47.2737,-122.153,2400.0,21715.0,32.0,0.0,0.0]},"out":{"variable":[254622.98]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2620.0,13777.0,1.5,0.0,2.0,4.0,9.0,1720.0,900.0,47.58,-122.285,3530.0,9287.0,88.0,0.0,0.0]},"out":{"variable":[1223838.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2260.0,41236.0,1.0,0.0,0.0,4.0,8.0,1690.0,570.0,47.5528,-122.034,3080.0,30240.0,53.0,0.0,0.0]},"out":{"variable":[695842.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1640.0,9234.0,1.0,0.0,0.0,5.0,7.0,1060.0,580.0,47.5162,-122.274,2230.0,10354.0,47.0,0.0,0.0]},"out":{"variable":[332134.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.25,3320.0,13138.0,1.0,0.0,2.0,4.0,9.0,1900.0,1420.0,47.759,-122.269,2820.0,13138.0,51.0,0.0,0.0]},"out":{"variable":[1108000.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1320.0,1824.0,1.5,0.0,0.0,4.0,6.0,1320.0,0.0,47.585,-122.294,1320.0,4000.0,105.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2250.0,10160.0,2.0,0.0,0.0,5.0,8.0,2250.0,0.0,47.5645,-122.219,2660.0,10125.0,48.0,0.0,0.0]},"out":{"variable":[684559.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1740.0,3690.0,2.0,0.0,0.0,3.0,8.0,1740.0,0.0,47.5345,-121.867,2100.0,4944.0,14.0,0.0,0.0]},"out":{"variable":[517771.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1730.0,4286.0,2.0,0.0,0.0,3.0,7.0,1730.0,0.0,47.432,-122.329,1780.0,4343.0,16.0,0.0,0.0]},"out":{"variable":[310164.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[1.0,1.0,1020.0,3200.0,1.0,0.0,0.0,3.0,7.0,1020.0,0.0,47.6361,-122.343,1670.0,3480.0,87.0,0.0,0.0]},"out":{"variable":[449699.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1420.0,1438.0,2.0,0.0,0.0,3.0,9.0,1280.0,140.0,47.6265,-122.323,1490.0,1439.0,11.0,0.0,0.0]},"out":{"variable":[684577.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1180.0,1800.0,2.0,0.0,2.0,3.0,8.0,1180.0,0.0,47.6168,-122.301,1500.0,1948.0,21.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2800.0,12831.0,2.0,0.0,0.0,3.0,8.0,2800.0,0.0,47.7392,-121.966,2810.0,10235.0,13.0,0.0,0.0]},"out":{"variable":[536371.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1320.0,9675.0,1.5,0.0,0.0,4.0,7.0,1320.0,0.0,47.5695,-121.902,1160.0,9675.0,44.0,0.0,0.0]},"out":{"variable":[438346.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1380.0,4590.0,1.0,0.0,0.0,2.0,7.0,930.0,450.0,47.6841,-122.293,1320.0,4692.0,64.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1340.0,4000.0,1.5,0.0,0.0,4.0,7.0,1340.0,0.0,47.6652,-122.288,1510.0,4000.0,87.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1580.0,14398.0,1.0,0.0,0.0,3.0,7.0,1080.0,500.0,47.6328,-122.174,1650.0,14407.0,34.0,0.0,0.0]},"out":{"variable":[558463.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,920.0,43560.0,1.0,0.0,0.0,4.0,5.0,920.0,0.0,47.5245,-121.931,1530.0,11875.0,91.0,0.0,0.0]},"out":{"variable":[243300.83]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1720.0,37363.0,1.0,0.0,0.0,4.0,8.0,1350.0,370.0,47.6608,-122.035,2740.0,40635.0,41.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.0,2940.0,5763.0,1.0,0.0,0.0,5.0,8.0,1640.0,1300.0,47.5589,-122.295,2020.0,7320.0,59.0,0.0,0.0]},"out":{"variable":[727968.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1730.0,10396.0,1.0,0.0,0.0,3.0,7.0,1730.0,0.0,47.4497,-122.168,1510.0,10396.0,51.0,0.0,0.0]},"out":{"variable":[300988.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1320.0,11090.0,1.0,0.0,0.0,3.0,7.0,1320.0,0.0,47.7748,-122.304,1320.0,8319.0,59.0,0.0,0.0]},"out":{"variable":[341649.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2250.0,8076.0,2.0,0.0,0.0,3.0,8.0,2250.0,0.0,47.3667,-122.041,2180.0,7244.0,19.0,0.0,0.0]},"out":{"variable":[343304.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.75,2260.0,280962.0,2.0,0.0,2.0,3.0,9.0,1890.0,370.0,47.6359,-121.94,2860.0,219542.0,9.0,0.0,0.0]},"out":{"variable":[735392.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2210.0,14073.0,1.0,0.0,0.0,3.0,8.0,1630.0,580.0,47.4774,-122.142,2340.0,11340.0,37.0,0.0,0.0]},"out":{"variable":[416774.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,3800.0,9606.0,2.0,0.0,0.0,3.0,9.0,3800.0,0.0,47.7368,-122.208,3400.0,9677.0,6.0,0.0,0.0]},"out":{"variable":[1039781.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.0,1200.0,43560.0,1.0,0.0,0.0,3.0,5.0,1200.0,0.0,47.3375,-122.123,1400.0,54450.0,46.0,0.0,0.0]},"out":{"variable":[242656.47]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1380.0,5198.0,1.0,0.0,0.0,4.0,7.0,1380.0,0.0,47.5514,-122.357,1320.0,6827.0,33.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1480.0,8165.0,1.0,0.0,0.0,4.0,7.0,1480.0,0.0,47.3624,-122.079,1450.0,7939.0,30.0,0.0,0.0]},"out":{"variable":[256845.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1890.0,9646.0,1.0,0.0,0.0,3.0,8.0,1890.0,0.0,47.4838,-122.299,1580.0,9488.0,49.0,0.0,0.0]},"out":{"variable":[307947.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1260.0,11224.0,1.0,0.0,0.0,5.0,7.0,1260.0,0.0,47.7444,-122.321,1570.0,11052.0,67.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.5,4150.0,13232.0,2.0,0.0,0.0,3.0,11.0,4150.0,0.0,47.3417,-122.182,3840.0,15121.0,9.0,0.0,0.0]},"out":{"variable":[1042119.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.0,3070.0,8474.0,2.0,0.0,0.0,3.0,9.0,3070.0,0.0,47.6852,-122.184,3070.0,8527.0,3.0,0.0,0.0]},"out":{"variable":[837085.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2160.0,9540.0,2.0,0.0,0.0,3.0,8.0,2160.0,0.0,47.7668,-122.243,1720.0,12593.0,36.0,0.0,0.0]},"out":{"variable":[513083.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1280.0,10716.0,1.0,0.0,0.0,4.0,7.0,1280.0,0.0,47.4755,-122.145,1440.0,9870.0,45.0,0.0,0.0]},"out":{"variable":[243585.28]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2190.0,3746.0,2.0,0.0,0.0,3.0,8.0,2190.0,0.0,47.3896,-122.034,2200.0,3591.0,9.0,0.0,0.0]},"out":{"variable":[341472.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[1.0,1.0,930.0,7129.0,1.0,0.0,0.0,3.0,6.0,930.0,0.0,47.7234,-122.333,1300.0,8075.0,66.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,720.0,8040.0,1.0,0.0,0.0,3.0,6.0,720.0,0.0,47.4662,-122.359,2300.0,9500.0,71.0,0.0,0.0]},"out":{"variable":[251194.61]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1120.0,7250.0,1.0,0.0,0.0,4.0,7.0,1120.0,0.0,47.7143,-122.211,1340.0,7302.0,42.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1760.0,6150.0,1.5,0.0,0.0,3.0,7.0,1760.0,0.0,47.3871,-122.224,1760.0,8276.0,63.0,0.0,0.0]},"out":{"variable":[289684.22]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,1920.0,8259.0,2.0,0.0,0.0,4.0,8.0,1920.0,0.0,47.5616,-122.088,2030.0,8910.0,35.0,0.0,0.0]},"out":{"variable":[553463.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.75,2590.0,6120.0,2.0,0.0,0.0,3.0,8.0,2590.0,0.0,47.6667,-122.327,1390.0,3060.0,105.0,0.0,0.0]},"out":{"variable":[715530.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.5,1650.0,2710.0,2.0,0.0,2.0,3.0,8.0,1650.0,0.0,47.5173,-121.878,1760.0,2992.0,6.0,0.0,0.0]},"out":{"variable":[354512.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,1280.0,8710.0,1.0,0.0,0.0,3.0,7.0,1280.0,0.0,47.4472,-122.16,1520.0,9375.0,47.0,0.0,0.0]},"out":{"variable":[245070.97]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1420.0,11040.0,1.0,0.0,0.0,4.0,7.0,1420.0,0.0,47.5969,-122.14,1530.0,8208.0,54.0,0.0,0.0]},"out":{"variable":[453195.78]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2330.0,33750.0,2.0,0.0,0.0,3.0,9.0,2330.0,0.0,47.4787,-121.723,2270.0,35000.0,32.0,1.0,18.0]},"out":{"variable":[521639.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1790.0,12000.0,1.0,0.0,0.0,3.0,7.0,1040.0,750.0,47.3945,-122.313,1840.0,12000.0,54.0,0.0,0.0]},"out":{"variable":[291872.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.0,3520.0,36558.0,2.0,0.0,0.0,4.0,8.0,2100.0,1420.0,47.4658,-122.007,3000.0,36558.0,29.0,0.0,0.0]},"out":{"variable":[475971.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2090.0,7416.0,1.0,0.0,0.0,4.0,7.0,1050.0,1040.0,47.4107,-122.179,1710.0,7527.0,44.0,0.0,0.0]},"out":{"variable":[349619.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1760.0,6300.0,1.0,0.0,0.0,3.0,7.0,1060.0,700.0,47.5003,-122.26,1340.0,7300.0,52.0,0.0,0.0]},"out":{"variable":[300446.72]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,1610.0,11201.0,1.0,0.0,0.0,5.0,7.0,1020.0,590.0,47.7024,-122.198,1610.0,9000.0,32.0,0.0,0.0]},"out":{"variable":[465401.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2630.0,48706.0,2.0,0.0,0.0,3.0,8.0,2630.0,0.0,47.775,-122.125,2680.0,48706.0,28.0,0.0,0.0]},"out":{"variable":[536371.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1570.0,10824.0,2.0,0.0,0.0,3.0,7.0,1570.0,0.0,47.54,-122.275,1530.0,8125.0,107.0,0.0,0.0]},"out":{"variable":[544392.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.25,2910.0,9454.0,1.0,0.0,1.0,3.0,8.0,1910.0,1000.0,47.5871,-122.173,2400.0,10690.0,42.0,0.0,0.0]},"out":{"variable":[711565.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2120.0,7620.0,2.0,0.0,0.0,3.0,8.0,2120.0,0.0,47.457,-122.346,1820.0,7620.0,43.0,1.0,31.0]},"out":{"variable":[404040.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,2320.0,9420.0,1.0,0.0,0.0,5.0,7.0,2320.0,0.0,47.5133,-122.196,2030.0,9420.0,62.0,0.0,0.0]},"out":{"variable":[413013.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1010.0,1546.0,2.0,0.0,0.0,3.0,8.0,1010.0,0.0,47.5998,-122.311,1010.0,1517.0,44.0,1.0,43.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.75,2730.0,5820.0,2.0,0.0,0.0,3.0,8.0,2730.0,0.0,47.4856,-122.154,2730.0,5700.0,0.0,0.0,0.0]},"out":{"variable":[457449.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,870.0,7227.0,1.0,0.0,0.0,3.0,7.0,870.0,0.0,47.7288,-122.331,1250.0,7252.0,66.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,820.0,681.0,3.0,0.0,0.0,3.0,8.0,820.0,0.0,47.6619,-122.352,820.0,1156.0,8.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2450.0,4187.0,2.0,0.0,2.0,3.0,8.0,2450.0,0.0,47.5471,-122.016,2320.0,4187.0,4.0,0.0,0.0]},"out":{"variable":[705013.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,3380.0,7074.0,2.0,0.0,0.0,3.0,8.0,2200.0,1180.0,47.7462,-121.978,2060.0,6548.0,15.0,0.0,0.0]},"out":{"variable":[597475.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1050.0,6250.0,1.0,0.0,0.0,4.0,6.0,840.0,210.0,47.5024,-122.333,1310.0,12500.0,72.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,770.0,4800.0,1.0,0.0,0.0,3.0,7.0,770.0,0.0,47.527,-122.383,1390.0,4800.0,71.0,0.0,0.0]},"out":{"variable":[313906.47]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1600.0,7350.0,1.0,0.0,0.0,4.0,7.0,1600.0,0.0,47.6977,-122.126,1600.0,7200.0,35.0,0.0,0.0]},"out":{"variable":[469038.03]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1930.0,13350.0,1.0,0.0,0.0,3.0,8.0,1930.0,0.0,47.3317,-122.365,2270.0,13350.0,48.0,0.0,0.0]},"out":{"variable":[306159.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1330.0,13102.0,1.0,0.0,0.0,3.0,7.0,1330.0,0.0,47.3172,-122.322,1270.0,11475.0,46.0,0.0,0.0]},"out":{"variable":[244380.27]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2640.0,4000.0,2.0,0.0,0.0,5.0,8.0,1730.0,910.0,47.6727,-122.297,1530.0,3740.0,89.0,0.0,0.0]},"out":{"variable":[718445.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,850.0,2340.0,1.0,0.0,0.0,3.0,7.0,850.0,0.0,47.6707,-122.328,1300.0,3000.0,92.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2070.0,7995.0,1.0,0.0,0.0,3.0,7.0,1350.0,720.0,47.403,-122.175,1620.0,6799.0,28.0,0.0,0.0]},"out":{"variable":[349100.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1680.0,4226.0,2.0,0.0,0.0,3.0,8.0,1680.0,0.0,47.3684,-122.123,1800.0,5559.0,12.0,0.0,0.0]},"out":{"variable":[289727.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.0,1350.0,2560.0,1.0,0.0,0.0,4.0,8.0,1350.0,0.0,47.6338,-122.106,1800.0,2560.0,41.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,3010.0,7215.0,2.0,0.0,0.0,3.0,9.0,3010.0,0.0,47.6952,-122.178,3010.0,7215.0,0.0,0.0,0.0]},"out":{"variable":[795841.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1260.0,10350.0,1.0,0.0,0.0,3.0,7.0,1260.0,0.0,47.6357,-122.123,1800.0,10350.0,55.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.75,1930.0,5570.0,1.0,0.0,0.0,3.0,8.0,1930.0,0.0,47.7173,-122.034,1810.0,5178.0,9.0,0.0,0.0]},"out":{"variable":[444885.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1850.0,7850.0,2.0,0.0,0.0,3.0,8.0,1850.0,0.0,47.6914,-122.103,1830.0,8140.0,29.0,0.0,0.0]},"out":{"variable":[550183.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1100.0,11824.0,1.0,0.0,0.0,4.0,7.0,1100.0,0.0,47.5704,-122.141,1380.0,11796.0,60.0,0.0,0.0]},"out":{"variable":[438346.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2910.0,6334.0,2.0,0.0,0.0,3.0,8.0,2910.0,0.0,47.4826,-121.771,2790.0,6352.0,1.0,0.0,0.0]},"out":{"variable":[463718.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2060.0,7080.0,2.0,0.0,0.0,3.0,9.0,1800.0,260.0,47.6455,-122.409,3070.0,7500.0,75.0,0.0,0.0]},"out":{"variable":[904204.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2700.0,37011.0,2.0,0.0,0.0,3.0,9.0,2700.0,0.0,47.3496,-122.088,2700.0,37457.0,30.0,0.0,0.0]},"out":{"variable":[490051.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,1990.0,4740.0,1.0,0.0,0.0,3.0,7.0,1080.0,910.0,47.6112,-122.303,1560.0,2370.0,89.0,0.0,0.0]},"out":{"variable":[581002.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.5,4300.0,70407.0,2.0,0.0,0.0,3.0,10.0,2710.0,1590.0,47.4472,-122.092,3520.0,26727.0,22.0,0.0,0.0]},"out":{"variable":[1115274.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1680.0,81893.0,1.0,0.0,0.0,3.0,7.0,1680.0,0.0,47.3248,-122.179,2480.0,38637.0,23.0,0.0,0.0]},"out":{"variable":[290987.34]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2040.0,12000.0,1.0,0.0,0.0,4.0,7.0,1300.0,740.0,47.7362,-122.241,1930.0,12000.0,52.0,0.0,0.0]},"out":{"variable":[477541.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2190.0,125452.0,1.0,0.0,2.0,3.0,9.0,2190.0,0.0,47.2703,-122.069,3000.0,125017.0,46.0,0.0,0.0]},"out":{"variable":[656707.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2220.0,11646.0,1.0,0.0,0.0,3.0,7.0,1270.0,950.0,47.7762,-122.27,1490.0,10003.0,64.0,0.0,0.0]},"out":{"variable":[513264.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1680.0,11193.0,2.0,0.0,0.0,3.0,8.0,1680.0,0.0,47.4482,-122.125,2080.0,8084.0,30.0,0.0,0.0]},"out":{"variable":[311515.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.75,3460.0,7977.0,2.0,0.0,0.0,3.0,9.0,3460.0,0.0,47.5908,-122.062,3390.0,6630.0,3.0,0.0,0.0]},"out":{"variable":[879092.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2717.0,4513.0,2.0,0.0,0.0,3.0,8.0,2717.0,0.0,47.3373,-122.266,2550.0,4841.0,9.0,0.0,0.0]},"out":{"variable":[371040.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,0.75,1020.0,1076.0,2.0,0.0,0.0,3.0,7.0,1020.0,0.0,47.5941,-122.299,1020.0,1357.0,6.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1490.0,4522.0,2.0,0.0,0.0,3.0,7.0,1490.0,0.0,47.7611,-122.233,1580.0,4667.0,5.0,0.0,0.0]},"out":{"variable":[417980.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,840.0,7020.0,1.5,0.0,0.0,4.0,7.0,840.0,0.0,47.5513,-122.394,1310.0,7072.0,72.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1970.0,4590.0,2.5,0.0,0.0,3.0,7.0,1970.0,0.0,47.666,-122.332,1900.0,4590.0,105.0,0.0,0.0]},"out":{"variable":[572710.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,820.0,3700.0,1.0,0.0,0.0,5.0,7.0,820.0,0.0,47.588,-122.251,1750.0,9000.0,46.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2701.0,4500.0,2.0,0.0,0.0,3.0,9.0,2701.0,0.0,47.2586,-122.194,2570.0,4800.0,0.0,0.0,0.0]},"out":{"variable":[467855.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1340.0,8867.0,2.0,0.0,0.0,3.0,8.0,1340.0,0.0,47.724,-122.327,1630.0,7287.0,30.0,0.0,0.0]},"out":{"variable":[342604.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2420.0,10200.0,1.0,0.0,0.0,3.0,8.0,1220.0,1200.0,47.72,-122.236,2240.0,9750.0,53.0,0.0,0.0]},"out":{"variable":[538316.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1340.0,7788.0,1.0,0.0,2.0,3.0,7.0,1340.0,0.0,47.5094,-122.244,2550.0,7788.0,67.0,0.0,0.0]},"out":{"variable":[278475.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,940.0,9839.0,1.0,0.0,0.0,3.0,6.0,940.0,0.0,47.5379,-122.386,1330.0,8740.0,104.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,4.0,2080.0,2250.0,3.0,0.0,4.0,3.0,8.0,2080.0,0.0,47.6598,-122.355,2080.0,2250.0,17.0,0.0,0.0]},"out":{"variable":[656923.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1810.0,8232.0,1.0,0.0,0.0,3.0,8.0,1810.0,0.0,47.3195,-122.273,2260.0,8491.0,27.0,0.0,0.0]},"out":{"variable":[291239.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1030.0,3000.0,1.0,0.0,0.0,3.0,7.0,830.0,200.0,47.6813,-122.317,1830.0,3000.0,90.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,1170.0,5248.0,1.0,0.0,0.0,5.0,6.0,1170.0,0.0,47.5318,-122.374,1170.0,5120.0,74.0,0.0,0.0]},"out":{"variable":[260266.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2830.0,3750.0,3.0,0.0,0.0,3.0,10.0,2830.0,0.0,47.6799,-122.385,1780.0,5000.0,0.0,0.0,0.0]},"out":{"variable":[937359.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,3310.0,8540.0,1.0,0.0,4.0,4.0,9.0,1660.0,1650.0,47.5603,-122.158,3450.0,9566.0,41.0,0.0,0.0]},"out":{"variable":[921561.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[1.0,2.0,3000.0,204732.0,2.5,0.0,2.0,3.0,8.0,3000.0,0.0,47.6331,-121.945,2330.0,213008.0,35.0,0.0,0.0]},"out":{"variable":[716558.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,900.0,5000.0,1.0,0.0,0.0,3.0,7.0,900.0,0.0,47.6883,-122.395,1280.0,5000.0,70.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2580.0,7344.0,2.0,0.0,0.0,3.0,8.0,2580.0,0.0,47.5647,-122.09,2390.0,7507.0,37.0,0.0,0.0]},"out":{"variable":[701940.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1990.0,4040.0,1.5,0.0,0.0,5.0,8.0,1390.0,600.0,47.6867,-122.354,1180.0,3030.0,88.0,0.0,0.0]},"out":{"variable":[581002.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1440.0,1102.0,3.0,0.0,0.0,3.0,8.0,1440.0,0.0,47.6995,-122.346,1440.0,1434.0,6.0,0.0,0.0]},"out":{"variable":[408956.34]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,5010.0,49222.0,2.0,0.0,0.0,5.0,9.0,3710.0,1300.0,47.5489,-122.092,3140.0,54014.0,36.0,0.0,0.0]},"out":{"variable":[1092274.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1770.0,5750.0,2.0,0.0,0.0,3.0,7.0,1770.0,0.0,47.5621,-122.394,970.0,5750.0,67.0,0.0,0.0]},"out":{"variable":[544392.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,1790.0,7203.0,1.0,0.0,0.0,4.0,7.0,1110.0,680.0,47.7709,-122.294,2270.0,9000.0,41.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,2470.0,4760.0,1.5,0.0,0.0,5.0,9.0,1890.0,580.0,47.6331,-122.31,2470.0,4760.0,108.0,0.0,0.0]},"out":{"variable":[957189.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2060.0,5721.0,1.0,0.0,2.0,3.0,9.0,1140.0,920.0,47.5268,-122.388,2060.0,8124.0,50.0,0.0,0.0]},"out":{"variable":[764936.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.25,1240.0,720.0,2.0,0.0,0.0,3.0,7.0,1150.0,90.0,47.5322,-122.072,1260.0,810.0,7.0,0.0,0.0]},"out":{"variable":[258321.64]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,4.0,4100.0,8120.0,2.0,0.0,0.0,3.0,9.0,4100.0,0.0,47.6917,-122.02,4100.0,7625.0,3.0,0.0,0.0]},"out":{"variable":[987974.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1680.0,7910.0,1.0,0.0,0.0,3.0,7.0,1680.0,0.0,47.5085,-122.385,1330.0,7910.0,66.0,0.0,0.0]},"out":{"variable":[323856.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1400.0,6600.0,1.0,0.0,0.0,3.0,6.0,1280.0,120.0,47.4845,-122.331,1730.0,6600.0,60.0,0.0,0.0]},"out":{"variable":[259955.61]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.75,4410.0,8112.0,3.0,0.0,4.0,3.0,11.0,3570.0,840.0,47.5888,-122.392,2770.0,5750.0,12.0,0.0,0.0]},"out":{"variable":[1967344.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.75,3710.0,34412.0,2.0,0.0,0.0,3.0,10.0,2910.0,800.0,47.5888,-122.04,2390.0,34412.0,36.0,0.0,0.0]},"out":{"variable":[924823.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3450.0,35100.0,2.0,0.0,0.0,3.0,10.0,3450.0,0.0,47.7302,-122.106,3110.0,35894.0,27.0,0.0,0.0]},"out":{"variable":[921695.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1810.0,5080.0,1.0,0.0,0.0,3.0,7.0,1030.0,780.0,47.6819,-122.287,1780.0,7620.0,57.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.75,1850.0,16960.0,1.0,0.0,2.0,4.0,8.0,1850.0,0.0,47.7128,-122.365,2470.0,13761.0,61.0,0.0,0.0]},"out":{"variable":[440821.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1570.0,6300.0,1.0,0.0,0.0,3.0,7.0,820.0,750.0,47.5565,-122.275,1510.0,4281.0,61.0,1.0,52.0]},"out":{"variable":[544392.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2140.0,13260.0,1.0,0.0,0.0,3.0,7.0,1240.0,900.0,47.5074,-122.353,1640.0,13260.0,66.0,0.0,0.0]},"out":{"variable":[388243.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.0,4750.0,21701.0,1.5,0.0,0.0,5.0,11.0,4750.0,0.0,47.6454,-122.218,3120.0,18551.0,38.0,0.0,0.0]},"out":{"variable":[2002393.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1270.0,2509.0,2.0,0.0,0.0,3.0,8.0,1270.0,0.0,47.5357,-122.365,1420.0,2206.0,10.0,0.0,0.0]},"out":{"variable":[431992.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2030.0,4867.0,2.0,0.0,0.0,3.0,7.0,2030.0,0.0,47.3747,-122.128,2030.0,5000.0,12.0,0.0,0.0]},"out":{"variable":[327625.28]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1520.0,4170.0,2.0,0.0,0.0,3.0,7.0,1520.0,0.0,47.3842,-122.04,1560.0,4237.0,10.0,0.0,0.0]},"out":{"variable":[261886.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,1570.0,499571.0,1.0,0.0,3.0,4.0,7.0,1570.0,0.0,47.1808,-122.023,1700.0,181708.0,42.0,0.0,0.0]},"out":{"variable":[303082.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.25,2500.0,7620.0,1.0,0.0,3.0,3.0,7.0,1250.0,1250.0,47.5298,-122.344,2020.0,7620.0,53.0,0.0,0.0]},"out":{"variable":[474010.38]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.25,3070.0,64033.0,1.0,0.0,0.0,3.0,9.0,2730.0,340.0,47.3238,-122.292,1560.0,28260.0,31.0,0.0,0.0]},"out":{"variable":[536388.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.5,3060.0,8862.0,2.0,0.0,0.0,3.0,8.0,3060.0,0.0,47.5322,-122.185,2680.0,8398.0,26.0,0.0,0.0]},"out":{"variable":[481600.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1250.0,8400.0,1.0,0.0,0.0,3.0,7.0,960.0,290.0,47.7505,-122.315,1560.0,8400.0,64.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1670.0,8800.0,1.0,0.0,0.0,4.0,7.0,1150.0,520.0,47.6096,-122.132,2020.0,8250.0,53.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.25,4115.0,7910.0,2.0,0.0,0.0,3.0,9.0,4115.0,0.0,47.6847,-122.016,3950.0,6765.0,0.0,0.0,0.0]},"out":{"variable":[988481.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.0,2680.0,15438.0,2.0,0.0,2.0,3.0,8.0,2680.0,0.0,47.6109,-122.226,4480.0,14406.0,113.0,1.0,54.0]},"out":{"variable":[747075.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1150.0,6600.0,1.5,0.0,0.0,4.0,6.0,1150.0,0.0,47.6709,-122.185,1530.0,6600.0,44.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1650.0,1180.0,3.0,0.0,0.0,3.0,8.0,1650.0,0.0,47.6636,-122.319,1720.0,1960.0,0.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1850.0,2575.0,2.0,0.0,0.0,3.0,9.0,1850.0,0.0,47.5525,-122.273,1080.0,4120.0,1.0,0.0,0.0]},"out":{"variable":[723935.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,1560.0,3840.0,1.0,0.0,0.0,4.0,6.0,960.0,600.0,47.6882,-122.365,1560.0,4800.0,90.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.25,5790.0,13726.0,2.0,0.0,3.0,3.0,10.0,4430.0,1360.0,47.5388,-122.114,5790.0,13726.0,0.0,0.0,0.0]},"out":{"variable":[1189654.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2070.0,2992.0,2.0,0.0,0.0,3.0,8.0,2070.0,0.0,47.4496,-122.12,1900.0,2957.0,13.0,0.0,0.0]},"out":{"variable":[400536.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,980.0,6380.0,1.0,0.0,0.0,3.0,7.0,760.0,220.0,47.692,-122.308,1390.0,6380.0,73.0,0.0,0.0]},"out":{"variable":[444933.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,1.75,2110.0,5000.0,1.5,0.0,0.0,4.0,7.0,1250.0,860.0,47.6745,-122.287,1720.0,5000.0,69.0,0.0,0.0]},"out":{"variable":[656396.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,740.0,6250.0,1.0,0.0,0.0,3.0,6.0,740.0,0.0,47.506,-122.335,980.0,6957.0,72.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1140.0,5258.0,1.5,0.0,0.0,3.0,6.0,1140.0,0.0,47.5122,-122.383,1140.0,5280.0,103.0,0.0,0.0]},"out":{"variable":[312719.84]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,2298.0,10140.0,1.0,0.0,0.0,3.0,7.0,2298.0,0.0,47.6909,-122.083,2580.0,24724.0,46.0,0.0,0.0]},"out":{"variable":[683869.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,890.0,9465.0,1.0,0.0,0.0,3.0,6.0,890.0,0.0,47.4388,-122.328,1590.0,9147.0,57.0,0.0,0.0]},"out":{"variable":[240212.23]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1950.0,5451.0,2.0,0.0,0.0,3.0,7.0,1950.0,0.0,47.4341,-122.144,2240.0,6221.0,10.0,0.0,0.0]},"out":{"variable":[350049.28]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,830.0,26329.0,1.0,1.0,3.0,4.0,6.0,830.0,0.0,47.4012,-122.425,2030.0,27338.0,86.0,0.0,0.0]},"out":{"variable":[379398.38]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.0,3570.0,6250.0,2.0,0.0,2.0,3.0,10.0,2710.0,860.0,47.5624,-122.399,2550.0,7596.0,30.0,0.0,0.0]},"out":{"variable":[1124493.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.75,3170.0,34850.0,1.0,0.0,0.0,5.0,9.0,3170.0,0.0,47.6611,-122.169,3920.0,36740.0,58.0,0.0,0.0]},"out":{"variable":[1227073.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,3260.0,19542.0,1.0,0.0,0.0,4.0,10.0,2170.0,1090.0,47.6245,-122.236,3480.0,19863.0,46.0,0.0,0.0]},"out":{"variable":[1364650.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.75,1210.0,4141.0,1.0,0.0,0.0,4.0,7.0,910.0,300.0,47.686,-122.382,1310.0,4141.0,72.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,4020.0,18745.0,2.0,0.0,4.0,4.0,10.0,2830.0,1190.0,47.6042,-122.21,3150.0,20897.0,26.0,0.0,0.0]},"out":{"variable":[1322835.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1930.0,10183.0,1.0,0.0,0.0,4.0,8.0,1480.0,450.0,47.5624,-122.135,2320.0,10000.0,39.0,0.0,0.0]},"out":{"variable":[559453.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1240.0,5758.0,1.5,0.0,0.0,4.0,6.0,960.0,280.0,47.5675,-122.396,1460.0,5750.0,105.0,0.0,0.0]},"out":{"variable":[437002.72]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1730.0,4102.0,3.0,1.0,4.0,3.0,8.0,1730.0,0.0,47.645,-122.084,2340.0,16994.0,19.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2160.0,10987.0,1.0,0.0,0.0,4.0,8.0,1440.0,720.0,47.6333,-122.034,1280.0,11617.0,34.0,1.0,22.0]},"out":{"variable":[673288.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1770.0,30689.0,1.0,0.0,4.0,3.0,9.0,1770.0,0.0,47.7648,-122.37,2650.0,30280.0,62.0,0.0,0.0]},"out":{"variable":[836230.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.5,3001.0,5710.0,2.0,0.0,0.0,3.0,8.0,3001.0,0.0,47.3727,-122.177,2340.0,5980.0,8.0,0.0,0.0]},"out":{"variable":[379076.34]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,962.0,1992.0,2.0,0.0,0.0,3.0,7.0,962.0,0.0,47.6911,-122.313,1130.0,1992.0,3.0,0.0,0.0]},"out":{"variable":[446768.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2350.0,5100.0,2.0,0.0,0.0,3.0,8.0,2350.0,0.0,47.3512,-122.008,2350.0,5363.0,11.0,0.0,0.0]},"out":{"variable":[349102.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1750.0,14400.0,1.0,0.0,0.0,4.0,7.0,1750.0,0.0,47.4535,-122.361,2030.0,14400.0,63.0,0.0,0.0]},"out":{"variable":[313096.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1620.0,1171.0,3.0,0.0,4.0,3.0,8.0,1470.0,150.0,47.6681,-122.355,1620.0,1505.0,6.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1410.0,5101.0,1.5,0.0,0.0,3.0,8.0,1410.0,0.0,47.6872,-122.333,1410.0,4224.0,87.0,0.0,0.0]},"out":{"variable":[450928.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,990.0,8140.0,1.0,0.0,0.0,1.0,6.0,990.0,0.0,47.5828,-122.382,2150.0,5000.0,105.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2690.0,7000.0,2.0,0.0,0.0,5.0,7.0,1840.0,850.0,47.6784,-122.277,1800.0,6435.0,71.0,0.0,0.0]},"out":{"variable":[718445.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2240.0,4616.0,2.0,0.0,0.0,3.0,7.0,1840.0,400.0,47.5118,-122.194,2260.0,5200.0,14.0,0.0,0.0]},"out":{"variable":[407385.34]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,810.0,4080.0,1.0,0.0,0.0,4.0,6.0,810.0,0.0,47.5337,-122.379,1400.0,4080.0,73.0,0.0,0.0]},"out":{"variable":[375011.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1240.0,10956.0,1.0,0.0,0.0,3.0,6.0,1240.0,0.0,47.3705,-122.15,1240.0,8137.0,27.0,0.0,0.0]},"out":{"variable":[243063.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2550.0,7555.0,2.0,0.0,0.0,3.0,8.0,2550.0,0.0,47.2614,-122.29,2550.0,6800.0,13.0,0.0,0.0]},"out":{"variable":[353912.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,770.0,6731.0,1.0,0.0,0.0,4.0,6.0,770.0,0.0,47.7505,-122.312,1120.0,9212.0,72.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.5,3450.0,7832.0,2.0,0.0,0.0,3.0,10.0,3450.0,0.0,47.5637,-122.123,3220.0,8567.0,7.0,0.0,0.0]},"out":{"variable":[921695.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1660.0,2890.0,2.0,0.0,0.0,3.0,7.0,1660.0,0.0,47.5434,-122.293,1540.0,2890.0,14.0,0.0,0.0]},"out":{"variable":[544392.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,1450.0,5456.0,1.0,0.0,0.0,5.0,7.0,1450.0,0.0,47.5442,-122.297,980.0,6100.0,63.0,0.0,0.0]},"out":{"variable":[451058.16]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1170.0,6543.0,1.0,0.0,0.0,3.0,7.0,1170.0,0.0,47.537,-122.385,1550.0,7225.0,101.0,0.0,0.0]},"out":{"variable":[434534.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2750.0,11830.0,2.0,0.0,0.0,3.0,9.0,2750.0,0.0,47.4698,-122.121,2310.0,11830.0,0.0,0.0,0.0]},"out":{"variable":[515844.28]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,2060.0,2900.0,1.5,0.0,0.0,5.0,8.0,1330.0,730.0,47.5897,-122.292,1910.0,3900.0,84.0,0.0,0.0]},"out":{"variable":[630865.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2350.0,20820.0,1.0,0.0,0.0,4.0,8.0,1800.0,550.0,47.6095,-122.059,2040.0,10800.0,36.0,0.0,0.0]},"out":{"variable":[700294.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1160.0,3700.0,1.5,0.0,0.0,3.0,7.0,1160.0,0.0,47.5651,-122.359,1340.0,3750.0,105.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,2070.0,9600.0,1.0,0.0,1.0,3.0,7.0,1590.0,480.0,47.616,-122.239,3000.0,16215.0,68.0,0.0,0.0]},"out":{"variable":[636559.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2490.0,5812.0,2.0,0.0,0.0,3.0,8.0,2490.0,0.0,47.3875,-122.155,2690.0,6012.0,14.0,0.0,0.0]},"out":{"variable":[352272.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,980.0,3800.0,1.0,0.0,0.0,3.0,7.0,980.0,0.0,47.6903,-122.34,1520.0,5010.0,89.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1130.0,2640.0,1.0,0.0,0.0,4.0,8.0,1130.0,0.0,47.6438,-122.357,1680.0,3200.0,87.0,0.0,0.0]},"out":{"variable":[449699.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,0.75,1240.0,4000.0,1.0,0.0,0.0,4.0,7.0,1240.0,0.0,47.6239,-122.297,1460.0,4000.0,47.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1400.0,2036.0,2.0,0.0,0.0,3.0,7.0,1400.0,0.0,47.5516,-122.382,1500.0,2036.0,11.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1530.0,8500.0,1.0,0.0,0.0,5.0,7.0,1030.0,500.0,47.3592,-122.046,1850.0,8140.0,19.0,0.0,0.0]},"out":{"variable":[281411.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1830.0,8133.0,1.0,0.0,0.0,3.0,8.0,1390.0,440.0,47.7478,-122.247,2310.0,11522.0,19.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2570.0,10431.0,2.0,0.0,0.0,3.0,9.0,2570.0,0.0,47.4188,-122.213,2590.0,10078.0,25.0,0.0,0.0]},"out":{"variable":[482485.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1760.0,4125.0,1.5,0.0,3.0,4.0,7.0,1760.0,0.0,47.6748,-122.352,1760.0,4000.0,87.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2990.0,5669.0,2.0,0.0,0.0,3.0,8.0,2990.0,0.0,47.6119,-122.011,3110.0,5058.0,11.0,0.0,0.0]},"out":{"variable":[728707.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.0,2480.0,5500.0,2.0,0.0,3.0,3.0,10.0,1730.0,750.0,47.6466,-122.404,2950.0,5670.0,64.0,1.0,55.0]},"out":{"variable":[1100884.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1240.0,8410.0,1.0,0.0,0.0,5.0,6.0,1240.0,0.0,47.753,-122.328,1630.0,8410.0,67.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.5,2720.0,5000.0,1.5,0.0,0.0,4.0,7.0,1530.0,1190.0,47.6827,-122.376,1210.0,5000.0,75.0,0.0,0.0]},"out":{"variable":[718445.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3880.0,14550.0,2.0,0.0,0.0,3.0,10.0,3880.0,0.0,47.6378,-122.04,3240.0,14045.0,27.0,0.0,0.0]},"out":{"variable":[946325.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2460.0,38794.0,2.0,0.0,0.0,3.0,9.0,2460.0,0.0,47.7602,-122.022,2470.0,51400.0,16.0,0.0,0.0]},"out":{"variable":[721143.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,920.0,4095.0,1.0,0.0,0.0,4.0,6.0,920.0,0.0,47.5484,-122.278,1460.0,4945.0,100.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.75,2330.0,7280.0,1.0,0.0,0.0,3.0,7.0,1450.0,880.0,47.4282,-122.28,1830.0,12178.0,33.0,0.0,0.0]},"out":{"variable":[392393.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,1900.0,8160.0,1.0,0.0,0.0,3.0,7.0,1900.0,0.0,47.2114,-121.986,1280.0,6532.0,40.0,0.0,0.0]},"out":{"variable":[287576.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.5,3820.0,17745.0,2.0,0.0,2.0,3.0,8.0,2440.0,1380.0,47.557,-122.295,2520.0,9640.0,59.0,0.0,0.0]},"out":{"variable":[785664.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.0,2840.0,7199.0,1.0,0.0,0.0,3.0,7.0,1710.0,1130.0,47.5065,-122.275,2210.0,10800.0,11.0,0.0,0.0]},"out":{"variable":[432908.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1580.0,507038.0,1.0,0.0,2.0,4.0,7.0,1580.0,0.0,47.2303,-121.936,2040.0,210394.0,29.0,0.0,0.0]},"out":{"variable":[311536.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2650.0,18295.0,2.0,0.0,0.0,3.0,8.0,2650.0,0.0,47.6075,-122.154,2230.0,19856.0,28.0,0.0,0.0]},"out":{"variable":[706407.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1260.0,8092.0,1.0,0.0,0.0,3.0,7.0,1260.0,0.0,47.3635,-122.054,1950.0,8092.0,28.0,0.0,0.0]},"out":{"variable":[253958.77]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1960.0,8136.0,1.0,0.0,0.0,3.0,7.0,980.0,980.0,47.5208,-122.364,1070.0,7480.0,66.0,0.0,0.0]},"out":{"variable":[365436.22]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2210.0,7620.0,2.0,0.0,0.0,3.0,8.0,2210.0,0.0,47.6938,-122.13,1920.0,7440.0,20.0,0.0,0.0]},"out":{"variable":[677870.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.25,3160.0,10587.0,1.0,0.0,0.0,5.0,7.0,2190.0,970.0,47.7238,-122.165,2200.0,7761.0,55.0,0.0,0.0]},"out":{"variable":[573403.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2280.0,7500.0,1.0,0.0,0.0,4.0,7.0,1140.0,1140.0,47.4182,-122.332,1660.0,8000.0,51.0,0.0,0.0]},"out":{"variable":[380461.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2870.0,6658.0,2.0,0.0,0.0,3.0,8.0,2870.0,0.0,47.5394,-121.878,2770.0,6658.0,11.0,0.0,0.0]},"out":{"variable":[713358.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.0,2570.0,4000.0,2.0,0.0,0.0,3.0,8.0,1750.0,820.0,47.6743,-122.313,1970.0,4000.0,105.0,1.0,105.0]},"out":{"variable":[713978.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1220.0,3000.0,1.5,0.0,0.0,3.0,6.0,1220.0,0.0,47.6506,-122.346,1350.0,3000.0,114.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1140.0,1069.0,3.0,0.0,0.0,3.0,8.0,1140.0,0.0,47.6907,-122.342,1230.0,1276.0,10.0,0.0,0.0]},"out":{"variable":[446768.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1700.0,6356.0,1.5,0.0,0.0,3.0,7.0,1700.0,0.0,47.5677,-122.281,2080.0,6000.0,107.0,0.0,0.0]},"out":{"variable":[548006.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1280.0,4366.0,2.0,0.0,0.0,4.0,6.0,1280.0,0.0,47.335,-122.215,1280.0,4366.0,29.0,0.0,0.0]},"out":{"variable":[243063.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.75,1465.0,972.0,2.0,0.0,0.0,3.0,7.0,1050.0,415.0,47.621,-122.298,1480.0,1430.0,8.0,0.0,0.0]},"out":{"variable":[498579.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[7.0,3.25,4340.0,8521.0,2.0,0.0,0.0,3.0,7.0,2550.0,1790.0,47.52,-122.338,1890.0,8951.0,28.0,0.0,0.0]},"out":{"variable":[474651.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2640.0,3750.0,2.0,0.0,0.0,5.0,7.0,1840.0,800.0,47.6783,-122.363,1690.0,5000.0,103.0,0.0,0.0]},"out":{"variable":[718445.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1397.0,18000.0,1.0,0.0,0.0,3.0,7.0,1397.0,0.0,47.3388,-122.166,1950.0,31294.0,49.0,1.0,49.0]},"out":{"variable":[261201.19]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1590.0,87120.0,1.0,0.0,3.0,3.0,8.0,1590.0,0.0,47.2241,-122.072,2780.0,183161.0,16.0,0.0,0.0]},"out":{"variable":[318011.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,4.0,4660.0,9900.0,2.0,0.0,2.0,4.0,9.0,2600.0,2060.0,47.5135,-122.2,3380.0,9900.0,35.0,0.0,0.0]},"out":{"variable":[1058105.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.25,1260.0,1312.0,3.0,0.0,0.0,3.0,8.0,1260.0,0.0,47.6538,-122.356,1300.0,1312.0,7.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,930.0,6098.0,1.0,0.0,0.0,4.0,6.0,930.0,0.0,47.5289,-122.03,1730.0,9000.0,95.0,0.0,0.0]},"out":{"variable":[246901.17]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[1.0,1.0,1230.0,3774.0,1.0,0.0,0.0,4.0,6.0,830.0,400.0,47.6886,-122.354,1300.0,3774.0,90.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1470.0,1703.0,2.0,0.0,0.0,3.0,8.0,1470.0,0.0,47.5478,-121.999,1380.0,1107.0,9.0,0.0,0.0]},"out":{"variable":[504122.38]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2120.0,5277.0,2.0,0.0,0.0,3.0,7.0,2120.0,0.0,47.6811,-122.034,2370.0,5257.0,10.0,0.0,0.0]},"out":{"variable":[657905.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1780.0,2778.0,2.0,0.0,0.0,3.0,8.0,1530.0,250.0,47.5487,-122.372,1380.0,1998.0,7.0,0.0,0.0]},"out":{"variable":[544392.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1860.0,3840.0,1.5,0.0,0.0,3.0,7.0,1170.0,690.0,47.6886,-122.359,1400.0,3840.0,87.0,1.0,86.0]},"out":{"variable":[560013.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,1700.0,7495.0,1.0,0.0,0.0,4.0,7.0,1200.0,500.0,47.7589,-122.354,1650.0,7495.0,51.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.5,3770.0,8501.0,2.0,0.0,0.0,3.0,10.0,3770.0,0.0,47.6744,-122.196,1520.0,9660.0,6.0,0.0,0.0]},"out":{"variable":[1169643.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1740.0,30886.0,2.0,0.0,0.0,3.0,8.0,1740.0,0.0,47.46,-121.707,1740.0,39133.0,22.0,0.0,0.0]},"out":{"variable":[306037.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,980.0,9135.0,1.0,0.0,0.0,3.0,7.0,980.0,0.0,47.3496,-122.289,1780.0,9135.0,59.0,0.0,0.0]},"out":{"variable":[247640.27]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1680.0,5000.0,2.0,0.0,0.0,3.0,8.0,1680.0,0.0,47.3196,-122.395,1720.0,5000.0,27.0,0.0,0.0]},"out":{"variable":[296411.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,2330.0,4950.0,1.5,0.0,0.0,3.0,6.0,1430.0,900.0,47.5585,-122.29,1160.0,5115.0,114.0,0.0,0.0]},"out":{"variable":[689450.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1630.0,1526.0,3.0,0.0,0.0,3.0,8.0,1630.0,0.0,47.6536,-122.354,1570.0,1274.0,0.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2040.0,3810.0,2.0,0.0,0.0,3.0,8.0,2040.0,0.0,47.3537,-122.0,2370.0,4590.0,9.0,0.0,0.0]},"out":{"variable":[328513.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.0,2280.0,5750.0,1.0,0.0,0.0,4.0,8.0,1140.0,1140.0,47.5672,-122.39,1780.0,5750.0,64.0,0.0,0.0]},"out":{"variable":[682284.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1350.0,10125.0,1.0,0.0,0.0,3.0,8.0,1350.0,0.0,47.3334,-122.298,1520.0,9720.0,48.0,0.0,0.0]},"out":{"variable":[248495.05]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.0,2050.0,10200.0,1.0,0.0,0.0,3.0,6.0,1430.0,620.0,47.4136,-122.333,1940.0,8625.0,58.0,0.0,0.0]},"out":{"variable":[355371.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1480.0,4800.0,2.0,0.0,0.0,4.0,7.0,1140.0,340.0,47.6567,-122.397,1810.0,4800.0,70.0,0.0,0.0]},"out":{"variable":[536175.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.5,2990.0,15085.0,2.0,0.0,0.0,3.0,9.0,2990.0,0.0,47.746,-122.218,3150.0,13076.0,8.0,0.0,0.0]},"out":{"variable":[810731.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1450.0,4500.0,1.5,0.0,0.0,4.0,7.0,1450.0,0.0,47.6739,-122.396,1470.0,5000.0,93.0,0.0,0.0]},"out":{"variable":[464057.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1200.0,2002.0,2.0,0.0,0.0,3.0,8.0,1200.0,0.0,47.4659,-122.189,1270.0,1848.0,48.0,0.0,0.0]},"out":{"variable":[241852.31]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[6.0,2.75,3500.0,5150.0,2.0,0.0,0.0,5.0,8.0,2430.0,1070.0,47.6842,-122.363,1430.0,3860.0,105.0,0.0,0.0]},"out":{"variable":[784103.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2190.0,4944.0,2.0,0.0,0.0,3.0,8.0,2190.0,0.0,47.5341,-121.866,2190.0,5108.0,16.0,0.0,0.0]},"out":{"variable":[598725.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2630.0,4611.0,2.0,0.0,0.0,3.0,8.0,2630.0,0.0,47.5322,-121.868,2220.0,5250.0,14.0,0.0,0.0]},"out":{"variable":[470087.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2550.0,5395.0,2.0,0.0,0.0,3.0,8.0,2550.0,0.0,47.5355,-121.874,2850.0,6109.0,14.0,0.0,0.0]},"out":{"variable":[699002.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[6.0,3.75,2930.0,14980.0,2.0,0.0,3.0,3.0,9.0,2930.0,0.0,47.5441,-122.117,3210.0,10787.0,1.0,0.0,0.0]},"out":{"variable":[788215.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.5,1630.0,20750.0,1.0,0.0,0.0,4.0,7.0,1100.0,530.0,47.3657,-122.113,1630.0,8640.0,40.0,0.0,0.0]},"out":{"variable":[278094.97]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,1.75,2320.0,7700.0,1.0,0.0,0.0,5.0,7.0,1290.0,1030.0,47.3426,-122.285,1740.0,7210.0,52.0,0.0,0.0]},"out":{"variable":[347191.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.5,1900.0,5065.0,2.0,0.0,0.0,3.0,8.0,1900.0,0.0,47.7175,-122.034,1350.0,4664.0,10.0,0.0,0.0]},"out":{"variable":[441512.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1020.0,7020.0,1.5,0.0,0.0,4.0,7.0,1020.0,0.0,47.7362,-122.314,1020.0,5871.0,61.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1280.0,16738.0,1.5,0.0,0.0,4.0,5.0,1280.0,0.0,47.3895,-122.023,1590.0,16317.0,82.0,0.0,0.0]},"out":{"variable":[246525.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3500.0,7048.0,2.0,0.0,0.0,3.0,9.0,3500.0,0.0,47.6811,-122.025,3920.0,7864.0,9.0,0.0,0.0]},"out":{"variable":[879092.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1810.0,8158.0,1.0,0.0,0.0,3.0,8.0,1450.0,360.0,47.6258,-122.038,1740.0,9532.0,30.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.0,1220.0,5120.0,1.5,0.0,0.0,5.0,6.0,1220.0,0.0,47.205,-121.996,1540.0,7670.0,75.0,0.0,0.0]},"out":{"variable":[244351.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.25,1720.0,1587.0,2.5,0.0,2.0,3.0,9.0,1410.0,310.0,47.6187,-122.299,1490.0,1620.0,11.0,0.0,0.0]},"out":{"variable":[725184.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1270.0,1333.0,3.0,0.0,0.0,3.0,8.0,1270.0,0.0,47.6933,-122.342,1330.0,1333.0,9.0,0.0,0.0]},"out":{"variable":[442168.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1250.0,3880.0,1.0,0.0,0.0,4.0,7.0,750.0,500.0,47.6869,-122.392,1240.0,3880.0,70.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3710.0,7491.0,2.0,0.0,0.0,3.0,9.0,3710.0,0.0,47.5596,-122.016,3040.0,7491.0,12.0,0.0,0.0]},"out":{"variable":[879092.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1500.0,10227.0,1.0,0.0,0.0,4.0,7.0,1000.0,500.0,47.2043,-121.996,1490.0,7670.0,69.0,0.0,0.0]},"out":{"variable":[256845.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,1160.0,1269.0,2.0,0.0,0.0,3.0,7.0,970.0,190.0,47.6608,-122.335,1700.0,3150.0,9.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2000.0,7414.0,2.0,0.0,0.0,4.0,7.0,2000.0,0.0,47.3508,-122.057,2000.0,7414.0,21.0,0.0,0.0]},"out":{"variable":[320863.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1880.0,11700.0,1.0,0.0,0.0,4.0,7.0,1880.0,0.0,47.3213,-122.187,2230.0,35200.0,46.0,0.0,0.0]},"out":{"variable":[296253.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.25,1710.0,2171.0,2.0,0.0,0.0,3.0,7.0,1400.0,310.0,47.5434,-122.368,1380.0,1300.0,0.0,0.0,0.0]},"out":{"variable":[544392.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,2240.0,3800.0,2.0,0.0,0.0,3.0,8.0,1370.0,870.0,47.6887,-122.307,1690.0,4275.0,85.0,0.0,0.0]},"out":{"variable":[682284.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,2720.0,4000.0,2.0,0.0,1.0,3.0,10.0,2070.0,650.0,47.5554,-122.267,1450.0,4000.0,0.0,0.0,0.0]},"out":{"variable":[941029.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2390.0,7875.0,1.0,0.0,1.0,3.0,10.0,1980.0,410.0,47.6515,-122.278,3720.0,9075.0,66.0,0.0,0.0]},"out":{"variable":[1364149.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.75,2050.0,3320.0,1.5,0.0,0.0,4.0,7.0,1580.0,470.0,47.6719,-122.301,1760.0,4150.0,87.0,0.0,0.0]},"out":{"variable":[630865.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.5,1100.0,1737.0,2.0,0.0,0.0,3.0,8.0,1100.0,0.0,47.4499,-122.189,1610.0,2563.0,8.0,0.0,0.0]},"out":{"variable":[241657.14]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[1.0,0.75,770.0,4600.0,1.0,0.0,0.0,4.0,6.0,770.0,0.0,47.5565,-122.377,1550.0,4600.0,104.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2920.0,6300.0,1.0,0.0,0.0,3.0,8.0,1710.0,1210.0,47.7065,-122.37,1940.0,6300.0,58.0,0.0,0.0]},"out":{"variable":[719351.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3340.0,34238.0,1.0,0.0,0.0,4.0,8.0,2060.0,1280.0,47.7654,-122.076,2400.0,36590.0,37.0,0.0,0.0]},"out":{"variable":[597475.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,4.25,4110.0,42755.0,2.0,0.0,2.0,3.0,10.0,2970.0,1140.0,47.3375,-122.337,2730.0,12750.0,14.0,0.0,0.0]},"out":{"variable":[900814.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.0,1560.0,1466.0,3.0,0.0,0.0,3.0,8.0,1560.0,0.0,47.6604,-122.352,1530.0,2975.0,8.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1900.0,7225.0,1.0,0.0,0.0,3.0,8.0,1220.0,680.0,47.6394,-122.113,1900.0,7399.0,44.0,0.0,0.0]},"out":{"variable":[563844.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1480.0,6210.0,1.0,0.0,0.0,3.0,7.0,1080.0,400.0,47.774,-122.351,1290.0,7509.0,64.0,0.0,0.0]},"out":{"variable":[415964.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1070.0,5000.0,1.0,0.0,0.0,3.0,7.0,1070.0,0.0,47.6666,-122.331,1710.0,5000.0,91.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,2080.0,11375.0,1.0,0.0,0.0,3.0,8.0,2080.0,0.0,47.214,-121.993,1080.0,12899.0,12.0,0.0,0.0]},"out":{"variable":[337248.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,1670.0,9880.0,1.0,0.0,0.0,4.0,7.0,1670.0,0.0,47.4864,-122.348,1670.0,9807.0,73.0,1.0,22.0]},"out":{"variable":[299854.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,1920.0,7500.0,1.0,0.0,0.0,4.0,7.0,1920.0,0.0,47.4222,-122.318,1490.0,8000.0,52.0,0.0,0.0]},"out":{"variable":[303936.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2780.0,6000.0,1.0,0.0,3.0,4.0,9.0,1670.0,1110.0,47.6442,-122.406,2780.0,6000.0,66.0,0.0,0.0]},"out":{"variable":[998351.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2990.0,6037.0,2.0,0.0,0.0,3.0,9.0,2990.0,0.0,47.4766,-121.735,2990.0,5992.0,2.0,0.0,0.0]},"out":{"variable":[524275.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.5,5430.0,10327.0,2.0,0.0,2.0,3.0,10.0,4010.0,1420.0,47.5476,-122.116,4340.0,10324.0,7.0,0.0,0.0]},"out":{"variable":[1207858.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1980.0,4500.0,2.0,0.0,0.0,3.0,7.0,1980.0,0.0,47.3671,-122.113,2200.0,4500.0,2.0,0.0,0.0]},"out":{"variable":[320395.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1240.0,12400.0,1.0,0.0,0.0,3.0,7.0,1240.0,0.0,47.607,-122.132,1640.0,9600.0,56.0,0.0,0.0]},"out":{"variable":[449699.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2360.0,4080.0,2.0,0.0,0.0,3.0,7.0,2360.0,0.0,47.6825,-122.038,2290.0,4080.0,11.0,0.0,0.0]},"out":{"variable":[701940.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.5,3260.0,24300.0,1.5,0.0,1.0,4.0,8.0,2310.0,950.0,47.7587,-122.274,2390.0,32057.0,64.0,0.0,0.0]},"out":{"variable":[611431.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2280.0,27441.0,2.0,0.0,0.0,3.0,8.0,2280.0,0.0,47.7628,-122.123,2350.0,35020.0,18.0,0.0,0.0]},"out":{"variable":[519346.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1480.0,4200.0,1.5,0.0,0.0,3.0,7.0,1480.0,0.0,47.6147,-122.298,1460.0,3600.0,89.0,0.0,0.0]},"out":{"variable":[533935.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1710.0,1664.0,2.0,0.0,0.0,5.0,8.0,1300.0,410.0,47.6456,-122.383,1470.0,5400.0,11.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.5,2800.0,7694.0,1.0,0.0,0.0,3.0,9.0,2800.0,0.0,47.7095,-122.022,2420.0,7694.0,10.0,0.0,0.0]},"out":{"variable":[759983.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,0.75,1080.0,5025.0,1.0,0.0,0.0,3.0,5.0,1080.0,0.0,47.4936,-122.335,1370.0,6000.0,66.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2840.0,8800.0,2.0,0.0,0.0,3.0,9.0,2840.0,0.0,47.7029,-122.171,1840.0,7700.0,6.0,0.0,0.0]},"out":{"variable":[765468.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2000.0,8700.0,1.0,0.0,0.0,5.0,7.0,1010.0,990.0,47.374,-122.141,1490.0,7350.0,39.0,0.0,0.0]},"out":{"variable":[317551.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1410.0,7700.0,1.0,0.0,0.0,3.0,7.0,980.0,430.0,47.4577,-122.171,1510.0,7700.0,52.0,0.0,0.0]},"out":{"variable":[257630.27]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1000.0,4171.0,1.0,0.0,0.0,3.0,7.0,1000.0,0.0,47.6834,-122.097,1090.0,3479.0,29.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1150.0,4000.0,1.0,0.0,0.0,3.0,7.0,1150.0,0.0,47.6575,-122.394,1150.0,4288.0,67.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1050.0,18304.0,1.0,0.0,0.0,4.0,7.0,1050.0,0.0,47.3206,-122.269,1690.0,15675.0,61.0,0.0,0.0]},"out":{"variable":[241809.89]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2580.0,21115.0,2.0,0.0,0.0,4.0,9.0,2580.0,0.0,47.5566,-122.219,2690.0,10165.0,37.0,0.0,0.0]},"out":{"variable":[793214.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1750.0,5200.0,1.0,0.0,1.0,4.0,8.0,1750.0,0.0,47.6995,-122.383,2060.0,5200.0,58.0,0.0,0.0]},"out":{"variable":[467742.97]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.0,3410.0,9600.0,1.0,0.0,0.0,4.0,8.0,1870.0,1540.0,47.6358,-122.103,2390.0,9679.0,46.0,0.0,0.0]},"out":{"variable":[732736.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.5,1500.0,3608.0,2.0,0.0,0.0,3.0,8.0,1500.0,0.0,47.5472,-121.994,2080.0,2686.0,9.0,0.0,0.0]},"out":{"variable":[525737.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,901.0,1245.0,3.0,0.0,0.0,3.0,7.0,901.0,0.0,47.6774,-122.325,1138.0,1137.0,13.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.75,2130.0,6222.0,1.0,0.0,0.0,3.0,7.0,1300.0,830.0,47.5272,-122.276,2130.0,6222.0,23.0,0.0,0.0]},"out":{"variable":[391460.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2640.0,8800.0,1.0,0.0,0.0,3.0,8.0,1620.0,1020.0,47.7552,-122.148,2500.0,11700.0,35.0,0.0,0.0]},"out":{"variable":[536371.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2100.0,2200.0,2.0,0.0,0.0,4.0,7.0,1500.0,600.0,47.614,-122.294,1750.0,4400.0,96.0,0.0,0.0]},"out":{"variable":[642519.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1080.0,9225.0,1.0,0.0,0.0,2.0,7.0,1080.0,0.0,47.4842,-122.346,1410.0,9840.0,59.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2590.0,12600.0,2.0,0.0,0.0,3.0,9.0,2590.0,0.0,47.5566,-122.162,2620.0,11050.0,36.0,0.0,0.0]},"out":{"variable":[758714.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2180.0,7876.0,1.0,0.0,0.0,4.0,7.0,1290.0,890.0,47.5157,-122.191,1960.0,7225.0,38.0,0.0,0.0]},"out":{"variable":[395096.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,810.0,5100.0,1.0,0.0,0.0,3.0,6.0,810.0,0.0,47.7317,-122.343,1500.0,5100.0,59.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2430.0,88426.0,1.0,0.0,0.0,4.0,7.0,1570.0,860.0,47.4828,-121.718,1560.0,56827.0,29.0,0.0,0.0]},"out":{"variable":[418823.38]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,4.0,4360.0,8030.0,2.0,0.0,0.0,3.0,10.0,4360.0,0.0,47.5923,-121.973,3570.0,6185.0,0.0,0.0,0.0]},"out":{"variable":[1160512.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.5,3190.0,29982.0,1.0,0.0,3.0,4.0,8.0,2630.0,560.0,47.458,-122.368,2600.0,19878.0,74.0,0.0,0.0]},"out":{"variable":[509102.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.75,2210.0,4000.0,2.0,0.0,0.0,3.0,8.0,2210.0,0.0,47.6954,-122.017,2230.0,4674.0,6.0,0.0,0.0]},"out":{"variable":[673519.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1453.0,2225.0,2.0,0.0,0.0,4.0,8.0,1453.0,0.0,47.5429,-122.188,1860.0,2526.0,29.0,0.0,0.0]},"out":{"variable":[453298.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1790.0,3962.0,2.0,0.0,0.0,3.0,8.0,1790.0,0.0,47.6894,-122.391,1340.0,3960.0,22.0,0.0,0.0]},"out":{"variable":[554922.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,730.0,5040.0,1.0,0.0,0.0,3.0,6.0,730.0,0.0,47.5387,-122.374,790.0,5040.0,87.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.75,2690.0,4000.0,2.0,0.0,3.0,4.0,9.0,2120.0,570.0,47.6418,-122.372,2830.0,4000.0,105.0,1.0,80.0]},"out":{"variable":[999203.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1240.0,10800.0,1.0,0.0,0.0,5.0,7.0,1240.0,0.0,47.5233,-122.185,1810.0,10800.0,55.0,0.0,0.0]},"out":{"variable":[268856.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1300.0,8284.0,1.0,0.0,0.0,3.0,7.0,1300.0,0.0,47.3327,-122.306,1360.0,7848.0,46.0,0.0,0.0]},"out":{"variable":[243560.81]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1340.0,8505.0,1.0,0.0,0.0,3.0,6.0,1340.0,0.0,47.4727,-122.297,1370.0,9000.0,83.0,0.0,0.0]},"out":{"variable":[246026.86]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[1.0,1.0,900.0,4368.0,1.0,0.0,0.0,5.0,6.0,900.0,0.0,47.2107,-121.99,1290.0,5000.0,100.0,1.0,35.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2120.0,9706.0,1.0,0.0,0.0,3.0,7.0,1370.0,750.0,47.4939,-122.297,1730.0,11337.0,49.0,0.0,0.0]},"out":{"variable":[385561.78]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,2500.0,5801.0,1.5,0.0,0.0,3.0,8.0,1960.0,540.0,47.632,-122.29,3670.0,7350.0,88.0,0.0,0.0]},"out":{"variable":[739536.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1670.0,5400.0,2.0,0.0,0.0,5.0,8.0,1670.0,0.0,47.635,-122.284,2100.0,5400.0,102.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1200.0,9266.0,1.0,0.0,0.0,4.0,7.0,1200.0,0.0,47.314,-122.208,1200.0,9266.0,54.0,0.0,0.0]},"out":{"variable":[241330.19]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1330.0,36537.0,1.0,0.0,0.0,4.0,7.0,1330.0,0.0,47.3126,-122.129,1650.0,35100.0,26.0,0.0,0.0]},"out":{"variable":[249455.83]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.5,2340.0,5670.0,2.0,0.0,0.0,3.0,7.0,2340.0,0.0,47.4913,-122.152,2190.0,4869.0,5.0,0.0,0.0]},"out":{"variable":[407019.03]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2160.0,4297.0,2.0,0.0,0.0,3.0,9.0,2160.0,0.0,47.5476,-122.012,2160.0,3968.0,15.0,0.0,0.0]},"out":{"variable":[723867.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1120.0,8661.0,1.0,0.0,0.0,3.0,7.0,1120.0,0.0,47.7034,-122.307,1470.0,7205.0,68.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1350.0,8220.0,1.0,0.0,0.0,3.0,7.0,1060.0,290.0,47.7224,-122.358,1540.0,8280.0,66.0,0.0,0.0]},"out":{"variable":[342604.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2800.0,9538.0,2.0,0.0,0.0,3.0,8.0,2800.0,0.0,47.2675,-122.307,1970.0,7750.0,21.0,0.0,0.0]},"out":{"variable":[375104.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1260.0,1488.0,3.0,0.0,0.0,3.0,7.0,1260.0,0.0,47.7071,-122.336,1190.0,1095.0,5.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,1990.0,7712.0,1.0,0.0,0.0,3.0,8.0,1210.0,780.0,47.5688,-122.087,1720.0,7393.0,41.0,0.0,0.0]},"out":{"variable":[575724.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2330.0,16300.0,2.0,0.0,0.0,3.0,9.0,2330.0,0.0,47.7037,-122.24,2330.0,16300.0,51.0,0.0,0.0]},"out":{"variable":[920796.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[9.0,3.0,3680.0,4400.0,2.0,0.0,0.0,3.0,7.0,2830.0,850.0,47.6374,-122.324,1960.0,2450.0,107.0,0.0,0.0]},"out":{"variable":[784103.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2340.0,6183.0,1.0,0.0,0.0,3.0,7.0,1210.0,1130.0,47.6979,-122.31,1970.0,6183.0,85.0,0.0,0.0]},"out":{"variable":[676904.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2140.0,159865.0,1.0,0.0,0.0,4.0,7.0,1140.0,1000.0,47.4235,-122.218,1830.0,15569.0,54.0,0.0,0.0]},"out":{"variable":[385957.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.25,3080.0,12100.0,2.0,0.0,0.0,3.0,8.0,2080.0,1000.0,47.695,-122.399,2100.0,6581.0,30.0,0.0,0.0]},"out":{"variable":[756699.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3190.0,24170.0,2.0,0.0,0.0,3.0,10.0,3190.0,0.0,47.6209,-122.052,2110.0,26321.0,13.0,0.0,0.0]},"out":{"variable":[886958.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2570.0,7221.0,1.0,0.0,0.0,4.0,8.0,1570.0,1000.0,47.6921,-122.387,2440.0,7274.0,57.0,0.0,0.0]},"out":{"variable":[713978.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2830.0,5932.0,2.0,0.0,0.0,3.0,9.0,2830.0,0.0,47.6479,-122.408,2840.0,5593.0,0.0,0.0,0.0]},"out":{"variable":[800304.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1440.0,11787.0,1.0,0.0,0.0,3.0,8.0,1440.0,0.0,47.6276,-122.033,2190.0,11787.0,31.0,0.0,0.0]},"out":{"variable":[462431.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.5,3740.0,41458.0,2.0,0.0,2.0,3.0,11.0,3740.0,0.0,47.7375,-122.139,3750.0,38325.0,14.0,0.0,0.0]},"out":{"variable":[957666.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1680.0,4584.0,2.0,0.0,0.0,3.0,7.0,1680.0,0.0,47.4794,-122.182,2160.0,4621.0,11.0,0.0,0.0]},"out":{"variable":[311515.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,3230.0,17833.0,2.0,0.0,0.0,4.0,9.0,3230.0,0.0,47.5683,-122.188,3690.0,17162.0,41.0,0.0,0.0]},"out":{"variable":[937281.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1800.0,4357.0,2.0,0.0,0.0,3.0,8.0,1800.0,0.0,47.5337,-121.841,1800.0,3663.0,2.0,0.0,0.0]},"out":{"variable":[450996.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1460.0,11481.0,1.0,0.0,0.0,2.0,7.0,1170.0,290.0,47.4493,-121.777,1540.0,9680.0,19.0,0.0,0.0]},"out":{"variable":[266405.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,3470.0,212639.0,2.0,0.0,0.0,3.0,7.0,2070.0,1400.0,47.7066,-121.968,2370.0,233917.0,21.0,0.0,0.0]},"out":{"variable":[727923.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,3310.0,5300.0,2.0,0.0,2.0,3.0,8.0,2440.0,870.0,47.5178,-122.389,2140.0,7500.0,6.0,0.0,0.0]},"out":{"variable":[480151.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1770.0,6014.0,1.5,0.0,0.0,4.0,7.0,1240.0,530.0,47.5773,-122.393,1740.0,6014.0,68.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1560.0,1020.0,3.0,0.0,0.0,3.0,8.0,1560.0,0.0,47.605,-122.304,1560.0,1728.0,0.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1350.0,14200.0,1.0,0.0,0.0,3.0,7.0,1350.0,0.0,47.7315,-121.972,2100.0,15101.0,25.0,0.0,0.0]},"out":{"variable":[342604.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1840.0,5000.0,1.5,0.0,0.0,5.0,7.0,1340.0,500.0,47.6652,-122.362,1840.0,5000.0,99.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2480.0,10804.0,1.0,0.0,0.0,3.0,8.0,1800.0,680.0,47.7721,-122.367,2480.0,10400.0,38.0,0.0,0.0]},"out":{"variable":[536371.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1030.0,8414.0,1.0,0.0,0.0,4.0,7.0,1030.0,0.0,47.7654,-122.297,1750.0,8414.0,47.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2450.0,15002.0,1.0,0.0,0.0,5.0,9.0,2450.0,0.0,47.4268,-122.343,2650.0,15055.0,40.0,0.0,0.0]},"out":{"variable":[508746.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1010.0,4000.0,1.0,0.0,0.0,3.0,6.0,1010.0,0.0,47.5536,-122.267,1040.0,4000.0,103.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1330.0,1200.0,3.0,0.0,0.0,3.0,7.0,1330.0,0.0,47.7034,-122.344,1330.0,1206.0,12.0,0.0,0.0]},"out":{"variable":[342604.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.75,2770.0,19700.0,2.0,0.0,0.0,3.0,8.0,1780.0,990.0,47.7581,-122.365,2360.0,9700.0,31.0,0.0,0.0]},"out":{"variable":[536371.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1900.0,5520.0,1.0,0.0,0.0,3.0,7.0,1280.0,620.0,47.5549,-122.292,1330.0,5196.0,32.0,0.0,0.0]},"out":{"variable":[551223.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.5,1470.0,2034.0,2.0,0.0,0.0,4.0,8.0,1470.0,0.0,47.6213,-122.153,1510.0,2055.0,29.0,0.0,0.0]},"out":{"variable":[517121.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.5,2770.0,8763.0,2.0,0.0,0.0,3.0,8.0,2100.0,670.0,47.2625,-122.308,2030.0,7242.0,18.0,0.0,0.0]},"out":{"variable":[373955.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3550.0,35689.0,2.0,0.0,0.0,4.0,9.0,3550.0,0.0,47.7503,-122.074,3350.0,35711.0,23.0,0.0,0.0]},"out":{"variable":[873314.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2510.0,47044.0,2.0,0.0,0.0,3.0,9.0,2510.0,0.0,47.7699,-122.085,2600.0,42612.0,27.0,0.0,0.0]},"out":{"variable":[721143.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,4090.0,11225.0,2.0,0.0,0.0,3.0,10.0,4090.0,0.0,47.581,-121.971,3510.0,8762.0,9.0,0.0,0.0]},"out":{"variable":[1048372.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,720.0,5000.0,1.0,0.0,0.0,5.0,6.0,720.0,0.0,47.5195,-122.374,810.0,5000.0,63.0,0.0,0.0]},"out":{"variable":[244566.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2930.0,22000.0,1.0,0.0,3.0,4.0,9.0,1580.0,1350.0,47.3227,-122.384,2930.0,9758.0,36.0,0.0,0.0]},"out":{"variable":[518869.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,850.0,5000.0,1.0,0.0,0.0,3.0,6.0,850.0,0.0,47.3817,-122.314,1160.0,5000.0,39.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1570.0,1433.0,3.0,0.0,0.0,3.0,8.0,1570.0,0.0,47.6858,-122.336,1570.0,2652.0,4.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2390.0,15669.0,2.0,0.0,0.0,3.0,9.0,2390.0,0.0,47.7446,-122.193,2640.0,12500.0,24.0,0.0,0.0]},"out":{"variable":[741973.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,0.75,920.0,20412.0,1.0,1.0,2.0,5.0,6.0,920.0,0.0,47.4781,-122.49,1162.0,54705.0,64.0,0.0,0.0]},"out":{"variable":[338418.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2800.0,246114.0,2.0,0.0,0.0,3.0,9.0,2800.0,0.0,47.6586,-121.962,2750.0,60351.0,15.0,0.0,0.0]},"out":{"variable":[765468.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1120.0,9912.0,1.0,0.0,0.0,4.0,6.0,1120.0,0.0,47.3735,-122.43,1540.0,9750.0,34.0,0.0,0.0]},"out":{"variable":[309800.72]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.5,2760.0,4500.0,2.0,0.0,0.0,3.0,9.0,2120.0,640.0,47.6529,-122.372,1950.0,6000.0,10.0,0.0,0.0]},"out":{"variable":[798189.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2710.0,11400.0,1.0,0.0,0.0,4.0,9.0,1430.0,1280.0,47.561,-122.153,2640.0,11000.0,38.0,0.0,0.0]},"out":{"variable":[772047.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1700.0,7496.0,2.0,0.0,0.0,3.0,8.0,1700.0,0.0,47.432,-122.189,2280.0,7496.0,20.0,0.0,0.0]},"out":{"variable":[310992.97]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.5,3420.0,33106.0,2.0,0.0,0.0,3.0,9.0,3420.0,0.0,47.3554,-121.986,3420.0,36590.0,10.0,0.0,0.0]},"out":{"variable":[539867.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.0,1060.0,5750.0,1.0,0.0,0.0,3.0,6.0,950.0,110.0,47.6562,-122.389,1790.0,5857.0,110.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2720.0,54048.0,2.0,0.0,0.0,3.0,8.0,2720.0,0.0,47.7181,-122.089,2580.0,37721.0,30.0,0.0,0.0]},"out":{"variable":[546009.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2740.0,43101.0,2.0,0.0,0.0,3.0,9.0,2740.0,0.0,47.7649,-122.049,2740.0,33447.0,21.0,0.0,0.0]},"out":{"variable":[727898.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,3320.0,8587.0,3.0,0.0,0.0,3.0,11.0,2950.0,370.0,47.691,-122.337,1860.0,5668.0,6.0,0.0,0.0]},"out":{"variable":[1130661.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3130.0,13202.0,2.0,0.0,0.0,3.0,10.0,3130.0,0.0,47.5878,-121.976,2840.0,10470.0,19.0,0.0,0.0]},"out":{"variable":[879083.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.75,1370.0,5125.0,1.0,0.0,0.0,5.0,6.0,1370.0,0.0,47.6926,-122.346,1200.0,5100.0,70.0,0.0,0.0]},"out":{"variable":[444933.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2040.0,5508.0,2.0,0.0,0.0,4.0,8.0,2040.0,0.0,47.5719,-122.007,2130.0,5496.0,18.0,0.0,0.0]},"out":{"variable":[627853.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3140.0,12792.0,2.0,0.0,0.0,4.0,9.0,3140.0,0.0,47.3863,-122.156,2510.0,12792.0,37.0,0.0,0.0]},"out":{"variable":[513583.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.0,1190.0,7620.0,1.5,0.0,0.0,3.0,6.0,1190.0,0.0,47.5281,-122.348,1060.0,7320.0,88.0,0.0,0.0]},"out":{"variable":[247792.72]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2927.0,12171.0,2.0,0.0,0.0,3.0,10.0,2927.0,0.0,47.5948,-121.983,2967.0,12166.0,17.0,0.0,0.0]},"out":{"variable":[829775.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.75,4170.0,8142.0,2.0,0.0,2.0,3.0,10.0,4170.0,0.0,47.5354,-122.181,3030.0,7980.0,9.0,0.0,0.0]},"out":{"variable":[1098628.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2520.0,5000.0,3.0,0.0,0.0,3.0,9.0,2520.0,0.0,47.5664,-122.359,1130.0,5000.0,24.0,0.0,0.0]},"out":{"variable":[793214.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.5,2738.0,6031.0,2.0,0.0,0.0,3.0,8.0,2738.0,0.0,47.2962,-122.35,2738.0,5201.0,0.0,0.0,0.0]},"out":{"variable":[371040.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1110.0,8724.0,1.0,0.0,0.0,4.0,7.0,1110.0,0.0,47.3056,-122.206,1390.0,7750.0,24.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1180.0,1231.0,3.0,0.0,0.0,3.0,7.0,1180.0,0.0,47.6845,-122.315,1280.0,3360.0,7.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,910.0,6000.0,1.0,0.0,0.0,2.0,6.0,910.0,0.0,47.5065,-122.338,1090.0,6957.0,58.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1540.0,7168.0,1.0,0.0,0.0,3.0,7.0,1160.0,380.0,47.455,-122.198,1540.0,7176.0,51.0,0.0,0.0]},"out":{"variable":[293808.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,1570.0,9415.0,2.0,0.0,0.0,4.0,7.0,1570.0,0.0,47.3168,-122.174,1550.0,8978.0,30.0,0.0,0.0]},"out":{"variable":[276709.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2160.0,9682.0,2.0,0.0,0.0,3.0,8.0,2160.0,0.0,47.4106,-122.204,1770.0,9600.0,15.0,0.0,0.0]},"out":{"variable":[363491.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1190.0,9199.0,1.0,0.0,0.0,3.0,7.0,1190.0,0.0,47.4258,-122.322,1190.0,9364.0,59.0,0.0,0.0]},"out":{"variable":[241330.19]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,4.25,3500.0,8750.0,1.0,0.0,4.0,5.0,9.0,2140.0,1360.0,47.7222,-122.367,3110.0,8750.0,63.0,0.0,0.0]},"out":{"variable":[1140733.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2230.0,9625.0,1.0,0.0,4.0,3.0,8.0,1180.0,1050.0,47.508,-122.244,2300.0,8211.0,59.0,0.0,0.0]},"out":{"variable":[473591.84]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2420.0,9147.0,2.0,0.0,0.0,3.0,10.0,2420.0,0.0,47.3221,-122.322,1400.0,7200.0,16.0,0.0,0.0]},"out":{"variable":[559139.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1750.0,7208.0,2.0,0.0,0.0,3.0,8.0,1750.0,0.0,47.4315,-122.192,2050.0,7524.0,20.0,0.0,0.0]},"out":{"variable":[311909.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,1.75,2330.0,6450.0,1.0,0.0,1.0,3.0,8.0,1330.0,1000.0,47.4959,-122.367,2330.0,8258.0,57.0,0.0,0.0]},"out":{"variable":[448720.22]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.5,4460.0,16271.0,2.0,0.0,2.0,3.0,11.0,4460.0,0.0,47.5862,-121.97,4540.0,17122.0,13.0,0.0,0.0]},"out":{"variable":[1208638.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.75,3010.0,1842.0,2.0,0.0,0.0,3.0,9.0,3010.0,0.0,47.5836,-121.994,2950.0,4200.0,3.0,0.0,0.0]},"out":{"variable":[795841.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,1780.0,4750.0,1.0,0.0,0.0,4.0,7.0,1080.0,700.0,47.6859,-122.395,1690.0,5962.0,67.0,0.0,0.0]},"out":{"variable":[558463.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1650.0,2201.0,3.0,0.0,0.0,3.0,8.0,1650.0,0.0,47.7108,-122.333,1650.0,1965.0,8.0,0.0,0.0]},"out":{"variable":[439977.38]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2370.0,11310.0,1.0,0.0,0.0,3.0,8.0,1550.0,820.0,47.7684,-122.289,1890.0,8621.0,47.0,0.0,0.0]},"out":{"variable":[536371.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.0,1520.0,1884.0,3.0,0.0,0.0,3.0,8.0,1520.0,0.0,47.7176,-122.284,1360.0,1939.0,5.0,0.0,0.0]},"out":{"variable":[424966.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.0,1300.0,4659.0,1.0,0.0,0.0,3.0,8.0,1300.0,0.0,47.7132,-122.033,1640.0,4780.0,9.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2074.0,4900.0,2.0,0.0,0.0,3.0,8.0,2074.0,0.0,47.7327,-122.233,1840.0,7382.0,17.0,0.0,0.0]},"out":{"variable":[483519.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3200.0,6691.0,2.0,0.0,0.0,3.0,7.0,3200.0,0.0,47.367,-122.031,2610.0,6510.0,13.0,0.0,0.0]},"out":{"variable":[383833.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1060.0,12690.0,1.0,0.0,0.0,3.0,7.0,1060.0,0.0,47.6736,-122.167,1920.0,10200.0,45.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.75,1740.0,7290.0,1.0,0.0,0.0,3.0,8.0,1280.0,460.0,47.6461,-122.397,1820.0,6174.0,64.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2490.0,8700.0,1.0,0.0,0.0,3.0,7.0,1890.0,600.0,47.7397,-122.324,1470.0,7975.0,39.0,0.0,0.0]},"out":{"variable":[530288.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1050.0,7854.0,1.0,0.0,0.0,4.0,7.0,1050.0,0.0,47.3011,-122.369,1360.0,7668.0,40.0,0.0,0.0]},"out":{"variable":[239734.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1800.0,6750.0,1.5,0.0,0.0,4.0,7.0,1800.0,0.0,47.6868,-122.285,1420.0,5900.0,64.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2570.0,6466.0,2.0,0.0,0.0,3.0,9.0,2570.0,0.0,47.3324,-122.17,2520.0,6667.0,15.0,0.0,0.0]},"out":{"variable":[464060.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1590.0,21600.0,1.5,0.0,0.0,4.0,7.0,1590.0,0.0,47.2159,-121.966,1780.0,21600.0,44.0,0.0,0.0]},"out":{"variable":[283759.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,1400.0,1650.0,3.0,0.0,0.0,3.0,7.0,1400.0,0.0,47.7222,-122.29,1430.0,1650.0,15.0,0.0,0.0]},"out":{"variable":[342604.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3010.0,7953.0,2.0,0.0,0.0,3.0,9.0,3010.0,0.0,47.522,-122.19,2670.0,6202.0,14.0,0.0,0.0]},"out":{"variable":[660749.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1280.0,9000.0,1.5,0.0,0.0,4.0,6.0,1280.0,0.0,47.4915,-122.338,1430.0,4500.0,60.0,0.0,0.0]},"out":{"variable":[243585.28]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1270.0,6760.0,1.0,0.0,0.0,5.0,7.0,1270.0,0.0,47.7381,-122.179,1550.0,5734.0,42.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.75,2160.0,7200.0,1.5,0.0,0.0,3.0,7.0,1220.0,940.0,47.5576,-122.273,1900.0,7200.0,59.0,0.0,0.0]},"out":{"variable":[673288.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.5,4285.0,9567.0,2.0,0.0,1.0,5.0,10.0,3485.0,800.0,47.6434,-122.409,2960.0,6902.0,68.0,0.0,0.0]},"out":{"variable":[1886959.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.75,2060.0,8906.0,1.0,0.0,0.0,4.0,7.0,1220.0,840.0,47.5358,-122.289,1840.0,8906.0,36.0,0.0,0.0]},"out":{"variable":[627884.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,2670.0,5001.0,1.0,0.0,0.0,3.0,9.0,1640.0,1030.0,47.5666,-122.293,1610.0,5001.0,1.0,0.0,0.0]},"out":{"variable":[793214.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2380.0,13550.0,2.0,0.0,0.0,3.0,7.0,2380.0,0.0,47.4486,-122.288,1230.0,9450.0,15.0,0.0,0.0]},"out":{"variable":[397096.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.75,3600.0,9437.0,2.0,0.0,0.0,3.0,9.0,3600.0,0.0,47.4822,-122.131,3550.0,9421.0,0.0,0.0,0.0]},"out":{"variable":[542342.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[1.0,1.0,1090.0,32010.0,1.0,0.0,0.0,4.0,6.0,1090.0,0.0,47.6928,-121.87,1870.0,25346.0,56.0,0.0,0.0]},"out":{"variable":[444407.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,1700.0,6375.0,1.0,0.0,0.0,4.0,7.0,850.0,850.0,47.6973,-122.295,1470.0,8360.0,65.0,0.0,0.0]},"out":{"variable":[469038.03]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.0,2000.0,4211.0,1.5,0.0,2.0,4.0,7.0,1280.0,720.0,47.6283,-122.301,1680.0,4000.0,106.0,0.0,0.0]},"out":{"variable":[582564.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1780.0,9969.0,1.0,0.0,0.0,3.0,8.0,1450.0,330.0,47.7286,-122.168,1950.0,7974.0,29.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2190.0,6450.0,1.0,0.0,0.0,3.0,8.0,1480.0,710.0,47.5284,-122.391,2190.0,6450.0,58.0,0.0,0.0]},"out":{"variable":[421402.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2220.0,10530.0,1.0,0.0,0.0,4.0,8.0,1700.0,520.0,47.6383,-122.098,2500.0,10014.0,41.0,0.0,0.0]},"out":{"variable":[680620.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,1940.0,4400.0,1.0,0.0,0.0,3.0,7.0,970.0,970.0,47.6371,-122.279,1480.0,3080.0,92.0,0.0,0.0]},"out":{"variable":[567502.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,2120.0,6290.0,1.0,0.0,0.0,4.0,8.0,1220.0,900.0,47.5658,-122.318,1620.0,5400.0,65.0,0.0,0.0]},"out":{"variable":[657905.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2242.0,4800.0,2.0,0.0,0.0,3.0,8.0,2242.0,0.0,47.2581,-122.2,2009.0,4800.0,2.0,0.0,0.0]},"out":{"variable":[343304.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,770.0,5040.0,1.0,0.0,0.0,3.0,5.0,770.0,0.0,47.5964,-122.299,1330.0,2580.0,85.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.0,3320.0,5354.0,2.0,0.0,0.0,3.0,9.0,3320.0,0.0,47.6542,-122.331,2330.0,4040.0,11.0,0.0,0.0]},"out":{"variable":[974485.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,840.0,9480.0,1.0,0.0,0.0,3.0,6.0,840.0,0.0,47.3277,-122.341,840.0,9420.0,55.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,2000.0,5000.0,1.5,0.0,0.0,5.0,7.0,2000.0,0.0,47.5787,-122.293,2200.0,5000.0,90.0,0.0,0.0]},"out":{"variable":[581002.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,800.0,6016.0,1.0,0.0,0.0,3.0,6.0,800.0,0.0,47.6913,-122.369,1470.0,3734.0,72.0,0.0,0.0]},"out":{"variable":[446768.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2300.0,35287.0,2.0,0.0,0.0,3.0,8.0,2300.0,0.0,47.2477,-121.937,1760.0,47916.0,38.0,0.0,0.0]},"out":{"variable":[400628.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,4.0,1680.0,7268.0,1.0,0.0,0.0,3.0,8.0,1370.0,310.0,47.5571,-122.356,2040.0,8259.0,7.0,0.0,0.0]},"out":{"variable":[546632.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1180.0,10277.0,1.0,0.0,0.0,3.0,6.0,1180.0,0.0,47.488,-121.787,1680.0,11104.0,31.0,0.0,0.0]},"out":{"variable":[246775.17]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.0,1460.0,3840.0,1.5,0.0,0.0,3.0,8.0,1340.0,120.0,47.533,-122.347,990.0,4200.0,87.0,0.0,0.0]},"out":{"variable":[288798.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1540.0,3570.0,1.5,0.0,0.0,5.0,7.0,1490.0,50.0,47.6692,-122.325,1620.0,4080.0,84.0,0.0,0.0]},"out":{"variable":[552992.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,870.0,4600.0,1.0,0.0,0.0,4.0,7.0,870.0,0.0,47.5274,-122.379,930.0,4600.0,72.0,0.0,0.0]},"out":{"variable":[313906.47]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.0,2010.0,3797.0,1.5,0.0,0.0,3.0,7.0,1450.0,560.0,47.5596,-122.315,1660.0,4650.0,92.0,1.0,82.0]},"out":{"variable":[578362.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,1790.0,47250.0,1.0,0.0,0.0,3.0,7.0,1220.0,570.0,47.5302,-121.746,1250.0,43791.0,27.0,0.0,0.0]},"out":{"variable":[323826.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2230.0,4372.0,2.0,0.0,0.0,5.0,8.0,1540.0,690.0,47.6698,-122.334,2020.0,4372.0,79.0,0.0,0.0]},"out":{"variable":[682284.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1310.0,9855.0,1.0,0.0,0.0,3.0,7.0,1310.0,0.0,47.7296,-122.241,1310.0,8370.0,52.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.0,2170.0,2500.0,2.0,0.0,0.0,3.0,8.0,1710.0,460.0,47.6742,-122.303,2170.0,4080.0,17.0,0.0,0.0]},"out":{"variable":[675545.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1180.0,14258.0,2.0,0.0,0.0,3.0,7.0,1180.0,0.0,47.7112,-122.238,1860.0,10390.0,27.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1910.0,5040.0,1.5,0.0,0.0,3.0,8.0,1910.0,0.0,47.6312,-122.061,1980.0,4275.0,43.0,0.0,0.0]},"out":{"variable":[563844.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,0.75,900.0,3527.0,1.0,0.0,0.0,3.0,6.0,900.0,0.0,47.5083,-122.336,1220.0,4080.0,76.0,0.0,0.0]},"out":{"variable":[236815.78]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2180.0,8000.0,1.0,0.0,0.0,4.0,9.0,1630.0,550.0,47.317,-122.378,2310.0,8000.0,39.0,0.0,0.0]},"out":{"variable":[444141.97]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.75,1670.0,3330.0,1.5,0.0,0.0,4.0,7.0,1670.0,0.0,47.6551,-122.36,1370.0,5000.0,89.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3250.0,8970.0,2.0,0.0,0.0,3.0,10.0,3250.0,0.0,47.5862,-122.037,3240.0,8449.0,20.0,0.0,0.0]},"out":{"variable":[886958.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1720.0,5525.0,1.0,0.0,2.0,5.0,7.0,960.0,760.0,47.559,-122.298,1760.0,5525.0,73.0,0.0,0.0]},"out":{"variable":[546632.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1060.0,10228.0,1.0,0.0,0.0,3.0,7.0,1060.0,0.0,47.7481,-122.3,1570.0,10228.0,66.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2640.0,8625.0,2.0,0.0,0.0,3.0,8.0,2640.0,0.0,47.4598,-122.15,2240.0,8700.0,27.0,0.0,0.0]},"out":{"variable":[441465.78]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1440.0,27505.0,1.0,0.0,0.0,3.0,8.0,1440.0,0.0,47.7553,-122.37,2430.0,16400.0,64.0,0.0,0.0]},"out":{"variable":[359614.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.0,3120.0,157875.0,2.0,0.0,0.0,4.0,8.0,3120.0,0.0,47.444,-122.187,1580.0,7050.0,38.0,0.0,0.0]},"out":{"variable":[448180.78]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1730.0,7442.0,2.0,0.0,0.0,3.0,7.0,1730.0,0.0,47.3507,-122.178,1630.0,6458.0,27.0,0.0,0.0]},"out":{"variable":[285253.16]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,1540.0,7200.0,1.0,0.0,0.0,3.0,7.0,1260.0,280.0,47.3424,-122.308,1540.0,8416.0,48.0,0.0,0.0]},"out":{"variable":[275408.03]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1220.0,8040.0,1.5,0.0,0.0,3.0,7.0,1220.0,0.0,47.7133,-122.308,1360.0,8040.0,50.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1460.0,9759.0,1.0,0.0,0.0,5.0,7.0,1460.0,0.0,47.6644,-122.144,1620.0,8421.0,42.0,0.0,0.0]},"out":{"variable":[498579.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1650.0,9780.0,1.0,0.0,0.0,3.0,7.0,950.0,700.0,47.6549,-122.394,1650.0,5458.0,72.0,0.0,0.0]},"out":{"variable":[558463.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1700.0,7532.0,1.0,0.0,0.0,3.0,7.0,1700.0,0.0,47.355,-122.176,1690.0,7405.0,27.0,0.0,0.0]},"out":{"variable":[284336.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2206.0,82031.0,1.0,0.0,2.0,3.0,6.0,866.0,1340.0,47.6302,-122.069,2590.0,53024.0,31.0,0.0,0.0]},"out":{"variable":[682181.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,750.0,4000.0,1.0,0.0,0.0,4.0,6.0,750.0,0.0,47.5547,-122.272,1120.0,5038.0,89.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1100.0,5400.0,1.5,0.0,0.0,3.0,7.0,1100.0,0.0,47.6604,-122.396,1770.0,4400.0,106.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2340.0,8990.0,2.0,0.0,0.0,3.0,8.0,2340.0,0.0,47.3781,-122.03,2980.0,6718.0,11.0,0.0,0.0]},"out":{"variable":[349102.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2120.0,3220.0,2.0,0.0,0.0,3.0,9.0,2120.0,0.0,47.6662,-122.083,2120.0,3547.0,9.0,0.0,0.0]},"out":{"variable":[713948.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2520.0,53143.0,1.5,0.0,0.0,3.0,7.0,2520.0,0.0,47.743,-121.925,2020.0,56628.0,26.0,0.0,0.0]},"out":{"variable":[530288.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.5,2960.0,8968.0,1.0,0.0,0.0,4.0,8.0,1640.0,1320.0,47.6233,-122.102,1890.0,9077.0,49.0,0.0,0.0]},"out":{"variable":[713485.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.0,1510.0,7200.0,1.5,0.0,0.0,4.0,7.0,1510.0,0.0,47.761,-122.307,1950.0,10656.0,60.0,0.0,0.0]},"out":{"variable":[421306.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2350.0,18800.0,1.0,0.0,2.0,3.0,8.0,2350.0,0.0,47.5904,-122.177,3050.0,14640.0,55.0,0.0,0.0]},"out":{"variable":[718588.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1980.0,6566.0,2.0,0.0,0.0,3.0,8.0,1980.0,0.0,47.3809,-122.097,2590.0,6999.0,11.0,0.0,0.0]},"out":{"variable":[320395.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2170.0,3200.0,1.5,0.0,0.0,5.0,7.0,1280.0,890.0,47.6543,-122.347,1180.0,1224.0,91.0,0.0,0.0]},"out":{"variable":[675545.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.5,3940.0,11632.0,2.0,0.0,0.0,3.0,10.0,3940.0,0.0,47.438,-122.344,2015.0,11632.0,0.0,0.0,0.0]},"out":{"variable":[655009.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1390.0,2815.0,2.0,0.0,0.0,3.0,8.0,1390.0,0.0,47.566,-122.366,1390.0,3700.0,16.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1400.0,7384.0,1.0,0.0,0.0,3.0,7.0,1150.0,250.0,47.4655,-122.174,1820.0,7992.0,35.0,0.0,0.0]},"out":{"variable":[267013.97]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2780.0,32880.0,1.0,0.0,0.0,3.0,9.0,2780.0,0.0,47.4798,-121.727,2780.0,40091.0,21.0,0.0,0.0]},"out":{"variable":[533212.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1250.0,1150.0,2.0,0.0,0.0,3.0,8.0,1080.0,170.0,47.5582,-122.363,1250.0,1150.0,6.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2360.0,8290.0,1.0,0.0,0.0,4.0,7.0,1180.0,1180.0,47.6738,-122.281,1880.0,7670.0,65.0,0.0,0.0]},"out":{"variable":[713978.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3070.0,6923.0,2.0,0.0,0.0,3.0,9.0,3070.0,0.0,47.669,-122.172,2190.0,9218.0,5.0,0.0,0.0]},"out":{"variable":[831483.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2370.0,10631.0,2.0,0.0,0.0,3.0,8.0,2370.0,0.0,47.3473,-122.302,2200.0,8297.0,15.0,0.0,0.0]},"out":{"variable":[349102.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1780.0,7800.0,1.0,0.0,0.0,3.0,7.0,1060.0,720.0,47.4932,-122.263,1450.0,7800.0,57.0,0.0,0.0]},"out":{"variable":[300446.72]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,4240.0,25639.0,2.0,0.0,3.0,3.0,10.0,3550.0,690.0,47.3241,-122.378,3590.0,24967.0,25.0,0.0,0.0]},"out":{"variable":[1156651.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.5,3440.0,9776.0,2.0,0.0,0.0,3.0,10.0,3440.0,0.0,47.5374,-122.216,2400.0,11000.0,9.0,0.0,0.0]},"out":{"variable":[1124493.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,3.25,1400.0,1243.0,3.0,0.0,0.0,3.0,8.0,1400.0,0.0,47.6534,-122.353,1400.0,1335.0,14.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2020.0,7070.0,1.0,0.0,0.0,5.0,7.0,1010.0,1010.0,47.5202,-122.378,1390.0,6000.0,56.0,0.0,0.0]},"out":{"variable":[379752.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,2070.0,5386.0,1.0,0.0,0.0,4.0,7.0,1140.0,930.0,47.6896,-122.374,1770.0,5386.0,66.0,0.0,0.0]},"out":{"variable":[628260.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2570.0,7980.0,2.0,0.0,0.0,3.0,9.0,2570.0,0.0,47.6378,-122.065,2760.0,6866.0,16.0,0.0,0.0]},"out":{"variable":[758714.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1290.0,3140.0,2.0,0.0,0.0,3.0,7.0,1290.0,0.0,47.6971,-122.026,1290.0,2628.0,6.0,0.0,0.0]},"out":{"variable":[400561.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.0,2230.0,12968.0,2.0,0.0,0.0,3.0,9.0,2230.0,0.0,47.6271,-122.197,2260.0,10160.0,25.0,0.0,0.0]},"out":{"variable":[757403.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1110.0,9762.0,1.0,0.0,0.0,4.0,7.0,1110.0,0.0,47.676,-122.15,1900.0,9720.0,51.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1220.0,1086.0,3.0,0.0,0.0,3.0,8.0,1220.0,0.0,47.7049,-122.353,1220.0,1422.0,7.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2160.0,3139.0,1.0,0.0,0.0,3.0,7.0,1080.0,1080.0,47.6662,-122.338,1650.0,3740.0,74.0,0.0,0.0]},"out":{"variable":[673288.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,4700.0,38412.0,2.0,0.0,0.0,3.0,10.0,3420.0,1280.0,47.6445,-122.167,3640.0,35571.0,36.0,0.0,0.0]},"out":{"variable":[1164589.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1880.0,4499.0,2.0,0.0,0.0,3.0,8.0,1880.0,0.0,47.5664,-121.999,2130.0,5114.0,22.0,0.0,0.0]},"out":{"variable":[553463.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2160.0,15817.0,2.0,0.0,0.0,3.0,8.0,2160.0,0.0,47.4166,-122.183,1990.0,15817.0,16.0,0.0,0.0]},"out":{"variable":[404551.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.75,2340.0,16500.0,1.0,0.0,0.0,4.0,8.0,1500.0,840.0,47.5952,-122.051,2210.0,15251.0,42.0,0.0,0.0]},"out":{"variable":[687786.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,960.0,1829.0,2.0,0.0,0.0,3.0,7.0,960.0,0.0,47.6032,-122.308,1470.0,1829.0,10.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1270.0,1062.0,2.0,0.0,0.0,3.0,8.0,1060.0,210.0,47.6568,-122.321,1260.0,1112.0,7.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2560.0,5800.0,2.0,0.0,0.0,3.0,9.0,2560.0,0.0,47.3474,-122.025,3040.0,5800.0,10.0,0.0,0.0]},"out":{"variable":[464060.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1090.0,4000.0,1.5,0.0,0.0,4.0,7.0,1090.0,0.0,47.6846,-122.386,1520.0,4000.0,70.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1610.0,14000.0,1.0,0.0,0.0,4.0,7.0,1050.0,560.0,47.3429,-122.036,1550.0,10080.0,38.0,0.0,0.0]},"out":{"variable":[276709.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1578.0,7340.0,2.0,0.0,0.0,3.0,7.0,1578.0,0.0,47.3771,-122.186,1850.0,7200.0,4.0,0.0,0.0]},"out":{"variable":[284081.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1370.0,22326.0,2.0,0.0,0.0,3.0,7.0,1370.0,0.0,47.4469,-121.775,1580.0,10920.0,21.0,0.0,0.0]},"out":{"variable":[252192.89]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1780.0,14810.0,1.0,0.0,0.0,4.0,8.0,1180.0,600.0,47.3581,-122.288,1450.0,6728.0,65.0,0.0,0.0]},"out":{"variable":[281823.16]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1630.0,15600.0,1.0,0.0,0.0,3.0,7.0,1630.0,0.0,47.68,-122.165,1830.0,10850.0,56.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1100.0,17334.0,1.0,0.0,0.0,3.0,7.0,1100.0,0.0,47.3003,-122.27,1530.0,18694.0,36.0,0.0,0.0]},"out":{"variable":[238078.05]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,950.0,11835.0,1.0,0.0,0.0,3.0,5.0,950.0,0.0,47.7494,-122.237,1690.0,12586.0,82.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.0,1390.0,1080.0,2.0,0.0,0.0,3.0,7.0,1140.0,250.0,47.5325,-122.282,1450.0,1461.0,9.0,0.0,0.0]},"out":{"variable":[271309.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2150.0,13789.0,1.0,0.0,0.0,4.0,8.0,1610.0,540.0,47.7591,-122.295,2150.0,15480.0,48.0,0.0,0.0]},"out":{"variable":[508926.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.0,3920.0,13085.0,2.0,1.0,4.0,4.0,11.0,3920.0,0.0,47.5716,-122.204,3450.0,13287.0,18.0,0.0,0.0]},"out":{"variable":[1452224.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2720.0,8000.0,1.0,0.0,0.0,4.0,7.0,1360.0,1360.0,47.5237,-122.391,1790.0,8000.0,60.0,0.0,0.0]},"out":{"variable":[434958.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1200.0,9085.0,1.0,0.0,0.0,4.0,7.0,1200.0,0.0,47.2795,-122.353,1200.0,9085.0,46.0,0.0,0.0]},"out":{"variable":[241330.19]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[1.0,1.0,1310.0,8667.0,1.5,0.0,0.0,1.0,6.0,1310.0,0.0,47.6059,-122.313,1130.0,4800.0,96.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2400.0,3844.0,1.0,0.0,0.0,5.0,7.0,1200.0,1200.0,47.7027,-122.347,1100.0,3844.0,40.0,0.0,0.0]},"out":{"variable":[712309.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,2160.0,15788.0,1.0,0.0,0.0,3.0,8.0,2160.0,0.0,47.6227,-122.207,2260.0,9787.0,63.0,0.0,0.0]},"out":{"variable":[673288.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2280.0,15347.0,1.0,0.0,0.0,5.0,7.0,2280.0,0.0,47.5218,-122.164,2280.0,15347.0,54.0,0.0,0.0]},"out":{"variable":[416617.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.25,3230.0,7800.0,2.0,0.0,3.0,3.0,10.0,3230.0,0.0,47.6348,-122.403,3030.0,6600.0,9.0,0.0,0.0]},"out":{"variable":[1077279.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2210.0,8465.0,1.0,0.0,0.0,3.0,8.0,1490.0,720.0,47.2647,-122.221,2210.0,7917.0,24.0,0.0,0.0]},"out":{"variable":[338137.97]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2380.0,16236.0,1.0,0.0,0.0,3.0,7.0,1540.0,840.0,47.6126,-122.12,2230.0,8925.0,53.0,0.0,0.0]},"out":{"variable":[701940.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1080.0,4000.0,1.0,0.0,0.0,3.0,7.0,1080.0,0.0,47.6902,-122.387,1530.0,4240.0,75.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2950.0,10254.0,2.0,0.0,0.0,3.0,9.0,2950.0,0.0,47.4888,-122.14,2800.0,9323.0,8.0,0.0,0.0]},"out":{"variable":[523152.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2100.0,5060.0,2.0,0.0,0.0,3.0,7.0,2100.0,0.0,47.563,-122.298,1520.0,2468.0,8.0,0.0,0.0]},"out":{"variable":[642519.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.0,1540.0,115434.0,1.5,0.0,0.0,4.0,7.0,1540.0,0.0,47.4163,-122.22,2027.0,23522.0,91.0,0.0,0.0]},"out":{"variable":[301714.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,940.0,5000.0,1.0,0.0,0.0,3.0,7.0,880.0,60.0,47.6771,-122.398,1420.0,5000.0,74.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.25,1620.0,1841.0,2.0,0.0,0.0,3.0,8.0,1540.0,80.0,47.5483,-122.004,1530.0,1831.0,10.0,0.0,0.0]},"out":{"variable":[544392.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.5,3180.0,12528.0,2.0,0.0,1.0,4.0,9.0,2060.0,1120.0,47.7058,-122.379,2850.0,11410.0,36.0,0.0,0.0]},"out":{"variable":[944006.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1810.0,6583.0,1.0,0.0,0.0,3.0,7.0,1500.0,310.0,47.7273,-122.351,860.0,8670.0,47.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.0,3230.0,5167.0,1.5,0.0,0.0,4.0,8.0,2000.0,1230.0,47.7053,-122.34,1509.0,1626.0,106.0,0.0,0.0]},"out":{"variable":[756699.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1540.0,9154.0,1.0,0.0,0.0,3.0,8.0,1540.0,0.0,47.6207,-122.042,1990.0,10273.0,31.0,0.0,0.0]},"out":{"variable":[555231.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.5,3080.0,6495.0,2.0,0.0,3.0,3.0,11.0,2530.0,550.0,47.6321,-122.393,4120.0,8620.0,18.0,1.0,10.0]},"out":{"variable":[1122811.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1420.0,7420.0,1.5,0.0,0.0,3.0,6.0,1420.0,0.0,47.4949,-122.239,1290.0,6600.0,70.0,0.0,0.0]},"out":{"variable":[256630.31]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1020.0,5410.0,1.0,0.0,0.0,4.0,7.0,880.0,140.0,47.6924,-122.31,1580.0,5376.0,86.0,0.0,0.0]},"out":{"variable":[444933.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2480.0,6112.0,2.0,0.0,0.0,3.0,7.0,2480.0,0.0,47.4387,-122.114,3220.0,6727.0,10.0,0.0,0.0]},"out":{"variable":[400456.03]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1770.0,7251.0,1.0,0.0,0.0,4.0,8.0,1770.0,0.0,47.4087,-122.17,2560.0,7210.0,24.0,0.0,0.0]},"out":{"variable":[294203.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,820.0,5100.0,1.0,0.0,0.0,4.0,6.0,820.0,0.0,47.5175,-122.205,2270.0,5100.0,60.0,0.0,0.0]},"out":{"variable":[258377.03]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2420.0,7548.0,1.0,0.0,0.0,4.0,8.0,1370.0,1050.0,47.3112,-122.376,2150.0,8000.0,47.0,0.0,0.0]},"out":{"variable":[350835.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[6.0,4.5,3500.0,8504.0,2.0,0.0,0.0,3.0,7.0,3500.0,0.0,47.7349,-122.295,1550.0,8460.0,35.0,0.0,0.0]},"out":{"variable":[637376.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2701.0,5821.0,2.0,0.0,0.0,3.0,7.0,2701.0,0.0,47.2873,-122.177,2566.0,5843.0,1.0,0.0,0.0]},"out":{"variable":[371040.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1620.0,997.0,2.5,0.0,0.0,3.0,8.0,1540.0,80.0,47.54,-122.026,1620.0,1068.0,4.0,0.0,0.0]},"out":{"variable":[544392.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,2050.0,5000.0,2.0,0.0,0.0,4.0,8.0,1370.0,680.0,47.6235,-122.298,1720.0,5000.0,27.0,0.0,0.0]},"out":{"variable":[630865.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2400.0,7738.0,1.5,0.0,0.0,3.0,8.0,2400.0,0.0,47.4562,-122.33,2170.0,8452.0,50.0,0.0,0.0]},"out":{"variable":[424253.22]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,710.0,4618.0,1.0,0.0,1.0,3.0,5.0,710.0,0.0,47.64,-122.394,1810.0,4988.0,90.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1720.0,6000.0,1.0,0.0,2.0,3.0,7.0,1000.0,720.0,47.4999,-122.223,1690.0,6000.0,60.0,0.0,0.0]},"out":{"variable":[332793.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,4200.0,35267.0,2.0,0.0,0.0,3.0,11.0,4200.0,0.0,47.7108,-122.071,3540.0,22234.0,24.0,0.0,0.0]},"out":{"variable":[1181336.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,4160.0,47480.0,2.0,0.0,0.0,3.0,10.0,4160.0,0.0,47.7266,-122.115,3400.0,40428.0,19.0,0.0,0.0]},"out":{"variable":[1082353.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1500.0,7482.0,1.0,0.0,0.0,4.0,7.0,1210.0,290.0,47.4619,-122.187,1480.0,7308.0,46.0,0.0,0.0]},"out":{"variable":[265691.34]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1720.0,8100.0,2.0,0.0,0.0,3.0,8.0,1720.0,0.0,47.6746,-122.4,2210.0,8100.0,107.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1590.0,11745.0,1.0,0.0,0.0,3.0,7.0,1090.0,500.0,47.3553,-122.28,1540.0,12530.0,36.0,0.0,0.0]},"out":{"variable":[276046.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2860.0,4500.0,2.0,0.0,2.0,3.0,8.0,1980.0,880.0,47.6463,-122.371,2310.0,4500.0,99.0,0.0,0.0]},"out":{"variable":[721518.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2120.0,6710.0,1.0,0.0,0.0,5.0,7.0,1420.0,700.0,47.7461,-122.324,1880.0,6960.0,55.0,0.0,0.0]},"out":{"variable":[494083.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2660.0,4975.0,2.0,0.0,0.0,3.0,8.0,2660.0,0.0,47.5487,-122.272,1840.0,6653.0,0.0,0.0,0.0]},"out":{"variable":[718445.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.5,980.0,1020.0,3.0,0.0,0.0,3.0,8.0,980.0,0.0,47.6844,-122.387,980.0,1023.0,6.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2040.0,5616.0,2.0,0.0,0.0,3.0,8.0,2040.0,0.0,47.7737,-122.238,2380.0,4737.0,2.0,0.0,0.0]},"out":{"variable":[481460.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1360.0,9603.0,1.0,0.0,0.0,3.0,7.0,1360.0,0.0,47.3959,-122.309,2240.0,10605.0,52.0,0.0,0.0]},"out":{"variable":[257407.33]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.5,3450.0,28324.0,1.0,0.0,0.0,5.0,8.0,2350.0,1100.0,47.6991,-122.196,2640.0,14978.0,42.0,0.0,0.0]},"out":{"variable":[782434.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.75,1810.0,4220.0,2.0,0.0,0.0,3.0,8.0,1810.0,0.0,47.7177,-122.034,1350.0,4479.0,10.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,4.5,5770.0,10050.0,1.0,0.0,3.0,5.0,9.0,3160.0,2610.0,47.677,-122.275,2950.0,6700.0,65.0,0.0,0.0]},"out":{"variable":[1689843.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1720.0,6417.0,1.0,0.0,0.0,3.0,7.0,1720.0,0.0,47.7268,-122.311,1430.0,6240.0,61.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.0,1470.0,18581.0,1.5,0.0,0.0,3.0,6.0,1470.0,0.0,47.4336,-122.197,1770.0,18581.0,90.0,0.0,0.0]},"out":{"variable":[275884.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2340.0,10495.0,1.0,0.0,0.0,4.0,8.0,2340.0,0.0,47.5386,-122.226,3120.0,11068.0,47.0,0.0,0.0]},"out":{"variable":[704672.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.75,2080.0,8112.0,1.0,1.0,4.0,4.0,8.0,1040.0,1040.0,47.7134,-122.277,2030.0,8408.0,75.0,1.0,45.0]},"out":{"variable":[987157.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1360.0,16000.0,1.0,0.0,0.0,3.0,7.0,1360.0,0.0,47.7583,-122.306,1360.0,12939.0,36.0,0.0,0.0]},"out":{"variable":[342604.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,1080.0,6760.0,1.0,0.0,2.0,3.0,6.0,1080.0,0.0,47.7008,-122.386,2080.0,5800.0,91.0,0.0,0.0]},"out":{"variable":[392867.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.0,1750.0,68841.0,1.0,0.0,0.0,3.0,7.0,1750.0,0.0,47.4442,-122.081,1550.0,32799.0,72.0,0.0,0.0]},"out":{"variable":[303002.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[1.0,1.0,670.0,5750.0,1.0,0.0,0.0,3.0,7.0,670.0,0.0,47.5624,-122.394,1170.0,5750.0,73.0,1.0,69.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.0,2990.0,9773.0,2.0,0.0,0.0,4.0,8.0,2990.0,0.0,47.6344,-122.174,2230.0,11553.0,42.0,0.0,0.0]},"out":{"variable":[713485.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1360.0,9688.0,1.0,0.0,0.0,4.0,7.0,1360.0,0.0,47.2574,-122.31,1390.0,9685.0,31.0,0.0,0.0]},"out":{"variable":[247009.34]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.0,2500.0,35802.0,1.5,0.0,0.0,3.0,7.0,2500.0,0.0,47.6488,-122.153,2880.0,40510.0,59.0,0.0,0.0]},"out":{"variable":[713003.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2300.0,41900.0,1.0,0.0,0.0,4.0,8.0,1310.0,990.0,47.477,-122.292,1160.0,8547.0,76.0,0.0,0.0]},"out":{"variable":[430252.34]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,5403.0,24069.0,2.0,1.0,4.0,4.0,12.0,5403.0,0.0,47.4169,-122.348,3980.0,104374.0,39.0,0.0,0.0]},"out":{"variable":[1946437.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1530.0,4944.0,1.0,0.0,0.0,3.0,7.0,1530.0,0.0,47.6857,-122.341,1500.0,4944.0,64.0,0.0,0.0]},"out":{"variable":[550275.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.75,1120.0,758.0,2.0,0.0,0.0,3.0,7.0,1120.0,0.0,47.5325,-122.072,1150.0,758.0,2.0,0.0,0.0]},"out":{"variable":[247726.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2350.0,6958.0,2.0,0.0,0.0,3.0,9.0,2350.0,0.0,47.3321,-122.172,2480.0,6395.0,16.0,0.0,0.0]},"out":{"variable":[461279.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.0,2300.0,7897.0,2.5,0.0,0.0,4.0,8.0,2300.0,0.0,47.7556,-122.356,2030.0,7902.0,59.0,0.0,0.0]},"out":{"variable":[523576.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2130.0,9100.0,1.0,0.0,0.0,3.0,8.0,1290.0,840.0,47.3815,-122.169,1770.0,7700.0,36.0,0.0,0.0]},"out":{"variable":[334257.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1560.0,7000.0,1.0,0.0,0.0,4.0,7.0,1560.0,0.0,47.3355,-122.284,1560.0,7200.0,46.0,0.0,0.0]},"out":{"variable":[276709.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.5,2030.0,8400.0,1.0,0.0,0.0,3.0,7.0,1330.0,700.0,47.6713,-122.152,1920.0,8400.0,47.0,0.0,0.0]},"out":{"variable":[595497.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,660.0,6509.0,1.0,0.0,0.0,4.0,5.0,660.0,0.0,47.4938,-122.171,970.0,5713.0,63.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.0,3420.0,18129.0,2.0,0.0,0.0,3.0,9.0,2540.0,880.0,47.5333,-122.217,3750.0,16316.0,62.0,1.0,53.0]},"out":{"variable":[1325960.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2460.0,4399.0,2.0,0.0,0.0,3.0,7.0,2460.0,0.0,47.5415,-121.884,2060.0,4399.0,7.0,0.0,0.0]},"out":{"variable":[701940.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.25,4560.0,13363.0,1.0,0.0,4.0,3.0,11.0,2760.0,1800.0,47.6205,-122.214,4060.0,13362.0,20.0,0.0,0.0]},"out":{"variable":[2005883.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2670.0,189486.0,2.0,0.0,4.0,3.0,8.0,2670.0,0.0,47.2585,-122.061,2190.0,218610.0,42.0,0.0,0.0]},"out":{"variable":[495822.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1350.0,2325.0,3.0,0.0,0.0,3.0,7.0,1350.0,0.0,47.6965,-122.35,1520.0,1652.0,16.0,0.0,0.0]},"out":{"variable":[422481.38]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3670.0,54450.0,2.0,0.0,0.0,3.0,10.0,3670.0,0.0,47.6211,-122.016,2900.0,49658.0,15.0,0.0,0.0]},"out":{"variable":[921695.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.5,4200.0,5400.0,2.0,0.0,0.0,3.0,9.0,3140.0,1060.0,47.7077,-122.12,3300.0,5564.0,2.0,0.0,0.0]},"out":{"variable":[1052897.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,1810.0,9240.0,2.0,0.0,0.0,3.0,7.0,1810.0,0.0,47.4362,-122.187,1660.0,9240.0,54.0,0.0,0.0]},"out":{"variable":[303866.03]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,700.0,5829.0,1.0,0.0,0.0,3.0,6.0,700.0,0.0,47.6958,-122.357,1160.0,6700.0,70.0,0.0,0.0]},"out":{"variable":[432971.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3220.0,38448.0,2.0,0.0,0.0,3.0,10.0,3220.0,0.0,47.6854,-122.053,3090.0,38448.0,21.0,0.0,0.0]},"out":{"variable":[886958.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,1520.0,7983.0,1.0,0.0,0.0,5.0,7.0,1520.0,0.0,47.7357,-122.193,1520.0,7783.0,47.0,0.0,0.0]},"out":{"variable":[424966.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1910.0,5000.0,2.0,0.0,0.0,3.0,7.0,1910.0,0.0,47.3608,-122.036,2020.0,5000.0,9.0,0.0,0.0]},"out":{"variable":[296202.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2840.0,216493.0,2.0,0.0,0.0,3.0,9.0,2840.0,0.0,47.702,-121.892,2820.0,175111.0,23.0,0.0,0.0]},"out":{"variable":[765468.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.75,3430.0,15119.0,2.0,0.0,0.0,3.0,10.0,3430.0,0.0,47.5678,-122.032,3430.0,12045.0,17.0,0.0,0.0]},"out":{"variable":[919031.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1760.0,5390.0,1.0,0.0,0.0,3.0,8.0,1760.0,0.0,47.3482,-122.042,2310.0,5117.0,0.0,0.0,0.0]},"out":{"variable":[291239.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.25,1110.0,1290.0,3.0,0.0,0.0,3.0,8.0,1110.0,0.0,47.6968,-122.34,1360.0,1251.0,8.0,0.0,0.0]},"out":{"variable":[405552.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1350.0,941.0,3.0,0.0,0.0,3.0,9.0,1350.0,0.0,47.6265,-122.364,1640.0,1369.0,8.0,0.0,0.0]},"out":{"variable":[684577.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,2980.0,7000.0,2.0,0.0,3.0,3.0,10.0,2140.0,840.0,47.5933,-122.292,2200.0,4800.0,114.0,1.0,114.0]},"out":{"variable":[1156206.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.0,2260.0,1834.0,2.0,0.0,0.0,3.0,8.0,1660.0,600.0,47.6111,-122.308,2260.0,1834.0,12.0,0.0,0.0]},"out":{"variable":[682284.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.25,3400.0,7452.0,2.0,0.0,0.0,3.0,10.0,3400.0,0.0,47.6141,-122.136,2650.0,8749.0,15.0,0.0,0.0]},"out":{"variable":[911794.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,4.5,6380.0,88714.0,2.0,0.0,0.0,3.0,12.0,6380.0,0.0,47.5592,-122.015,3040.0,7113.0,8.0,0.0,0.0]},"out":{"variable":[1355747.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.75,3180.0,9889.0,2.0,0.0,0.0,3.0,9.0,2500.0,680.0,47.6853,-122.204,2910.0,8558.0,2.0,0.0,0.0]},"out":{"variable":[946050.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.0,3130.0,12381.0,2.0,0.0,0.0,3.0,9.0,3130.0,0.0,47.5599,-122.118,3250.0,10049.0,20.0,0.0,0.0]},"out":{"variable":[836480.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1470.0,7694.0,1.0,0.0,0.0,4.0,7.0,1470.0,0.0,47.3539,-122.054,1580.0,7480.0,23.0,0.0,0.0]},"out":{"variable":[259333.95]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.25,2360.0,3873.0,2.0,0.0,0.0,3.0,8.0,1990.0,370.0,47.5635,-122.299,1720.0,3071.0,8.0,0.0,0.0]},"out":{"variable":[713978.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,4.0,2790.0,16117.0,2.0,0.0,0.0,4.0,9.0,2790.0,0.0,47.6033,-122.155,2740.0,25369.0,15.0,0.0,0.0]},"out":{"variable":[765468.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2600.0,4506.0,2.0,0.0,0.0,3.0,9.0,2600.0,0.0,47.5146,-122.188,2470.0,6041.0,9.0,0.0,0.0]},"out":{"variable":[575285.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.5,3270.0,15704.0,2.0,0.0,0.0,3.0,9.0,2110.0,1160.0,47.6256,-122.042,3020.0,8582.0,24.0,0.0,0.0]},"out":{"variable":[846775.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2810.0,6146.0,2.0,0.0,0.0,3.0,9.0,2810.0,0.0,47.4045,-122.208,2810.0,6180.0,16.0,0.0,0.0]},"out":{"variable":[488736.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1480.0,5600.0,1.0,0.0,0.0,4.0,6.0,940.0,540.0,47.5045,-122.27,1350.0,11100.0,67.0,0.0,0.0]},"out":{"variable":[267890.16]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2320.0,9240.0,1.0,0.0,0.0,3.0,7.0,1160.0,1160.0,47.4909,-122.257,2130.0,7320.0,55.0,1.0,55.0]},"out":{"variable":[404676.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,1880.0,12150.0,1.0,0.0,0.0,3.0,7.0,1280.0,600.0,47.3272,-122.363,1980.0,9680.0,38.0,0.0,0.0]},"out":{"variable":[293560.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.5,1785.0,779.0,2.0,0.0,0.0,3.0,7.0,1595.0,190.0,47.5959,-122.198,1780.0,794.0,39.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2590.0,9530.0,1.0,0.0,0.0,4.0,8.0,1640.0,950.0,47.7752,-122.285,2710.0,10970.0,36.0,0.0,0.0]},"out":{"variable":[536371.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,1910.0,8775.0,1.0,0.0,0.0,3.0,7.0,1210.0,700.0,47.7396,-122.247,2210.0,8778.0,59.0,0.0,0.0]},"out":{"variable":[438821.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.5,1900.0,7843.0,2.0,0.0,0.0,5.0,7.0,1900.0,0.0,47.6273,-122.123,1900.0,7350.0,49.0,0.0,0.0]},"out":{"variable":[563844.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2440.0,5001.0,2.0,0.0,0.0,3.0,8.0,2440.0,0.0,47.5108,-122.193,2260.0,5001.0,9.0,0.0,0.0]},"out":{"variable":[444931.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1670.0,4005.0,1.5,0.0,0.0,4.0,7.0,1170.0,500.0,47.6878,-122.38,1240.0,4005.0,75.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2810.0,6481.0,2.0,0.0,0.0,3.0,9.0,2810.0,0.0,47.333,-122.172,2660.0,6958.0,17.0,0.0,0.0]},"out":{"variable":[475271.03]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[6.0,2.5,2590.0,10890.0,1.0,0.0,0.0,4.0,7.0,1340.0,1250.0,47.4126,-122.165,2474.0,10454.0,44.0,0.0,0.0]},"out":{"variable":[383253.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2200.0,6967.0,2.0,0.0,0.0,3.0,8.0,2200.0,0.0,47.7355,-122.202,1970.0,7439.0,29.0,0.0,0.0]},"out":{"variable":[519346.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1520.0,1304.0,2.0,0.0,0.0,3.0,8.0,1180.0,340.0,47.5446,-122.385,1270.0,1718.0,8.0,0.0,0.0]},"out":{"variable":[530271.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,1580.0,9600.0,1.0,0.0,0.0,5.0,7.0,1050.0,530.0,47.4091,-122.313,1710.0,9600.0,49.0,0.0,0.0]},"out":{"variable":[281058.72]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1160.0,5000.0,1.0,0.0,0.0,4.0,8.0,1160.0,0.0,47.6865,-122.399,1750.0,5000.0,77.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.75,2770.0,6116.0,1.0,0.0,0.0,3.0,7.0,1490.0,1280.0,47.4847,-122.291,1920.0,6486.0,35.0,0.0,0.0]},"out":{"variable":[429876.34]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1260.0,7897.0,1.0,0.0,0.0,3.0,7.0,1260.0,0.0,47.1946,-122.003,1560.0,8285.0,35.0,0.0,0.0]},"out":{"variable":[246088.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1700.0,8481.0,2.0,0.0,0.0,3.0,7.0,1700.0,0.0,47.2623,-122.305,1830.0,6600.0,21.0,0.0,0.0]},"out":{"variable":[290323.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1890.0,6962.0,2.0,0.0,0.0,3.0,8.0,1890.0,0.0,47.3328,-122.187,2170.0,6803.0,17.0,0.0,0.0]},"out":{"variable":[293560.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2070.0,8685.0,2.0,0.0,0.0,3.0,7.0,2070.0,0.0,47.4697,-122.267,2170.0,9715.0,9.0,0.0,0.0]},"out":{"variable":[380009.28]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1010.0,6000.0,1.0,0.0,0.0,4.0,6.0,1010.0,0.0,47.771,-122.353,1610.0,7313.0,70.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,1540.0,5920.0,1.5,0.0,0.0,5.0,7.0,1540.0,0.0,47.7148,-122.301,1630.0,6216.0,79.0,0.0,0.0]},"out":{"variable":[433900.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1553.0,1991.0,3.0,0.0,0.0,3.0,8.0,1553.0,0.0,47.7049,-122.34,1509.0,2431.0,0.0,0.0,0.0]},"out":{"variable":[449229.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.0,1820.0,5500.0,1.5,0.0,0.0,3.0,6.0,1820.0,0.0,47.5185,-122.363,1140.0,5500.0,68.0,0.0,0.0]},"out":{"variable":[322015.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1780.0,10196.0,1.0,0.0,0.0,4.0,7.0,1270.0,510.0,47.3375,-122.291,1320.0,7875.0,48.0,0.0,0.0]},"out":{"variable":[281823.16]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1760.0,4000.0,2.0,0.0,0.0,3.0,8.0,1760.0,0.0,47.6401,-122.32,1760.0,4000.0,92.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1628.0,286355.0,1.0,0.0,0.0,3.0,7.0,1628.0,0.0,47.2558,-122.122,1490.0,216344.0,19.0,0.0,0.0]},"out":{"variable":[274207.16]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1300.0,1331.0,3.0,0.0,0.0,3.0,8.0,1300.0,0.0,47.6607,-122.352,1450.0,5270.0,8.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2180.0,7700.0,1.0,0.0,0.0,3.0,8.0,1480.0,700.0,47.7594,-122.361,2180.0,7604.0,53.0,0.0,0.0]},"out":{"variable":[516278.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1500.0,1312.0,3.0,0.0,0.0,3.0,8.0,1500.0,0.0,47.6534,-122.346,1500.0,1282.0,7.0,0.0,0.0]},"out":{"variable":[536497.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.25,1450.0,1468.0,2.0,0.0,0.0,3.0,8.0,1100.0,350.0,47.5664,-122.37,1450.0,1478.0,5.0,0.0,0.0]},"out":{"variable":[451058.16]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1400.0,4800.0,1.0,0.0,0.0,3.0,7.0,1200.0,200.0,47.6865,-122.379,1440.0,3840.0,93.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2009.0,5000.0,2.0,0.0,0.0,3.0,8.0,2009.0,0.0,47.2577,-122.198,2009.0,5182.0,0.0,0.0,0.0]},"out":{"variable":[320863.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,4.25,4860.0,9453.0,1.5,0.0,1.0,5.0,10.0,3100.0,1760.0,47.6196,-122.286,3150.0,8557.0,109.0,0.0,0.0]},"out":{"variable":[1910823.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1780.0,5720.0,1.0,0.0,0.0,5.0,7.0,980.0,800.0,47.6794,-122.351,1620.0,5050.0,89.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,1.75,2180.0,9178.0,1.0,0.0,0.0,3.0,7.0,1140.0,1040.0,47.4364,-122.28,2140.0,9261.0,51.0,0.0,0.0]},"out":{"variable":[382005.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.75,2980.0,9838.0,1.0,0.0,0.0,3.0,7.0,1710.0,1270.0,47.3807,-122.222,2240.0,9838.0,47.0,0.0,0.0]},"out":{"variable":[379076.34]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1520.0,12282.0,1.0,0.0,0.0,3.0,8.0,1220.0,300.0,47.7516,-122.299,1860.0,13500.0,36.0,0.0,0.0]},"out":{"variable":[424966.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2630.0,5701.0,2.0,0.0,0.0,3.0,7.0,2630.0,0.0,47.375,-122.16,2770.0,5939.0,4.0,0.0,0.0]},"out":{"variable":[368504.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2270.0,11500.0,1.0,0.0,0.0,3.0,8.0,1540.0,730.0,47.7089,-122.241,2020.0,10918.0,47.0,0.0,0.0]},"out":{"variable":[625990.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.5,2380.0,8204.0,1.0,0.0,0.0,3.0,8.0,1540.0,840.0,47.7,-122.287,2270.0,8204.0,58.0,0.0,0.0]},"out":{"variable":[712309.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1500.0,7420.0,1.0,0.0,0.0,3.0,7.0,1000.0,500.0,47.7236,-122.174,1840.0,7272.0,42.0,0.0,0.0]},"out":{"variable":[419677.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1620.0,12825.0,1.0,0.0,0.0,3.0,8.0,1340.0,280.0,47.3321,-122.323,2076.0,11200.0,53.0,0.0,0.0]},"out":{"variable":[284081.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1580.0,5000.0,1.0,0.0,0.0,3.0,8.0,1290.0,290.0,47.687,-122.386,1570.0,4500.0,75.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1590.0,8911.0,1.0,0.0,0.0,3.0,7.0,1590.0,0.0,47.7394,-122.252,1590.0,9625.0,58.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,1.75,2660.0,10637.0,1.5,0.0,0.0,5.0,7.0,1670.0,990.0,47.6945,-122.292,1570.0,6825.0,92.0,0.0,0.0]},"out":{"variable":[716776.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1780.0,16000.0,1.0,0.0,0.0,2.0,7.0,1240.0,540.0,47.419,-122.322,1860.0,9775.0,54.0,0.0,0.0]},"out":{"variable":[308049.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.75,2600.0,12860.0,1.0,0.0,0.0,3.0,7.0,1350.0,1250.0,47.695,-121.918,2260.0,12954.0,49.0,0.0,0.0]},"out":{"variable":[703282.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1460.0,2610.0,2.0,0.0,0.0,3.0,8.0,1460.0,0.0,47.6864,-122.345,1320.0,3000.0,27.0,0.0,0.0]},"out":{"variable":[498579.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2790.0,5450.0,2.0,0.0,0.0,3.0,10.0,1930.0,860.0,47.6453,-122.303,2320.0,5450.0,89.0,1.0,75.0]},"out":{"variable":[1097757.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2370.0,9619.0,1.0,0.0,0.0,4.0,8.0,1650.0,720.0,47.6366,-122.099,1960.0,9712.0,41.0,0.0,0.0]},"out":{"variable":[701940.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2340.0,8248.0,2.0,0.0,0.0,3.0,8.0,2340.0,0.0,47.6314,-122.03,2140.0,9963.0,25.0,0.0,0.0]},"out":{"variable":[687786.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,3280.0,79279.0,1.0,0.0,0.0,3.0,10.0,3280.0,0.0,47.3207,-122.293,1860.0,24008.0,13.0,0.0,0.0]},"out":{"variable":[634865.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3260.0,5974.0,2.0,0.0,1.0,3.0,9.0,2820.0,440.0,47.6772,-122.267,2260.0,6780.0,8.0,0.0,0.0]},"out":{"variable":[947562.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2180.0,4431.0,1.5,0.0,0.0,3.0,8.0,2020.0,160.0,47.636,-122.302,1890.0,4400.0,103.0,0.0,0.0]},"out":{"variable":[675545.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.0,2110.0,7794.0,1.0,0.0,0.0,3.0,6.0,2110.0,0.0,47.4005,-122.293,1330.0,10044.0,33.0,0.0,0.0]},"out":{"variable":[349668.47]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1960.0,1585.0,2.0,0.0,0.0,3.0,7.0,1750.0,210.0,47.5414,-122.288,1760.0,1958.0,11.0,0.0,0.0]},"out":{"variable":[559453.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,3190.0,52953.0,2.0,0.0,0.0,4.0,10.0,3190.0,0.0,47.5933,-122.075,3190.0,51400.0,35.0,0.0,0.0]},"out":{"variable":[886958.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1740.0,18000.0,1.0,0.0,0.0,3.0,7.0,1230.0,510.0,47.7397,-122.242,1740.0,11250.0,25.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,2060.0,15050.0,1.5,0.0,0.0,4.0,7.0,2060.0,0.0,47.3321,-122.373,1900.0,15674.0,76.0,0.0,0.0]},"out":{"variable":[355736.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2590.0,7720.0,2.0,0.0,0.0,3.0,9.0,2590.0,0.0,47.659,-122.146,2600.0,9490.0,26.0,0.0,0.0]},"out":{"variable":[758714.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.0,1900.0,6000.0,1.5,0.0,0.0,4.0,6.0,1900.0,0.0,47.6831,-122.186,2110.0,8400.0,95.0,0.0,0.0]},"out":{"variable":[563844.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2590.0,35640.0,1.0,0.0,0.0,3.0,9.0,2590.0,0.0,47.5516,-122.03,2590.0,31200.0,28.0,0.0,0.0]},"out":{"variable":[758714.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1820.0,78408.0,1.0,0.0,0.0,3.0,6.0,1220.0,600.0,47.3364,-122.128,1340.0,78408.0,65.0,0.0,0.0]},"out":{"variable":[281823.16]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.75,2330.0,10143.0,1.0,0.0,2.0,4.0,7.0,1220.0,1110.0,47.4899,-122.359,2560.0,9750.0,61.0,0.0,0.0]},"out":{"variable":[422508.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,3520.0,4933.0,1.5,0.0,0.0,4.0,8.0,2270.0,1250.0,47.5655,-122.315,1710.0,5400.0,86.0,0.0,0.0]},"out":{"variable":[784103.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1400.0,1581.0,1.5,0.0,0.0,5.0,8.0,1400.0,0.0,47.6221,-122.314,1860.0,3861.0,105.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1940.0,6386.0,1.0,0.0,0.0,3.0,7.0,1140.0,800.0,47.5533,-122.285,1340.0,6165.0,60.0,0.0,0.0]},"out":{"variable":[558381.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3620.0,42580.0,2.0,0.0,0.0,3.0,10.0,3620.0,0.0,47.7204,-122.115,2950.0,33167.0,30.0,0.0,0.0]},"out":{"variable":[921695.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,4.0,4620.0,130208.0,2.0,0.0,0.0,3.0,10.0,4620.0,0.0,47.5885,-121.939,4620.0,131007.0,1.0,0.0,0.0]},"out":{"variable":[1164589.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1560.0,35026.0,1.0,0.0,0.0,3.0,7.0,1290.0,270.0,47.3023,-122.069,1660.0,35160.0,30.0,0.0,0.0]},"out":{"variable":[278759.22]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2770.0,63118.0,2.0,0.0,0.0,3.0,9.0,2770.0,0.0,47.6622,-121.961,2770.0,44224.0,17.0,0.0,0.0]},"out":{"variable":[765468.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1660.0,10763.0,2.0,0.0,0.0,3.0,7.0,1660.0,0.0,47.3812,-122.029,2010.0,7983.0,20.0,0.0,0.0]},"out":{"variable":[284845.38]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1070.0,8130.0,1.0,0.0,0.0,3.0,7.0,1070.0,0.0,47.697,-122.37,1360.0,7653.0,73.0,0.0,0.0]},"out":{"variable":[403520.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2420.0,4750.0,2.0,0.0,0.0,3.0,8.0,2420.0,0.0,47.3663,-122.122,2690.0,4750.0,12.0,0.0,0.0]},"out":{"variable":[350835.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,560.0,7560.0,1.0,0.0,0.0,3.0,6.0,560.0,0.0,47.5271,-122.375,990.0,7560.0,70.0,0.0,0.0]},"out":{"variable":[253679.61]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.0,1651.0,18200.0,1.0,0.0,0.0,3.0,6.0,1651.0,0.0,47.4621,-122.461,1510.0,89595.0,68.0,0.0,0.0]},"out":{"variable":[316231.78]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1000.0,16376.0,1.0,0.0,0.0,3.0,7.0,1000.0,0.0,47.4825,-122.108,1420.0,16192.0,55.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,3340.0,10422.0,2.0,0.0,0.0,3.0,10.0,3340.0,0.0,47.6515,-122.197,1770.0,9490.0,18.0,0.0,0.0]},"out":{"variable":[1103101.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.5,3760.0,10207.0,2.0,0.0,0.0,3.0,10.0,3150.0,610.0,47.5605,-122.225,3550.0,12118.0,46.0,0.0,0.0]},"out":{"variable":[1489624.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2020.0,3600.0,2.0,0.0,0.0,3.0,8.0,2020.0,0.0,47.6678,-122.165,2070.0,3699.0,16.0,0.0,0.0]},"out":{"variable":[583765.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,1540.0,5120.0,1.0,0.0,0.0,5.0,6.0,770.0,770.0,47.5359,-122.372,1080.0,5120.0,71.0,0.0,0.0]},"out":{"variable":[538436.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2330.0,7642.0,1.0,0.0,0.0,3.0,8.0,1800.0,530.0,47.2946,-122.37,2320.0,7933.0,24.0,0.0,0.0]},"out":{"variable":[348323.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.5,2700.0,11675.0,1.5,0.0,2.0,4.0,6.0,1950.0,750.0,47.7172,-122.349,2160.0,8114.0,66.0,0.0,0.0]},"out":{"variable":[559923.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,700.0,8100.0,1.0,0.0,0.0,3.0,6.0,700.0,0.0,47.7492,-122.311,1230.0,8100.0,65.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,1890.0,4500.0,1.5,0.0,0.0,3.0,7.0,1490.0,400.0,47.6684,-122.356,1640.0,4010.0,108.0,1.0,86.0]},"out":{"variable":[562676.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.25,1061.0,2884.0,2.0,0.0,0.0,3.0,7.0,1061.0,0.0,47.346,-122.218,1481.0,2887.0,2.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2060.0,5649.0,1.0,0.0,0.0,5.0,8.0,1360.0,700.0,47.6496,-122.407,2060.0,5626.0,73.0,0.0,0.0]},"out":{"variable":[630865.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1390.0,10530.0,1.0,0.0,0.0,3.0,7.0,1390.0,0.0,47.7025,-122.196,1750.0,10530.0,54.0,0.0,0.0]},"out":{"variable":[376762.28]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1730.0,3600.0,2.0,0.0,0.0,3.0,8.0,1730.0,0.0,47.5014,-122.34,2030.0,3600.0,0.0,0.0,0.0]},"out":{"variable":[317132.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1550.0,7713.0,1.0,0.0,0.0,3.0,7.0,1550.0,0.0,47.7005,-122.358,1340.0,6350.0,84.0,1.0,49.0]},"out":{"variable":[465299.84]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1840.0,10125.0,1.0,0.0,0.0,4.0,8.0,1220.0,620.0,47.5607,-122.217,2320.0,10160.0,55.0,0.0,0.0]},"out":{"variable":[546632.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,630.0,6000.0,1.0,0.0,0.0,3.0,6.0,630.0,0.0,47.4973,-122.221,1470.0,6840.0,71.0,1.0,62.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1620.0,8125.0,2.0,0.0,0.0,4.0,7.0,1620.0,0.0,47.6255,-122.059,1480.0,8120.0,32.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1760.0,12874.0,1.0,0.0,0.0,4.0,7.0,1230.0,530.0,47.5906,-122.167,1950.0,10240.0,47.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1230.0,11601.0,1.0,0.0,0.0,4.0,7.0,910.0,320.0,47.1955,-121.991,1820.0,8465.0,23.0,0.0,0.0]},"out":{"variable":[253408.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1460.0,7800.0,1.0,0.0,0.0,2.0,7.0,1040.0,420.0,47.3035,-122.382,1310.0,7865.0,36.0,0.0,0.0]},"out":{"variable":[300480.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.0,1494.0,19271.0,2.0,1.0,4.0,3.0,7.0,1494.0,0.0,47.4728,-122.497,1494.0,43583.0,71.0,1.0,54.0]},"out":{"variable":[359947.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,3340.0,5677.0,2.0,0.0,0.0,3.0,9.0,3340.0,0.0,47.709,-122.118,3240.0,5643.0,4.0,0.0,0.0]},"out":{"variable":[857574.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2930.0,5973.0,2.0,0.0,0.0,3.0,10.0,2930.0,0.0,47.3846,-122.186,3038.0,7095.0,7.0,0.0,0.0]},"out":{"variable":[594678.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1310.0,18135.0,1.0,0.0,0.0,3.0,7.0,1310.0,0.0,47.6065,-122.113,2150.0,18135.0,67.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.75,1260.0,5300.0,1.0,0.0,0.0,4.0,7.0,840.0,420.0,47.6809,-122.348,1280.0,3000.0,64.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1000.0,10560.0,1.0,0.0,0.0,3.0,7.0,1000.0,0.0,47.3217,-122.317,1190.0,9375.0,60.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1790.0,6800.0,1.0,0.0,0.0,4.0,7.0,1240.0,550.0,47.728,-122.339,1470.0,6800.0,51.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.5,1830.0,1988.0,2.0,0.0,0.0,3.0,9.0,1530.0,300.0,47.5779,-122.409,1800.0,2467.0,3.0,0.0,0.0]},"out":{"variable":[725184.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2240.0,8664.0,1.0,0.0,0.0,5.0,8.0,1470.0,770.0,47.6621,-122.189,2210.0,8860.0,39.0,0.0,0.0]},"out":{"variable":[682284.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,1320.0,10800.0,1.0,0.0,0.0,4.0,8.0,1320.0,0.0,47.7145,-122.367,2120.0,12040.0,67.0,0.0,0.0]},"out":{"variable":[341649.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1610.0,8500.0,1.5,0.0,0.0,3.0,7.0,1610.0,0.0,47.3717,-122.297,1070.0,8750.0,56.0,0.0,0.0]},"out":{"variable":[274207.16]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1390.0,13200.0,2.0,0.0,0.0,3.0,7.0,1390.0,0.0,47.4429,-121.771,1430.0,10725.0,35.0,0.0,0.0]},"out":{"variable":[252539.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1430.0,3455.0,1.0,0.0,0.0,3.0,7.0,980.0,450.0,47.6873,-122.336,1450.0,4599.0,67.0,0.0,0.0]},"out":{"variable":[453195.78]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2350.0,7210.0,1.5,0.0,0.0,4.0,7.0,2350.0,0.0,47.7407,-122.183,1930.0,7519.0,42.0,0.0,0.0]},"out":{"variable":[529302.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1750.0,6397.0,2.0,0.0,0.0,3.0,7.0,1750.0,0.0,47.3082,-122.358,1940.0,6502.0,27.0,0.0,0.0]},"out":{"variable":[291239.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1690.0,4500.0,1.5,0.0,1.0,4.0,8.0,1690.0,0.0,47.5841,-122.383,2140.0,7200.0,86.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1260.0,8614.0,1.0,0.0,0.0,4.0,7.0,1260.0,0.0,47.3586,-122.056,1600.0,8614.0,29.0,0.0,0.0]},"out":{"variable":[246525.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1480.0,8381.0,1.0,0.0,0.0,4.0,7.0,1480.0,0.0,47.7078,-122.288,1710.0,8050.0,46.0,0.0,0.0]},"out":{"variable":[421153.03]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.5,3660.0,4903.0,2.0,0.0,0.0,3.0,9.0,2760.0,900.0,47.7184,-122.156,3630.0,4992.0,0.0,0.0,0.0]},"out":{"variable":[873848.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,2180.0,6000.0,1.5,0.0,0.0,3.0,8.0,2060.0,120.0,47.5958,-122.306,1370.0,4500.0,88.0,0.0,0.0]},"out":{"variable":[675545.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.75,1670.0,6460.0,1.0,0.0,0.0,3.0,8.0,1670.0,0.0,47.7123,-122.027,2170.0,6254.0,10.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,970.0,7503.0,1.0,0.0,0.0,4.0,6.0,970.0,0.0,47.4688,-122.163,1230.0,9504.0,48.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1780.0,6840.0,2.0,0.0,0.0,4.0,6.0,1780.0,0.0,47.727,-122.3,1410.0,7200.0,67.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1720.0,4050.0,1.0,0.0,0.0,5.0,7.0,860.0,860.0,47.6782,-122.314,1720.0,4410.0,108.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1680.0,8100.0,1.0,0.0,2.0,3.0,8.0,1680.0,0.0,47.7212,-122.364,1880.0,7750.0,65.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2714.0,17936.0,2.0,0.0,0.0,3.0,9.0,2714.0,0.0,47.3185,-122.275,2590.0,18386.0,10.0,0.0,0.0]},"out":{"variable":[487028.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1700.0,1481.0,3.0,0.0,0.0,3.0,8.0,1700.0,0.0,47.6598,-122.349,1560.0,1350.0,12.0,0.0,0.0]},"out":{"variable":[557391.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,1870.0,6000.0,1.5,0.0,0.0,3.0,7.0,1870.0,0.0,47.7155,-122.315,1520.0,7169.0,58.0,0.0,0.0]},"out":{"variable":[442856.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2030.0,5754.0,2.0,0.0,0.0,3.0,8.0,2030.0,0.0,47.3542,-122.137,2030.0,5784.0,14.0,0.0,0.0]},"out":{"variable":[327625.28]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2420.0,10603.0,1.0,0.0,0.0,5.0,7.0,1210.0,1210.0,47.7397,-122.259,1750.0,10800.0,56.0,0.0,0.0]},"out":{"variable":[530288.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1340.0,4320.0,1.0,0.0,0.0,3.0,5.0,920.0,420.0,47.299,-122.228,980.0,6480.0,102.0,1.0,81.0]},"out":{"variable":[244380.27]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2230.0,3939.0,2.0,0.0,0.0,3.0,8.0,2230.0,0.0,47.73,-122.335,2230.0,4200.0,1.0,0.0,0.0]},"out":{"variable":[519346.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,1860.0,12197.0,1.0,0.0,0.0,3.0,7.0,1860.0,0.0,47.729,-122.235,1510.0,11761.0,50.0,0.0,0.0]},"out":{"variable":[438514.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.75,1120.0,881.0,3.0,0.0,0.0,3.0,8.0,1120.0,0.0,47.6914,-122.343,1120.0,1087.0,15.0,0.0,0.0]},"out":{"variable":[446768.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2340.0,5940.0,1.0,0.0,0.0,3.0,8.0,1290.0,1050.0,47.6789,-122.281,1930.0,5940.0,61.0,0.0,0.0]},"out":{"variable":[689450.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1970.0,35100.0,2.0,0.0,0.0,4.0,9.0,1970.0,0.0,47.4635,-121.991,2340.0,35100.0,37.0,0.0,0.0]},"out":{"variable":[484757.22]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.5,2620.0,8050.0,1.0,0.0,0.0,3.0,8.0,1520.0,1100.0,47.6919,-122.115,2030.0,7676.0,39.0,0.0,0.0]},"out":{"variable":[706407.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1960.0,7230.0,2.0,0.0,0.0,3.0,8.0,1960.0,0.0,47.2855,-122.36,1850.0,7208.0,12.0,0.0,0.0]},"out":{"variable":[317765.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1190.0,1022.0,3.0,0.0,0.0,3.0,8.0,1190.0,0.0,47.6972,-122.349,1210.0,1171.0,17.0,0.0,0.0]},"out":{"variable":[400561.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2130.0,11900.0,2.0,0.0,0.0,3.0,9.0,2130.0,0.0,47.6408,-122.058,2590.0,11900.0,38.0,0.0,0.0]},"out":{"variable":[730767.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2420.0,8400.0,1.0,0.0,0.0,5.0,7.0,1620.0,800.0,47.5017,-122.165,1660.0,8400.0,50.0,0.0,0.0]},"out":{"variable":[411000.16]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2230.0,8560.0,2.0,0.0,0.0,3.0,8.0,2230.0,0.0,47.4877,-122.143,2400.0,7756.0,12.0,0.0,0.0]},"out":{"variable":[429339.13]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.5,1690.0,1765.0,2.0,0.0,0.0,3.0,8.0,1370.0,320.0,47.6536,-122.34,1690.0,1694.0,8.0,0.0,0.0]},"out":{"variable":[558463.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,4060.0,8547.0,2.0,0.0,0.0,3.0,9.0,2790.0,1270.0,47.3694,-122.056,2810.0,8313.0,7.0,0.0,0.0]},"out":{"variable":[634262.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,1620.0,8400.0,1.0,0.0,0.0,3.0,7.0,1180.0,440.0,47.6574,-122.128,2120.0,8424.0,46.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2130.0,8499.0,1.0,0.0,0.0,4.0,7.0,1600.0,530.0,47.3657,-122.21,1890.0,11368.0,39.0,0.0,0.0]},"out":{"variable":[334257.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2100.0,4440.0,2.0,0.0,4.0,3.0,7.0,2100.0,0.0,47.5865,-122.397,2100.0,6000.0,70.0,0.0,0.0]},"out":{"variable":[672052.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,1.0,1460.0,11726.0,1.5,0.0,0.0,3.0,6.0,1290.0,170.0,47.5039,-122.361,1460.0,10450.0,78.0,0.0,0.0]},"out":{"variable":[266253.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1210.0,8400.0,1.0,0.0,0.0,3.0,8.0,780.0,430.0,47.6503,-122.393,1860.0,6000.0,14.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,910.0,6490.0,1.0,0.0,0.0,3.0,7.0,910.0,0.0,47.6892,-122.306,1040.0,6490.0,72.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2790.0,5423.0,2.0,0.0,0.0,3.0,9.0,2790.0,0.0,47.6085,-122.017,2450.0,6453.0,15.0,0.0,0.0]},"out":{"variable":[765468.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1810.0,1585.0,3.0,0.0,0.0,3.0,7.0,1810.0,0.0,47.6957,-122.376,1560.0,1586.0,1.0,0.0,0.0]},"out":{"variable":[502022.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,3.5,1970.0,5079.0,2.0,0.0,0.0,3.0,8.0,1680.0,290.0,47.5816,-122.296,1940.0,6000.0,7.0,0.0,0.0]},"out":{"variable":[572710.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2641.0,8615.0,2.0,0.0,0.0,3.0,7.0,2641.0,0.0,47.4038,-122.213,2641.0,8091.0,16.0,0.0,0.0]},"out":{"variable":[384215.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1150.0,3000.0,1.0,0.0,0.0,5.0,6.0,1150.0,0.0,47.6867,-122.345,1460.0,3200.0,108.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,4470.0,60373.0,2.0,0.0,0.0,3.0,11.0,4470.0,0.0,47.7289,-122.127,3210.0,40450.0,26.0,0.0,0.0]},"out":{"variable":[1208638.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,1720.0,8750.0,1.0,0.0,0.0,3.0,7.0,860.0,860.0,47.726,-122.21,1790.0,8750.0,43.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2830.0,6000.0,1.0,0.0,3.0,3.0,9.0,1730.0,1100.0,47.5751,-122.378,2040.0,5300.0,60.0,0.0,0.0]},"out":{"variable":[981676.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,1070.0,1236.0,2.0,0.0,0.0,3.0,8.0,1000.0,70.0,47.5619,-122.382,1170.0,1888.0,10.0,0.0,0.0]},"out":{"variable":[435628.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.5,1090.0,1183.0,3.0,0.0,0.0,3.0,8.0,1090.0,0.0,47.6974,-122.349,1110.0,1384.0,6.0,0.0,0.0]},"out":{"variable":[400561.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,3270.0,6027.0,2.0,0.0,0.0,3.0,10.0,3270.0,0.0,47.6346,-122.063,3270.0,6546.0,13.0,0.0,0.0]},"out":{"variable":[889377.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.0,2410.0,8284.0,1.0,0.0,0.0,5.0,7.0,1210.0,1200.0,47.7202,-122.22,2050.0,7940.0,45.0,0.0,0.0]},"out":{"variable":[532234.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2490.0,12929.0,2.0,0.0,0.0,3.0,9.0,2490.0,0.0,47.6161,-122.021,2440.0,12929.0,31.0,0.0,0.0]},"out":{"variable":[758714.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2050.0,4080.0,2.0,0.0,0.0,3.0,8.0,2050.0,0.0,47.6698,-122.325,1890.0,4080.0,23.0,0.0,0.0]},"out":{"variable":[630865.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2540.0,5237.0,2.0,0.0,0.0,3.0,8.0,2540.0,0.0,47.5492,-122.276,1800.0,4097.0,3.0,0.0,0.0]},"out":{"variable":[713978.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1850.0,8667.0,1.0,0.0,0.0,4.0,7.0,880.0,970.0,47.7025,-122.198,1450.0,10530.0,32.0,0.0,0.0]},"out":{"variable":[467484.22]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2960.0,8330.0,1.0,0.0,3.0,4.0,10.0,2260.0,700.0,47.7035,-122.385,2960.0,8840.0,62.0,0.0,0.0]},"out":{"variable":[1178314.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,1670.0,13125.0,1.0,0.0,0.0,5.0,8.0,1670.0,0.0,47.6315,-122.101,2360.0,12500.0,42.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2240.0,5500.0,2.0,0.0,0.0,3.0,8.0,2240.0,0.0,47.496,-122.169,700.0,5500.0,1.0,0.0,0.0]},"out":{"variable":[426066.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1850.0,7151.0,2.0,0.0,0.0,3.0,7.0,1850.0,0.0,47.2843,-122.352,1710.0,6827.0,26.0,0.0,0.0]},"out":{"variable":[285870.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1330.0,9548.0,1.0,0.0,0.0,5.0,7.0,1330.0,0.0,47.3675,-122.11,1420.0,9548.0,47.0,0.0,0.0]},"out":{"variable":[244380.27]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,870.0,3121.0,1.0,0.0,0.0,4.0,7.0,870.0,0.0,47.6659,-122.369,1570.0,1777.0,91.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1430.0,9600.0,1.0,0.0,0.0,4.0,7.0,1430.0,0.0,47.4737,-122.15,1590.0,10240.0,48.0,0.0,0.0]},"out":{"variable":[260603.86]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,5180.0,19850.0,2.0,0.0,3.0,3.0,12.0,3540.0,1640.0,47.562,-122.162,3160.0,9750.0,9.0,0.0,0.0]},"out":{"variable":[1295531.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.75,2200.0,7000.0,1.0,0.0,0.0,4.0,7.0,1280.0,920.0,47.4574,-122.169,1670.0,7000.0,37.0,0.0,0.0]},"out":{"variable":[381737.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2430.0,7559.0,1.0,0.0,0.0,4.0,8.0,1580.0,850.0,47.7206,-122.11,1980.0,8750.0,34.0,0.0,0.0]},"out":{"variable":[538316.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,1.75,2340.0,9148.0,2.0,0.0,0.0,3.0,7.0,2340.0,0.0,47.4232,-122.324,1390.0,10019.0,57.0,0.0,0.0]},"out":{"variable":[385982.16]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.0,1870.0,4751.0,1.0,0.0,0.0,3.0,8.0,1870.0,0.0,47.7082,-122.015,2170.0,5580.0,8.0,0.0,0.0]},"out":{"variable":[451035.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2020.0,11935.0,1.0,0.0,0.0,4.0,9.0,2020.0,0.0,47.632,-122.092,2410.0,12350.0,38.0,0.0,0.0]},"out":{"variable":[716173.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1130.0,7920.0,1.0,0.0,0.0,3.0,7.0,1130.0,0.0,47.4852,-122.125,1390.0,8580.0,54.0,0.0,0.0]},"out":{"variable":[239033.16]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.5,2190.0,13660.0,1.0,0.0,3.0,3.0,8.0,2190.0,0.0,47.7658,-122.37,2520.0,20500.0,62.0,0.0,0.0]},"out":{"variable":[522414.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2800.0,5900.0,1.0,0.0,0.0,3.0,8.0,1660.0,1140.0,47.6809,-122.286,2580.0,5900.0,51.0,0.0,0.0]},"out":{"variable":[718445.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,650.0,5400.0,1.0,0.0,0.0,3.0,6.0,650.0,0.0,47.6185,-122.295,1310.0,4906.0,64.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,1120.0,6653.0,1.0,0.0,0.0,4.0,7.0,1120.0,0.0,47.7321,-122.334,1580.0,7355.0,78.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1340.0,10276.0,1.0,0.0,0.0,4.0,7.0,1340.0,0.0,47.7207,-122.222,2950.0,7987.0,53.0,0.0,0.0]},"out":{"variable":[342604.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1580.0,8206.0,1.0,0.0,0.0,3.0,7.0,1100.0,480.0,47.3676,-122.312,1600.0,8196.0,52.0,0.0,0.0]},"out":{"variable":[277145.72]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[6.0,2.5,3180.0,9375.0,1.0,0.0,0.0,4.0,8.0,1590.0,1590.0,47.5707,-122.129,2670.0,9625.0,47.0,0.0,0.0]},"out":{"variable":[725875.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2030.0,4997.0,2.0,0.0,0.0,3.0,8.0,2030.0,0.0,47.393,-122.184,2095.0,5500.0,10.0,0.0,0.0]},"out":{"variable":[332104.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1100.0,5132.0,1.0,0.0,0.0,3.0,6.0,840.0,260.0,47.7011,-122.336,1280.0,5132.0,66.0,0.0,0.0]},"out":{"variable":[392867.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,970.0,2970.0,1.0,0.0,0.0,3.0,7.0,970.0,0.0,47.6233,-122.319,1670.0,3000.0,104.0,0.0,0.0]},"out":{"variable":[449699.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1190.0,11400.0,1.0,0.0,0.0,3.0,7.0,1190.0,0.0,47.5012,-122.265,1410.0,11400.0,63.0,0.0,0.0]},"out":{"variable":[242591.38]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,1920.0,8910.0,1.0,0.0,0.0,4.0,8.0,1200.0,720.0,47.5897,-122.156,2250.0,8800.0,45.0,0.0,0.0]},"out":{"variable":[563844.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2250.0,13515.0,1.0,0.0,0.0,4.0,8.0,2150.0,100.0,47.3789,-122.229,2150.0,12508.0,74.0,0.0,0.0]},"out":{"variable":[348616.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1150.0,4800.0,1.5,0.0,0.0,4.0,6.0,1150.0,0.0,47.3101,-122.212,1310.0,9510.0,76.0,0.0,0.0]},"out":{"variable":[240834.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,2.25,1660.0,2128.0,2.0,0.0,0.0,4.0,8.0,1660.0,0.0,47.7528,-122.252,1640.0,2128.0,41.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.75,3770.0,4000.0,2.5,0.0,0.0,5.0,9.0,2890.0,880.0,47.6157,-122.287,2800.0,5000.0,98.0,0.0,0.0]},"out":{"variable":[1182820.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1070.0,3000.0,1.0,0.0,0.0,4.0,7.0,870.0,200.0,47.6828,-122.353,1890.0,3300.0,89.0,0.0,0.0]},"out":{"variable":[450867.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.75,3860.0,12786.0,2.0,0.0,0.0,4.0,10.0,3860.0,0.0,47.549,-122.141,2820.0,14636.0,30.0,0.0,0.0]},"out":{"variable":[946325.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2160.0,5298.0,2.5,0.0,0.0,4.0,9.0,2160.0,0.0,47.6106,-122.31,1720.0,2283.0,112.0,0.0,0.0]},"out":{"variable":[904378.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2120.0,9297.0,2.0,0.0,0.0,4.0,8.0,2120.0,0.0,47.5561,-122.154,2620.0,10352.0,33.0,0.0,0.0]},"out":{"variable":[657905.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,780.0,6250.0,1.0,0.0,0.0,3.0,6.0,780.0,0.0,47.5099,-122.33,1280.0,7100.0,73.0,0.0,0.0]},"out":{"variable":[236815.78]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.0,2160.0,4800.0,2.0,0.0,0.0,4.0,7.0,1290.0,870.0,47.4777,-122.212,1570.0,4800.0,86.0,0.0,0.0]},"out":{"variable":[384558.4]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[1.0,1.0,620.0,8261.0,1.0,0.0,0.0,3.0,5.0,620.0,0.0,47.5138,-122.364,1180.0,8244.0,75.0,0.0,0.0]},"out":{"variable":[236815.78]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[1.0,1.0,390.0,2000.0,1.0,0.0,0.0,4.0,6.0,390.0,0.0,47.6938,-122.347,1340.0,5100.0,95.0,0.0,0.0]},"out":{"variable":[442168.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1600.0,3200.0,1.5,0.0,0.0,3.0,7.0,1600.0,0.0,47.653,-122.331,1860.0,3420.0,105.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,980.0,5110.0,1.0,0.0,0.0,4.0,7.0,780.0,200.0,47.7002,-122.314,1430.0,5110.0,75.0,0.0,0.0]},"out":{"variable":[393833.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,2100.0,31550.0,1.0,0.0,0.0,3.0,8.0,2100.0,0.0,47.6907,-121.917,1860.0,18452.0,4.0,0.0,0.0]},"out":{"variable":[641162.25]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,1460.0,1353.0,2.0,0.0,0.0,3.0,8.0,1050.0,410.0,47.5774,-122.412,1690.0,3776.0,2.0,0.0,0.0]},"out":{"variable":[499651.47]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1360.0,18123.0,1.0,0.0,0.0,3.0,8.0,1360.0,0.0,47.4716,-121.756,1570.0,16817.0,31.0,0.0,0.0]},"out":{"variable":[252192.89]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2110.0,7350.0,1.0,0.0,0.0,3.0,8.0,1530.0,580.0,47.3088,-122.341,2640.0,7777.0,34.0,0.0,0.0]},"out":{"variable":[333878.22]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1700.0,5750.0,1.5,0.0,2.0,5.0,7.0,1450.0,250.0,47.5643,-122.38,1700.0,5750.0,89.0,0.0,0.0]},"out":{"variable":[546632.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1820.0,3899.0,2.0,0.0,0.0,3.0,7.0,1820.0,0.0,47.735,-121.985,1820.0,3899.0,16.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1560.0,4350.0,2.0,0.0,0.0,3.0,7.0,1560.0,0.0,47.5025,-122.186,1560.0,4350.0,11.0,0.0,0.0]},"out":{"variable":[298900.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.25,2510.0,6339.0,1.5,0.0,2.0,5.0,8.0,1810.0,700.0,47.6496,-122.391,1820.0,5741.0,82.0,0.0,0.0]},"out":{"variable":[717051.75]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1640.0,5200.0,1.0,0.0,0.0,4.0,7.0,1040.0,600.0,47.6426,-122.403,1780.0,5040.0,77.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1580.0,7424.0,1.0,0.0,0.0,3.0,7.0,1010.0,570.0,47.4607,-122.171,1710.0,7772.0,52.0,0.0,0.0]},"out":{"variable":[296494.97]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.0,1190.0,7500.0,1.0,0.0,0.0,5.0,7.0,1190.0,0.0,47.3248,-122.142,1200.0,9750.0,46.0,0.0,0.0]},"out":{"variable":[241330.19]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2160.0,7000.0,2.0,0.0,0.0,4.0,9.0,2160.0,0.0,47.5659,-122.013,2300.0,7440.0,25.0,0.0,0.0]},"out":{"variable":[723867.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.5,2220.0,8994.0,1.0,0.0,0.0,4.0,8.0,1110.0,1110.0,47.5473,-122.172,2220.0,8994.0,52.0,0.0,0.0]},"out":{"variable":[680620.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[6.0,4.0,5310.0,12741.0,2.0,0.0,2.0,3.0,10.0,3600.0,1710.0,47.5696,-122.213,4190.0,12632.0,48.0,0.0,0.0]},"out":{"variable":[2016006.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.0,1330.0,2400.0,1.5,0.0,0.0,4.0,6.0,1330.0,0.0,47.65,-122.34,1330.0,4400.0,114.0,0.0,0.0]},"out":{"variable":[448627.7]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.0,3750.0,11025.0,2.0,0.0,0.0,3.0,10.0,3750.0,0.0,47.6367,-122.059,2930.0,12835.0,38.0,0.0,0.0]},"out":{"variable":[950677.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2460.0,6454.0,2.0,0.0,0.0,3.0,7.0,2460.0,0.0,47.5381,-121.89,2320.0,4578.0,9.0,0.0,0.0]},"out":{"variable":[701940.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,1600.0,2231.0,2.0,0.0,0.0,3.0,7.0,1600.0,0.0,47.3314,-122.29,1600.0,2962.0,11.0,0.0,0.0]},"out":{"variable":[277145.72]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2370.0,5353.0,2.0,0.0,0.0,3.0,8.0,2370.0,0.0,47.7333,-121.975,2130.0,6850.0,5.0,0.0,0.0]},"out":{"variable":[536371.2]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.75,1740.0,4736.0,1.5,0.0,2.0,4.0,7.0,1040.0,700.0,47.5994,-122.287,2020.0,4215.0,107.0,0.0,0.0]},"out":{"variable":[559631.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1540.0,1044.0,3.0,0.0,0.0,3.0,8.0,1540.0,0.0,47.6765,-122.32,1580.0,3090.0,0.0,0.0,0.0]},"out":{"variable":[552992.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.0,3540.0,9970.0,2.0,0.0,3.0,3.0,9.0,3540.0,0.0,47.7108,-122.277,2280.0,7195.0,44.0,0.0,0.0]},"out":{"variable":[1085835.8]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,2.75,3230.0,13572.0,1.0,0.0,2.0,3.0,8.0,1880.0,1350.0,47.4393,-122.347,2910.0,15292.0,50.0,0.0,0.0]},"out":{"variable":[473287.38]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,960.0,4920.0,1.0,0.0,0.0,3.0,6.0,960.0,0.0,47.6946,-122.362,1010.0,5040.0,72.0,0.0,0.0]},"out":{"variable":[441284.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2800.0,49149.0,1.0,0.0,0.0,4.0,7.0,1400.0,1400.0,47.4649,-122.034,2560.0,61419.0,36.0,0.0,0.0]},"out":{"variable":[439212.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.25,2560.0,12100.0,1.0,0.0,0.0,4.0,8.0,1760.0,800.0,47.631,-122.108,2240.0,12100.0,38.0,0.0,0.0]},"out":{"variable":[701940.6]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2040.0,9225.0,1.0,0.0,0.0,5.0,8.0,1610.0,430.0,47.636,-122.097,1730.0,9225.0,46.0,0.0,0.0]},"out":{"variable":[627853.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2010.0,7000.0,2.0,0.0,0.0,5.0,8.0,2010.0,0.0,47.6607,-122.396,1420.0,4400.0,113.0,0.0,0.0]},"out":{"variable":[583765.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.75,2890.0,12130.0,2.0,0.0,3.0,4.0,10.0,2830.0,60.0,47.6505,-122.203,2415.0,11538.0,27.0,0.0,0.0]},"out":{"variable":[987149.56]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1170.0,7142.0,1.0,0.0,0.0,3.0,7.0,1170.0,0.0,47.7497,-122.313,1170.0,7615.0,63.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1980.0,5909.0,2.0,0.0,0.0,3.0,8.0,1980.0,0.0,47.3913,-122.185,2550.0,5487.0,11.0,0.0,0.0]},"out":{"variable":[324875.06]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1890.0,93218.0,1.0,0.0,0.0,4.0,7.0,1890.0,0.0,47.2568,-122.07,1690.0,172062.0,50.0,0.0,0.0]},"out":{"variable":[291799.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1430.0,3000.0,1.5,0.0,0.0,3.0,7.0,1300.0,130.0,47.6415,-122.303,1750.0,4000.0,85.0,0.0,0.0]},"out":{"variable":[455435.63]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,2230.0,5650.0,2.0,0.0,0.0,3.0,7.0,2230.0,0.0,47.5073,-122.168,1590.0,7241.0,3.0,0.0,0.0]},"out":{"variable":[401263.84]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,1380.0,4390.0,1.0,0.0,0.0,4.0,8.0,880.0,500.0,47.6947,-122.323,1390.0,5234.0,83.0,0.0,0.0]},"out":{"variable":[441284.0]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[5.0,3.0,2230.0,6551.0,1.0,0.0,0.0,3.0,7.0,1330.0,900.0,47.487,-122.32,2230.0,9476.0,1.0,0.0,0.0]},"out":{"variable":[399760.3]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2010.0,2287.0,2.0,0.0,0.0,3.0,8.0,1390.0,620.0,47.5517,-121.998,1690.0,1662.0,0.0,0.0,0.0]},"out":{"variable":[578362.9]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.75,2500.0,4950.0,2.0,0.0,0.0,3.0,8.0,2500.0,0.0,47.6964,-122.017,2500.0,4950.0,4.0,0.0,0.0]},"out":{"variable":[700271.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1600.0,9579.0,1.0,0.0,0.0,3.0,8.0,1180.0,420.0,47.7662,-122.159,1750.0,9829.0,38.0,0.0,0.0]},"out":{"variable":[437177.88]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[2.0,1.0,870.0,8487.0,1.0,0.0,0.0,4.0,6.0,870.0,0.0,47.4955,-122.239,1350.0,6850.0,71.0,0.0,0.0]},"out":{"variable":[236238.66]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,2.5,1690.0,7260.0,1.0,0.0,0.0,3.0,7.0,1080.0,610.0,47.3001,-122.368,1690.0,7700.0,36.0,0.0,0.0]},"out":{"variable":[284336.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2140.0,8925.0,2.0,0.0,0.0,3.0,8.0,2140.0,0.0,47.6314,-122.027,2310.0,8956.0,23.0,0.0,0.0]},"out":{"variable":[669645.44]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,1300.0,10030.0,1.0,0.0,0.0,4.0,7.0,1300.0,0.0,47.7359,-122.192,1520.0,7713.0,48.0,0.0,0.0]},"out":{"variable":[340764.53]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.5,2900.0,23550.0,1.0,0.0,0.0,3.0,10.0,1490.0,1410.0,47.5708,-122.153,2900.0,19604.0,27.0,0.0,0.0]},"out":{"variable":[827411.1]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,1.75,2700.0,7875.0,1.5,0.0,0.0,4.0,8.0,2700.0,0.0,47.454,-122.144,2220.0,7875.0,46.0,0.0,0.0]},"out":{"variable":[441960.38]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[4.0,3.25,2910.0,1880.0,2.0,0.0,3.0,5.0,9.0,1830.0,1080.0,47.616,-122.282,3100.0,8200.0,100.0,0.0,0.0]},"out":{"variable":[1060847.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,1.75,2910.0,37461.0,1.0,0.0,0.0,4.0,7.0,1530.0,1380.0,47.7015,-122.164,2520.0,18295.0,47.0,0.0,0.0]},"out":{"variable":[706823.5]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}},{"time":1702405553622,"in":{"tensor":[3.0,2.0,2005.0,7000.0,1.0,0.0,0.0,3.0,7.0,1605.0,400.0,47.6039,-122.298,1750.0,4500.0,34.0,0.0,0.0]},"out":{"variable":[581002.94]},"check_failures":[],"metadata":{"last_model":"{\"model_name\":\"housepricesagacontrol\",\"model_sha\":\"e22a0831aafd9917f3cc87a15ed267797f80e2afa12ad7d8810ca58f173b8cc6\"}","pipeline_version":"","elapsed":[5708247,1425087],"dropped":[],"partition":"house-price-edge-jch"}}]
time.sleep(10)
edge_datetime_end = datetime.datetime.now()
logs = mainpipeline.logs(start_datetime = edge_datetime_start,
end_datetime = edge_datetime_end,
dataset=['time', 'out.variable', 'metadata'])
edge_locations = [pd.unique(logs['metadata.partition']).tolist()][0]
print(edge_locations)
Warning: Pipeline log size limit exceeded. Please request logs using export_logs
['house-price-edge-jch']
Edge Observability
We can now update our assay to include separate edge locations and display the results using the wallaroo.assay_config.AssayBuilder.window_builder.add_location_filter()
. We’ll start by just including our Wallaroo Ops pipeline, with the dates limited to the start of of the Ops Pipeline inferences to include the edge inference results.
assay_builder_from_numpy.add_run_until(edge_datetime_end)
assay_builder_from_numpy.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start).add_location_filter([ops_location])
assay_config_from_numpy = assay_builder_from_numpy.build()
assay_analysis_from_numpy = assay_config_from_numpy.interactive_run()
# Show how many assay windows were analyzed, then show the chart
print(f"Generated {len(assay_analysis_from_numpy)} analyses")
assay_analysis_from_numpy.chart_scores()
Generated 1 analyses
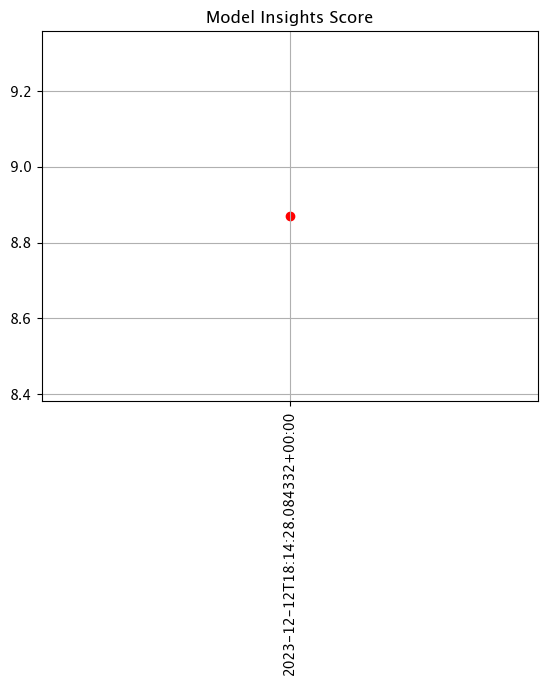
Now we’ll update with for just the edge location.
assay_builder_from_numpy.add_run_until(edge_datetime_end)
(assay_builder_from_numpy
.window_builder()
.add_width(minutes=1)
.add_interval(minutes=1)
.add_start(assay_window_start)
.add_location_filter([edge_name]))
assay_config_from_numpy = assay_builder_from_numpy.build()
assay_analysis_from_numpy = assay_config_from_numpy.interactive_run()
# Show how many assay windows were analyzed, then show the chart
print(f"Generated {len(assay_analysis_from_numpy)} analyses")
assay_analysis_from_numpy.chart_scores()
Generated 1 analyses
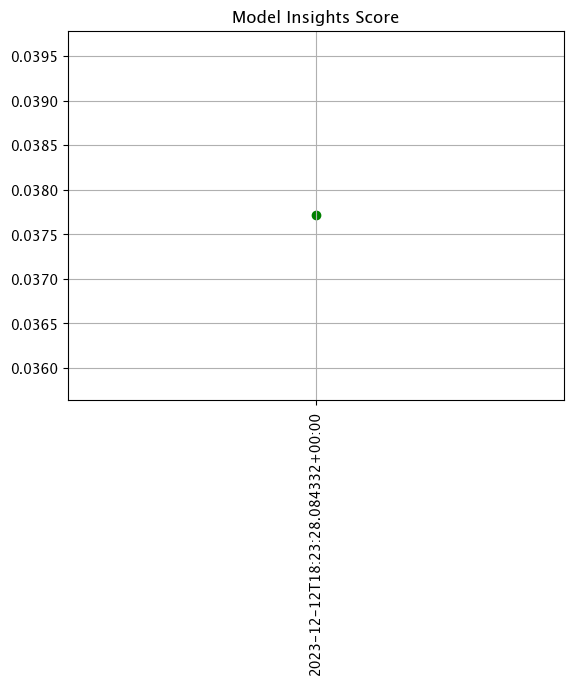
And finally we will use both locations in a cumulative assay window.
assay_builder_from_numpy.add_run_until(edge_datetime_end)
(assay_builder_from_numpy
.window_builder()
.add_width(minutes=1)
.add_interval(minutes=1)
.add_start(assay_window_start)
.add_location_filter([ops_location, edge_name]))
assay_config_from_numpy = assay_builder_from_numpy.build()
assay_analysis_from_numpy = assay_config_from_numpy.interactive_run()
# Show how many assay windows were analyzed, then show the chart
print(f"Generated {len(assay_analysis_from_numpy)} analyses")
assay_analysis_from_numpy.chart_scores()
Generated 2 analyses
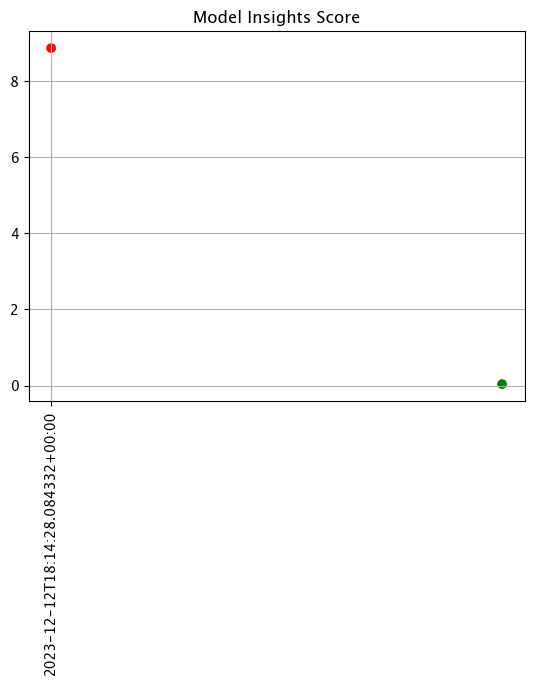
11 - House Price Testing Life Cycle
This tutorial and the assets can be downloaded as part of the Wallaroo Tutorials repository.
House Price Testing Life Cycle Comprehensive Tutorial
This tutorial simulates using Wallaroo for testing a model for inference outliers, potential model drift, and methods to test competitive models against each other and deploy the final version to use. This demonstrates using assays to detect model or data drift, then Wallaroo Shadow Deploy to compare different models to determine which one is most fit for an organization’s needs. These features allow organizations to monitor model performance and accuracy then swap out models as needed.
- IMPORTANT NOTE: This tutorial assumes that the House Price Model Life Cycle Preparation notebook was run before this notebook, and that the workspace, pipeline and models used are the same. This is critical for the section on Assays below. If the preparation notebook has not been run, skip the Assays section as there will be no historical data for the assays to function on.
This tutorial will demonstrate how to:
- Select or create a workspace, pipeline and upload the champion model.
- Add a pipeline step with the champion model, then deploy the pipeline and perform sample inferences.
- Create an assay and set a baseline, then demonstrate inferences that trigger the assay alert threshold.
- Swap out the pipeline step with the champion model with a shadow deploy step that compares the champion model against two competitors.
- Evaluate the results of the champion versus competitor models.
- Change the pipeline step from a shadow deploy step to an A/B testing step, and show the different results.
- Change the A/B testing step back to standard pipeline step with the original control model, then demonstrate hot swapping the control model with a challenger model without undeploying the pipeline.
- Undeploy the pipeline.
This tutorial provides the following:
- Models:
models/rf_model.onnx
: The champion model that has been used in this environment for some time.models/xgb_model.onnx
andmodels/gbr_model.onnx
: Rival models that will be tested against the champion.
- Data:
data/xtest-1.df.json
anddata/xtest-1k.df.json
: DataFrame JSON inference inputs with 1 input and 1,000 inputs.data/xtest-1k.arrow
: Apache Arrow inference inputs with 1 input and 1,000 inputs.
Prerequisites
- A deployed Wallaroo instance
- The following Python libraries installed:
Initial Steps
Import libraries
The first step is to import the libraries needed for this notebook.
import wallaroo
from wallaroo.object import EntityNotFoundError
from wallaroo.framework import Framework
from IPython.display import display
# used to display DataFrame information without truncating
from IPython.display import display
import pandas as pd
pd.set_option('display.max_colwidth', None)
import datetime
import time
# used for unique connection names
import string
import random
suffix= ''.join(random.choice(string.ascii_lowercase) for i in range(4))
suffix='baselines'
import json
Connect to the Wallaroo Instance
The first step is to connect to Wallaroo through the Wallaroo client. The Python library is included in the Wallaroo install and available through the Jupyter Hub interface provided with your Wallaroo environment.
This is accomplished using the wallaroo.Client()
command, which provides a URL to grant the SDK permission to your specific Wallaroo environment. When displayed, enter the URL into a browser and confirm permissions. Store the connection into a variable that can be referenced later.
If logging into the Wallaroo instance through the internal JupyterHub service, use wl = wallaroo.Client()
. For more information on Wallaroo Client settings, see the Client Connection guide.
# Login through local Wallaroo instance
wl = wallaroo.Client()
Create Workspace
We will create a workspace to manage our pipeline and models. The following variables will set the name of our sample workspace then set it as the current workspace.
Workspace, pipeline, and model names should be unique to each user, so we’ll add in a randomly generated suffix so multiple people can run this tutorial in a Wallaroo instance without effecting each other.
workspace_name = f'housepricesagaworkspace{suffix}'
main_pipeline_name = f'housepricesagapipeline'
model_name_control = f'housepricesagacontrol'
model_file_name_control = './models/rf_model.onnx'
def get_workspace(name):
workspace = None
for ws in wl.list_workspaces():
if ws.name() == name:
workspace= ws
if(workspace == None):
workspace = wl.create_workspace(name)
return workspace
def get_pipeline(name, workspace):
pipelines = workspace.pipelines()
pipe_filter = filter(lambda x: x.name() == name, pipelines)
pipes = list(pipe_filter)
# we can't have a pipe in the workspace with the same name, so it's always the first
if pipes:
pipeline = pipes[0]
else:
pipeline = wl.build_pipeline(name)
return pipeline
workspace = get_workspace(workspace_name)
wl.set_current_workspace(workspace)
{'name': 'housepricesagaworkspacebaselines', 'id': 87, 'archived': False, 'created_by': 'd6a42dd8-1da9-4405-bb80-7c4b42e38b52', 'created_at': '2023-10-31T16:56:03.395454+00:00', 'models': [], 'pipelines': []}
Upload The Champion Model
For our example, we will upload the champion model that has been trained to derive house prices from a variety of inputs. The model file is rf_model.onnx
, and is uploaded with the name housingcontrol
.
housing_model_control = (wl.upload_model(model_name_control,
model_file_name_control,
framework=Framework.ONNX)
.configure(tensor_fields=["tensor"])
)
Standard Pipeline Steps
Build the Pipeline
This pipeline is made to be an example of an existing situation where a model is deployed and being used for inferences in a production environment. We’ll call it housepricepipeline
, set housingcontrol
as a pipeline step, then run a few sample inferences.
This pipeline will be a simple one - just a single pipeline step.
mainpipeline = get_pipeline(main_pipeline_name, workspace)
# clearing from previous runs and verifying it is undeployed
mainpipeline.clear()
mainpipeline.undeploy()
mainpipeline.add_model_step(housing_model_control)
#minimum deployment config
deploy_config = wallaroo.DeploymentConfigBuilder().replica_count(1).cpus(0.5).memory("1Gi").build()
mainpipeline.deploy(deployment_config = deploy_config)
Waiting for deployment - this will take up to 45s ............. ok
name | housepricesagapipeline |
---|---|
created | 2023-10-31 16:56:07.345831+00:00 |
last_updated | 2023-10-31 16:56:07.444932+00:00 |
deployed | True |
tags | |
versions | 2ea0cc42-c955-4fc3-bac9-7a2c7c22ddc1, dca84ab2-274a-4391-95dd-a99bda7621e1 |
steps | housepricesagacontrol |
published | False |
Testing
We’ll use two inferences as a quick sample test - one that has a house that should be determined around $700k, the other with a house determined to be around $1.5 million. We’ll also save the start and end periods for these events to for later log functionality.
normal_input = pd.DataFrame.from_records({"tensor": [[4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0]]})
result = mainpipeline.infer(normal_input)
display(result)
time | in.tensor | out.variable | check_failures | |
---|---|---|---|---|
0 | 2023-10-31 16:57:07.307 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.7] | 0 |
large_house_input = pd.DataFrame.from_records({'tensor': [[4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0, 10.0, 2760.0, 950.0, 47.6696, -122.261, 3970.0, 20000.0, 79.0, 0.0, 0.0]]})
large_house_result = mainpipeline.infer(large_house_input)
display(large_house_result)
time | in.tensor | out.variable | check_failures | |
---|---|---|---|---|
0 | 2023-10-31 16:57:07.746 | [4.0, 3.0, 3710.0, 20000.0, 2.0, 0.0, 2.0, 5.0, 10.0, 2760.0, 950.0, 47.6696, -122.261, 3970.0, 20000.0, 79.0, 0.0, 0.0] | [1514079.4] | 0 |
As one last sample, we’ll run through roughly 1,000 inferences at once and show a few of the results. For this example we’ll use an Apache Arrow table, which has a smaller file size compared to uploading a pandas DataFrame JSON file. The inference result is returned as an arrow table, which we’ll convert into a pandas DataFrame to display the first 20 results.
time.sleep(5)
control_model_start = datetime.datetime.now()
batch_inferences = mainpipeline.infer_from_file('./data/xtest-1k.arrow')
large_inference_result = batch_inferences.to_pandas()
display(large_inference_result.head(20))
time | in.tensor | out.variable | check_failures | |
---|---|---|---|---|
0 | 2023-10-31 16:57:13.771 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.7] | 0 |
1 | 2023-10-31 16:57:13.771 | [2.0, 2.5, 2170.0, 6361.0, 1.0, 0.0, 2.0, 3.0, 8.0, 2170.0, 0.0, 47.7109, -122.017, 2310.0, 7419.0, 6.0, 0.0, 0.0] | [615094.6] | 0 |
2 | 2023-10-31 16:57:13.771 | [3.0, 2.5, 1300.0, 812.0, 2.0, 0.0, 0.0, 3.0, 8.0, 880.0, 420.0, 47.5893, -122.317, 1300.0, 824.0, 6.0, 0.0, 0.0] | [448627.8] | 0 |
3 | 2023-10-31 16:57:13.771 | [4.0, 2.5, 2500.0, 8540.0, 2.0, 0.0, 0.0, 3.0, 9.0, 2500.0, 0.0, 47.5759, -121.994, 2560.0, 8475.0, 24.0, 0.0, 0.0] | [758714.3] | 0 |
4 | 2023-10-31 16:57:13.771 | [3.0, 1.75, 2200.0, 11520.0, 1.0, 0.0, 0.0, 4.0, 7.0, 2200.0, 0.0, 47.7659, -122.341, 1690.0, 8038.0, 62.0, 0.0, 0.0] | [513264.66] | 0 |
5 | 2023-10-31 16:57:13.771 | [3.0, 2.0, 2140.0, 4923.0, 1.0, 0.0, 0.0, 4.0, 8.0, 1070.0, 1070.0, 47.6902, -122.339, 1470.0, 4923.0, 86.0, 0.0, 0.0] | [668287.94] | 0 |
6 | 2023-10-31 16:57:13.771 | [4.0, 3.5, 3590.0, 5334.0, 2.0, 0.0, 2.0, 3.0, 9.0, 3140.0, 450.0, 47.6763, -122.267, 2100.0, 6250.0, 9.0, 0.0, 0.0] | [1004846.56] | 0 |
7 | 2023-10-31 16:57:13.771 | [3.0, 2.0, 1280.0, 960.0, 2.0, 0.0, 0.0, 3.0, 9.0, 1040.0, 240.0, 47.602, -122.311, 1280.0, 1173.0, 0.0, 0.0, 0.0] | [684577.25] | 0 |
8 | 2023-10-31 16:57:13.771 | [4.0, 2.5, 2820.0, 15000.0, 2.0, 0.0, 0.0, 4.0, 9.0, 2820.0, 0.0, 47.7255, -122.101, 2440.0, 15000.0, 29.0, 0.0, 0.0] | [727898.25] | 0 |
9 | 2023-10-31 16:57:13.771 | [3.0, 2.25, 1790.0, 11393.0, 1.0, 0.0, 0.0, 3.0, 8.0, 1790.0, 0.0, 47.6297, -122.099, 2290.0, 11894.0, 36.0, 0.0, 0.0] | [559631.06] | 0 |
10 | 2023-10-31 16:57:13.771 | [3.0, 1.5, 1010.0, 7683.0, 1.5, 0.0, 0.0, 5.0, 7.0, 1010.0, 0.0, 47.72, -122.318, 1550.0, 7271.0, 61.0, 0.0, 0.0] | [340764.53] | 0 |
11 | 2023-10-31 16:57:13.771 | [3.0, 2.0, 1270.0, 1323.0, 3.0, 0.0, 0.0, 3.0, 8.0, 1270.0, 0.0, 47.6934, -122.342, 1330.0, 1323.0, 8.0, 0.0, 0.0] | [442168.12] | 0 |
12 | 2023-10-31 16:57:13.771 | [4.0, 1.75, 2070.0, 9120.0, 1.0, 0.0, 0.0, 4.0, 7.0, 1250.0, 820.0, 47.6045, -122.123, 1650.0, 8400.0, 57.0, 0.0, 0.0] | [630865.5] | 0 |
13 | 2023-10-31 16:57:13.771 | [4.0, 1.0, 1620.0, 4080.0, 1.5, 0.0, 0.0, 3.0, 7.0, 1620.0, 0.0, 47.6696, -122.324, 1760.0, 4080.0, 91.0, 0.0, 0.0] | [559631.06] | 0 |
14 | 2023-10-31 16:57:13.771 | [4.0, 3.25, 3990.0, 9786.0, 2.0, 0.0, 0.0, 3.0, 9.0, 3990.0, 0.0, 47.6784, -122.026, 3920.0, 8200.0, 10.0, 0.0, 0.0] | [909441.25] | 0 |
15 | 2023-10-31 16:57:13.771 | [4.0, 2.0, 1780.0, 19843.0, 1.0, 0.0, 0.0, 3.0, 7.0, 1780.0, 0.0, 47.4414, -122.154, 2210.0, 13500.0, 52.0, 0.0, 0.0] | [313096.0] | 0 |
16 | 2023-10-31 16:57:13.771 | [4.0, 2.5, 2130.0, 6003.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2130.0, 0.0, 47.4518, -122.12, 1940.0, 4529.0, 11.0, 0.0, 0.0] | [404040.78] | 0 |
17 | 2023-10-31 16:57:13.771 | [3.0, 1.75, 1660.0, 10440.0, 1.0, 0.0, 0.0, 3.0, 7.0, 1040.0, 620.0, 47.4448, -121.77, 1240.0, 10380.0, 36.0, 0.0, 0.0] | [292859.44] | 0 |
18 | 2023-10-31 16:57:13.771 | [3.0, 2.5, 2110.0, 4118.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2110.0, 0.0, 47.3878, -122.153, 2110.0, 4044.0, 25.0, 0.0, 0.0] | [338357.88] | 0 |
19 | 2023-10-31 16:57:13.771 | [4.0, 2.25, 2200.0, 11250.0, 1.5, 0.0, 0.0, 5.0, 7.0, 1300.0, 900.0, 47.6845, -122.201, 2320.0, 10814.0, 94.0, 0.0, 0.0] | [682284.56] | 0 |
Graph of Prices
Here’s a distribution plot of the inferences to view the values, with the X axis being the house price in millions, and the Y axis the number of houses fitting in a bin grouping. The majority of houses are in the $250,000 to $500,000 range, with some outliers in the far end.
import matplotlib.pyplot as plt
houseprices = pd.DataFrame({'sell_price': large_inference_result['out.variable'].apply(lambda x: x[0])})
houseprices.hist(column='sell_price', bins=75, grid=False, figsize=(12,8))
plt.axvline(x=0, color='gray', ls='--')
_ = plt.title('Distribution of predicted home sales price')
time.sleep(5)
control_model_end = datetime.datetime.now()
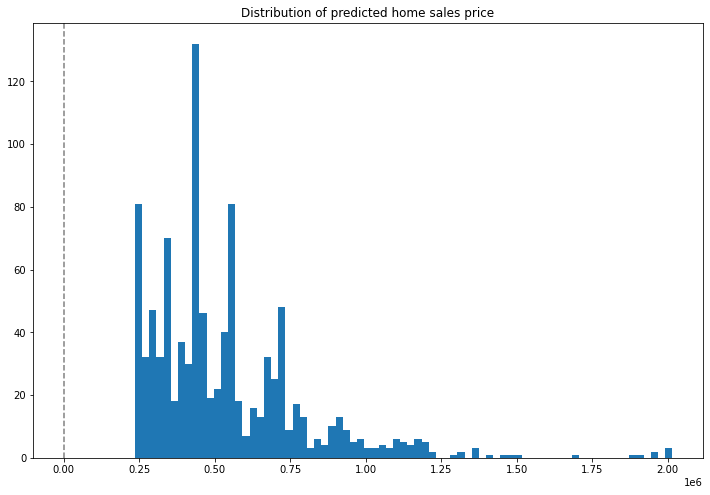
Pipeline Logs
Pipeline logs with standard pipeline steps are retrieved either with:
- Pipeline
logs
which returns either a pandas DataFrame or Apache Arrow table. - Pipeline
export_logs
which saves the logs either a pandas DataFrame JSON file or Apache Arrow table.
For full details, see the Wallaroo Documentation Pipeline Log Management guide.
Pipeline Log Methods
The Pipeline logs
method accepts the following parameters.
Parameter | Type | Description |
---|---|---|
limit | Int (Optional) | Limits how many log records to display. Defaults to 100 . If there are more pipeline logs than are being displayed, the Warning message Pipeline log record limit exceeded will be displayed. For example, if 100 log files were requested and there are a total of 1,000, the warning message will be displayed. |
start_datetimert and end_datetime | DateTime (Optional) | Limits logs to all logs between the start_datetime and end_datetime DateTime parameters. Both parameters must be provided. Submitting a logs() request with only start_datetime or end_datetime will generate an exception.If start_datetime and end_datetime are provided as parameters, then the records are returned in chronological order, with the oldest record displayed first. |
arrow | Boolean (Optional) | Defaults to False. If arrow is set to True , then the logs are returned as an Apache Arrow table. If arrow=False , then the logs are returned as a pandas DataFrame. |
The following examples demonstrate displaying the logs, then displaying the logs between the control_model_start
and control_model_end
periods, then again retrieved as an Arrow table.
# pipeline log retrieval - reverse chronological order
display(mainpipeline.logs())
# pipeline log retrieval between two dates - chronological order
display(mainpipeline.logs(start_datetime=control_model_start, end_datetime=control_model_end))
# pipeline log retrieval limited to the last 5 an an arrow table
display(mainpipeline.logs(arrow=True))
Warning: There are more logs available. Please set a larger limit or request a file using export_logs.
time | in.tensor | out.variable | check_failures | |
---|---|---|---|---|
0 | 2023-10-31 16:57:13.771 | [3.0, 2.0, 2005.0, 7000.0, 1.0, 0.0, 0.0, 3.0, 7.0, 1605.0, 400.0, 47.6039, -122.298, 1750.0, 4500.0, 34.0, 0.0, 0.0] | [581002.94] | 0 |
1 | 2023-10-31 16:57:13.771 | [3.0, 1.75, 2910.0, 37461.0, 1.0, 0.0, 0.0, 4.0, 7.0, 1530.0, 1380.0, 47.7015, -122.164, 2520.0, 18295.0, 47.0, 0.0, 0.0] | [706823.6] | 0 |
2 | 2023-10-31 16:57:13.771 | [4.0, 3.25, 2910.0, 1880.0, 2.0, 0.0, 3.0, 5.0, 9.0, 1830.0, 1080.0, 47.616, -122.282, 3100.0, 8200.0, 100.0, 0.0, 0.0] | [1060847.5] | 0 |
3 | 2023-10-31 16:57:13.771 | [4.0, 1.75, 2700.0, 7875.0, 1.5, 0.0, 0.0, 4.0, 8.0, 2700.0, 0.0, 47.454, -122.144, 2220.0, 7875.0, 46.0, 0.0, 0.0] | [441960.3] | 0 |
4 | 2023-10-31 16:57:13.771 | [3.0, 2.5, 2900.0, 23550.0, 1.0, 0.0, 0.0, 3.0, 10.0, 1490.0, 1410.0, 47.5708, -122.153, 2900.0, 19604.0, 27.0, 0.0, 0.0] | [827411.25] | 0 |
... | ... | ... | ... | ... |
95 | 2023-10-31 16:57:13.771 | [2.0, 1.5, 1070.0, 1236.0, 2.0, 0.0, 0.0, 3.0, 8.0, 1000.0, 70.0, 47.5619, -122.382, 1170.0, 1888.0, 10.0, 0.0, 0.0] | [435628.72] | 0 |
96 | 2023-10-31 16:57:13.771 | [3.0, 2.5, 2830.0, 6000.0, 1.0, 0.0, 3.0, 3.0, 9.0, 1730.0, 1100.0, 47.5751, -122.378, 2040.0, 5300.0, 60.0, 0.0, 0.0] | [981676.7] | 0 |
97 | 2023-10-31 16:57:13.771 | [4.0, 1.75, 1720.0, 8750.0, 1.0, 0.0, 0.0, 3.0, 7.0, 860.0, 860.0, 47.726, -122.21, 1790.0, 8750.0, 43.0, 0.0, 0.0] | [437177.97] | 0 |
98 | 2023-10-31 16:57:13.771 | [4.0, 2.25, 4470.0, 60373.0, 2.0, 0.0, 0.0, 3.0, 11.0, 4470.0, 0.0, 47.7289, -122.127, 3210.0, 40450.0, 26.0, 0.0, 0.0] | [1208638.1] | 0 |
99 | 2023-10-31 16:57:13.771 | [3.0, 1.0, 1150.0, 3000.0, 1.0, 0.0, 0.0, 5.0, 6.0, 1150.0, 0.0, 47.6867, -122.345, 1460.0, 3200.0, 108.0, 0.0, 0.0] | [448627.8] | 0 |
100 rows × 4 columns
Warning: Pipeline log size limit exceeded. Please request logs using export_logs
time | in.tensor | out.variable | check_failures | |
---|---|---|---|---|
0 | 2023-10-31 16:57:13.771 | [4.0, 2.5, 2900.0, 5505.0, 2.0, 0.0, 0.0, 3.0, 8.0, 2900.0, 0.0, 47.6063, -122.02, 2970.0, 5251.0, 12.0, 0.0, 0.0] | [718013.7] | 0 |
1 | 2023-10-31 16:57:13.771 | [2.0, 2.5, 2170.0, 6361.0, 1.0, 0.0, 2.0, 3.0, 8.0, 2170.0, 0.0, 47.7109, -122.017, 2310.0, 7419.0, 6.0, 0.0, 0.0] | [615094.6] | 0 |
2 | 2023-10-31 16:57:13.771 | [3.0, 2.5, 1300.0, 812.0, 2.0, 0.0, 0.0, 3.0, 8.0, 880.0, 420.0, 47.5893, -122.317, 1300.0, 824.0, 6.0, 0.0, 0.0] | [448627.8] | 0 |
3 | 2023-10-31 16:57:13.771 | [4.0, 2.5, 2500.0, 8540.0, 2.0, 0.0, 0.0, 3.0, 9.0, 2500.0, 0.0, 47.5759, -121.994, 2560.0, 8475.0, 24.0, 0.0, 0.0] | [758714.3] | 0 |
4 | 2023-10-31 16:57:13.771 | [3.0, 1.75, 2200.0, 11520.0, 1.0, 0.0, 0.0, 4.0, 7.0, 2200.0, 0.0, 47.7659, -122.341, 1690.0, 8038.0, 62.0, 0.0, 0.0] | [513264.66] | 0 |
... | ... | ... | ... | ... |
495 | 2023-10-31 16:57:13.771 | [4.0, 2.5, 3550.0, 35689.0, 2.0, 0.0, 0.0, 4.0, 9.0, 3550.0, 0.0, 47.7503, -122.074, 3350.0, 35711.0, 23.0, 0.0, 0.0] | [873315.2] | 0 |
496 | 2023-10-31 16:57:13.771 | [4.0, 2.5, 2510.0, 47044.0, 2.0, 0.0, 0.0, 3.0, 9.0, 2510.0, 0.0, 47.7699, -122.085, 2600.0, 42612.0, 27.0, 0.0, 0.0] | [721143.6] | 0 |
497 | 2023-10-31 16:57:13.771 | [4.0, 2.5, 4090.0, 11225.0, 2.0, 0.0, 0.0, 3.0, 10.0, 4090.0, 0.0, 47.581, -121.971, 3510.0, 8762.0, 9.0, 0.0, 0.0] | [1048372.44] | 0 |
498 | 2023-10-31 16:57:13.771 | [2.0, 1.0, 720.0, 5000.0, 1.0, 0.0, 0.0, 5.0, 6.0, 720.0, 0.0, 47.5195, -122.374, 810.0, 5000.0, 63.0, 0.0, 0.0] | [244566.39] | 0 |
499 | 2023-10-31 16:57:13.771 | [4.0, 2.75, 2930.0, 22000.0, 1.0, 0.0, 3.0, 4.0, 9.0, 1580.0, 1350.0, 47.3227, -122.384, 2930.0, 9758.0, 36.0, 0.0, 0.0] | [518869.03] | 0 |
500 rows × 4 columns
Warning: There are more logs available. Please set a larger limit or request a file using export_logs.
pyarrow.Table
time: timestamp[ms]
in.tensor: list<item: float> not null
child 0, item: float
out.variable: list<inner: float not null> not null
child 0, inner: float not null
check_failures: int8
----
time: [[2023-10-31 16:57:13.771,2023-10-31 16:57:13.771,2023-10-31 16:57:13.771,2023-10-31 16:57:13.771,2023-10-31 16:57:13.771,...,2023-10-31 16:57:13.771,2023-10-31 16:57:13.771,2023-10-31 16:57:13.771,2023-10-31 16:57:13.771,2023-10-31 16:57:13.771]]
in.tensor: [[[3,2,2005,7000,1,...,1750,4500,34,0,0],[3,1.75,2910,37461,1,...,2520,18295,47,0,0],...,[4,2.25,4470,60373,2,...,3210,40450,26,0,0],[3,1,1150,3000,1,...,1460,3200,108,0,0]]]
out.variable: [[[581002.94],[706823.6],...,[1208638.1],[448627.8]]]
check_failures: [[0,0,0,0,0,...,0,0,0,0,0]]
Anomaly Detection through Validations
Anomaly detection allows organizations to set validation parameters in a pipeline. A validation is added to a pipeline to test data based on an expression, and flag any inferences where the validation failed inference result and the pipeline logs.
Validations are added through the Pipeline add_validation(name, validation)
command which uses the following parameters:
Parameter | Type | Description |
---|---|---|
name | String (Required) | The name of the validation. |
Validation | Expression (Required) | The validation test command in the format model_name.outputs][field][index] {Operation} {Value} . |
For this example, we want to detect the outputs of housing_model_control
and validate that values are less than 1,500,000
. Any outputs greater than that will trigger a check_failure
which is shown in the output.
## Add the validation to the pipeline
mainpipeline = mainpipeline.add_validation('price too high', housing_model_control.outputs[0][0] < 1500000.0)
#minimum deployment config
deploy_config = wallaroo.DeploymentConfigBuilder().replica_count(1).cpus(0.5).memory("1Gi").build()
mainpipeline.deploy(deployment_config = deploy_config)
ok
name | housepricesagapipeline |
---|---|
created | 2023-10-31 16:56:07.345831+00:00 |
last_updated | 2023-10-31 16:57:24.026392+00:00 |
deployed | True |
tags | |
versions | f7e0deaf-63a2-491a-8ff9-e8148d3cabcb, 2ea0cc42-c955-4fc3-bac9-7a2c7c22ddc1, dca84ab2-274a-4391-95dd-a99bda7621e1 |
steps | housepricesagacontrol |
published | False |
Validation Testing
Two validations will be tested:
- One that should return a house value lower than 1,500,000. The validation will pass so
check_failure
will be 0. - The other than should return a house value greater than 1,500,000. The validation will fail, so
check_failure
will be 1.
validation_start = datetime.datetime.now()
# Small value home
normal_input = pd.DataFrame.from_records({
"tensor": [[
3.0,
2.25,
1620.0,
997.0,
2.5,
0.0,
0.0,
3.0,
8.0,
1540.0,
80.0,
47.5400009155,
-122.0260009766,
1620.0,
1068.0,
4.0,
0.0,
0.0
]]
}
)
small_result = mainpipeline.infer(normal_input)
display(small_result.loc[:,["time", "out.variable", "check_failures"]])
time | out.variable | check_failures | |
---|---|---|---|
0 | 2023-10-31 16:57:26.205 | [544392.06] | 0 |
# Big value home
big_input = pd.DataFrame.from_records({
"tensor": [[
4.0,
4.5,
5770.0,
10050.0,
1.0,
0.0,
3.0,
5.0,
9.0,
3160.0,
2610.0,
47.6769981384,
-122.2750015259,
2950.0,
6700.0,
65.0,
0.0,
0.0
]]
}
)
big_result = mainpipeline.infer(big_input)
display(big_result.loc[:,["time", "out.variable", "check_failures"]])
time | out.variable | check_failures | |
---|---|---|---|
0 | 2023-10-31 16:57:27.138 | [1689843.1] | 1 |
Anomaly Results
We’ll run through our previous batch, this time showing only those results outside of the validation, and a graph showing where the anomalies are against the other results.
batch_inferences = mainpipeline.infer_from_file('./data/xtest-1k.arrow')
large_inference_result = batch_inferences.to_pandas()
# Display only the anomalous results
display(large_inference_result[large_inference_result["check_failures"] > 0].loc[:,["time", "out.variable", "check_failures"]])
time | out.variable | check_failures | |
---|---|---|---|
30 | 2023-10-31 16:57:28.974 | [1514079.4] | 1 |
248 | 2023-10-31 16:57:28.974 | [1967344.1] | 1 |
255 | 2023-10-31 16:57:28.974 | [2002393.6] | 1 |
556 | 2023-10-31 16:57:28.974 | [1886959.2] | 1 |
698 | 2023-10-31 16:57:28.974 | [1689843.1] | 1 |
711 | 2023-10-31 16:57:28.974 | [1946437.8] | 1 |
722 | 2023-10-31 16:57:28.974 | [2005883.1] | 1 |
782 | 2023-10-31 16:57:28.974 | [1910824.0] | 1 |
965 | 2023-10-31 16:57:28.974 | [2016006.1] | 1 |
import matplotlib.pyplot as plt
houseprices = pd.DataFrame({'sell_price': large_inference_result['out.variable'].apply(lambda x: x[0])})
houseprices.hist(column='sell_price', bins=75, grid=False, figsize=(12,8))
plt.axvline(x=1500000, color='red', ls='--')
_ = plt.title('Distribution of predicted home sales price')
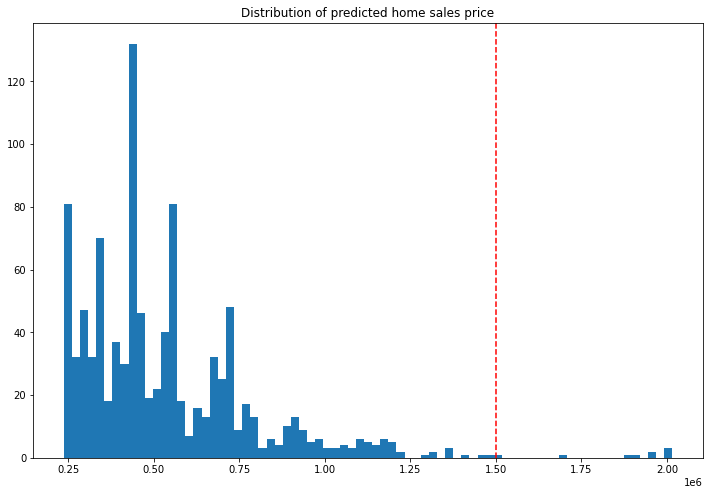
Assays
Wallaroo assays provide a method for detecting input or model drift. These can be triggered either when unexpected input is provided for the inference, or when the model needs to be retrained from changing environment conditions.
Wallaroo assays can track either an input field and its index, or an output field and its index. For full details, see the Wallaroo Assays Management Guide.
For this example, we will:
- Perform sample inferences based on lower priced houses.
- Create an assay with the baseline set off those lower priced houses.
- Generate inferences spread across all house values, plus specific set of high priced houses to trigger the assay alert.
- Run an interactive assay to show the detection of values outside the established baseline.
Assay Generation
To start the demonstration, we’ll create a baseline of values from houses with small estimated prices and set that as our baseline. Assays are typically run on a 24 hours interval based on a 24 hour window of data, but we’ll bypass that by setting our baseline time even shorter.
small_houses_inputs = pd.read_json('./data/smallinputs.df.json')
baseline_size = 500
# Where the baseline data will start
baseline_start = datetime.datetime.now()
# These inputs will be random samples of small priced houses. Around 30,000 is a good number
small_houses = small_houses_inputs.sample(baseline_size, replace=True).reset_index(drop=True)
small_results = mainpipeline.infer(small_houses)
# Set the baseline end
baseline_end = datetime.datetime.now()
# turn the inference results into a numpy array for the baseline
# set the results to a non-array value
small_results_baseline_df = small_results.copy()
small_results_baseline_df['variable']=small_results['out.variable'].map(lambda x: x[0])
# get the numpy values
small_results_baseline = small_results_baseline_df['variable'].to_numpy()
assay_baseline_from_numpy_name = "house price saga assay from numpy"
# assay builder by baseline
assay_builder_from_numpy = wl.build_assay(assay_name=assay_baseline_from_numpy_name,
pipeline=mainpipeline,
model_name=model_name_control,
iopath="output variable 0",
baseline_data = small_results_baseline)
# set the width from the recent results
assay_builder_from_numpy.window_builder().add_width(minutes=1)
assay_config_from_numpy = assay_builder_from_numpy.build()
assay_analysis_from_numpy = assay_config_from_numpy.interactive_run()
# get the histogram from the numpy baseline
assay_builder_from_numpy.baseline_histogram()
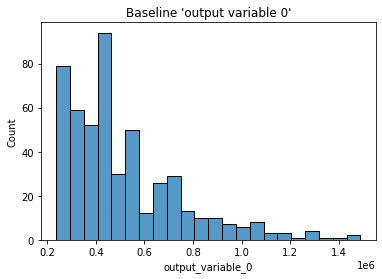
# show the baseline stats
assay_analysis_from_numpy[0].baseline_stats()
Baseline | |
---|---|
count | 500 |
min | 236238.67 |
max | 1489624.3 |
mean | 513762.85694 |
median | 448627.8 |
std | 235726.284713 |
start | None |
end | None |
Now we’ll perform some inferences with a spread of values, then a larger set with a set of larger house values to trigger our assay alert.
Because our assay windows are 1 minutes, we’ll need to stagger our inference values to be set into the proper windows. This will take about 4 minutes.
By default, assay start date is set to 24 hours from when the assay was created. For this example, we will set the assay.window_builder.add_start
to set the assay window to start at the beginning of our data, and assay.add_run_until
to set the time period to stop gathering data from.
# Get a spread of house values
time.sleep(35)
# regular_houses_inputs = pd.read_json('./data/xtest-1k.df.json', orient="records")
inference_size = 1000
# regular_houses = regular_houses_inputs.sample(inference_size, replace=True).reset_index(drop=True)
# And a spread of large house values
big_houses_inputs = pd.read_json('./data/biginputs.df.json', orient="records")
big_houses = big_houses_inputs.sample(inference_size, replace=True).reset_index(drop=True)
# Set the start for our assay window period.
assay_window_start = datetime.datetime.now()
mainpipeline.infer(big_houses)
# End our assay window period
time.sleep(35)
assay_window_end = datetime.datetime.now()
assay_builder_from_numpy.add_run_until(assay_window_end)
assay_builder_from_numpy.window_builder().add_width(minutes=1).add_interval(minutes=1).add_start(assay_window_start)
assay_config_from_dates = assay_builder_from_numpy.build()
assay_analysis_from_numpy = assay_config_from_numpy.interactive_run()
# Show how many assay windows were analyzed, then show the chart
print(f"Generated {len(assay_analysis_from_numpy)} analyses")
assay_analysis_from_numpy.chart_scores()
Generated 5 analyses
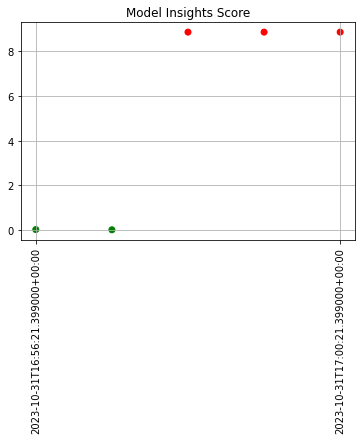
# Display the results as a DataFrame - we're mainly interested in the score and whether the
# alert threshold was triggered
display(assay_analysis_from_numpy.to_dataframe().loc[:, ["score", "start", "alert_threshold", "status"]])
score | start | alert_threshold | status | |
---|---|---|---|---|
0 | 0.016079 | 2023-10-31T16:56:21.399000+00:00 | 0.25 | Ok |
1 | 0.006042 | 2023-10-31T16:57:21.399000+00:00 | 0.25 | Ok |
2 | 8.868832 | 2023-10-31T16:58:21.399000+00:00 | 0.25 | Alert |
3 | 8.868832 | 2023-10-31T16:59:21.399000+00:00 | 0.25 | Alert |
4 | 8.868832 | 2023-10-31T17:00:21.399000+00:00 | 0.25 | Alert |
assay_builder_from_numpy.upload()
18
The assay is now visible through the Wallaroo UI by selecting the workspace, then the pipeline, then Insights.
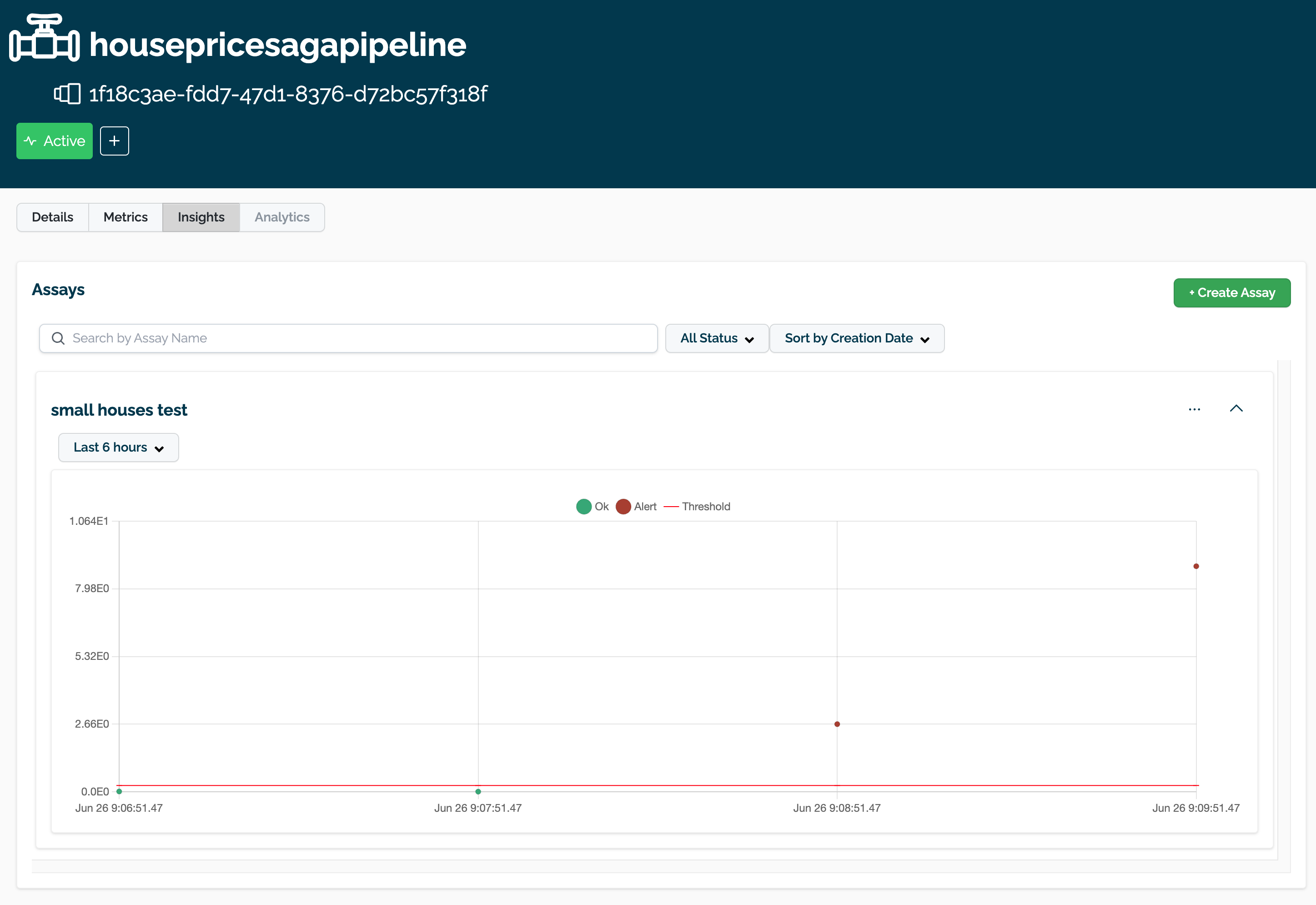
Shadow Deploy
Let’s assume that after analyzing the assay information we want to test two challenger models to our control. We do that with the Shadow Deploy pipeline step.
In Shadow Deploy, the pipeline step is added with the add_shadow_deploy
method, with the champion model listed first, then an array of challenger models after. All inference data is fed to all models, with the champion results displayed in the out.variable
column, and the shadow results in the format out_{model name}.variable
. For example, since we named our challenger models housingchallenger01
and housingchallenger02
, the columns out_housingchallenger01.variable
and out_housingchallenger02.variable
have the shadow deployed model results.
For this example, we will remove the previous pipeline step, then replace it with a shadow deploy step with rf_model.onnx
as our champion, and models xgb_model.onnx
and gbr_model.onnx
as the challengers. We’ll deploy the pipeline and prepare it for sample inferences.
# Upload the challenger models
model_name_challenger01 = 'housingchallenger01'
model_file_name_challenger01 = './models/xgb_model.onnx'
model_name_challenger02 = 'housingchallenger02'
model_file_name_challenger02 = './models/gbr_model.onnx'
housing_model_challenger01 = (wl.upload_model(model_name_challenger01,
model_file_name_challenger01,
framework=Framework.ONNX)
.configure(tensor_fields=["tensor"])
)
housing_model_challenger02 = (wl.upload_model(model_name_challenger02,
model_file_name_challenger02,
framework=Framework.ONNX)
.configure(tensor_fields=["tensor"])
)
# Undeploy the pipeline
mainpipeline.undeploy()
mainpipeline.clear()
# Add the new shadow deploy step with our challenger models
mainpipeline.add_shadow_deploy(housing_model_control, [housing_model_challenger01, housing_model_challenger02])
# Deploy the pipeline with the new shadow step
#minimum deployment config
deploy_config = wallaroo.DeploymentConfigBuilder().replica_count(1).cpus(0.5).memory("1Gi").build()
mainpipeline.deploy(deployment_config = deploy_config)
Waiting for undeployment - this will take up to 45s ................................... ok
Waiting for deployment - this will take up to 45s ................................. ok
name | housepricesagapipeline |
---|---|
created | 2023-10-31 16:56:07.345831+00:00 |
last_updated | 2023-10-31 17:04:57.709641+00:00 |
deployed | True |
tags | |
versions | 44d28e3e-094f-4507-8aa1-909c1f151dd5, b9f46ba3-3f14-4e5b-989e-e0b8d166392f, f7e0deaf-63a2-491a-8ff9-e8148d3cabcb, 2ea0cc42-c955-4fc3-bac9-7a2c7c22ddc1, dca84ab2-274a-4391-95dd-a99bda7621e1 |
steps | housepricesagacontrol |
published | False |
Shadow Deploy Sample Inference
We’ll now use our same sample data for an inference to our shadow deployed pipeline, then display the first 20 results with just the comparative outputs.
shadow_result = mainpipeline.infer_from_file('./data/xtest-1k.arrow')
shadow_outputs = shadow_result.to_pandas()
display(shadow_outputs.loc[0:20,['out.variable','out_housingchallenger01.variable','out_housingchallenger02.variable']])
out.variable | out_housingchallenger01.variable | out_housingchallenger02.variable | |
---|---|---|---|
0 | [718013.7] | [659806.0] | [704901.9] |
1 | [615094.6] | [732883.5] | [695994.44] |
2 | [448627.8] | [419508.84] | [416164.8] |
3 | [758714.3] | [634028.75] | [655277.2] |
4 | [513264.66] | [427209.47] | [426854.66] |
5 | [668287.94] | [615501.9] | [632556.06] |
6 | [1004846.56] | [1139732.4] | [1100465.2] |
7 | [684577.25] | [498328.88] | [528278.06] |
8 | [727898.25] | [722664.4] | [659439.94] |
9 | [559631.06] | [525746.44] | [534331.44] |
10 | [340764.53] | [376337.06] | [377187.2] |
11 | [442168.12] | [382053.12] | [403964.3] |
12 | [630865.5] | [505608.97] | [528991.3] |
13 | [559631.06] | [603260.5] | [612201.75] |
14 | [909441.25] | [969585.44] | [893874.7] |
15 | [313096.0] | [313633.7] | [318054.94] |
16 | [404040.78] | [360413.62] | [357816.7] |
17 | [292859.44] | [316674.88] | [294034.62] |
18 | [338357.88] | [299907.47] | [323254.28] |
19 | [682284.56] | [811896.75] | [770916.6] |
20 | [583765.94] | [573618.5] | [549141.4] |
A/B Testing
A/B Testing is another method of comparing and testing models. Like shadow deploy, multiple models are compared against the champion or control models. The difference is that instead of submitting the inference data to all models, then tracking the outputs of all of the models, the inference inputs are off of a ratio and other conditions.
For this example, we’ll be using a 1:1:1 ratio with a random split between the champion model and the two challenger models. Each time an inference request is made, there is a random equal chance of any one of them being selected.
When the inference results and log entries are displayed, they include the column out._model_split
which displays:
Field | Type | Description |
---|---|---|
name | String | The model name used for the inference. |
version | String | The version of the model. |
sha | String | The sha hash of the model version. |
This is used to determine which model was used for the inference request.
# remove the shadow deploy steps
mainpipeline.clear()
# Add the a/b test step to the pipeline
mainpipeline.add_random_split([(1, housing_model_control), (1, housing_model_challenger01), (1, housing_model_challenger02)], "session_id")
mainpipeline.deploy()
# Perform sample inferences of 20 rows and display the results
ab_date_start = datetime.datetime.now()
abtesting_inputs = pd.read_json('./data/xtest-1k.df.json')
df = pd.DataFrame(columns=["model", "value"])
for index, row in abtesting_inputs.sample(20).iterrows():
result = mainpipeline.infer(row.to_frame('tensor').reset_index())
value = result.loc[0]["out.variable"]
model = json.loads(result.loc[0]["out._model_split"][0])['name']
df = df.append({'model': model, 'value': value}, ignore_index=True)
display(df)
ab_date_end = datetime.datetime.now()
ok
model | value | |
---|---|---|
0 | housingchallenger01 | [278554.44] |
1 | housingchallenger02 | [615955.3] |
2 | housepricesagacontrol | [1092273.9] |
3 | housepricesagacontrol | [683845.75] |
4 | housepricesagacontrol | [682284.56] |
5 | housepricesagacontrol | [247792.75] |
6 | housingchallenger02 | [315142.44] |
7 | housingchallenger02 | [530408.94] |
8 | housepricesagacontrol | [340764.53] |
9 | housepricesagacontrol | [421153.16] |
10 | housingchallenger01 | [395150.63] |
11 | housingchallenger02 | [544343.3] |
12 | housingchallenger01 | [395284.4] |
13 | housepricesagacontrol | [701940.7] |
14 | housepricesagacontrol | [448627.8] |
15 | housepricesagacontrol | [320863.72] |
16 | housingchallenger01 | [558485.3] |
17 | housingchallenger02 | [236329.28] |
18 | housepricesagacontrol | [559631.06] |
19 | housingchallenger01 | [281437.56] |
Model Swap
Now that we’ve completed our testing, we can swap our deployed model in the original housepricingpipeline
with one we feel works better.
We’ll start by removing the A/B Testing pipeline step, then going back to the single pipeline step with the champion model and perform a test inference.
When going from a testing step such as A/B Testing or Shadow Deploy, it is best to undeploy the pipeline, change the steps, then deploy the pipeline. In a production environment, there should be two pipelines: One for production, the other for testing models. Since this example uses one pipeline for simplicity, we will undeploy our main pipeline and reset it back to a one-step pipeline with the current champion model as our pipeline step.
Once done, we’ll perform the hot swap with the model gbr_model.onnx
, which was labeled housing_model_challenger02
in a previous step. We’ll do an inference with the same data as used with the challenger model. Note that previously, the inference through the original model returned [718013.7]
.
mainpipeline.undeploy()
# remove the shadow deploy steps
mainpipeline.clear()
mainpipeline.add_model_step(housing_model_control).deploy()
# Inference test
normal_input = pd.DataFrame.from_records({"tensor": [[4.0,
2.25,
2200.0,
11250.0,
1.5,
0.0,
0.0,
5.0,
7.0,
1300.0,
900.0,
47.6845,
-122.201,
2320.0,
10814.0,
94.0,
0.0,
0.0]]})
controlresult = mainpipeline.infer(normal_input)
display(controlresult)
ok
Waiting for deployment - this will take up to 45s ........ ok
time | in.tensor | out.variable | check_failures | |
---|---|---|---|---|
0 | 2023-10-31 17:08:19.579 | [4.0, 2.25, 2200.0, 11250.0, 1.5, 0.0, 0.0, 5.0, 7.0, 1300.0, 900.0, 47.6845, -122.201, 2320.0, 10814.0, 94.0, 0.0, 0.0] | [682284.56] | 0 |
Now we’ll “hot swap” the control model. We don’t have to deploy the pipeline - we can just swap the model out in that pipeline step and continue with only a millisecond or two lost while the swap was performed.
# Perform hot swap
mainpipeline.replace_with_model_step(0, housing_model_challenger02).deploy()
# wait a moment for the database to be updated. The swap is near instantaneous but database writes may take a moment
import time
time.sleep(15)
# inference after model swap
normal_input = pd.DataFrame.from_records({"tensor": [[4.0,
2.25,
2200.0,
11250.0,
1.5,
0.0,
0.0,
5.0,
7.0,
1300.0,
900.0,
47.6845,
-122.201,
2320.0,
10814.0,
94.0,
0.0,
0.0]]})
challengerresult = mainpipeline.infer(normal_input)
display(challengerresult)
ok
time | in.tensor | out.variable | check_failures | |
---|---|---|---|---|
0 | 2023-10-31 17:09:23.932 | [4.0, 2.25, 2200.0, 11250.0, 1.5, 0.0, 0.0, 5.0, 7.0, 1300.0, 900.0, 47.6845, -122.201, 2320.0, 10814.0, 94.0, 0.0, 0.0] | [770916.6] | 0 |
# Display the difference between the two
display(f'Original model output: {controlresult.loc[0]["out.variable"]}')
display(f'Hot swapped model output: {challengerresult.loc[0]["out.variable"]}')
'Original model output: [682284.56]'
‘Hot swapped model output: [770916.6]’
Undeploy Main Pipeline
With the examples and tutorial complete, we will undeploy the main pipeline and return the resources back to the Wallaroo instance.
mainpipeline.undeploy()
Waiting for undeployment - this will take up to 45s ...................................... ok
name | housepricesagapipeline |
---|---|
created | 2023-10-31 16:56:07.345831+00:00 |
last_updated | 2023-10-31 17:09:08.758964+00:00 |
deployed | False |
tags | |
versions | 51034ba5-b58e-4475-908d-ec8fae069745, cd0f20e9-6923-4e6f-8114-1137676da5c5, 58274aab-0045-4557-a740-7d085af8574d, 4cf4f0dc-2c42-4f8b-975c-1f5c5d939a98, c5ae5a56-1c7f-4a94-9d4e-f08bf35e7f4f, f652a9d8-a4f6-428a-9b36-b29eec6b5198, 0e7d9d77-02d7-4c13-8062-9c77492145f8, 42b97784-fc16-43be-b89e-2d3dad20dd2b, 7d35285a-f8af-4bed-855b-60258c3435ee, 8b0dc4bb-3340-44f9-85d6-fbd091e19412, 06169fed-59e9-41f8-9dbd-0fc9f80ebb73, 44d28e3e-094f-4507-8aa1-909c1f151dd5, b9f46ba3-3f14-4e5b-989e-e0b8d166392f, f7e0deaf-63a2-491a-8ff9-e8148d3cabcb, 2ea0cc42-c955-4fc3-bac9-7a2c7c22ddc1, dca84ab2-274a-4391-95dd-a99bda7621e1 |
steps | housepricesagacontrol |
published | False |